java microservice telephonic interview of 10 years experienced
Summary
TLDRIn this interview, the candidate discusses their experience with microservices architecture and communication methods, including REST and Kafka. They address handling high-throughput scenarios and Java-related topics such as version differences, exceptions, and multi-threading. The candidate also explains key concepts in Java 7 and Java 8, including Comparable vs. Comparator and stream API. They touch on service discovery, service registry, and Spring Boot beans. The interview concludes with a coding challenge to check for anagrams, showcasing the candidate's logical approach to problem-solving.
Takeaways
- π Introduction and current roles and experiences discussed.
- π» Discussion about microservices architecture versus monolithic services.
- π Explanation of service communication using REST template for updates.
- π§ Query about the setup and handling of Kafka producer-consumer throughput.
- π Differences between Java 7 Comparable and Comparator explained.
- π€ Contract between equals and hash code methods in Java discussed.
- π Explanation of starvation and deadlock in multi-threading environments.
- βοΈ Difference between compile-time and runtime exceptions in Java.
- π« Explanation of exception handling using throw and throws keywords.
- π Differences between HashMap, HashTable, and ConcurrentHashMap and their use cases.
- π Discussion about the use of streams in Java 8 and their advantages over iterators.
- π Use of service discovery and service registry in applications explained.
- π Task to check if two strings are anagrams by comparing character counts.
- π« Explanation of the concept of a bean in Spring Boot.
Q & A
What are your current roles and how much experience do you have?
-The interviewee is involved in doing both reviews and credit card fixing at a call center. They have experience with these tasks and are proficient in both.
Are you using a microservice architecture or monolithic services?
-The interviewee is using microservice architecture.
Can you explain how service communication happens in your current project?
-The service communication depends on the type of call. For synchronous calls, they use REST with a REST template for updates and parameters. For asynchronous communication, they use Kafka for event-based messaging.
How would you handle a scenario where your Kafka producer is producing 100 records per second but the consumer is consuming only 20 records per second?
-In this scenario, the interviewee would handle the situation by increasing the throughput, likely by adding more consumers or optimizing the existing consumer logic.
What is the difference between Comparable and Comparator in Java?
-Comparable has a single method, compareTo, used to define the natural ordering of objects. Comparator, on the other hand, allows multiple comparison methods to define different orderings, often implemented as a functional interface in Java 8 and above.
What is the contract between the equals and hashCode methods in Java?
-The contract between equals and hashCode is that if two objects are equal according to the equals method, they must have the same hashCode. However, it is not required for two objects with the same hashCode to be equal according to the equals method.
What is the difference between starvation and deadlock in a multithreading environment?
-Starvation occurs when a thread is perpetually denied access to resources, while deadlock is a situation where two or more threads are blocked forever, each waiting on the other to release a resource.
What are some ways to prevent deadlock in a multithreading environment?
-To prevent deadlock, one can use techniques like ordering resource acquisition, using a timeout for resource requests, and employing deadlock detection algorithms.
What is the difference between a compile-time exception and a runtime exception in Java?
-Compile-time exceptions, also known as checked exceptions, must be handled with try-catch blocks or declared with the throws keyword. Runtime exceptions, or unchecked exceptions, occur during program execution and do not need to be explicitly handled.
What is the difference between the throw and throws keywords in Java?
-The throw keyword is used to explicitly throw an exception within a method. The throws keyword is used in a method's signature to declare that the method can throw exceptions of the specified types.
What will happen if a method in a parent class throws a NullPointerException and a method in a child class throws an Exception?
-The code will not compile because the child class method cannot throw a broader exception than the parent class method. The exceptions thrown by the child method must be the same or a subclass of the exceptions thrown by the parent method.
What are the differences between HashMap, Hashtable, and ConcurrentHashMap in Java?
-HashMap is not synchronized and is suitable for single-threaded environments. Hashtable is synchronized and thread-safe but generally slower. ConcurrentHashMap is designed for concurrent access, providing better performance in multithreaded environments.
Why is the Stream API faster than an iterator in Java?
-The Stream API can be faster because it supports parallel processing and allows more optimized operations on data collections.
What is the purpose of service discovery or a service registry in microservices architecture?
-Service discovery or service registry is used to register and manage all the services in an application. It helps in locating and distributing client requests to different services, checking the status of services, and managing service instances.
What is a Spring Bean and how is it used?
-A Spring Bean is an object managed by the Spring IoC container. It is defined in the configuration file or by using annotations, and the container instantiates, configures, and manages the bean's lifecycle.
Outlines
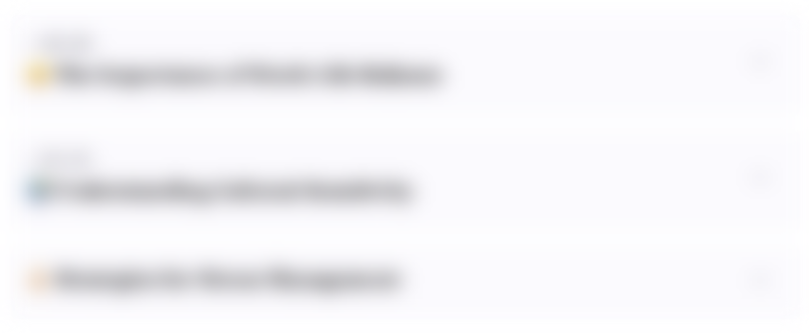
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
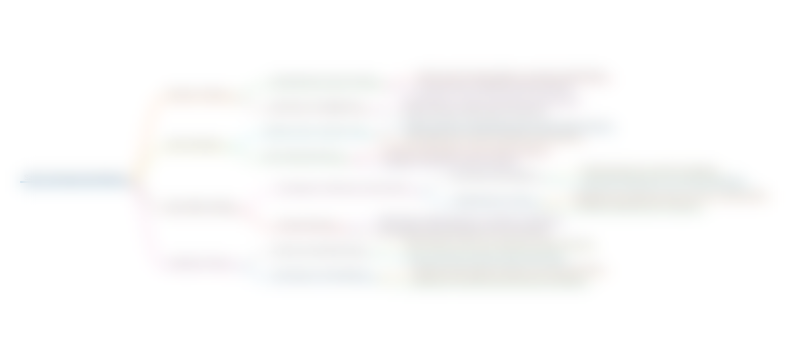
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
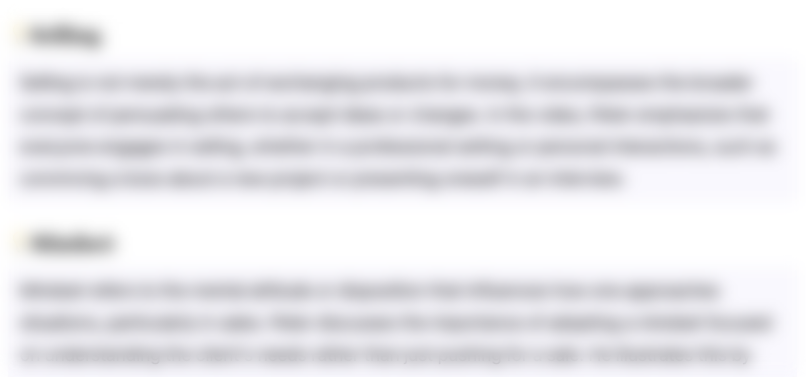
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
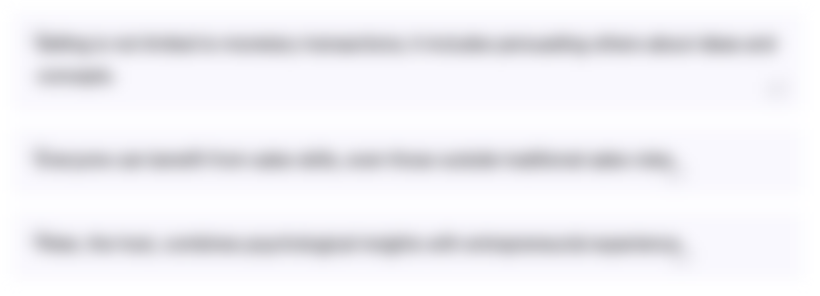
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
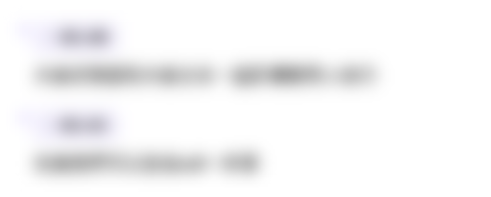
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

TCS Java | Spring Boot | Microservices | 4 Years | Selected | Mock Interview

Capgemini Java Developer 4 yrs interview Questions and Answers L2 round #capgemini

3+ years Java Developer Accenture Interview Experience| Java | Spring Boot | Microservices
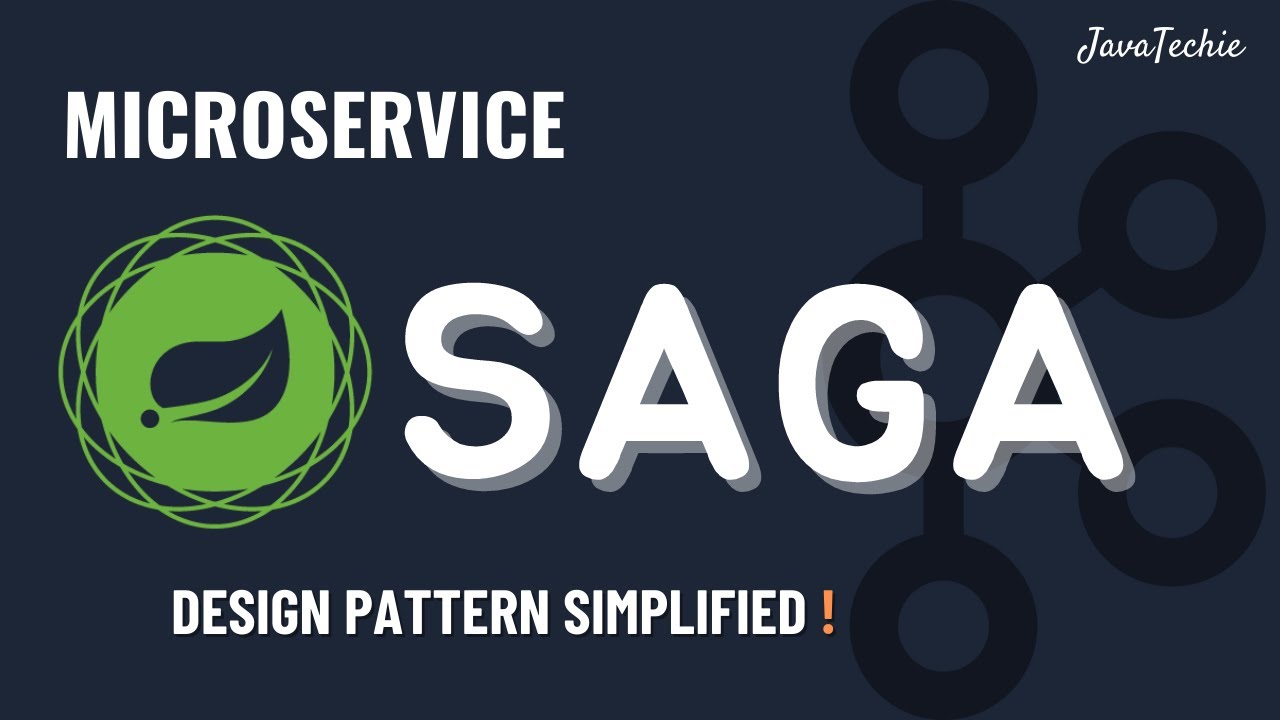
Microservices Architecture Patterns | SAGA Choreography Explained & Project Creation | JavaTechie

Capgemini Java Interview 2024 | Java | Spring Boot | Microservices | Database
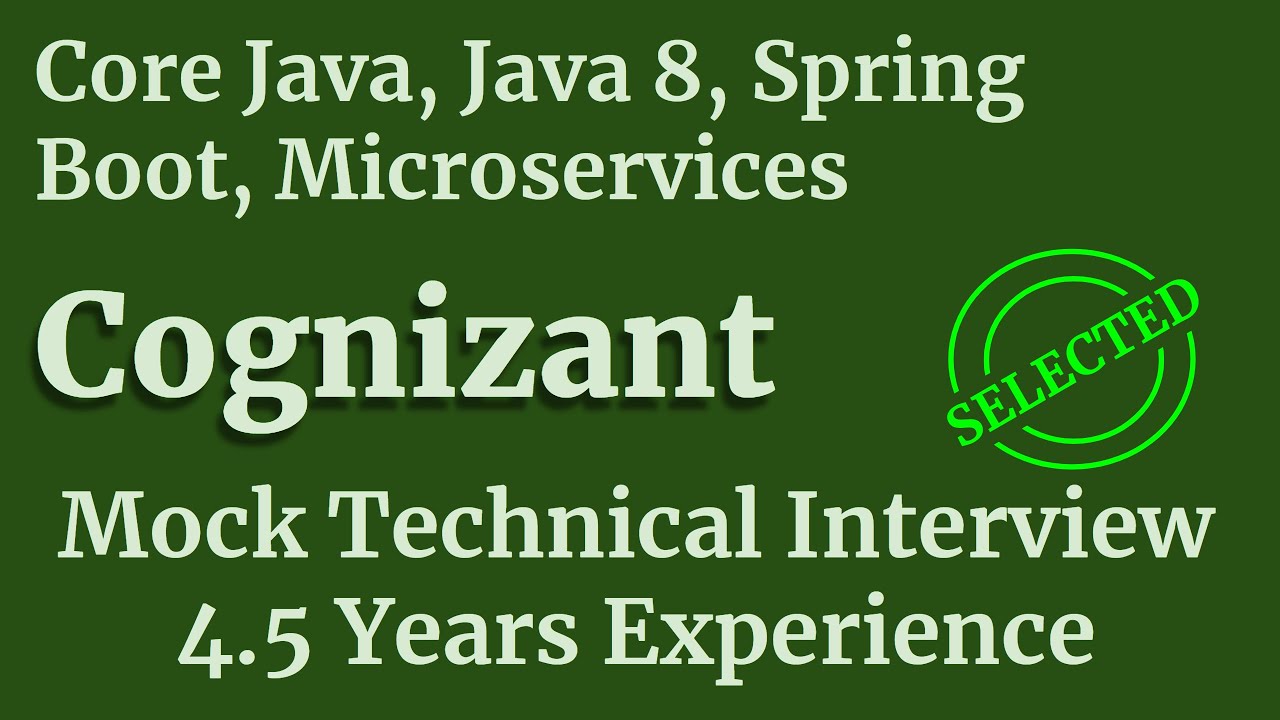
Core Java, Spring Boot, Microservice Interview Questions | Cognizant L1 Technical Interview
5.0 / 5 (0 votes)