Builder Design Pattern Explained in 10 Minutes
Summary
TLDRThe Builder pattern is a design approach that decouples the construction of a complex object from its representation. It allows for the creation of different object representations through a step-by-step building process. The pattern introduces a Builder class that handles the assembly of object parts and a Director class that manages the construction process. This separation simplifies the creation of complex objects and makes the system more maintainable and scalable.
Takeaways
- 🏠 The Builder pattern is designed to separate the construction of a complex object from its representation, allowing for more flexible and manageable object creation.
- 🔍 An object's representation refers to the specific values of its fields, which can vary widely, creating different instances of the same class.
- 🛠️ The construction of an object involves the process of creating an instance of that object, which is traditionally done through a class's constructor method.
- 🔑 The Builder pattern introduces a Builder class that has methods to set the fields of the object and a 'build' method to create the final object.
- 👷♂️ The Builder class is responsible for the construction of the object, while the actual class (e.g., House) is responsible for its representation.
- 🏡 The House class's constructor is modified to take a Builder instance as an argument, facilitating the separation of construction and representation.
- 🔄 The Builder pattern allows for step-by-step construction of an object, where each step sets a different aspect of the object before the final build.
- 👨🏫 The Director class can be used to manage the building process, encapsulating the logic for creating different types of objects and making the usage code cleaner.
- 🔄 The pattern supports the creation of multiple objects with different specifications without cluttering the client code with repetitive build steps.
- 🔧 The Builder pattern provides a flexible approach to object construction, making it easier to add new types of objects or change the construction process without altering the client code.
Q & A
What is the main goal of the Builder pattern?
-The main goal of the Builder pattern is to separate the construction of an object from its representation, allowing for more flexible and organized object creation.
What is the difference between an object's representation and its construction?
-An object's representation refers to the state or configuration of its fields, such as the number of stories, door type, and roof type in a House class. The construction, on the other hand, is the process of creating an instance of the object, often through a constructor method.
Why is it beneficial to separate the construction from the representation in object-oriented programming?
-Separating construction from representation allows for more flexibility in object creation. It enables the same construction process to produce different representations and makes the code more maintainable and scalable.
How does the Builder pattern help in managing complex object creation?
-The Builder pattern manages complex object creation by providing a step-by-step building interface through the Builder class, which allows setting various properties independently before constructing the final object.
What is the role of the 'Builder' in the Builder pattern?
-In the Builder pattern, the 'Builder' is responsible for assembling the parts of the complex object. It provides methods to set different properties and a 'build' method that constructs the final object.
Can you explain the concept of a 'Director' in the context of the Builder pattern?
-The 'Director' in the Builder pattern is a class that manages the construction process. It works with a Builder object to construct objects according to specific requirements or specifications, providing a way to encapsulate the construction logic.
Why might a developer choose to use the Builder pattern over other creational patterns?
-A developer might choose the Builder pattern over other creational patterns when dealing with complex objects that have multiple configurations or representations. It provides a clear separation of construction and representation and allows for more controlled object creation.
How does the Builder pattern improve the readability and maintainability of code?
-The Builder pattern improves code readability and maintainability by separating the construction logic from the client code. It encapsulates the construction details within the Builder and Director, making the client code cleaner and easier to understand.
What are some potential downsides to using the Builder pattern?
-Potential downsides of using the Builder pattern include increased complexity in the codebase due to the introduction of additional classes, and the overhead of creating a Builder for every complex object, which might be unnecessary for simpler objects.
Can you provide an example of how the Builder pattern might be implemented in a real-world scenario?
-In a real-world scenario, the Builder pattern could be used to construct a 'Car' object with various configurations. A CarBuilder class would handle the setting of features like engine type, body style, and interior options, and a Director class could manage the creation process for different car models.
Outlines
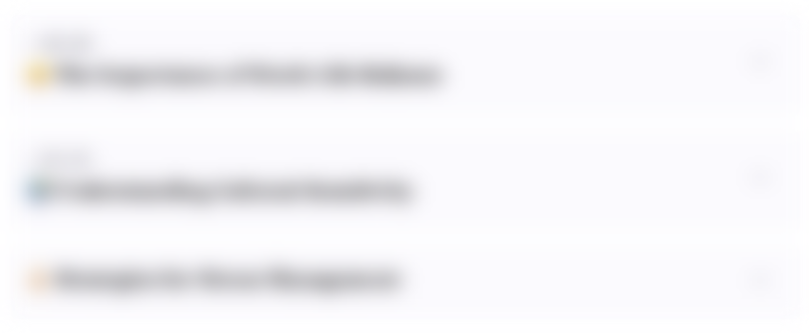
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
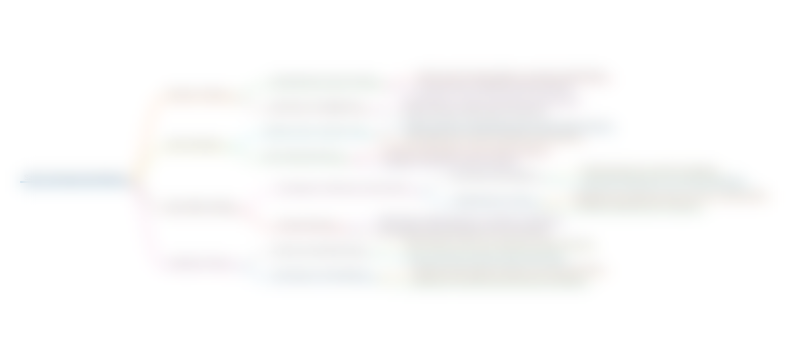
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
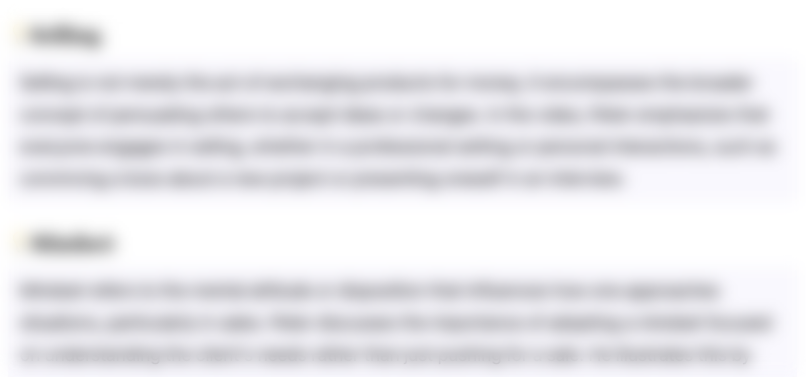
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
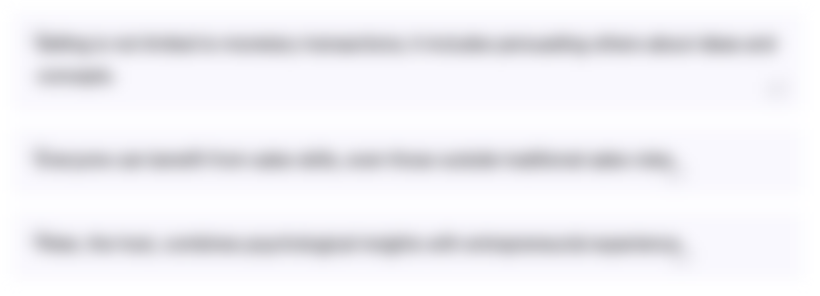
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
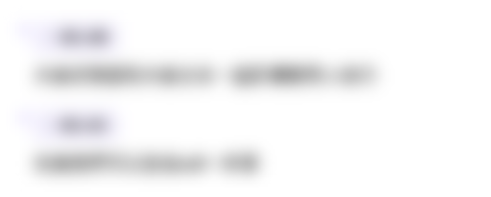
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
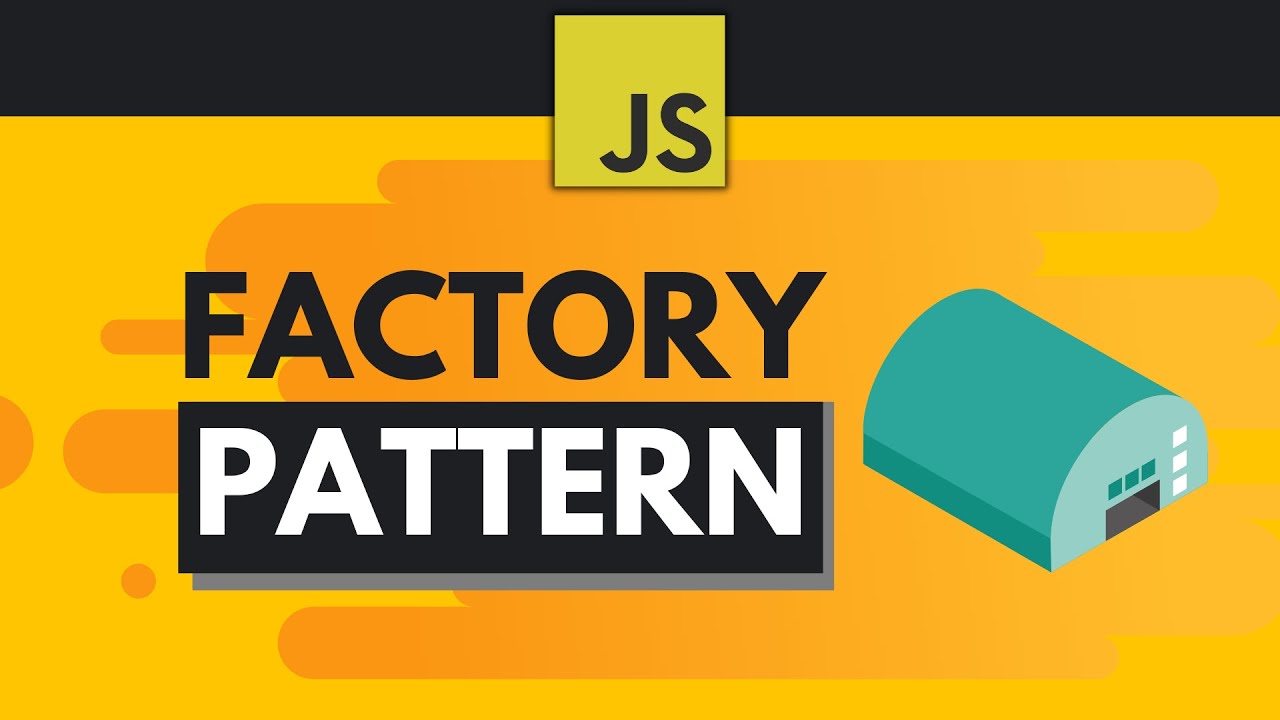
Javascript Design Patterns #1 - Factory Pattern

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
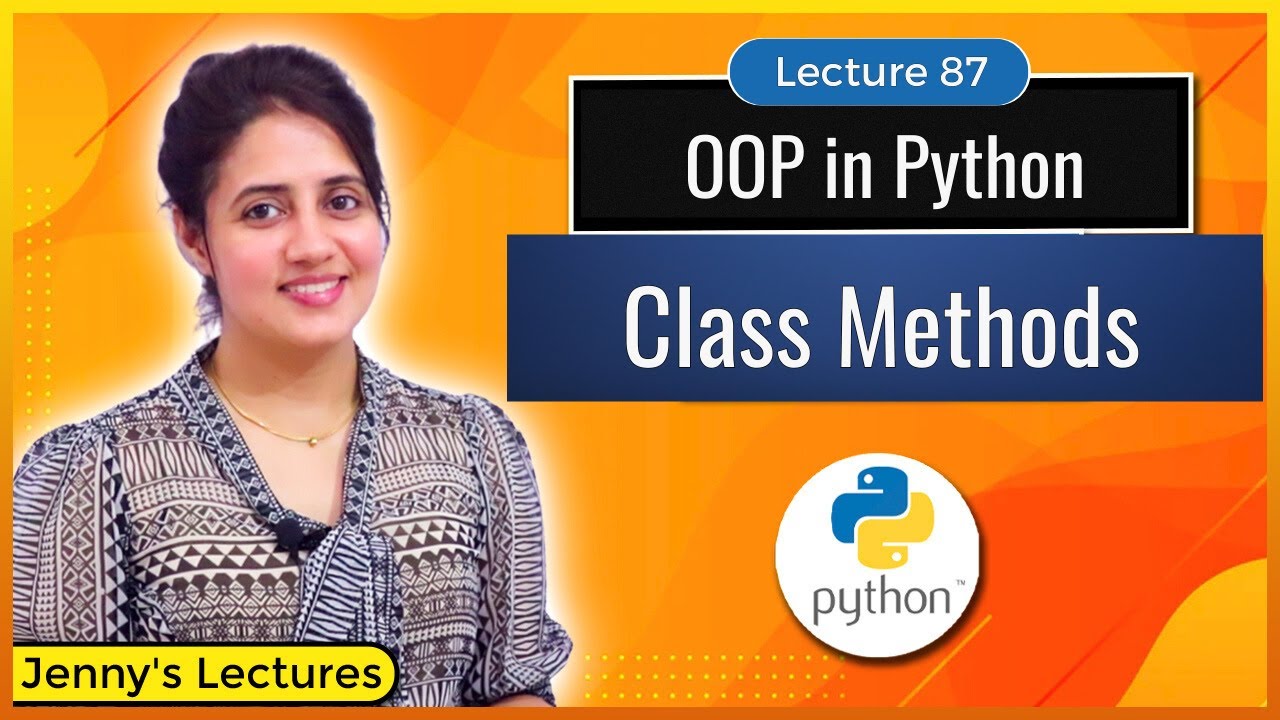
Class Methods in Python | How to add Methods in Class | Python Tutorials for Beginners #lec87
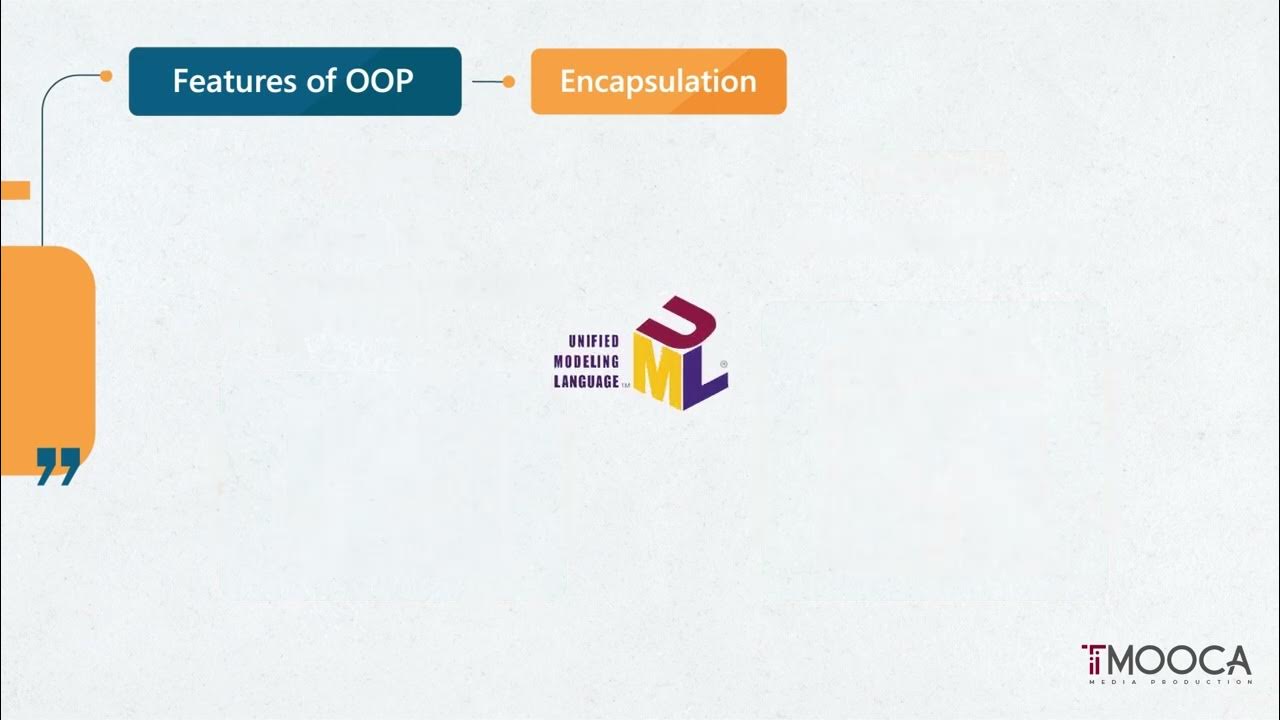
CH01_VID02_Encapsulation

Factory Pattern
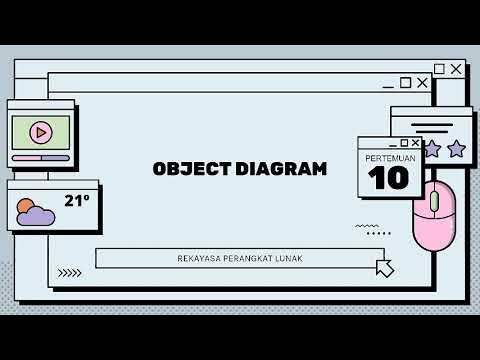
LANGKAH MUDAH MEMBUAT OBJECT DIAGRAM (AKU JADI PAHAM BEDANYA CLASS DAN OBJEK !!)
5.0 / 5 (0 votes)