Collision Detection - How to Make a 2D Game in Java #6
Summary
TLDRIn this tutorial, RyiSnow demonstrates how to implement collision detection in a game development project. The video guides through setting solid tiles in the TileManager, adjusting player collision areas using a Rectangle class, and creating a CollisionChecker class to prevent the player from walking through obstacles. RyiSnow also discusses the importance of precise collision settings for better game mechanics and player movement control, concluding with a preview of upcoming features like object creation and interaction.
Takeaways
- 🌏 The video is a tutorial on implementing collision detection in a game world map.
- 🛠️ The 'Tile' class has a 'collision' boolean that is used to define solid tiles like walls and water.
- 🎮 Player movement is restricted by setting the collision property to 'true' for solid tiles to prevent walking through them.
- 📏 Collision settings are applied to the player character by defining a 'solidArea' within the player's tile.
- 🔍 The 'Rectangle' class is used to create a collision area for the player character with specified x, y, width, and height.
- 👾 The 'solidArea' is made smaller than the player's tile to allow for better movement and control in the game.
- 🚫 Collision detection prevents the player from moving into a solid tile, enhancing game realism and mechanics.
- 🔄 A 'CollisionChecker' class is created to handle collision checks for the player, monsters, and NPCs.
- 📚 The 'GamePanel' class is used to instantiate the 'CollisionChecker' and integrate it with the game's update method.
- 🧩 By calculating the player's 'worldX' and 'worldY' based on the 'solidArea', the game checks for collisions in four directions.
- 🛑 The tutorial concludes with the successful implementation of collision detection, with plans to create and display game objects in the next video.
Q & A
What is the main topic of the video?
-The main topic of the video is implementing collision detection in a game development program to prevent the player from walking through solid objects like trees and water.
What is the purpose of the 'collision' boolean in the Tile class?
-The 'collision' boolean in the Tile class is used to designate whether a tile is solid or not, thus preventing the player from walking through it.
Why is it important to set only specific parts of the player character as solid?
-Setting only specific parts of the player character as solid helps to avoid issues like getting stuck on walls and improves the control mechanics, allowing for more precise movement through narrow paths.
What is the Rectangle class used for in the context of collision detection?
-The Rectangle class is used to create an invisible or abstract rectangle that defines the solid area of the player character for collision detection purposes.
What is the default value of the 'collisionOn' boolean?
-The default value of the 'collisionOn' boolean is false, meaning that by default, tiles are not considered solid for collision detection.
How does the video script suggest determining the solid area for the player character?
-The script suggests using the Rectangle class to define a specific area of the player character as solid, adjusting the x, y, width, and height parameters to fit the desired solid area.
What is the CollisionChecker class responsible for?
-The CollisionChecker class is responsible for checking collisions between entities, such as the player, monsters, and NPCs, and the game world's tiles.
Why is it necessary to check the worldX and worldY of the player's solidArea for collision detection?
-The worldX and worldY of the player's solidArea need to be checked because collision detection must be based on the coordinates of the solid part of the player character, not the player's overall position.
How does the script handle checking collisions in different directions?
-The script uses a switch statement to handle different directions, calculating the predicted position of the player after movement and checking the tiles that the player's solidArea would collide with in that direction.
What is the next step mentioned in the video script after implementing collision detection?
-The next step mentioned in the video script is to create objects and display them on the screen for the player to interact with, such as picking them up.
Outlines
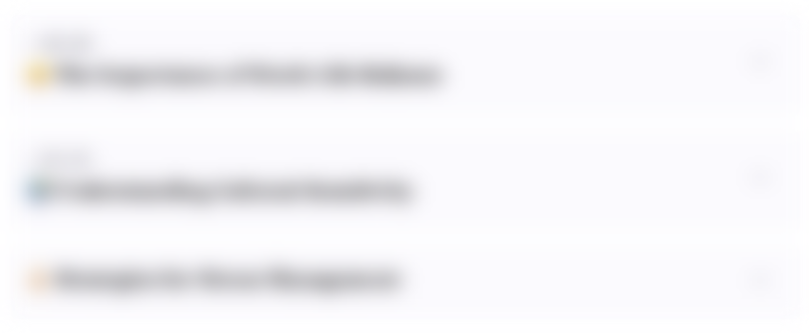
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
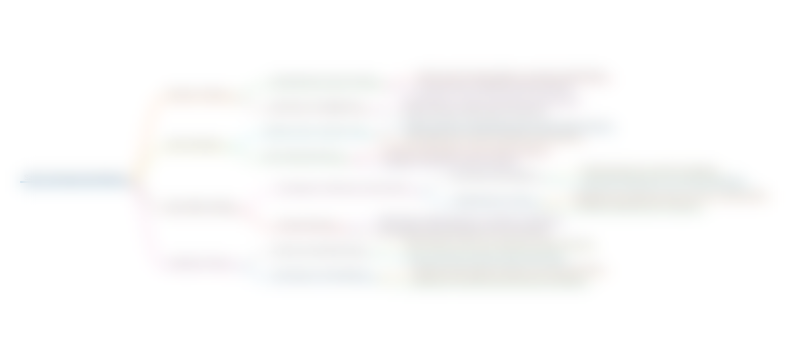
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
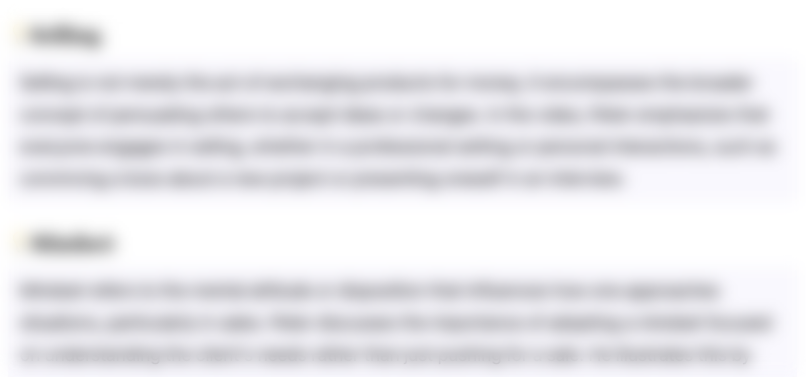
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
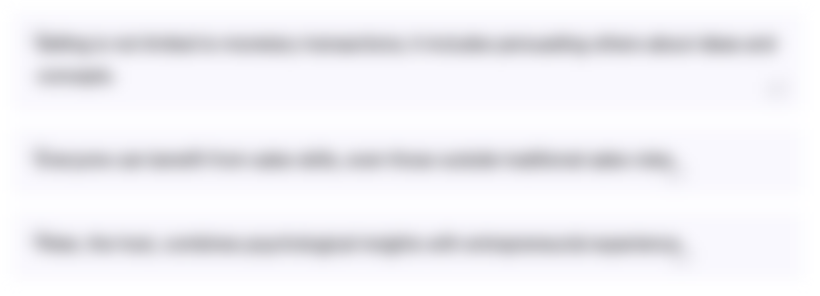
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
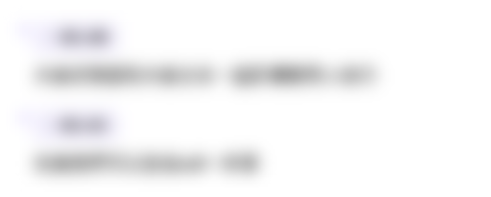
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
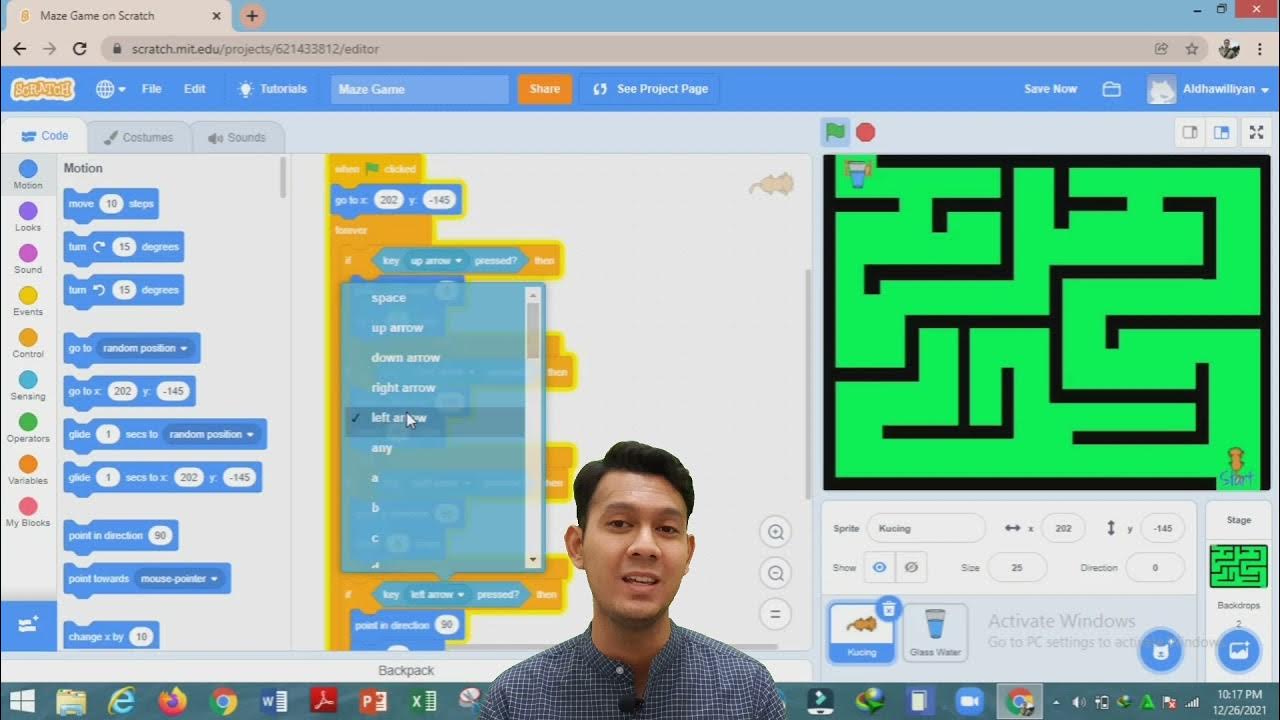
TUTORIAL MEMBUAT MAZE GAME DENGAN PROGRAM SCRATCH
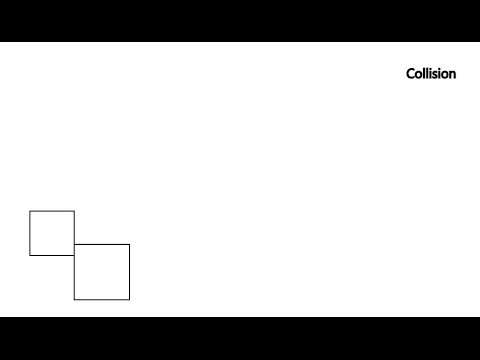
Game Dev: Collision Detection - Bounding Box
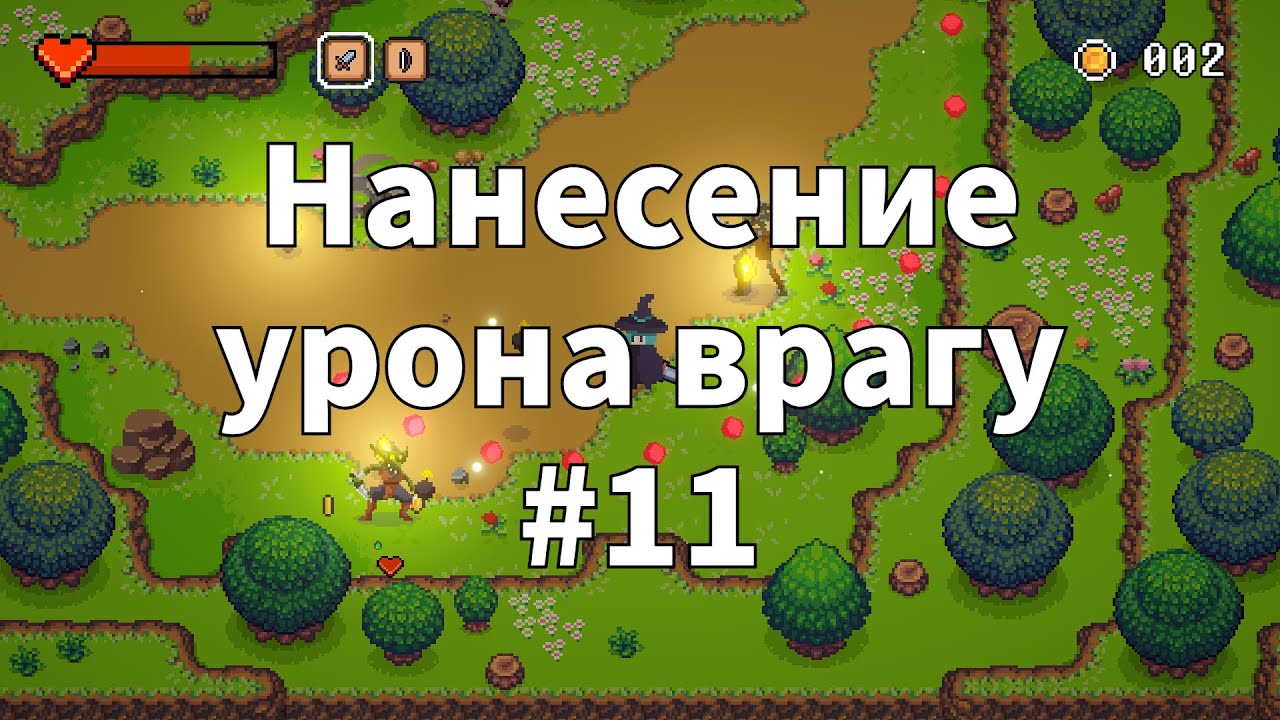
2D Top Down игра на Unity с нуля #11 | Коллайдер для меча, нанесение урона врагу
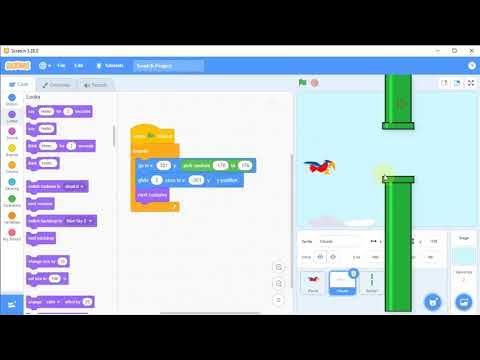
Tutorial Membuat Game Flappy Bird di Scratch, #3 Kelas 5 SD
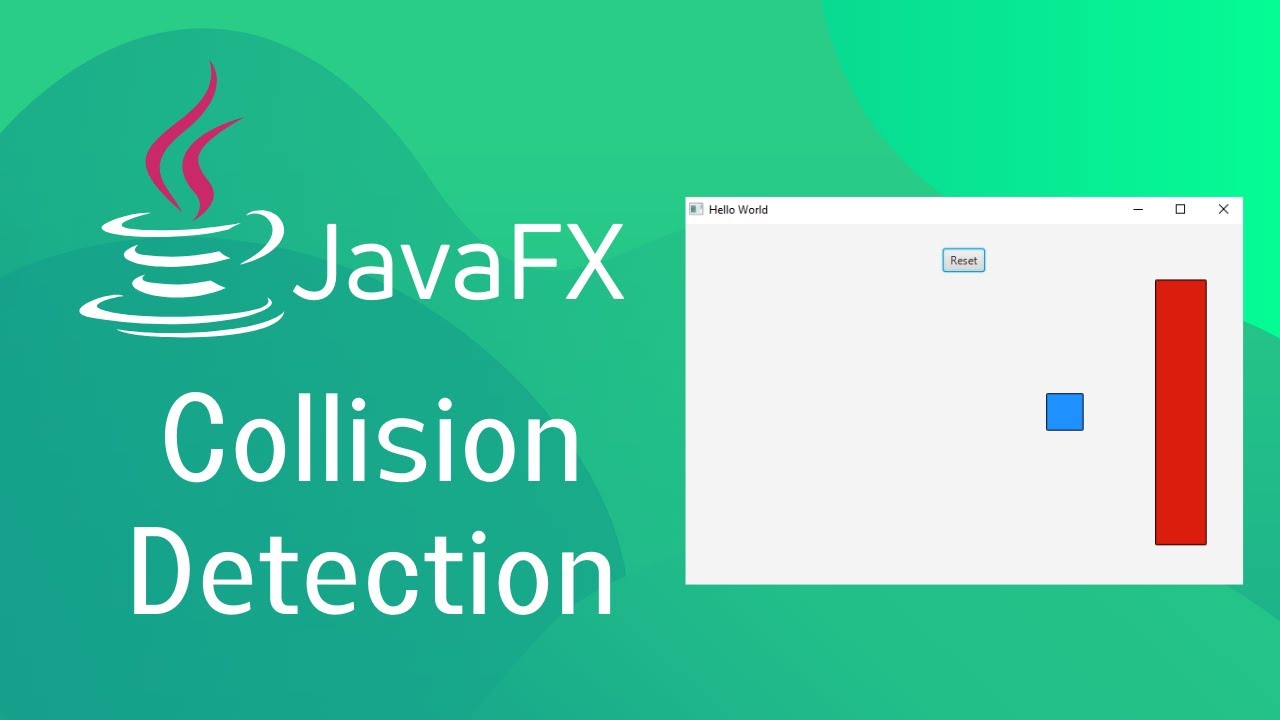
JavaFX and Scene Builder - Simple Collision Detection setup
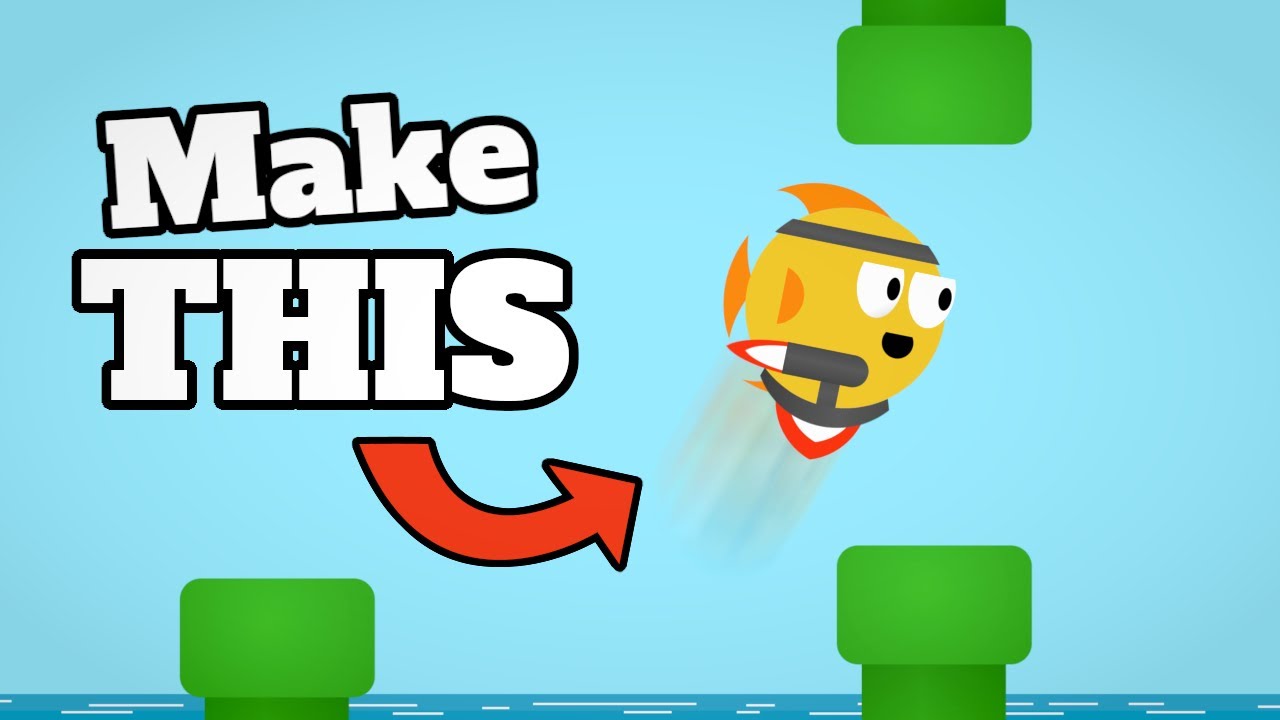
CREATE a Flappy Bird Game in Unity with CLEAN CODE Like a PRO!
5.0 / 5 (0 votes)