Core Java, Spring Boot, Microservice Interview Questions | Cognizant L1 Technical Interview
Summary
TLDRThis transcript is a detailed technical interview focused on Java, Spring Boot, and Microservices. The candidate discusses core concepts like abstraction, inheritance, collections, and multi-threading in Java, followed by features of Spring Boot such as actuator for monitoring and logging with Splunk. The candidate also demonstrates a solid understanding of microservices, explaining API gateways, service discovery, and tools like Histrix to ensure fault tolerance. Throughout, they provide clear, precise explanations of key technical concepts, offering insights into both theoretical knowledge and practical application in real-world projects.
Takeaways
- π Abstraction in Java involves hiding irrelevant details and exposing only necessary information, achieved through abstract classes and interfaces.
- π An interface in Java is a contract that ensures a class implements specific methods, with Java 8 introducing default and static methods along with functional interfaces.
- π In case of conflicting default methods from multiple interfaces, Java allows disambiguation using `i1.super.print()` to call the method from a specific interface.
- π Inheritance in Java has different types: single inheritance (one parent class), multi-level inheritance (parent, child, grandchild), and hierarchical inheritance (multiple subclasses inheriting from a common parent).
- π Strings in Java are immutable, meaning once a string is created, its value cannot be changed; modifying a string results in the creation of a new instance.
- π Collections like HashMap, ArrayList, and LinkedList are used to store and manage data, with ArrayList offering random access and LinkedList being more efficient for frequent insertions.
- π A HashMap stores key-value pairs in buckets, with the keyβs hashcode determining the bucket placement, and this mechanism is essential for quick retrieval of values.
- π Multithreading in Java involves states such as new, runnable, running, waiting, and terminated, with thread synchronization allowing threads to wait for conditions before proceeding.
- π The Comparable interface is used for natural ordering of objects (e.g., numeric or alphabetical), while the Comparator interface is used for custom sorting logic.
- π In Spring Boot, Actuator helps in monitoring application health and performance, while logs can be managed using tools like Splunk for better error detection and troubleshooting.
Q & A
What is abstraction in Java?
-Abstraction in Java refers to the concept of hiding the irrelevant details from the user and only exposing the essential information. For example, when calling a method, you only provide the method name and necessary parameters, hiding the internal workings of the method like how sorting happens.
How can abstraction be achieved in Java?
-Abstraction in Java can be achieved through two main ways: using abstract classes and interfaces. Interfaces are typically preferred for abstraction as they define a contract that the implementing classes must follow.
What are the features added to interfaces in Java 8?
-Java 8 introduced several features to interfaces, including default methods, static methods, and functional interfaces. Default methods allow method implementations within interfaces, static methods allow utility methods, and functional interfaces are those with only one abstract method.
How would you call a method from an interface when two interfaces have a default method with the same signature?
-If two interfaces (e.g., I1 and I2) have the same default method signature and a class implements both, you can call the method from a specific interface using the syntax `I1.super.print()` to explicitly call the method from I1.
What is inheritance in Java and what are its types?
-Inheritance in Java is the mechanism where one class inherits the functionality of another class. Types include single inheritance (a child class extends one parent class), multi-level inheritance (a chain of inheritance with multiple generations), and hierarchical inheritance (multiple classes inherit from a single parent class).
What is the difference between ArrayList and LinkedList in Java?
-ArrayList provides random access to elements using an underlying array, making it faster for retrieval. However, adding/removing elements in the middle of the list can be slower. LinkedList, on the other hand, uses nodes and is better for frequent insertion/deletion of elements but slower for random access.
How does a HashMap work internally in Java?
-HashMap uses a hashing mechanism where the hash code of an object determines which bucket the object will be placed in. The hash code is taken modulo the number of buckets to find the appropriate bucket index. When retrieving an object, the same hash code is used to locate the bucket.
What is multi-threading and can you explain the thread lifecycle in Java?
-Multi-threading allows the execution of multiple threads concurrently, improving performance. The thread lifecycle in Java includes the following states: New (when a thread is created), Runnable (when a thread is ready to run), Running (when the thread is executing), Waiting (when a thread is waiting for a specific condition), and Terminated (when the thread finishes execution).
What is an API Gateway in the context of microservices?
-An API Gateway acts as a single entry point for microservices. It handles incoming requests and routes them to the appropriate microservices. It can also manage common functionalities like authentication, logging, and load balancing. In this scenario, Zuul was used as the API Gateway.
What is the difference between vertical and horizontal scaling?
-Vertical scaling involves increasing the capacity of a single machine (e.g., upgrading RAM), while horizontal scaling involves adding more machines to distribute the load across multiple instances, providing more resources and redundancy.
Outlines
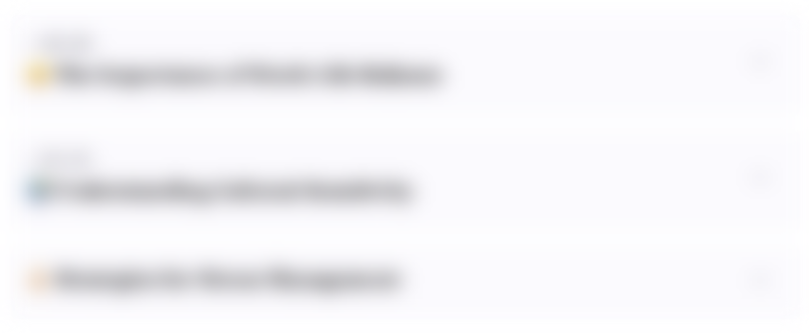
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
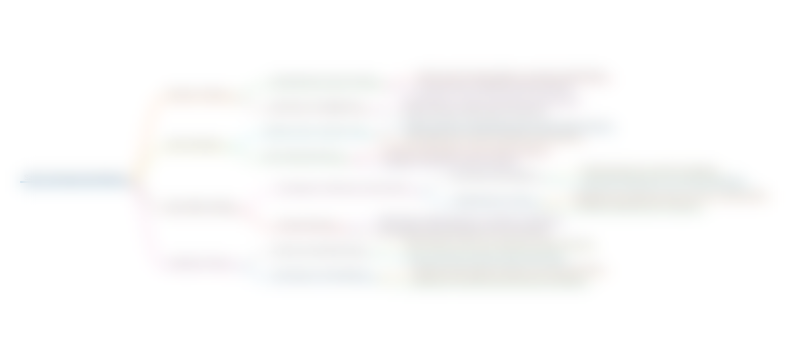
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
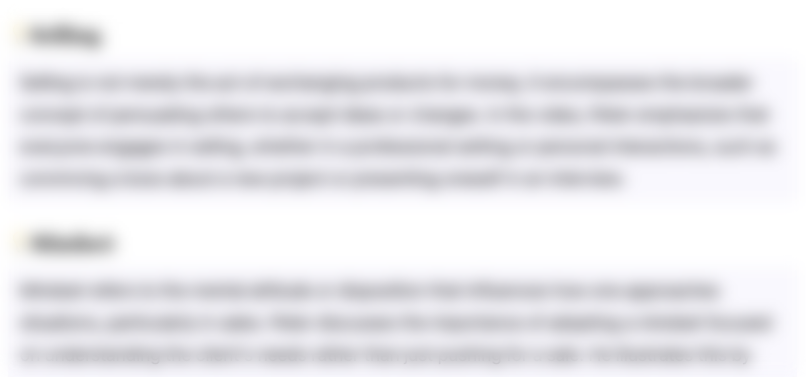
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
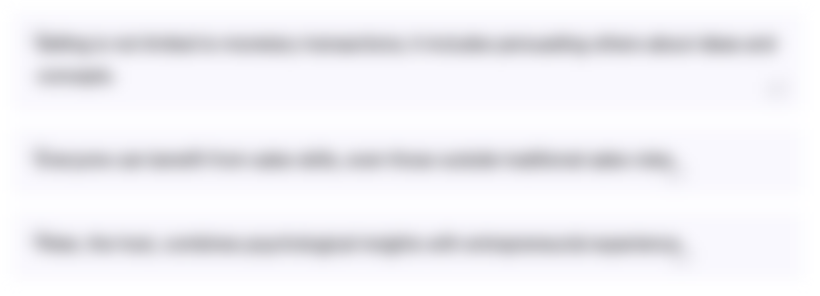
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
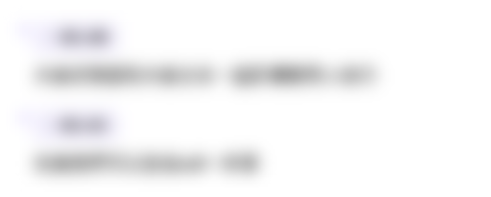
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Capgemini Java Developer 4 yrs interview Questions and Answers L2 round #capgemini

3+ years Java Developer Accenture Interview Experience| Java | Spring Boot | Microservices
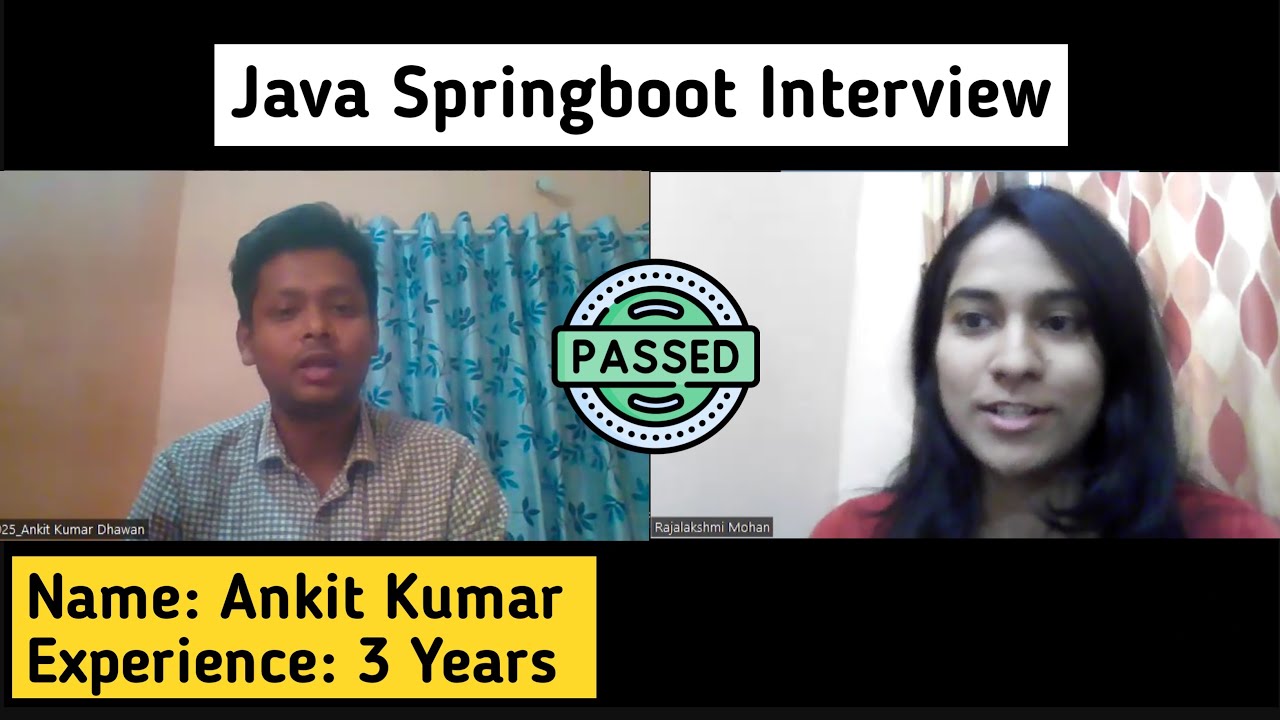
Java Spring Boot 3 Years Experience Interview

TCS Java | Spring Boot | Microservices | 4 Years | Selected | Mock Interview

HCL Java Fullstack Developer Interview Experience & Questions
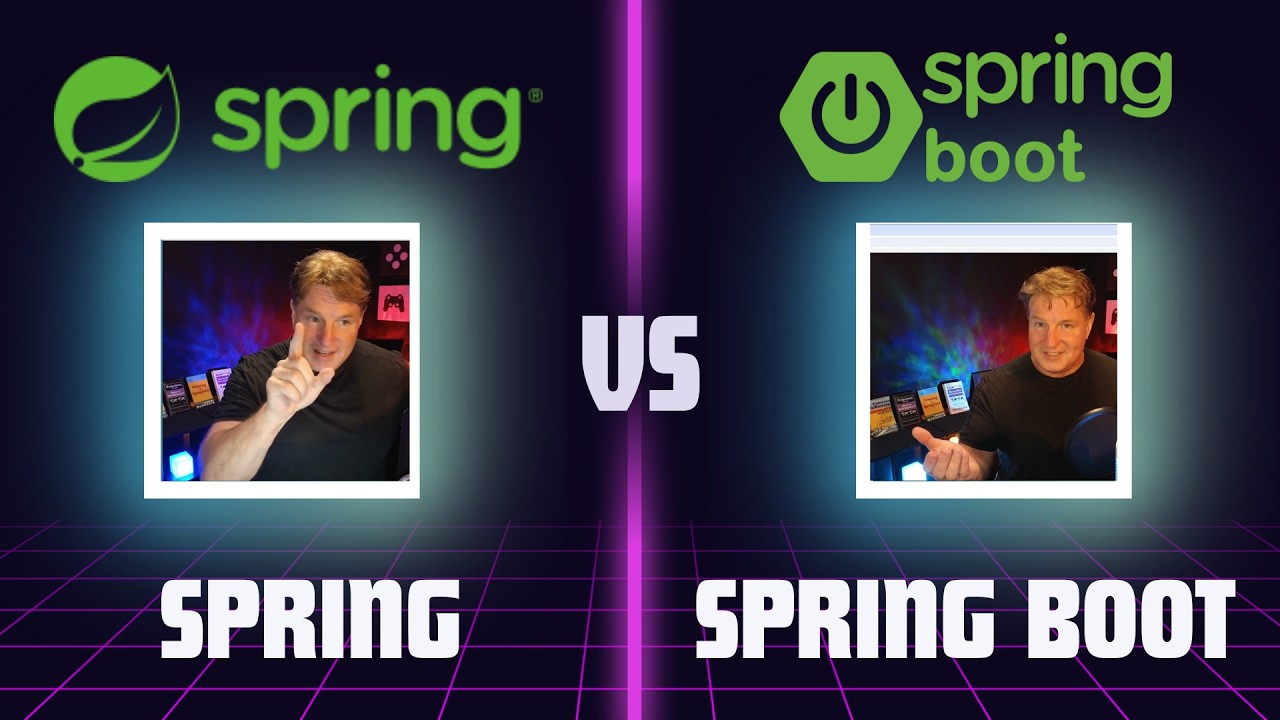
Spring Boot vs Spring vs the Spring Framework: What's the difference?
5.0 / 5 (0 votes)