05. Controllers - Laravel 11 tutorial for beginners
Summary
TLDRIn this instructional video, Tony introduces the concept of using controllers in Laravel to streamline and organize web application development. He demonstrates the process of creating a 'PostController' using Artisan commands, explaining the benefits of controllers for code maintainability and clarity. Tony also illustrates how to define routes for CRUD operations within a controller, transitioning from anonymous functions to a more structured approach. The video concludes with a clean routing setup and an overview of the controller methods, encouraging viewers to subscribe for more informative content.
Takeaways
- π The video discusses the use of routes and controllers in web development with Laravel.
- π§ In the script, the presenter demonstrates how to clean up and organize code by using controllers instead of anonymous functions for handling requests like POST, PUT, and DELETE.
- π Controllers act as middlemen between incoming requests and application logic, grouping related methods for handling specific types of requests.
- π The 'make:controller' Artisan command in Laravel is used to generate new controller classes for organizing code better.
- π The presenter creates a 'PostController' to handle all post-related requests, such as creating, updating, and deleting posts.
- π The script explains the difference between various types of controllers like 'empty', 'resource', and 'invocable', with 'resource' being chosen for CRUD operations.
- π The presenter shows how to bind models directly to controller methods, which simplifies the process of finding and displaying specific resources.
- π The 'route:list' Artisan command is used to display all defined routes in the application.
- π The 'route:resource' Artisan command is introduced as a shortcut to automatically create routes for a resourceful controller.
- π The script also touches on the use of route names for better route identification and maintenance.
- π Lastly, the presenter mentions the use of 'route:view' for returning views directly without the need for a controller when the logic is simple.
Q & A
What is the main topic of the video?
-The main topic of the video is about using controllers in Laravel to manage web routes and requests more efficiently and maintainably.
Why are anonymous functions not recommended for handling requests in real-world applications?
-Anonymous functions are not recommended because they can lead to large and unmanageable files when dealing with complex logic, such as validation and data manipulation, which are common in real-world applications.
What is a controller in Laravel?
-In Laravel, a controller is a class that acts as a middleman between incoming requests and the application's logic, grouping functions (methods) that handle specific requests.
What command is used to create a new controller in Laravel?
-The command 'php artisan make:controller [ControllerName]' is used to create a new controller in Laravel.
What are the different types of controllers that can be generated in Laravel?
-The different types of controllers that can be generated in Laravel are 'empty', 'resource', 'api', and 'invocable'.
What does the 'resource' type controller include?
-The 'resource' type controller includes methods for all the standard CRUD operations: index, create, store, show, edit, update, and destroy.
What is the purpose of using a model when creating a resource controller?
-Using a model when creating a resource controller helps to automatically inject the model into the controller methods, simplifying the code and making it more efficient.
How can you assign a specific model to a resource controller during its creation?
-You can assign a specific model to a resource controller by specifying the model's name after choosing the 'resource' type, for example, 'php artisan make:controller PostController --model=Post'.
What is the benefit of using the 'route:list' command in Laravel?
-The 'route:list' command in Laravel is beneficial for listing all the registered routes in the application, which helps in understanding and debugging the routing system.
How can you simplify the routing definition for a resource in Laravel?
-You can simplify the routing definition for a resource in Laravel by using the 'Route::resource' method, which automatically creates the necessary routes for the specified resource.
What is the purpose of the 'Route::view' method in Laravel?
-The 'Route::view' method in Laravel is used to return a view directly in response to a request, which is useful for simple routes that do not require complex logic.
Outlines
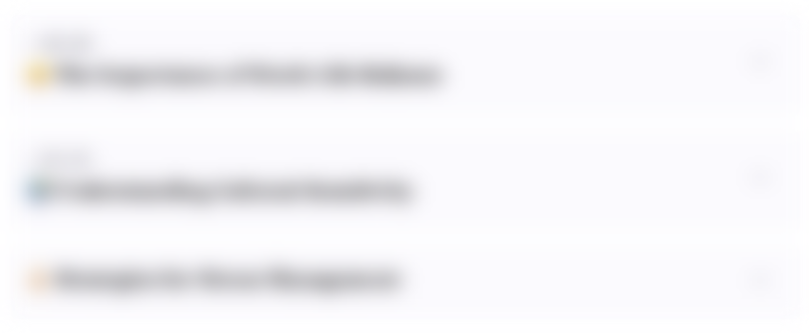
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
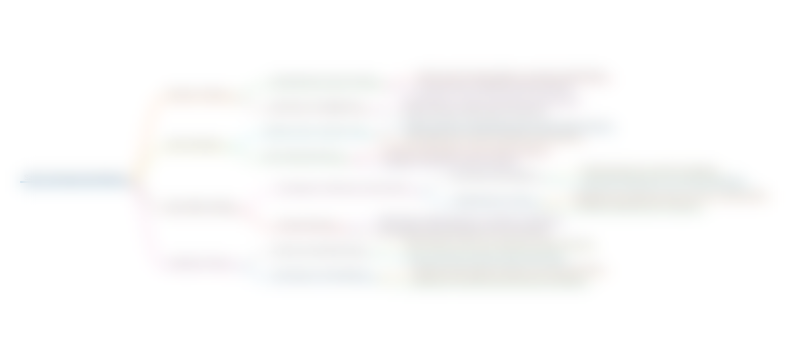
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
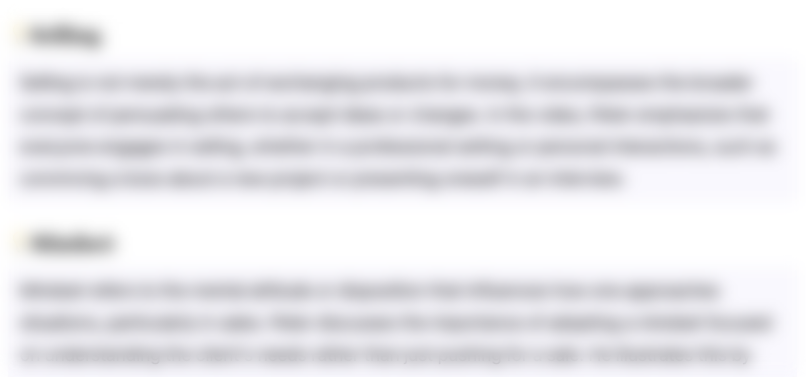
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
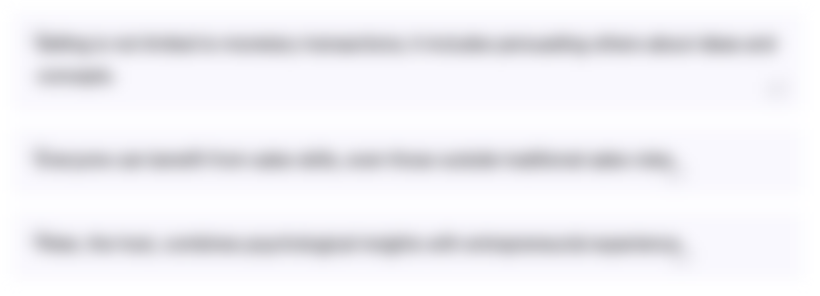
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
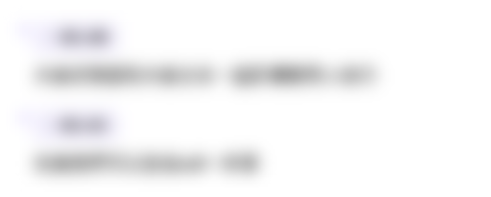
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)