Data Loaders (the N+1 problem) - GRAPHQL API IN .NET w/ HOT CHOCOLATE #6
Summary
TLDRThis video script discusses optimizing GraphQL application performance by integrating a DataLoader to address the 'n plus one problem'. It covers removing unnecessary joins, fetching data only when requested, and demonstrating how DataLoaders can batch multiple queries into a single database request, ultimately improving efficiency.
Takeaways
- 🔧 The video discusses performance considerations when integrating a GraphQL application with a database, specifically focusing on query optimization to prevent frequent or inefficient database hits.
- 🤔 The 'n plus one problem' in GraphQL is highlighted, where multiple queries are made for each item in a list, leading to performance degradation.
- 🔄 To address the n plus one problem, the video introduces the use of a DataLoader to batch multiple queries into a single database request, improving efficiency.
- 🛠 The script demonstrates removing unnecessary joins in the database queries to prevent over-fetching data when not required by the GraphQL client.
- 📚 The importance of conditional data fetching is emphasized, where data is fetched only when requested by the client to avoid unnecessary database hits.
- 🔧 The process of creating a service and repository for instructors is shown, to handle database operations related to instructor data separately from courses.
- 🔄 The concept of using a DataLoader is further explained by creating an 'InstructorDataLoader' that batches queries for multiple instructor IDs into one database call.
- 📝 The script covers the implementation of the DataLoader in the GraphQL resolver, showing how to pass the DataLoader into the resolver and use it to fetch data.
- 🔗 The video explains how to pass data from one resolver to a nested resolver using a property on a class, which can be included or excluded from the GraphQL schema.
- 🛠 The process of creating a method in the repository to handle batch fetching of instructors by multiple IDs is detailed, which is crucial for the DataLoader to function.
- 🔍 The script concludes with a demonstration of the DataLoader in action, showing how it reduces the number of database hits from multiple to a single query for all required data.
Q & A
What is the 'n plus one problem' in the context of GraphQL?
-The 'n plus one problem' in GraphQL refers to a performance issue where a single query returns a list of items, and for each item in the list, an additional query is made to fetch related data. This results in n+1 queries being executed, which can significantly degrade performance.
Why is over-fetching data a concern in GraphQL?
-Over-fetching data in GraphQL is a concern because it can lead to unnecessary network traffic and database load, which can impact the performance of the application. It happens when more data is requested than what is actually needed by the client.
How does a DataLoader help in improving GraphQL query performance?
-A DataLoader helps in improving GraphQL query performance by batching multiple requests for the same resource into a single query to the database. This reduces the number of database hits and solves the 'n plus one problem' by fetching all related data in one go.
What changes were made to the Courses repository in the script?
-In the script, the joins on the Instructor and Students tables were removed from the Courses repository to prevent unnecessary data fetching when the client does not request that data.
Why can't the properties for Instructor and Student be removed from the Course DTO?
-The properties for Instructor and Student cannot be removed from the Course DTO because they are used to describe the relationships between tables in the schema of the application, which is necessary for the GraphQL framework to understand the data structure.
How is the Instructor data fetched in the script's resolver?
-The Instructor data is fetched in the resolver by implementing a service that hits the database to retrieve the Instructor information only when the GraphQL client requests it, thus avoiding over-fetching.
What is the purpose of creating a separate Instructor repository in the script?
-The purpose of creating a separate Instructor repository is to encapsulate the data access logic for Instructor entities and to facilitate the use of DataLoader for batching queries to improve performance.
How does the script demonstrate passing data from one resolver to a nested resolver?
-The script demonstrates passing data from one resolver to a nested resolver by defining a property on the query type that can be used in the nested resolver, allowing the Instructor ID to be passed down to the Instructor resolver.
What is the role of the Instructor DataLoader in the script?
-The Instructor DataLoader in the script is used to batch multiple requests for Instructor data into a single database query, thus solving the 'n plus one problem' and improving the efficiency of data fetching.
How does the script address the potential inefficiency of making two separate database queries?
-The script addresses the potential inefficiency by using a DataLoader to batch all the Instructor queries into one database request, reducing the number of database hits and improving performance.
What is the trade-off mentioned in the script regarding the use of joins versus separate queries?
-The trade-off mentioned is that while using joins might be more efficient when querying for related data, making separate queries can be faster when the related data is not needed, as it avoids unnecessary data fetching.
Outlines
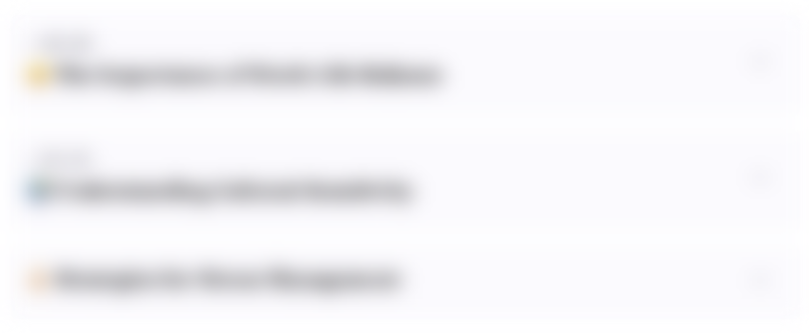
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
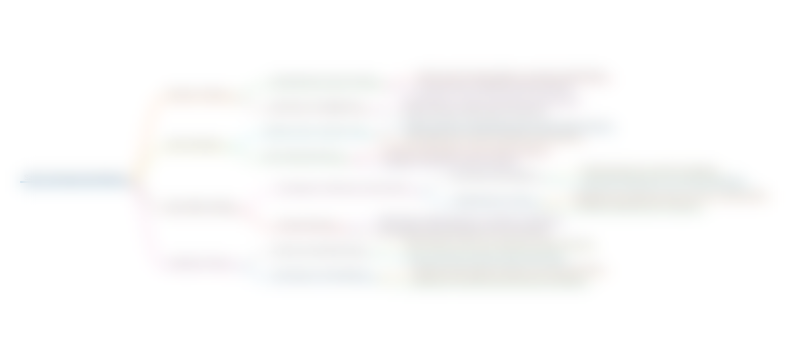
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
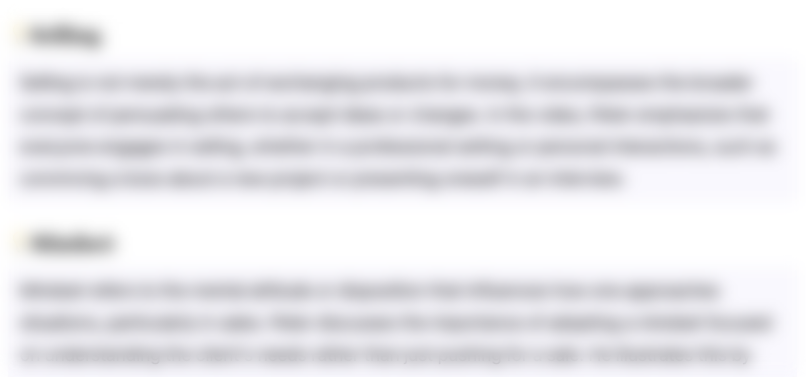
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
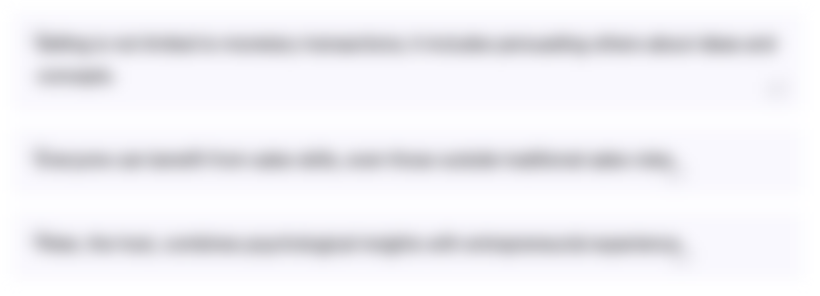
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
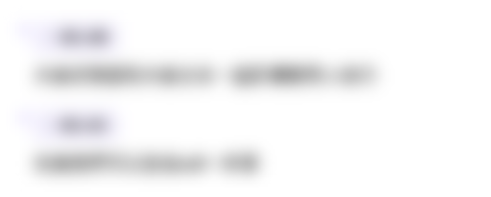
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Why GraphQL within CMS Projects can COST you thousands!

What Could Go Wrong with a GraphQL Query and Can OpenTelemetry Help? - Budhaditya Bhattacharya, Tyk
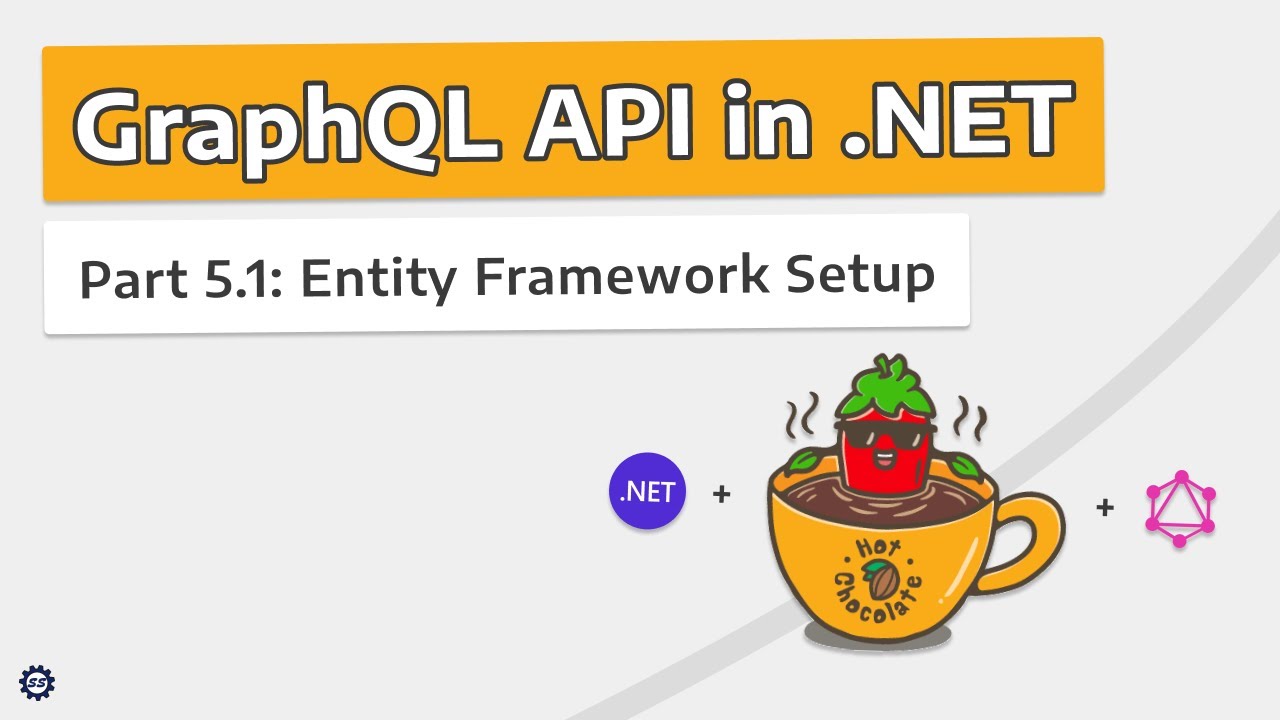
Entity Framework Setup - GRAPHQL API IN .NET w/ HOT CHOCOLATE #5.1
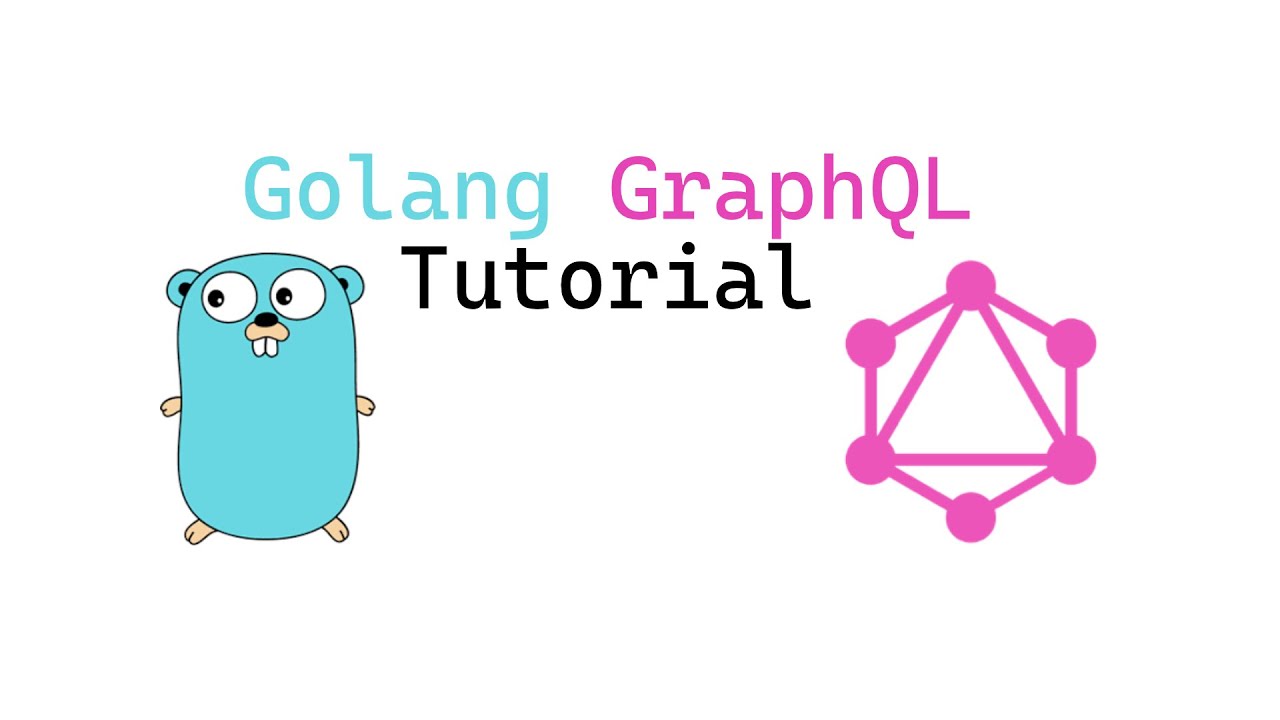
Golang GraphQL Tutorial - Intro
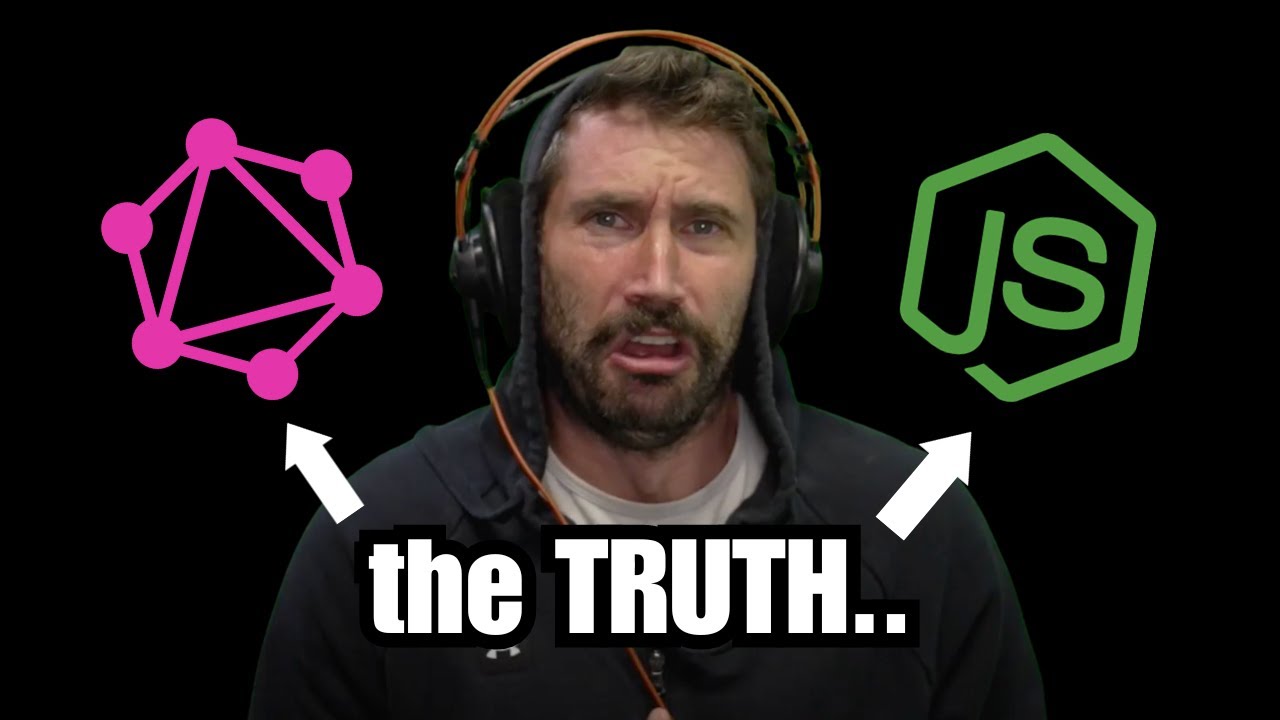
The Hidden Cost Of GraphQL And NodeJS
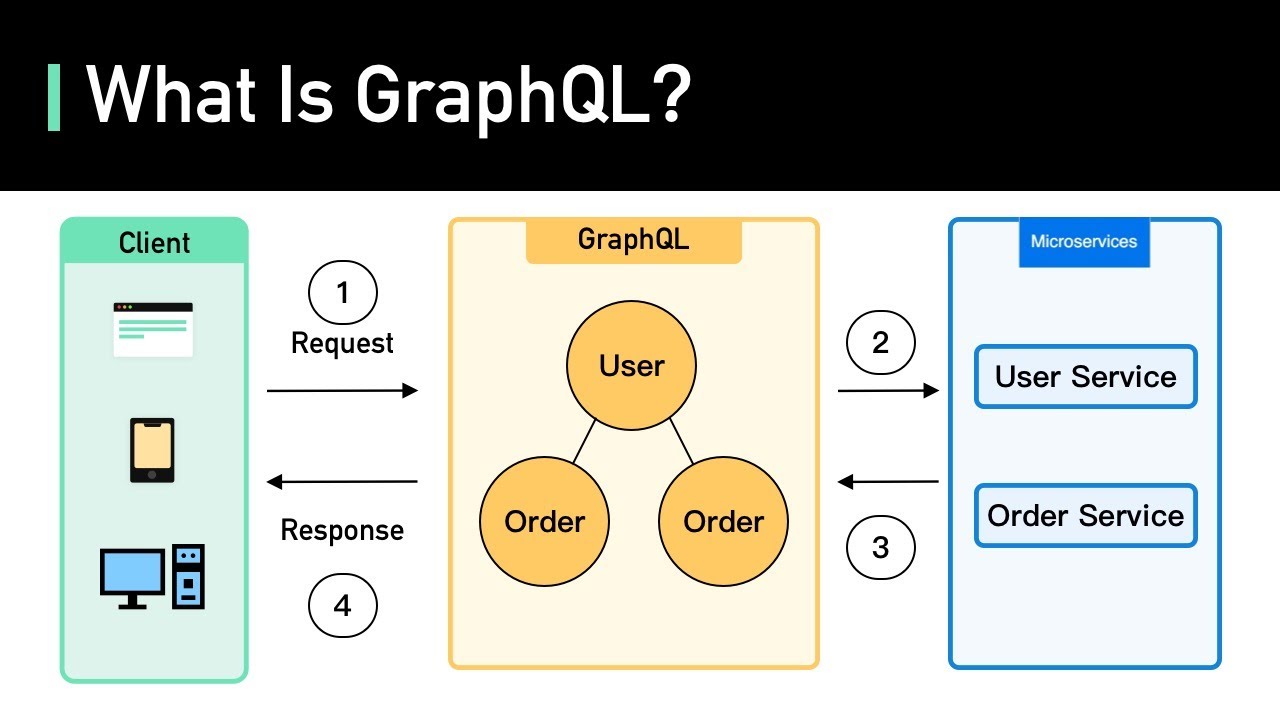
What Is GraphQL? REST vs. GraphQL
5.0 / 5 (0 votes)