P_11 Data Types in Python | Python Tutorials for Beginners
Summary
TLDRIn this video, the instructor explains Python's primitive data types, including int, float, string, and boolean, with examples. Unlike C or C++, Python treats data types as classes, and variables as instances of these classes. The `type()` function is demonstrated to check a variable's data type. Special emphasis is placed on Python's dynamic typing, where the interpreter automatically assigns the type. The video also covers concepts like binary, octal, and hexadecimal representations, string manipulation, and escape sequences. Additionally, the instructor touches on boolean logic and using conditions to evaluate expressions.
Takeaways
- π In Python, data types are classes, and variables are instances of these classes.
- π’ Python automatically determines the data type of a variable when it's assigned a value, unlike C or C++ where you have to specify the type.
- πΎ Python's integer data type can handle very large numbers limited only by the system's memory.
- π The `type()` function in Python is used to check the data type of a variable.
- π Python supports different number systems like binary, octal, and hexadecimal, which can be specified with prefixes.
- π Float numbers in Python represent real numbers and can also be represented in scientific notation with an 'e'.
- π Strings in Python are sequences of characters and can be defined using single or double quotes.
- π Boolean data type in Python has only two values: `True` and `False`, and they must be capitalized correctly.
- π Concatenation in Python works with strings but not with incompatible types like integers and strings.
- βοΈ Special characters in strings can be escaped using a backslash (`\`) to print them as they are, without their special meaning.
Q & A
What are the primitive data types in Python mentioned in the video?
-The primitive data types in Python mentioned are int (integer), float, string (str), and boolean (bool).
How are data types in Python different from C or C++?
-In Python, data types are considered as classes, and variables are instances or objects of these classes. Unlike C or C++, Python does not require explicit declaration of data types; the type is inferred automatically based on the value assigned.
How do you check the data type of a variable in Python?
-You can check the data type of a variable using the `type()` function. For example, `print(type(variable_name))` will display the data type of the variable.
What is the significance of prefixes like 0b, 0o, and 0x in Python?
-Prefixes like 0b, 0o, and 0x are used to represent binary, octal, and hexadecimal numbers, respectively. For example, `0b10` is binary for 2, `0o10` is octal for 8, and `0x10` is hexadecimal for 16.
Is there any limit on the length of an integer in Python?
-There is no limit on the length of an integer in Python, except for the constraints imposed by the available memory of the system.
How does Python handle floating-point numbers?
-Python represents floating-point numbers as decimal numbers or fractions. You can also represent them using scientific notation, such as `1.5e3` for 1500. These are classified as the `float` data type.
Can you concatenate an integer and a string in Python?
-No, you cannot directly concatenate an integer and a string in Python. Attempting to do so will result in a TypeError. To concatenate them, you need to convert the integer to a string using `str()`, for example: `str(123) + 'abc'`.
How do you escape special characters like single and double quotes in Python strings?
-You can escape special characters like single and double quotes by using a backslash (\) before the quote. For example, `'Jenny\'s book'` will allow you to use an apostrophe inside a single-quoted string.
What is the difference between a string defined with single quotes and one with double quotes in Python?
-There is no functional difference between using single or double quotes for defining strings in Python. However, using one type allows you to include the other type inside the string without needing to escape it, e.g., `"Jenny's"` or `'He said, "Hello"'`.
What is the boolean data type, and how is it used in Python?
-The boolean data type (`bool`) in Python has two values: `True` and `False`. It is often used in conditions and logical operations. For example, `a < b` will return `True` or `False` depending on the comparison result.
What is the error message if you attempt to use 'true' instead of 'True' in Python?
-If you use 'true' (lowercase) instead of 'True' (uppercase) in Python, you'll get a NameError, as Python is case-sensitive and recognizes only 'True' with a capital 'T' as a boolean value.
Outlines
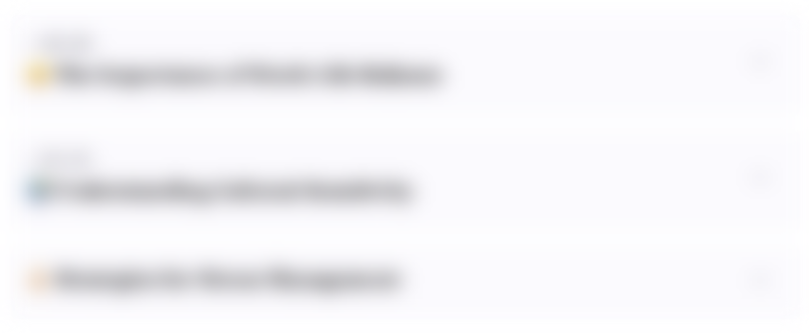
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
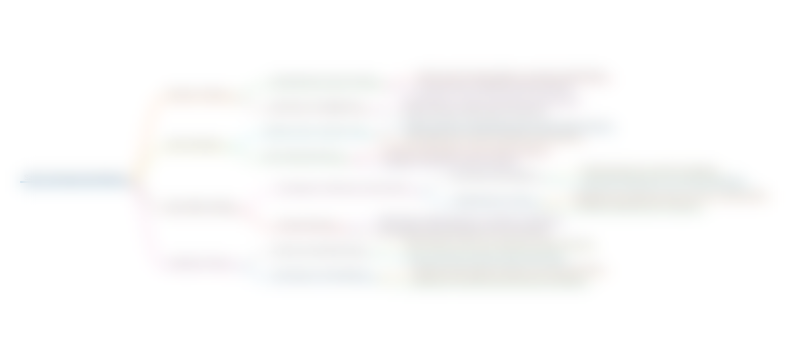
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
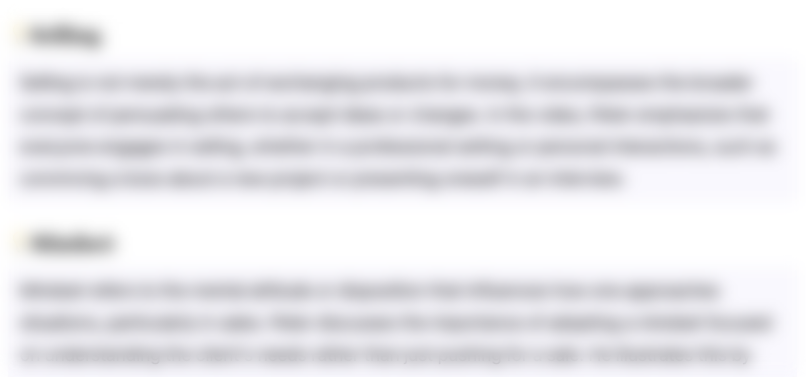
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
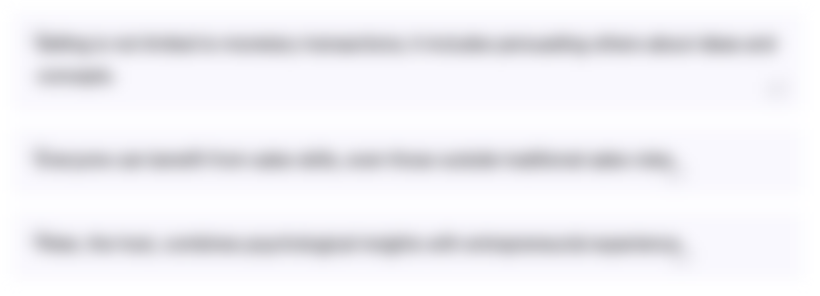
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
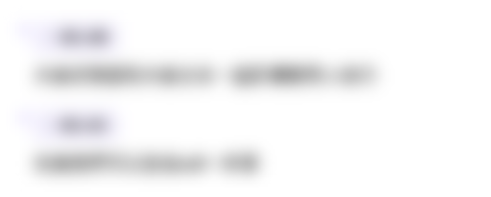
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)