The bind Method | JavaScript 🔥 | Lecture 125
Summary
TLDRThis video tutorial delves into JavaScript's bind method, which, unlike the call method, does not immediately invoke a function but returns a new function with 'this' keyword bound to a specified value. Using an airline booking example, it demonstrates how bind can simplify repetitive function calls by presetting 'this' to Eurowings, enabling easy flight bookings across different airlines. The video further explores partial application, showing how bind can predefine certain arguments for functions, such as specific flight numbers, thereby simplifying the function's usage. Additionally, it touches on the use of bind with event listeners and introduces the concept of creating functions that return other functions for advanced use cases.
Takeaways
- 🔑 The bind() method allows you to set the 'this' keyword for a function and returns a new function with that 'this' value bound.
- ➡️ Unlike call(), bind() does not immediately invoke the function; instead, it returns a new function with 'this' bound to the provided value.
- 🛫 bind() can be used to create function shortcuts for specific objects, like bookEW for Eurowings airline.
- 📋 With bind(), you can also pre-set arguments for a function, a technique known as 'partial application'.
- ⚡ bind() is especially useful when working with event listeners, where 'this' refers to the element the listener is attached to.
- 🔄 By binding the correct 'this' value, you can ensure methods have the intended context when called in event listeners.
- 🧩 Partial application allows you to create specialized functions from more general ones by pre-setting some arguments.
- 🏭 bind() can be used for partial application even when you don't care about the 'this' value (by passing null).
- 🔄 Returning a function from another function is an alternative technique to achieve partial application without using bind().
- 🚀 Mastering bind() and partial application unlocks powerful ways to create specialized, context-aware functions in JavaScript.
Q & A
What is the main difference between the bind and call methods in JavaScript?
-The main difference is that the call method immediately invokes the function with a specified this value and arguments, while the bind method returns a new function with a predefined this value and can include predefined arguments.
What is the purpose of using the bind method in JavaScript?
-The bind method is used to create a new function where the this keyword is set to a specific value, and it can also predefine arguments for the function. This is useful for setting the context of 'this' and for partial application of function parameters.
How does the bind method work with arguments in JavaScript?
-The bind method allows you to not only set the this value but also to preset arguments for the function. These preset arguments are fixed when the new function is created, and the function will always be called with these arguments.
Can you explain partial application with an example from the script?
-Partial application is when some arguments of a function are preset. For example, creating a bookEW23 function that is a specific instance of a book function with the flight number 23 preset. This results in a new function that only requires the passenger name as an argument.
Why does the script use bind in the context of an event listener?
-The script uses bind in an event listener to set the this keyword to a specific object (Lufthansa in the example) instead of the default this value (the button element), ensuring the function uses the correct context when invoked as an event handler.
How is the bind method used to solve the 'this' keyword issue in event listeners?
-Bind is used to return a new function with the this keyword set to the desired object. In event listeners, this ensures the function called as the event handler references the correct object instead of the DOM element that triggered the event.
What is the significance of null in the addTax.bind call in the script?
-Null is used as the first argument in the addTax.bind call because the function does not use the this keyword. It's a convention to pass null when the this value is irrelevant, allowing the focus to be on presetting the subsequent arguments.
How does the script demonstrate the use of bind for simplifying repetitive tasks?
-The script shows that by using bind to create new functions with preset parameters, such as airline or flight number, repetitive tasks like booking for specific flights or airlines can be simplified, avoiding the need to repeatedly set these parameters.
What is the concept of partial application as demonstrated in the script?
-Partial application, as demonstrated, involves creating a new function by presetting some of the original function's arguments, such as setting the flight number or tax rate in advance. This results in a function that requires fewer arguments in future calls.
How does the script illustrate the flexibility and power of the bind method in function customization?
-The script illustrates the flexibility and power of bind by showing how it can create more specific versions of a general function through partial application, such as presetting the flight number or tax rate, making the function simpler and tailored for specific uses.
Outlines
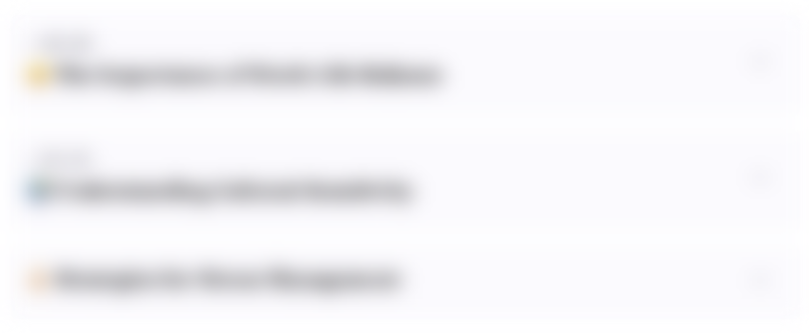
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
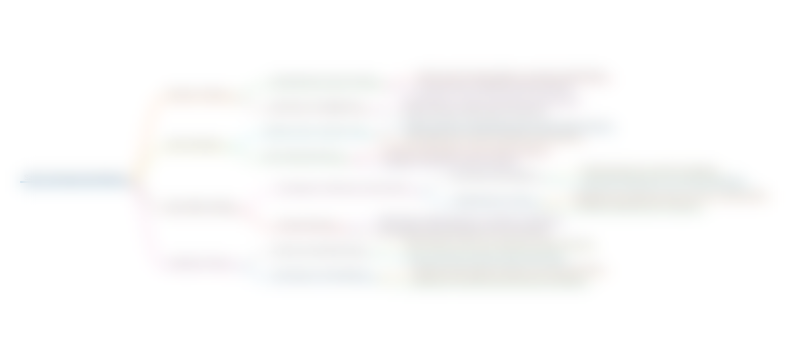
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
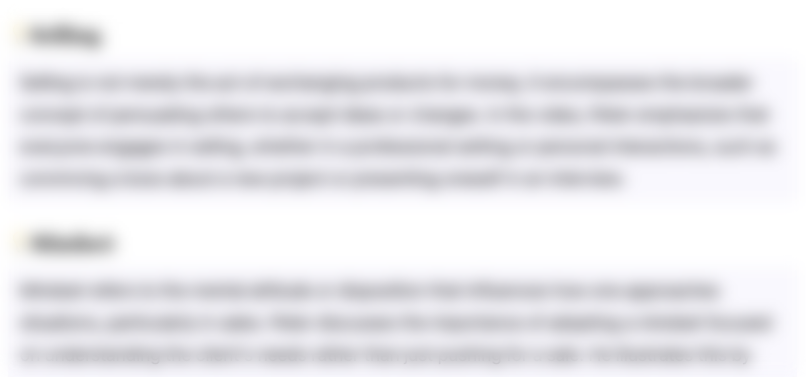
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
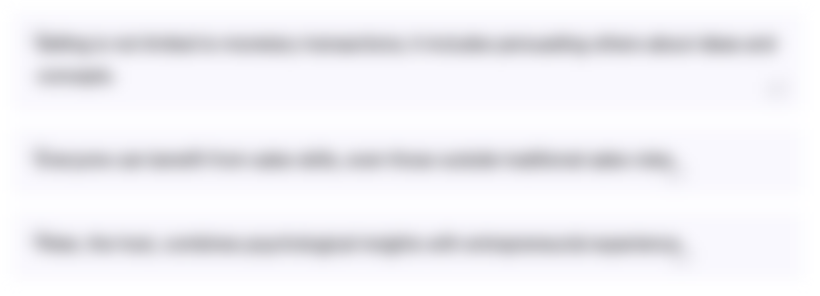
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
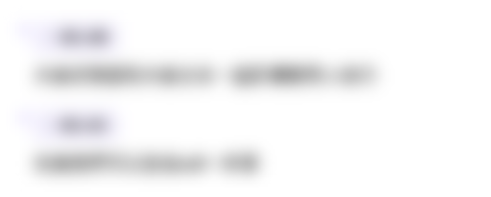
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)