ReactJS Course [6] - Component Lifecycle | UseEffect Tutorial
Summary
TLDRThis React.js tutorial delves into the component lifecycle and the powerful 'useEffect' hook. The instructor explains the three stages of a component's life: mounting, updating, and unmounting, using a simple project to illustrate these concepts. The 'useEffect' hook is highlighted for its role in controlling actions based on lifecycle stages, with examples demonstrating its use for API calls and state changes. The video also addresses Strict Mode's role in identifying potential issues with useEffect. The tutorial is designed to provide a foundational understanding before delving into more advanced applications in subsequent lessons.
Takeaways
- 📚 The video is the sixth episode of a React.js course, focusing on the component lifecycle and the use of the useEffect hook.
- 🔄 The React component lifecycle is divided into three stages: mounting, updating, and unmounting.
- 📈 Mounting refers to a component appearing on the screen or starting to exist in a project.
- 📉 Unmounting is the opposite of mounting, where a component stops appearing on the screen.
- 🛠 Updating is when a component changes the UI in response to changes in props, state, or other factors.
- 🔑 The useEffect hook is crucial for controlling actions based on the lifecycle stage of a component.
- 🎯 useEffect can be used to perform actions when a component mounts, updates, or unmounts.
- 🚀 useEffect is triggered by default on mount and update, but can be controlled with dependency arrays.
- 🔍 The video demonstrates the use of useEffect with a simple project involving a text component and a button.
- 👀 The use of Strict Mode in React can help identify potential issues by simulating mount and unmount cycles.
- 🔬 The video explains that useEffect may run twice in Strict Mode as a way to check for proper cleanup on unmount.
- 🔑 The video emphasizes the importance of understanding useEffect for efficient and clean React component management.
Q & A
What is the main focus of the sixth episode of the React.js course?
-The main focus of the sixth episode is to teach about the React.js component lifecycle and how to use the 'useEffect' hook to manipulate components based on their lifecycle stages.
What are the three stages of the React component lifecycle mentioned in the video?
-The three stages of the React component lifecycle are the mounting stage, the updating stage, and the unmounting stage.
What does the term 'mounting' refer to in the context of React components?
-'Mounting' refers to the process where a component appears on the screen or starts to exist in the project.
What is the purpose of the 'useEffect' hook in React?
-The 'useEffect' hook is used to control what happens depending on which stage of the component's lifecycle it is in, such as mounting, updating, or unmounting.
How does the 'useEffect' hook work by default?
-By default, the 'useEffect' hook executes the function provided to it when the component mounts.
Outlines
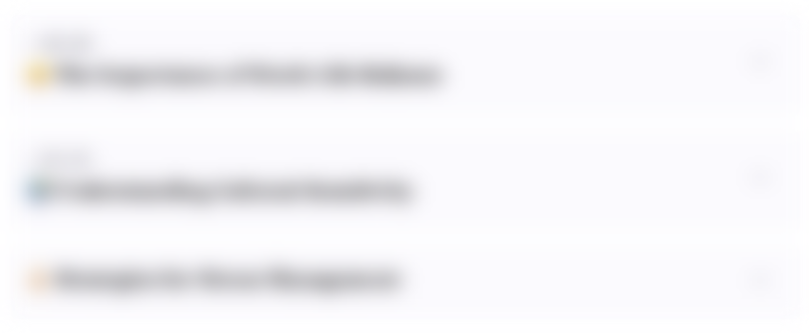
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
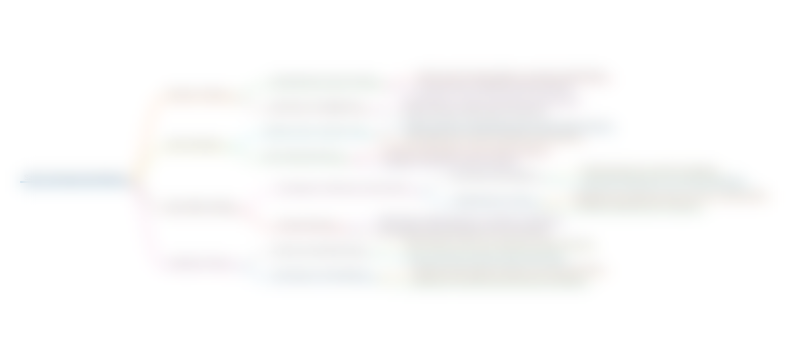
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
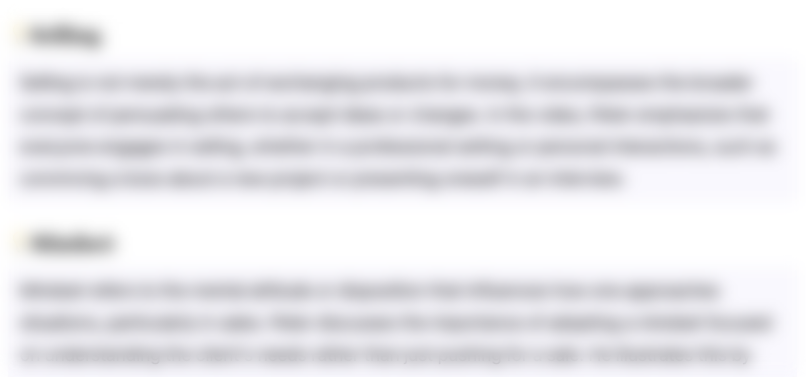
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
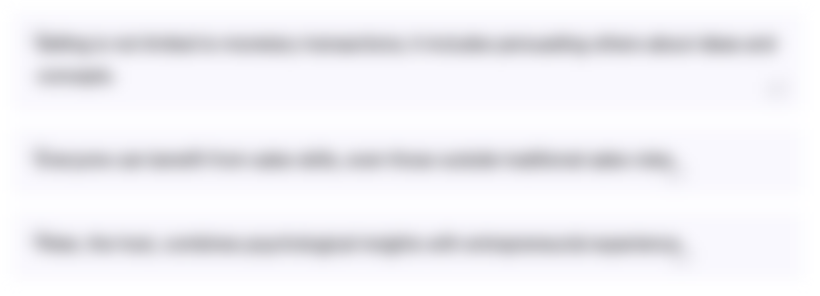
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
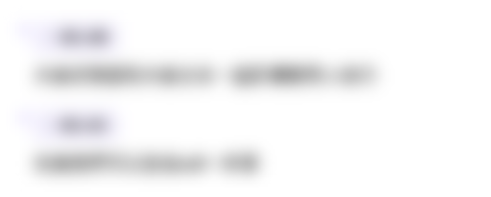
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
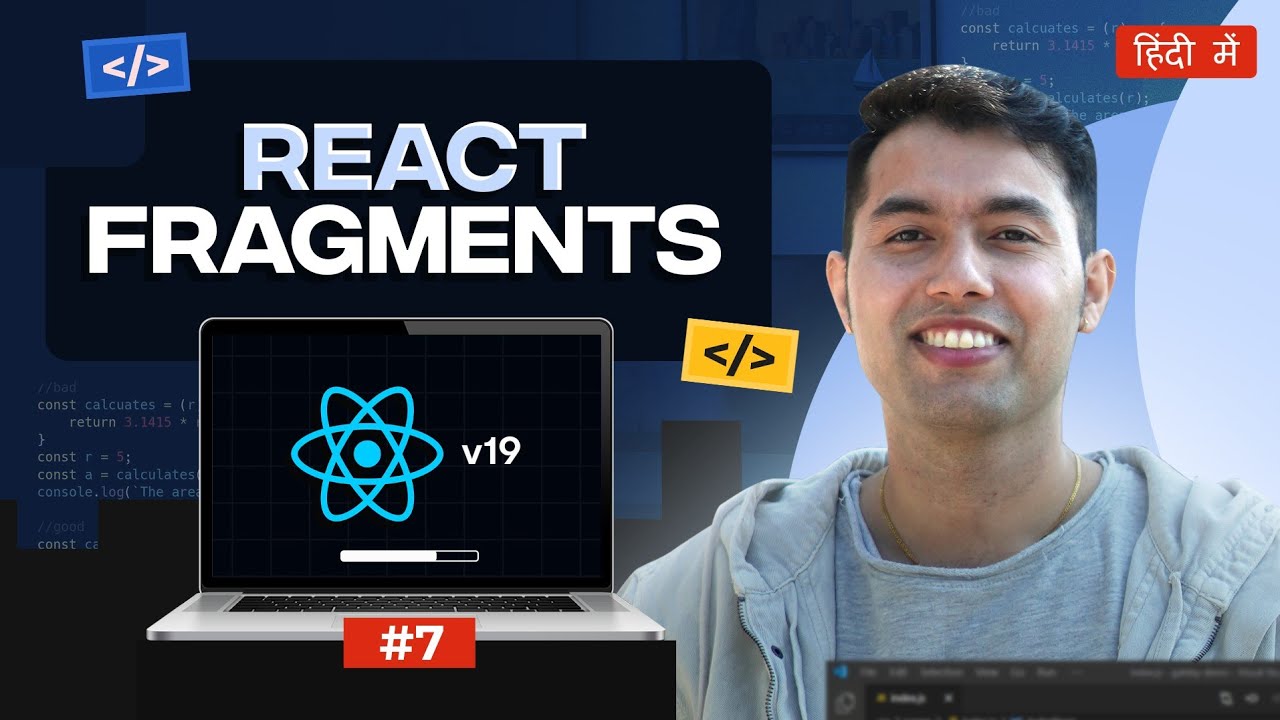
#7: React Fragments: Remove unwanted Nodes & Speed Up Rendering | React v19 Tutorial in Hindi

React Query - Complete Tutorial
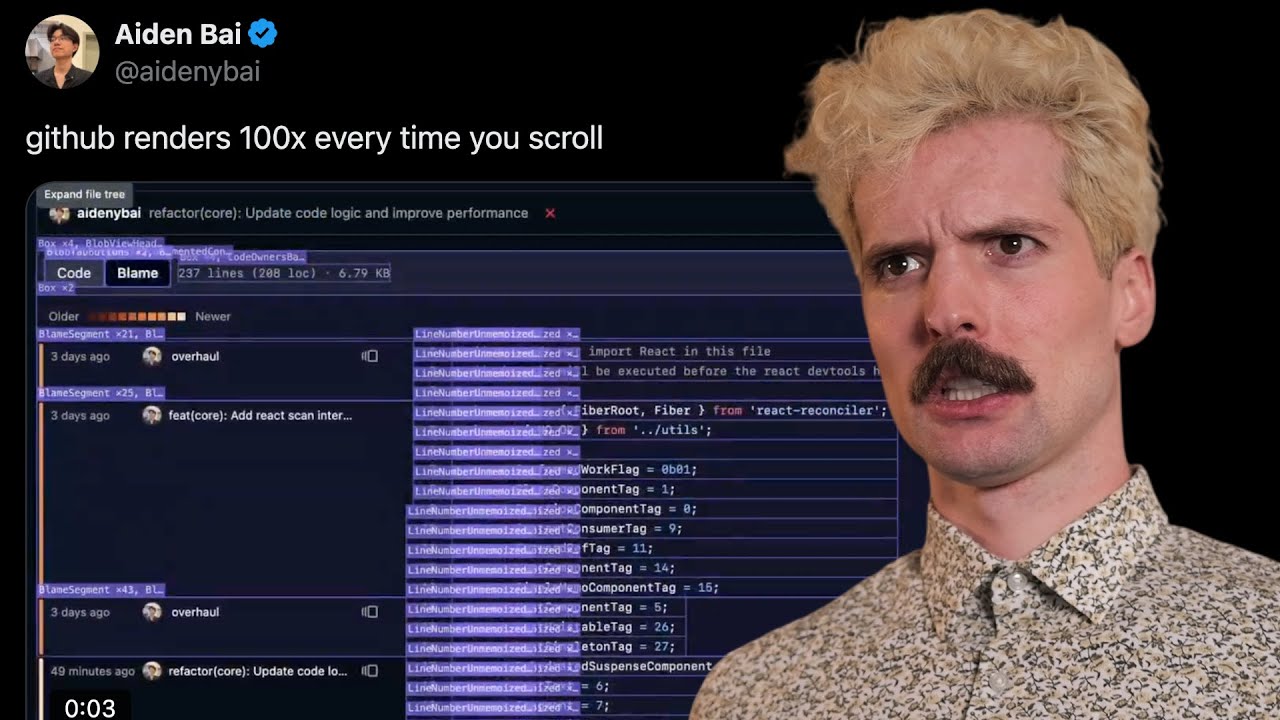
Why is every React site so slow?
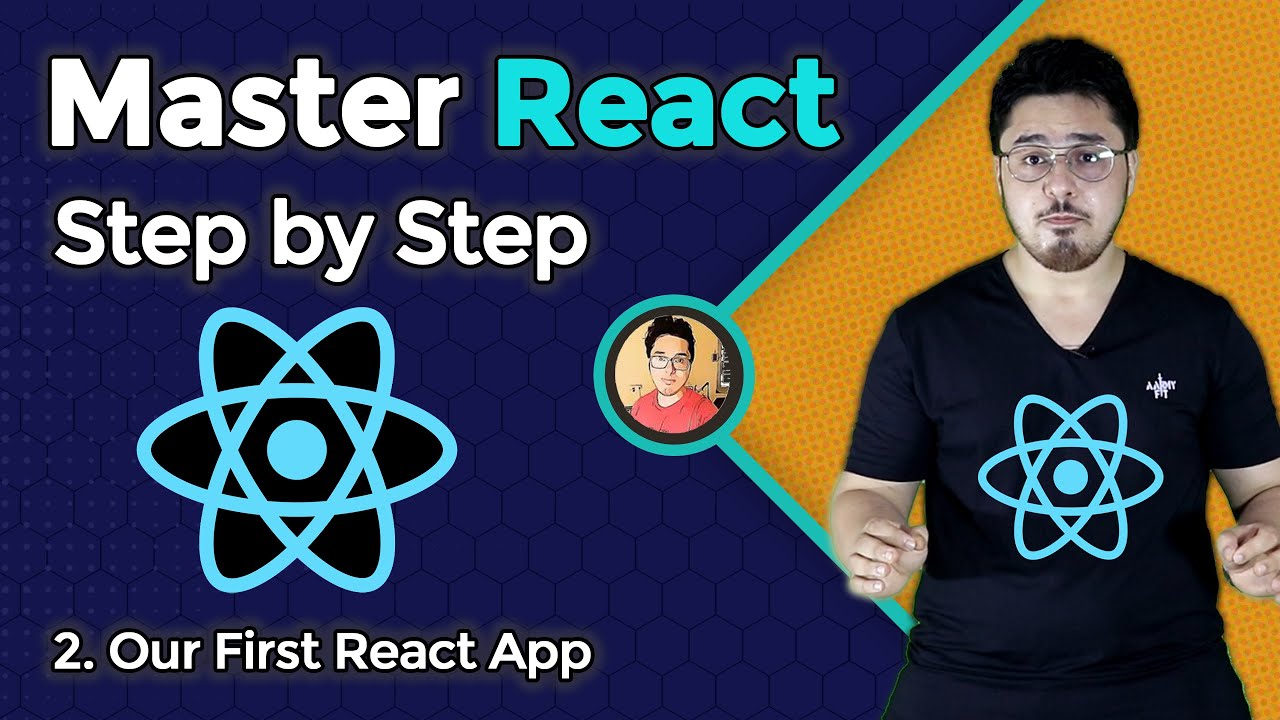
Creating our first react app using create-react-app | Complete React Course in Hindi #2
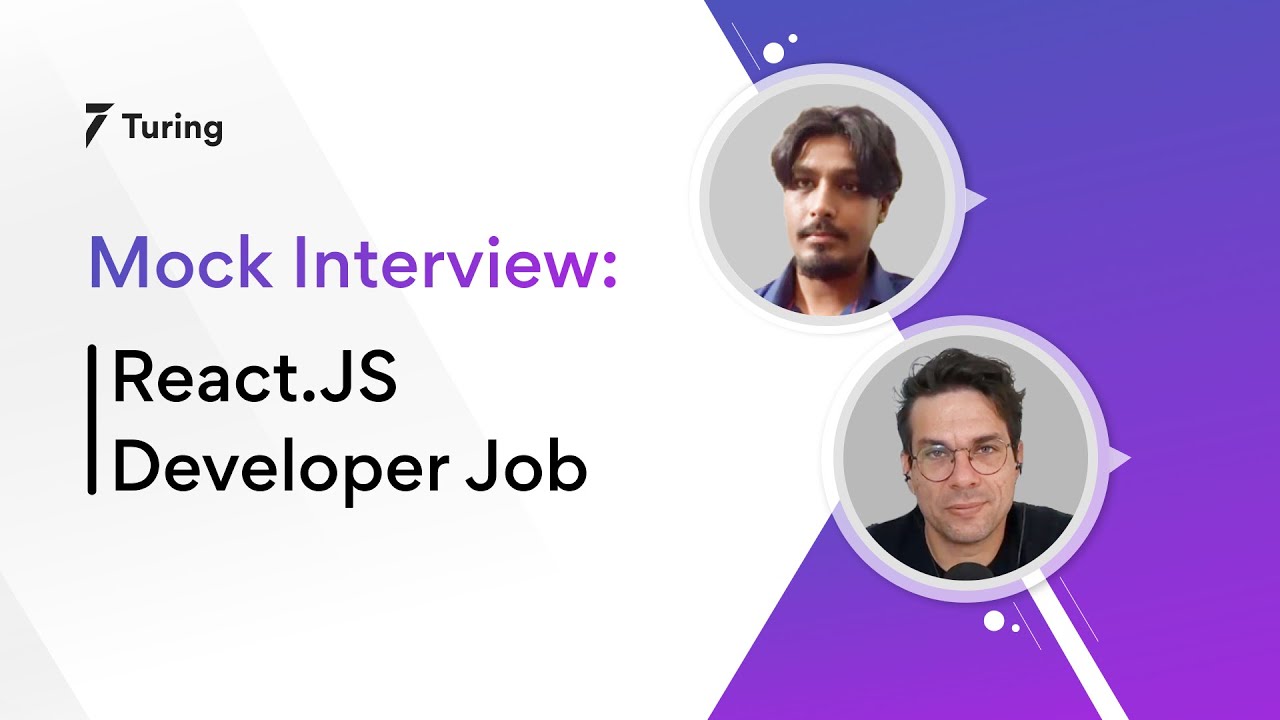
React.JS Mock Interview | Interview Questions for Senior React.JS Developers

A react interview question on counter
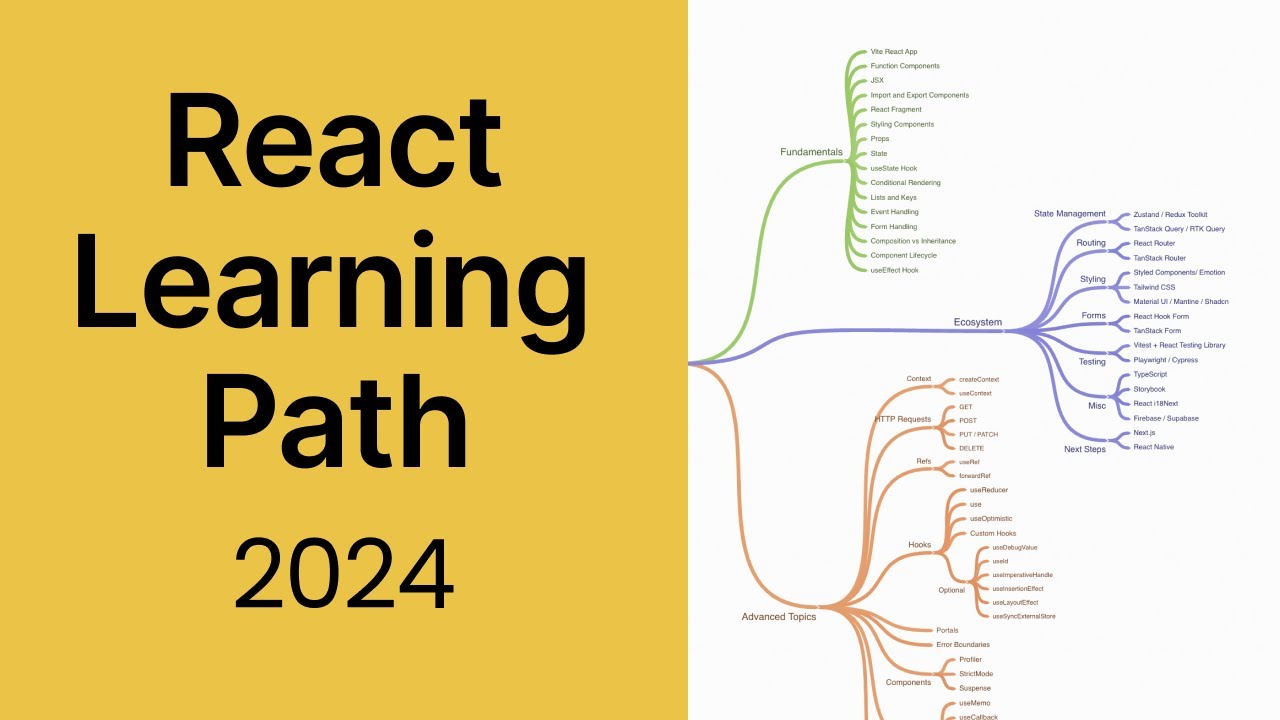
How to Master React in 2024 - The React Roadmap
5.0 / 5 (0 votes)