Immediately Invoked Function Expressions (IIFE) | JavaScript 🔥 | Lecture 127
Summary
TLDRThe video script delves into Immediately Invoked Function Expressions (IIFEs), a JavaScript pattern that allows executing a function immediately after its definition. IIFEs create a private scope, enabling data encapsulation and preventing accidental variable overwrites. While IIFEs were historically used for this purpose, modern JavaScript allows creating blocks with `let` and `const` for data privacy, reducing the need for IIFEs. However, IIFEs remain useful when a function needs to execute only once, as they serve that purpose elegantly. The script explores the rationale, syntax, and use cases of IIFEs, providing a comprehensive understanding of this intriguing JavaScript concept.
Takeaways
- 👉 Immediately Invoked Function Expressions (IIFEs) are functions that are executed immediately after being defined, without needing to be called later.
- 🔑 IIFEs are useful when you need a function to run only once and then disappear, for example, with async/await.
- 🌐 IIFEs create a new scope, allowing data encapsulation and privacy, which are important concepts in programming.
- 🔒 Data encapsulation and privacy help protect variables from being accidentally overwritten by other parts of the program or external scripts/libraries.
- 🆚 In modern JavaScript, using blocks with let or const can achieve data privacy without the need for IIFEs, unless you need to use var.
- ➡️ IIFEs are still useful if you specifically need to execute a function just once, even with modern JavaScript.
- 📝 IIFEs can be created with a normal function expression or an arrow function expression, wrapped in parentheses and immediately invoked.
- 🔄 While IIFEs were a popular pattern in older JavaScript, they are not as commonly used now due to the availability of blocks and let/const.
- 🧠 Understanding scopes and data encapsulation is crucial for writing clean and maintainable code.
- 🚀 IIFEs provide a way to execute a function immediately while also creating a new scope for data privacy and encapsulation.
Q & A
What is an Immediately Invoked Function Expression (IIFE) in JavaScript?
-An IIFE is a JavaScript function that runs as soon as it is defined. It is a design pattern used to execute a function immediately without saving it or having the ability to execute it again.
Why might we need to use an IIFE in JavaScript?
-We might use an IIFE for code that only needs to run once and then never again, such as setting up an initial state, or to immediately execute setup code in a scope that doesn't pollute the global namespace.
How do you create an IIFE?
-You create an IIFE by writing a function expression and immediately invoking it. This is done by wrapping the function in parentheses and adding another pair of parentheses at the end to call it.
Why does wrapping a function in parentheses turn it into an expression in JavaScript?
-Wrapping a function in parentheses in JavaScript tricks the parser into treating it as an expression rather than a statement. This allows the function to be immediately invoked without throwing an error.
Can arrow functions be used as IIFEs?
-Yes, arrow functions can also be used as IIFEs. The syntax is similar to using a regular function expression, where the arrow function is enclosed in parentheses and immediately invoked.
What is the purpose of using IIFEs for variable scope?
-IIFEs are used to create a new scope for variables, ensuring they are encapsulated and private within the function. This prevents the variables from being accessible or overwritten from the outside scope, enhancing data privacy and security.
How have ES6 features like let and const changed the use of IIFEs for scoping?
-With ES6, the introduction of `let` and `const` allows for block scoping, which reduces the need for IIFEs to create new scopes for data privacy. Variables declared with `let` or `const` are confined to the block scope, not requiring an IIFE for the same purpose.
Are IIFEs still relevant in modern JavaScript development?
-While the need for IIFEs for scoping has diminished with ES6 block scoping, they remain relevant for executing functions exactly once, especially in situations where an isolated scope is necessary without polluting the global namespace.
What is data encapsulation, and how do IIFEs contribute to it?
-Data encapsulation is a principle of hiding the internal state and functionality of a component, only exposing what is necessary. IIFEs contribute to data encapsulation by creating a private scope for variables and functions, preventing external access or modification.
Why is it important to hide variables using scopes in JavaScript?
-Hiding variables using scopes is important to prevent them from being accidentally overwritten by other parts of the program or external scripts. It ensures data privacy and integrity, and is a key aspect of secure and reliable programming practices.
Outlines
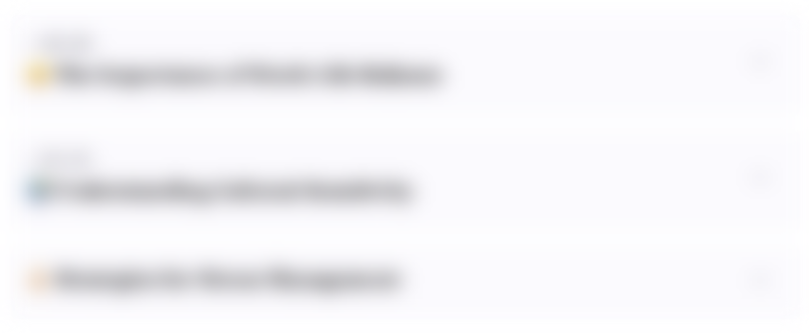
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
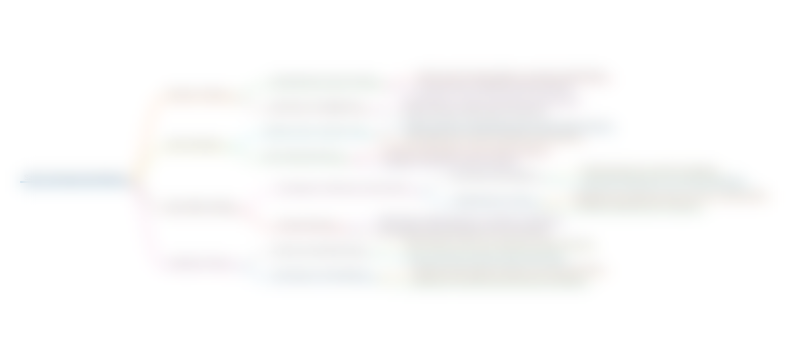
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
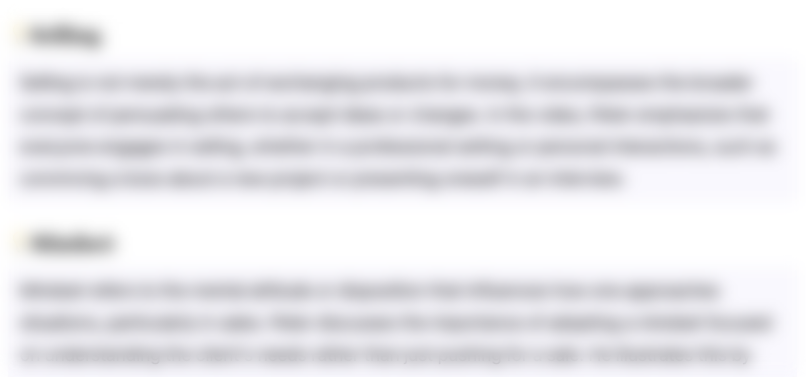
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
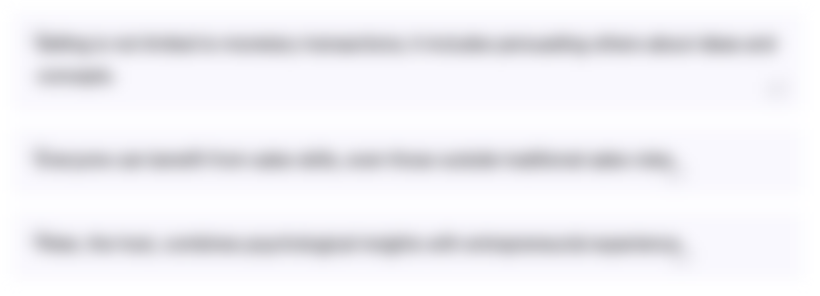
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
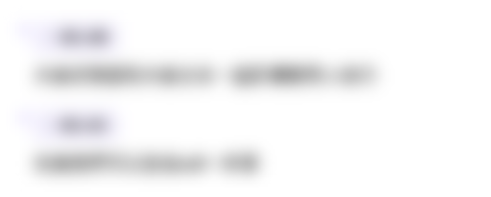
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
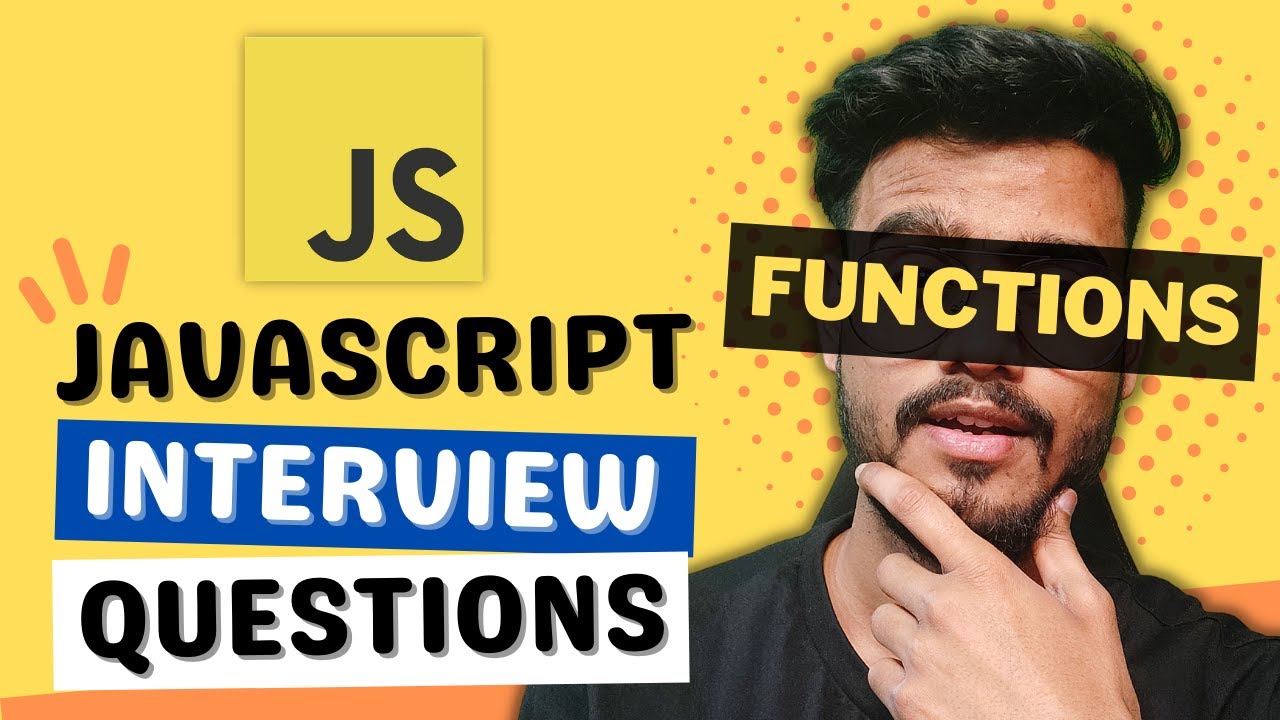
Javascript Interview Questions ( Functions ) - Hoisting, Scope, Callback, Arrow Functions etc

The bind Method | JavaScript 🔥 | Lecture 125

Learn Closures In 7 Minutes

Hoisting in JavaScript 🔥(variables & functions) | Namaste JavaScript Ep. 3

La diferencia de usar forEach() y map() en javascript #es6

More Closure Examples | JavaScript 🔥 | Lecture 129
5.0 / 5 (0 votes)