Merge Sorted Array - Leetcode 88 - Python
Summary
TLDRIn this coding tutorial, the presenter explores an efficient way to merge two sorted arrays into one without using additional memory. The focus is on merging 'nums1' and 'nums2' by starting from the end of 'nums1', leveraging the guaranteed space. The method involves using pointers to compare and insert the larger value from either array, then adjusting the pointers accordingly. An edge case is addressed where the remaining elements in 'nums2' are directly copied to 'nums1'. The script concludes with a concise code implementation and a reminder to consider zero-based indexing.
Takeaways
- ๐ The video discusses a coding challenge to merge two sorted arrays, nums1 and nums2, into a single sorted array within nums1.
- ๐ A lazy approach is suggested to create a new array and merge the elements by comparing the smallest values, but this requires extra memory.
- ๐ The optimal solution is to merge the arrays without a temporary array, aiming for a memory complexity of O(1).
- ๐ The merging process should start from the end of nums1, taking advantage of the guaranteed space for nums2's elements.
- ๐ Initialize a pointer at the end of nums1 to begin the merge, comparing the last elements of nums1 and nums2 to determine the largest value to insert.
- ๐ Update pointers for nums1 and nums2 as elements are compared and merged, always choosing the larger value to place in nums1.
- ๐ก Handle edge cases where the smallest value is not in the desired position by adjusting the pointers and merging accordingly.
- ๐ After merging, any remaining elements in nums2 should be directly copied to nums1 since they are already sorted.
- ๐ The final code provided is intended to be readable and beginner-friendly, with a focus on avoiding extra memory usage.
- ๐ A potential bug is identified related to index starting at zero, which is corrected in the script to ensure the code works correctly.
- ๐ The video encourages viewers to like, subscribe, and support the channel for more content.
Q & A
What is the primary task described in the script?
-The primary task is to merge two input arrays, nums1 and nums2, into a single sorted array, with nums1 having enough space to accommodate all elements from nums2.
Why might someone choose to create a new array instead of merging into nums1?
-Creating a new array is a lazy approach to avoid the complexity of merging into nums1. It simplifies the process by starting with an empty array and filling it with the smallest elements from both arrays.
What is the time complexity of the lazy approach mentioned?
-The time complexity of the lazy approach is O(n), where n is the total number of elements in both arrays.
What is the main disadvantage of the lazy approach in terms of memory usage?
-The main disadvantage is the need for extra memory due to the creation of a temporary array to hold the merged elements before copying them back to nums1.
Why is it beneficial to start merging from the end of nums1 instead of the beginning?
-Starting from the end of nums1 is beneficial because nums1 already has enough empty space at the end to accommodate all elements from nums2, making the merging process more efficient.
What is the strategy for finding the largest value to insert at the end of nums1?
-The strategy involves comparing the last elements of nums1 and nums2 and selecting the larger one to insert at the end of nums1, then moving the corresponding pointer in that array.
How does the script handle the case when all elements from nums2 have been merged?
-When all elements from nums2 have been merged, the remaining elements in nums1 that have not been replaced are left as they are, since they are already in the correct position.
What is the edge case that the script mentions and how is it handled?
-The edge case is when the smallest value is not in the desired position initially. In this case, the script suggests taking all remaining elements in nums2 and filling nums1 with them since they are already in sorted order.
What is the condition for filling nums1 with the leftover elements from nums2?
-The condition for filling nums1 with leftover elements from nums2 is when there are leftover elements in nums2 and the pointer n is greater than zero.
How does the script ensure the final result is stored in nums1 without extra memory?
-The script ensures the result is stored in nums1 by merging elements in reverse order and updating pointers accordingly, thus avoiding the need for a temporary array.
What is the potential bug mentioned in the script and how is it fixed?
-The potential bug is related to forgetting that indexes start at zero, which could lead to incorrect pointer decrements. The script fixes this by properly adjusting the index decrements.
Outlines
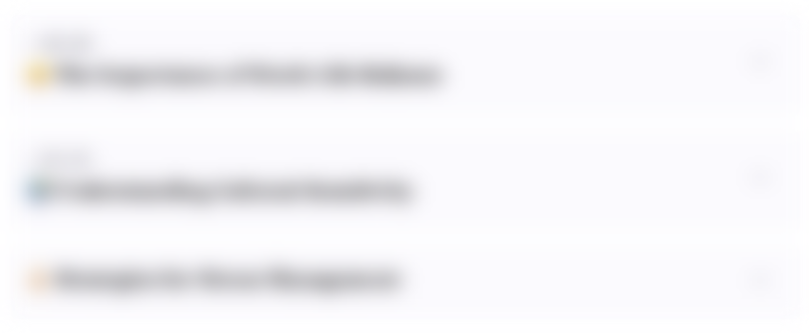
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
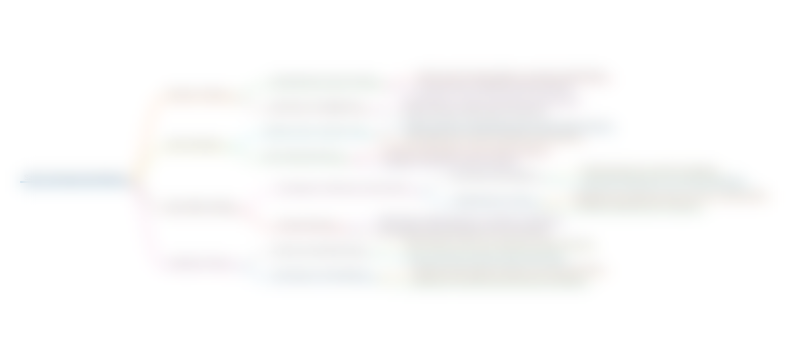
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
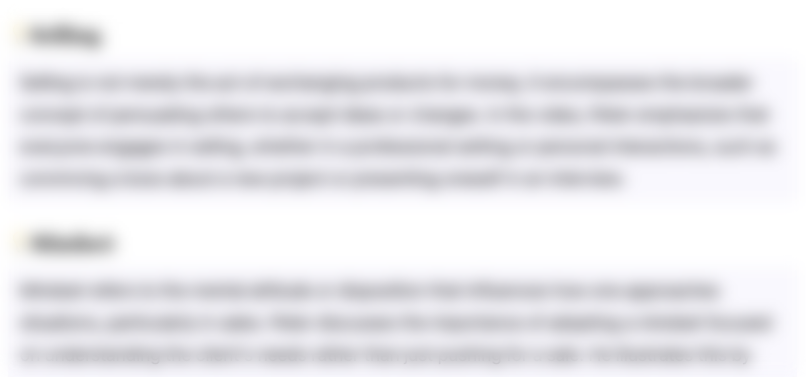
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
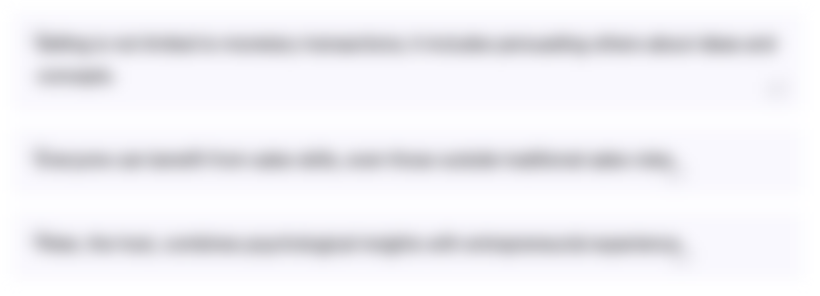
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
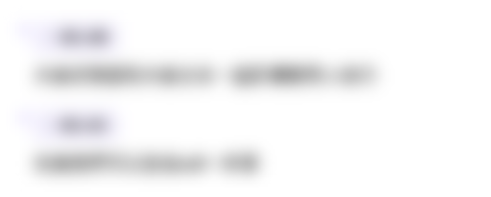
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)