Goodbye, useEffect - David Khourshid
Summary
TLDRThe speaker delivers an in-depth tutorial on the proper use of React's useEffect hook, emphasizing its purpose for synchronization rather than as a lifecycle hook. They address common misconceptions, illustrate the pitfalls of misuse, and provide guidance on alternative approaches for handling side effects, such as using event handlers for 'fire and forget' actions and leveraging new hooks like useSyncExternalStore for subscriptions. The talk concludes with a live demonstration of utilizing state machines for effect management, showcasing a simplified and efficient way to handle complex component behaviors in React.
Takeaways
- 🌟 The speaker emphasizes the importance of using `useEffect` correctly, highlighting that many developers struggle with it, even at a senior level.
- 🔄 React 18 introduces changes that can cause effects to run twice on mount in strict mode, which can be confusing and needs to be managed properly.
- 🤔 The speaker suggests that `useEffect` should be thought of as a synchronization tool rather than a lifecycle hook, aligning with the mental model proposed by Dan Abramov.
- 🚫 It's advised against using `useEffect` for transforming data, communicating with parents, or subscribing to external stores, as there are better alternatives.
- 📈 The script discusses the misuse of `useEffect` for fetching data, recommending instead to render as you fetch using libraries like React Query or framework-specific solutions.
- 🛑 The speaker warns against setting state inside `useEffect`, as it can lead to infinite loops and performance issues.
- 🔍 The script highlights the new React documentation at beta.reactjs.org as a valuable resource for understanding the proper use of `useEffect` and other hooks.
- 📚 The speaker introduces the concept of using state machines to model effects, making the logic clearer and more maintainable.
- 🔌 The script mentions `useSyncExternalStore` as a powerful hook for subscribing to external stores in a more efficient and less error-prone way.
- 🎯 The speaker concludes by summarizing that `useEffect` is for synchronization, state transitions trigger effects, and action effects should be handled in event handlers.
Q & A
What is the main focus of the talk at React Brussels?
-The main focus of the talk is to provide a crash course on the correct usage of the useEffect hook in React, and to address common issues and misconceptions about it.
Why might a React developer struggle with useEffect?
-A developer might struggle with useEffect due to its complexity, especially when dealing with large dependency arrays, conditional statements, and issues arising from React 18's behavior of running effects twice on mount in strict mode.
What is the 'frustrated react developer Smiley' mentioned in the script?
-The 'frustrated react developer Smiley' is a humorous reference to the common struggles developers face when dealing with complex or confusing aspects of React, such as the useEffect hook.
What is the difference between 'fire and forget' effects and synchronized effects in React?
-Fire and forget effects are those where the result of the effect is not important, like a console log or an analytics call. Synchronized effects, on the other hand, involve communication with an external system and may be long-lived, requiring synchronization with the component's state.
Why should you avoid setting state inside useEffect?
-Setting state inside useEffect can lead to unexpected behavior and potential infinite loops, especially when the state update triggers the effect again, creating a cycle of state changes and effect executions.
What is the correct mental model for understanding useEffect according to the talk?
-The correct mental model for understanding useEffect is 'synchronization' rather than 'lifecycle'. It should be used to synchronize the component's state with external changes or side effects that are not directly related to the component's rendering.
What is the purpose of the 'use sync external store' hook mentioned in the script?
-The 'use sync external store' hook is used for subscribing to external data stores. It simplifies the process of subscribing, reading, and unsubscribing from an external store, making it easier to manage state that comes from outside the React component.
Why is 'render-as-you-fetch' considered a better approach than using useEffect for fetching data?
-'Render-as-you-fetch' is considered better because it allows the component to render immediately with the fetched data, avoiding unnecessary re-renders and complexity associated with managing promises and cancellation inside useEffect.
What are some alternative solutions to using useEffect for fetching data?
-Alternative solutions include using framework-specific solutions like Remix's loader functions, Next.js's getServerSideProps, or third-party libraries like React Query, which are designed to handle data fetching and caching more efficiently.
What is the 'use' hook mentioned in the script, and what does it offer?
-The 'use' hook, which is part of an RFC for first-class support for promises and async, is a new approach that might become the future of using suspense inside of React. It allows components to automatically suspend when data is being fetched, providing a more streamlined way to handle asynchronous data.
How can state machines be used to model effects in React components?
-State machines can be used to model effects by defining state transitions and associating effects with those transitions. This approach helps visualize and reason about the component's behavior, making it easier to understand when and how effects should be triggered.
Outlines
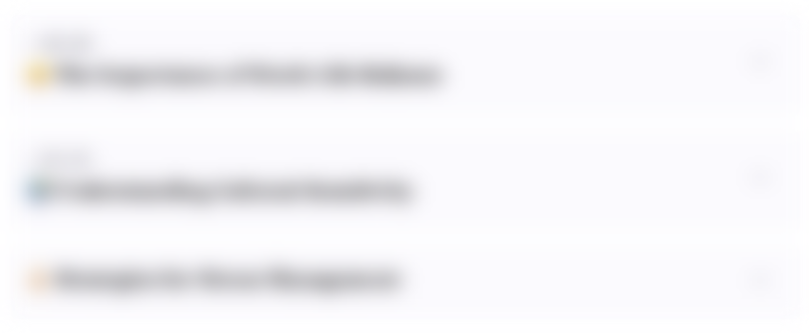
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
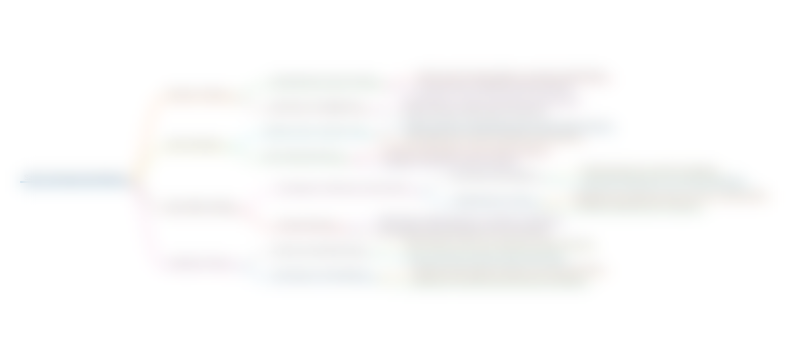
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
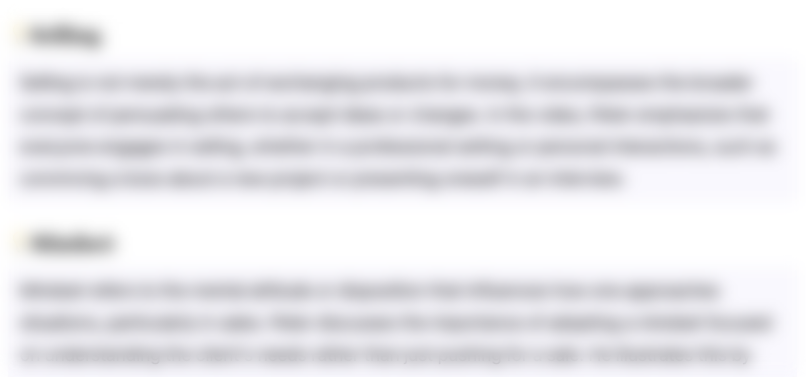
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
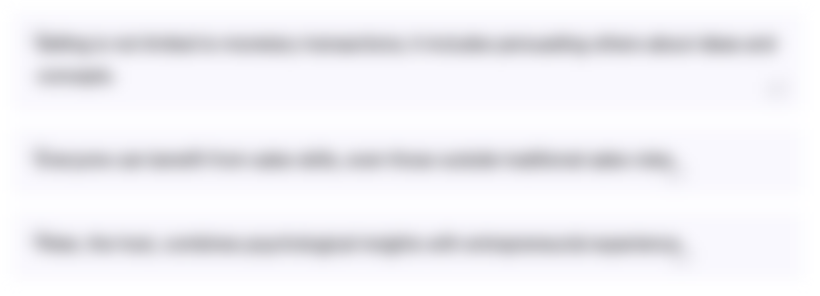
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
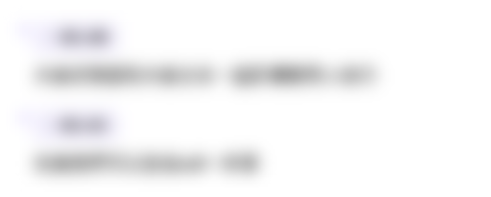
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

A First Look at Effects | Lecture 141 | React.JS 🔥

The useEffect Dependency Array | Lecture 145 | React.JS 🔥
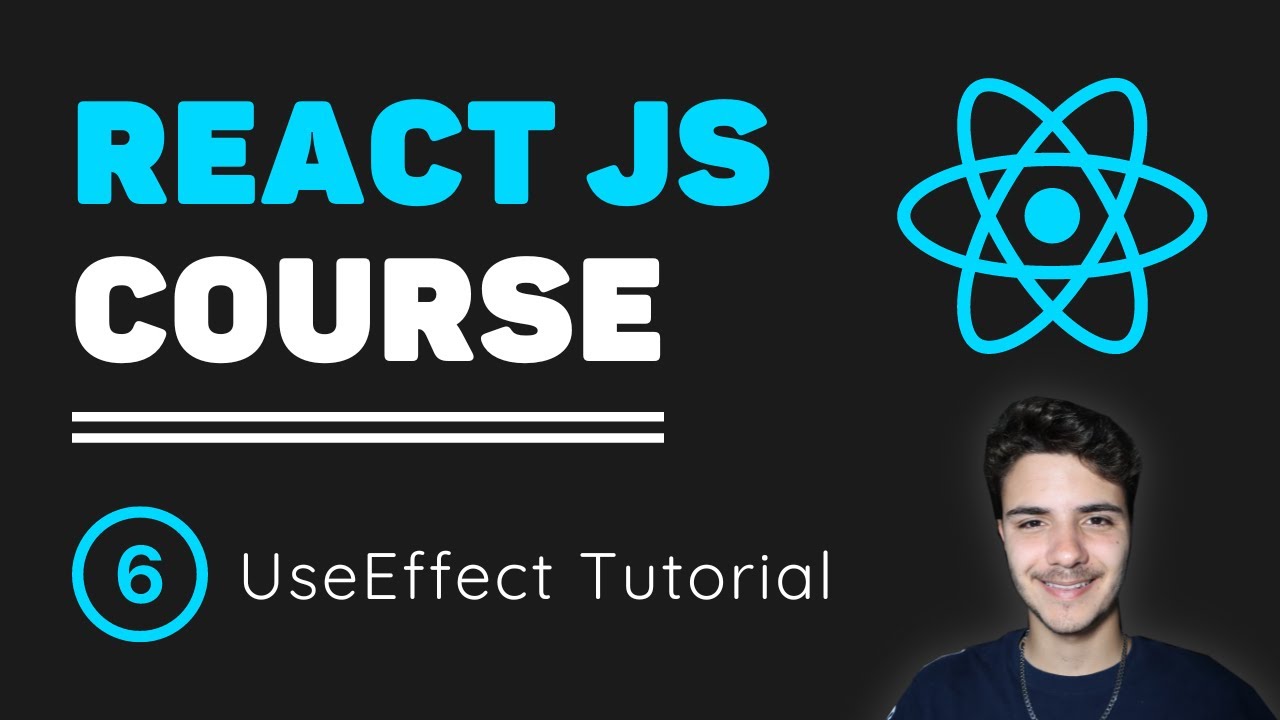
ReactJS Course [6] - Component Lifecycle | UseEffect Tutorial

useEffect to the Rescue | Lecture 140 | React.JS 🔥

The useEffect Cleanup Function | Lecture 151 | React.JS 🔥
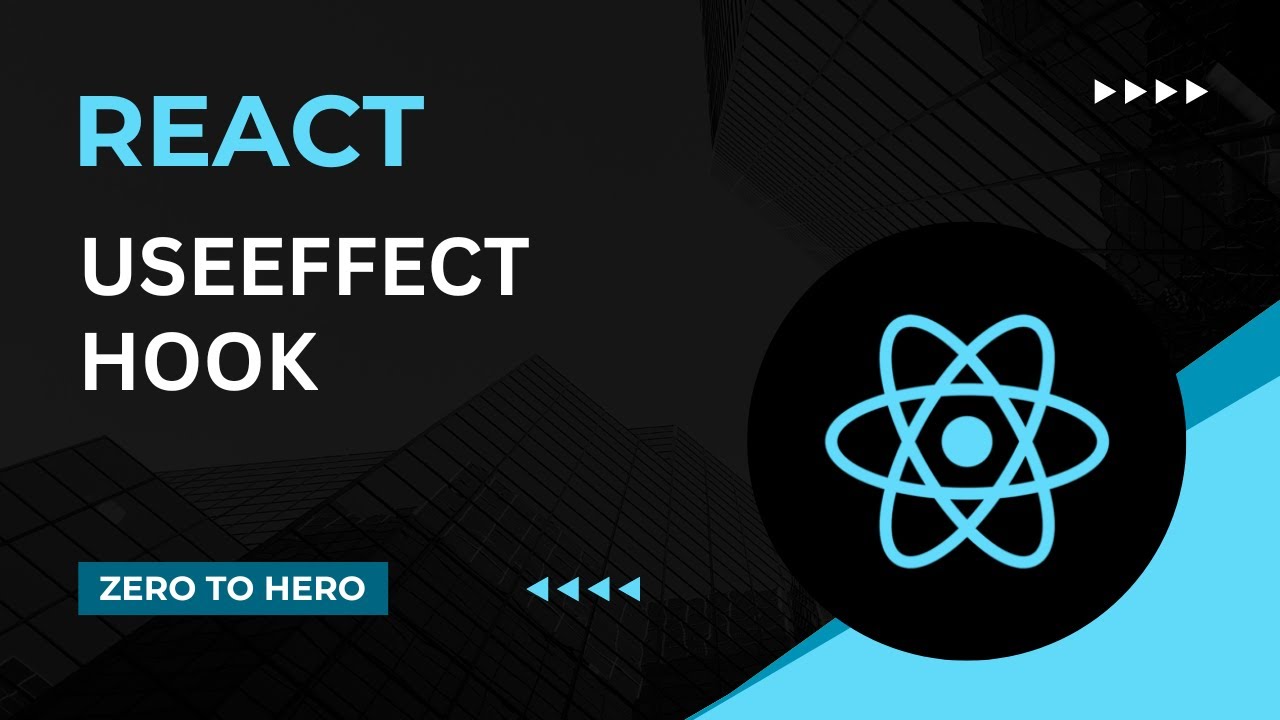
useEffect Hook | Mastering React: An In-Depth Zero to Hero Video Series
5.0 / 5 (0 votes)