Pydantic Tutorial • Solving Python's Biggest Problem
Summary
TLDRThis tutorial introduces the Pydantic module in Python, highlighting its benefits for data validation and type-hinting, which address Python's dynamic typing issues. Pydantic is compared to dataclasses, showing its advantages in validation and JSON serialization. The tutorial covers creating Pydantic models, ensuring data validity, using custom validators, and converting models to/from JSON. It demonstrates Pydantic's practical use in improving code reliability and IDE support, especially for larger or collaborative projects.
Takeaways
- 🐍 Python lacks static typing, which can lead to issues in large applications and unclear function arguments.
- 🔄 Dynamic typing in Python allows for easy variable type changes, but this flexibility can cause bugs that are hard to debug.
- 👤 Pydantic is a Python library that helps with data modeling and validation, enhancing type hints and reducing runtime errors.
- 📦 Pydantic is used by popular Python modules like HuggingFace, FastAPI, and LangChain, highlighting its reliability and utility.
- 🖥️ Pydantic models provide better IDE support, including type hints and autocomplete, making development more efficient.
- 🔒 Data validation with Pydantic ensures that objects are created with valid data, preventing future failures in the application.
- 🌐 Pydantic simplifies JSON serialization, making it easy to convert models to and from JSON, which is crucial for web applications and data storage.
- 📚 Pydantic allows for custom validation logic, enabling developers to enforce specific data requirements in their models.
- 📈 Compared to dataclasses, Pydantic offers more robust features like deep JSON serialization and data validation, making it ideal for complex data models.
- 🛠️ While dataclasses are built into Python and lightweight, they lack the advanced features of Pydantic, making them suitable for simpler data needs.
Q & A
What is the main issue with Python's dynamic typing?
-Python's dynamic typing allows variables to be created without declaring their type and can be overridden with different types. This can lead to problems as applications grow, making it difficult to track variable types and causing issues with function arguments where types are not obvious. It also allows the accidental creation of invalid objects.
Why can dynamic typing be problematic in larger applications?
-In larger applications, dynamic typing can make it harder to keep track of all variables and their expected types. It can also complicate debugging when type-related errors occur, as these errors might not be immediately apparent and could happen at any point in the program.
What is Pydantic and what problem does it solve?
-Pydantic is a data validation library in Python that helps model data and solve problems associated with dynamic typing. It provides better IDE support for type hints and autocomplete, ensures data validity upon object creation, and simplifies data serialization for formats like JSON.
How does Pydantic improve IDE support?
-Pydantic enhances IDE support by providing type hints and autocomplete features. This helps developers quickly understand the expected types of variables and functions, making it easier to work with the code.
What are the benefits of using Pydantic for data validation?
-Pydantic ensures that data is validated when objects are created, reducing the risk of runtime errors due to invalid data. It also allows for the validation of complex data types, such as ensuring that an email string is valid.
How can Pydantic be used to serialize data to JSON?
-Pydantic provides built-in support for JSON serialization. To convert a Pydantic model to JSON, you can call the JSON method on the model instance, which returns a JSON string representation of the model's data. Alternatively, the dict method can be used to obtain a plain Python dictionary.
How does Pydantic handle custom validation logic?
-Pydantic allows adding custom validation logic through the use of a validator decorator. Developers can define a class function that checks for specific conditions and raises a ValueError if the conditions are not met.
What is the difference between Pydantic and Python's built-in dataclasses?
-While both Pydantic and dataclasses provide type hints, Pydantic offers additional features like data validation and deep JSON serialization. Dataclasses are built into Python and are more lightweight, but they lack the robust validation and serialization capabilities of Pydantic.
Why might someone choose Pydantic over dataclasses?
-Developers might choose Pydantic over dataclasses if they need complex data models, require extensive data validation, or need to work with a lot of external APIs and JSON serialization.
How can Pydantic be used to ensure that a field like 'account ID' is always positive?
-Pydantic can enforce field constraints like a positive account ID through the use of a validator decorator. Developers can define a custom function that checks if the value is greater than zero and raises a ValueError if it is not.
What is the recommended approach for choosing between Pydantic and dataclasses?
-The choice between Pydantic and dataclasses depends on the project's needs. Pydantic is recommended for complex data models and extensive JSON serialization, while dataclasses might suffice for simpler data models where data validation is not a priority.
Outlines
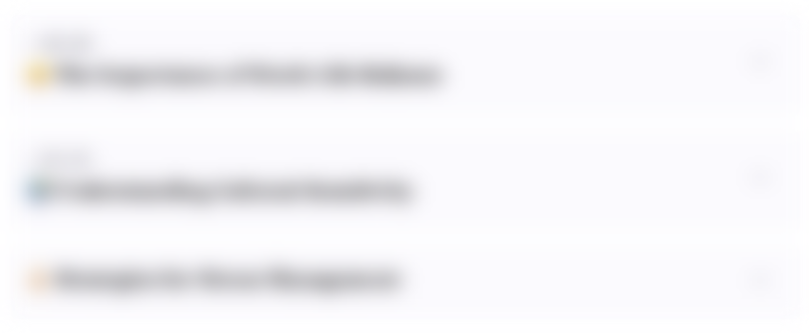
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
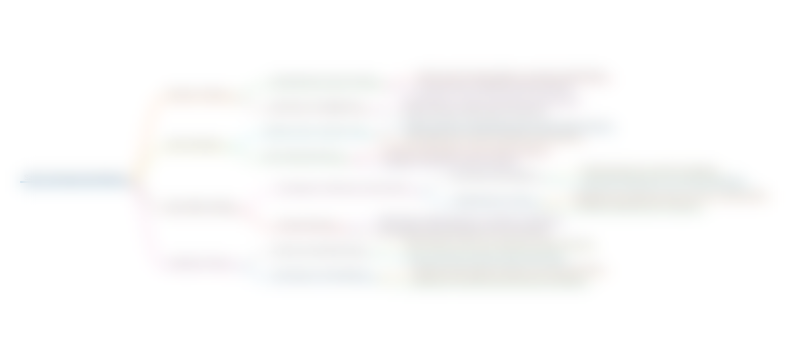
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
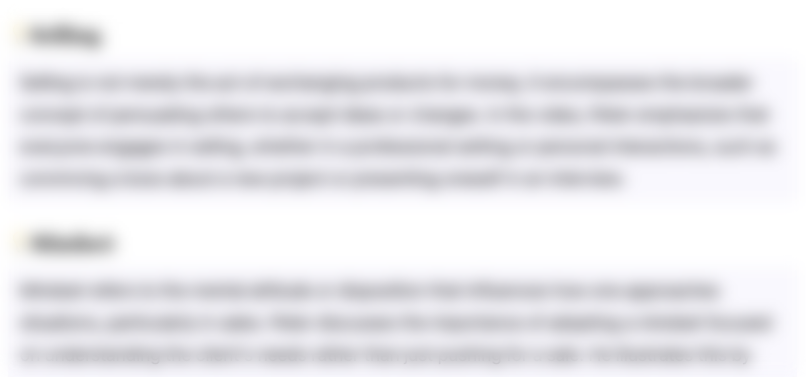
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
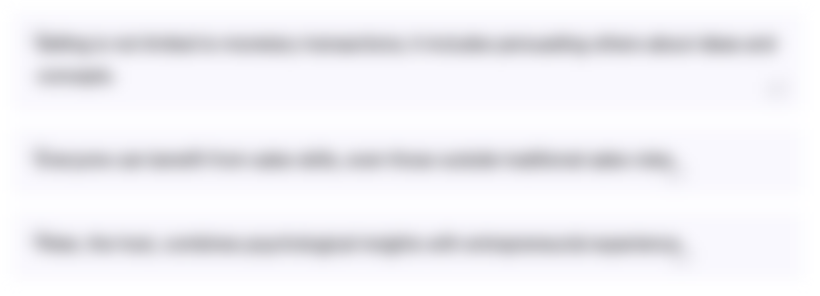
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
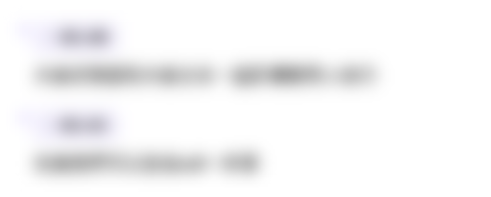
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
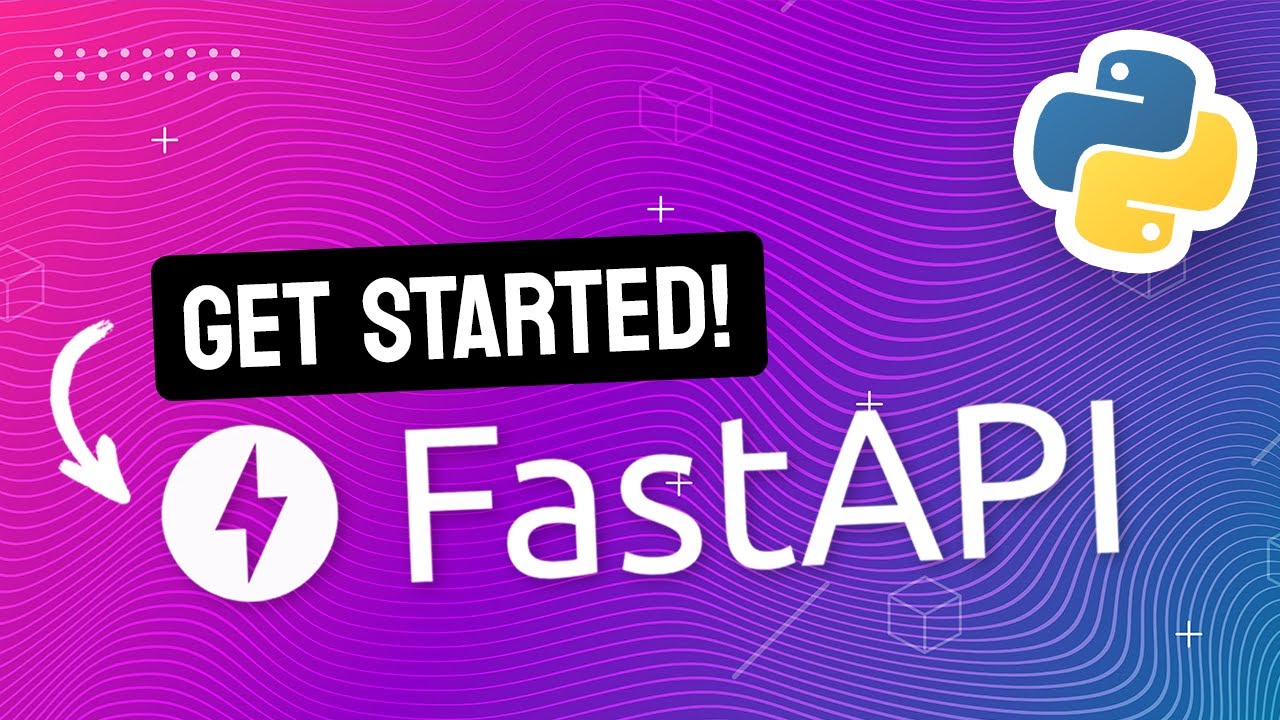
Python FastAPI Tutorial: Build a REST API in 15 Minutes
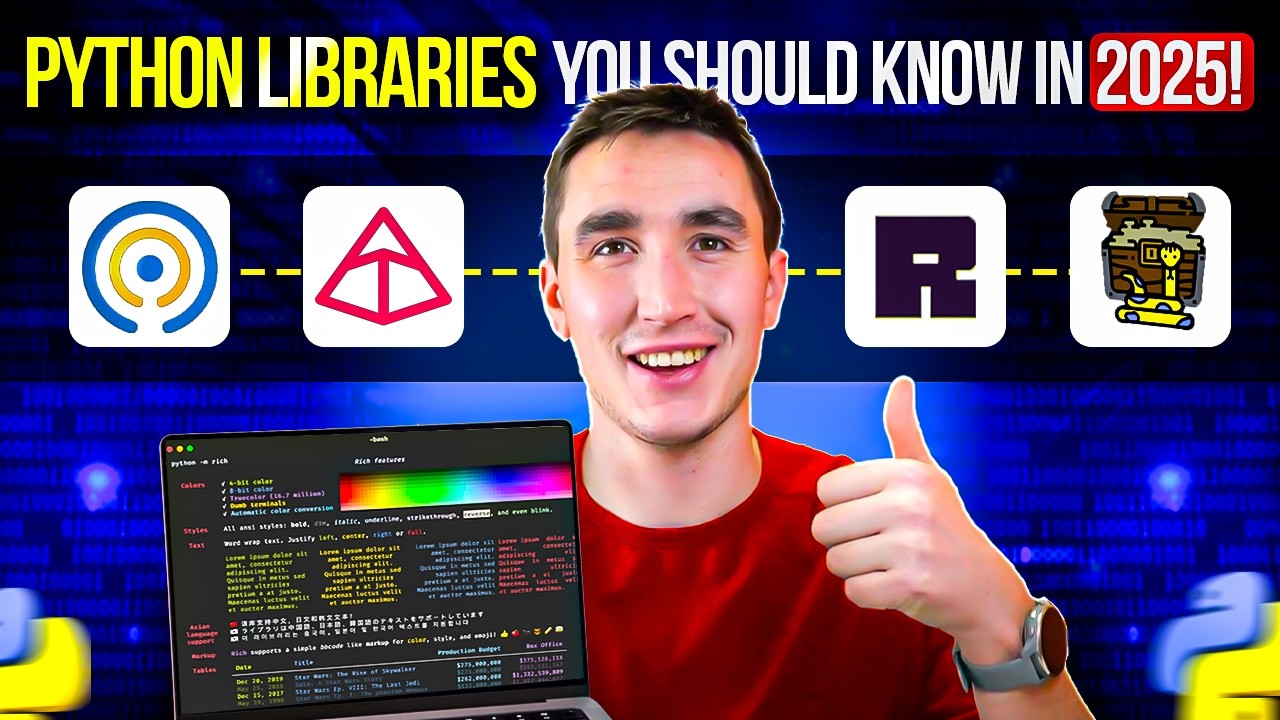
5 Python Libraries You Should Know in 2025!
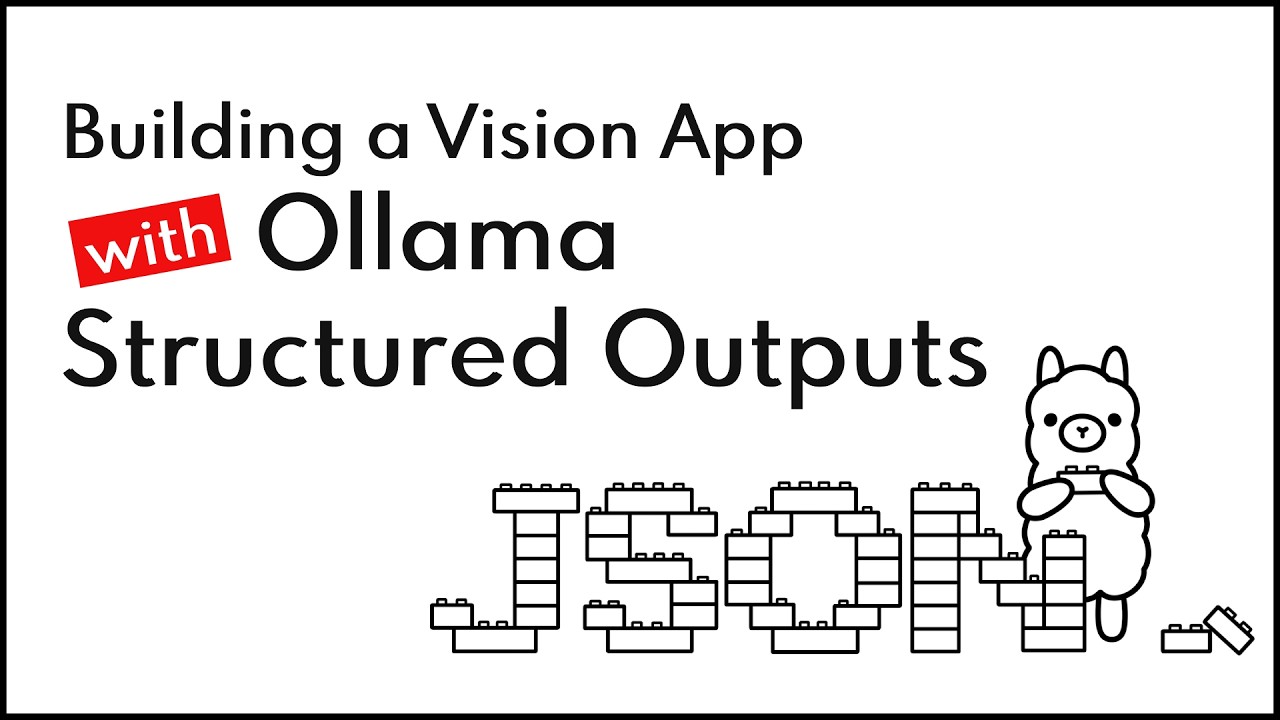
Building a Vision App with Ollama Structured Outputs
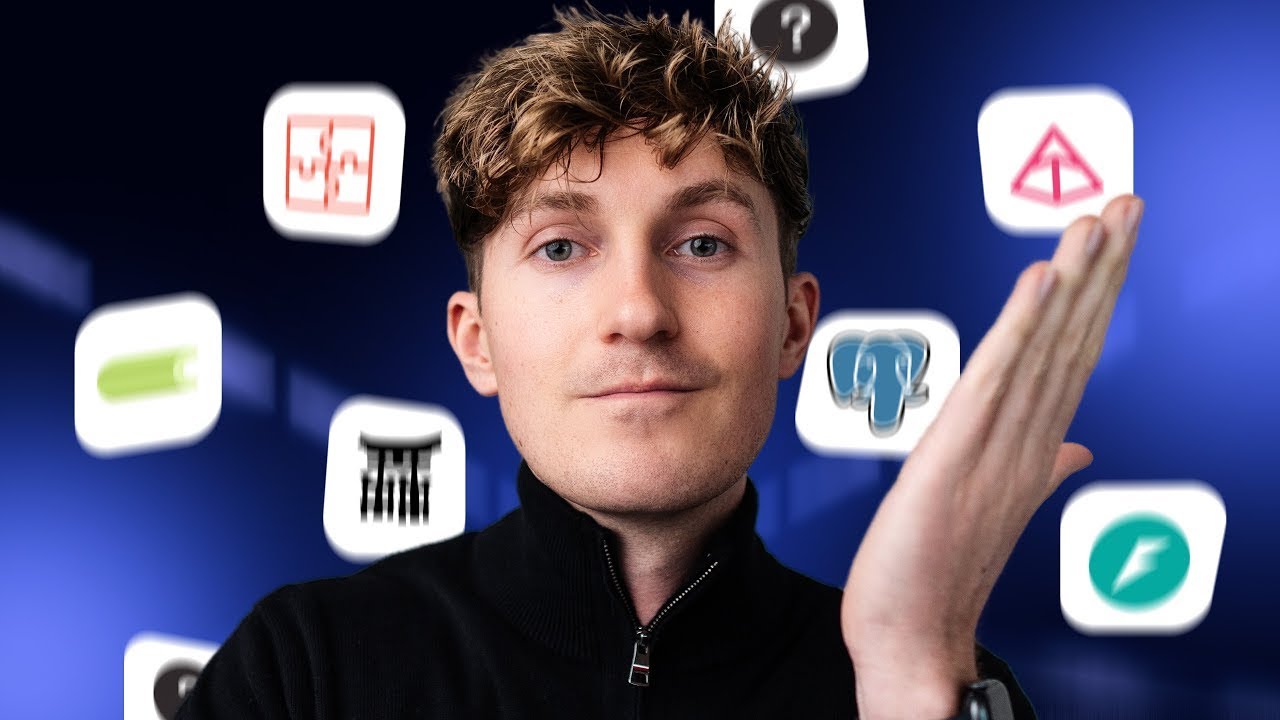
17 Python Libraries Every AI Engineer Should Know
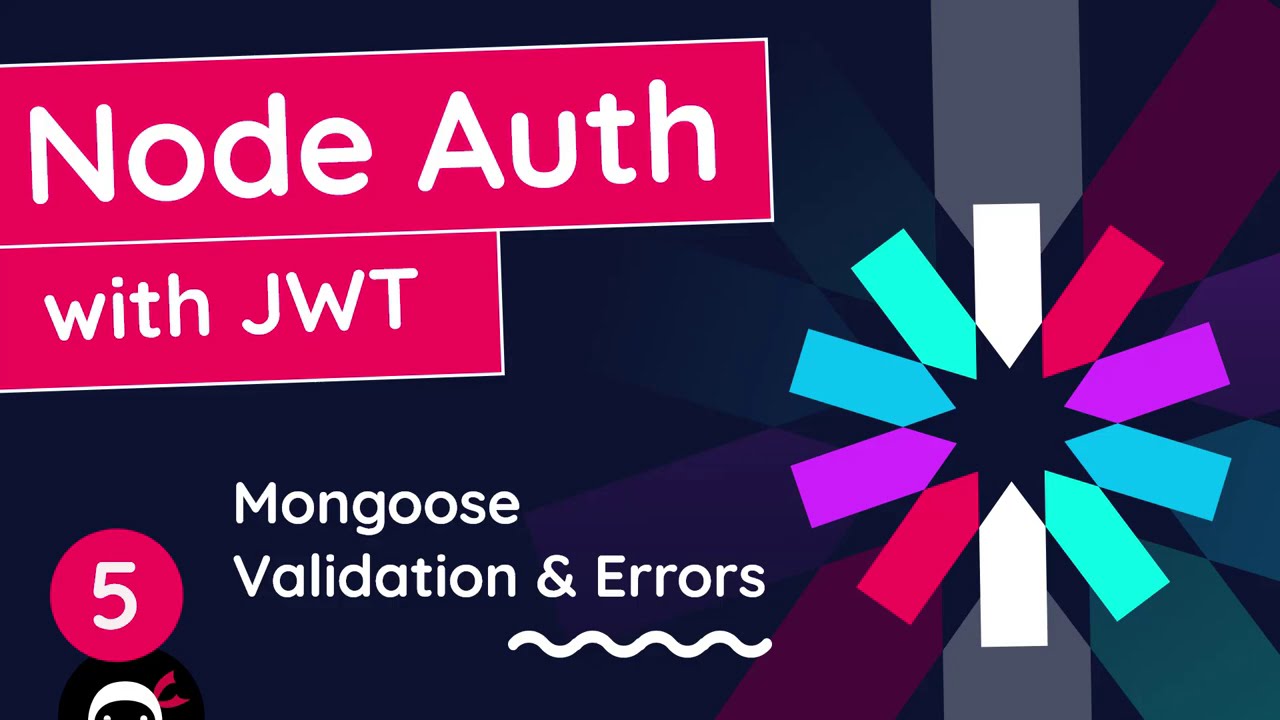
Node Auth Tutorial (JWT) #5 - Mongoose Validation
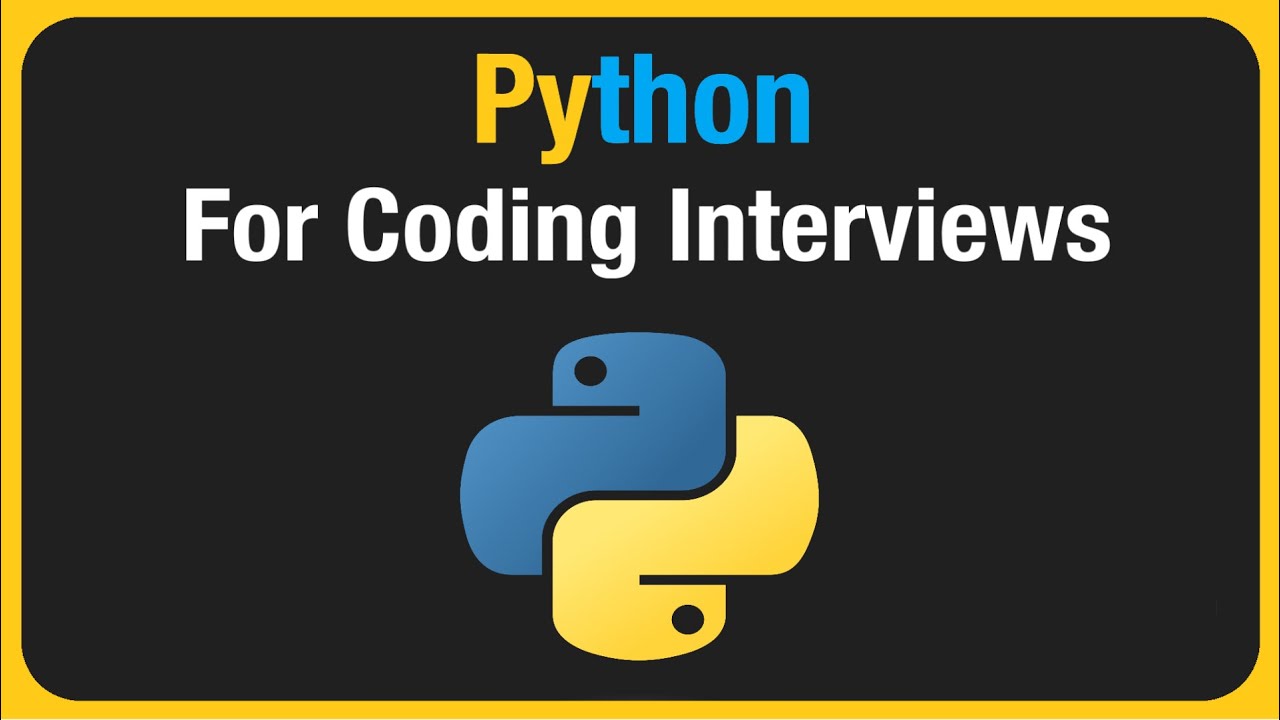
Python for Coding Interviews - Everything you need to Know
5.0 / 5 (0 votes)