Swift in 100 Seconds
Summary
TLDRSwift, a multi-paradigm, compiled language by Apple, was introduced in 2014 as a modern successor to Objective-C. It offers a more readable syntax with features like memory safety and type inference, enhancing developer productivity. Swift is versatile, supporting iOS, macOS, watchOS app development, and is open source, allowing use beyond Apple platforms. It compiles to native machine code, supports a playground for experimentation, and provides automatic memory management. Swift is beginner-friendly, with functions as first-class objects, and supports object-oriented programming. To get started, simply install Swift, create a .swift file, and begin coding in the global scope without a main function.
Takeaways
- 🍏 Swift is a multi-paradigm, compiled language developed by Apple.
- 📅 Introduced in 2014, Swift was designed to succeed Objective-C, which was used since the 1980s.
- 🔗 Swift interoperates with Objective-C but offers a more concise and readable syntax.
- 🛡️ It provides features like memory safety and type inference to enhance developer productivity.
- 📱 Swift is used for developing mobile apps on iOS, desktop apps on macOS, and wearable apps for watchOS.
- 🌐 As an open-source language, Swift can be used to build software beyond Apple platforms.
- 💻 It compiles to native machine code and is built on the LLVM toolchain like other modern languages.
- 🎨 Swift supports a 'playground' feature for experimenting with code without recompilation, making it beginner-friendly.
- 🔒 It ensures memory safety by preventing unsafe code by default and uses automatic reference counting for memory management.
- 📝 Variables in Swift are declared with 'var' for mutable and 'let' for immutable, with optional types indicated by a '?'.
- 🔑 Functions are declared with 'func', use named parameters by default, and can be manipulated as first-class objects.
- 🚀 To get started with Swift, install it, create a .swift file, and begin coding in the global scope without a 'main' function.
Q & A
What is Swift and why was it created?
-Swift is a multi-paradigm, compiled programming language developed by Apple. It was introduced in 2014 as a modern successor to Objective-C, the original language for Apple platforms, to provide a more readable syntax, memory safety, and type inference to improve developer productivity.
How does Swift interoperate with Objective-C?
-Swift can interoperate with Objective-C, allowing developers to use both languages in the same project and access Objective-C libraries and frameworks within Swift code.
What platforms can Swift be used to build applications for?
-Swift is used to build mobile apps on iOS, desktop apps on macOS, and wearable apps for watchOS. Being open source, it can also be used to build software outside of Apple platforms.
How does Swift handle memory management?
-Swift provides memory safety by preventing the writing of unsafe code by default and uses automatic reference counting to manage memory automatically, which simplifies memory management for developers.
What is the LLVM tool chain and how is it related to Swift?
-The LLVM tool chain is a set of modular and reusable compiler and toolchain technologies. Swift compiles to native machine code and is built on top of the LLVM tool chain, like many other modern languages.
What is a playground in the context of Swift?
-A playground in Swift is an interactive environment that allows developers to experiment with code without needing to recompile. It supports a readable print loop, making it a great tool for beginners to learn and test code snippets.
How do you define a variable in Swift?
-In Swift, you can define a variable with the 'var' keyword for mutable variables or 'let' for immutable variables or constants. Variables must be initialized with a value, and type inference is used to strongly type the value unless explicitly specified.
What is the significance of optional types in Swift?
-Optional types in Swift, indicated by a question mark '?', allow a variable to contain a 'nil' value. This feature helps in safely handling the absence of a value and is integral to Swift's approach to error handling and safety.
How are functions declared in Swift?
-Functions in Swift are declared with the 'func' keyword and use named parameters by default. Positional arguments can be used by placing an underscore before the parameter name. Functions are first-class objects and can be passed as arguments, returned from other functions, or nested to create closures.
What does it mean for functions to be first-class objects in Swift?
-In Swift, functions being first-class objects means they can be treated like any other value. You can pass functions as arguments to other functions, assign them to variables, store them in data structures, and return them as values from other functions.
How can you compile Swift code into an executable?
-To compile Swift code into a high-performance executable, you can use the Swift compiler by running it from the terminal. This process converts the source code into a format that can be executed on the target platform.
Outlines
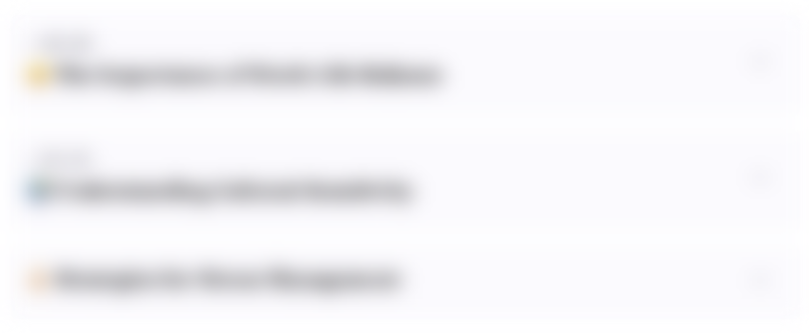
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
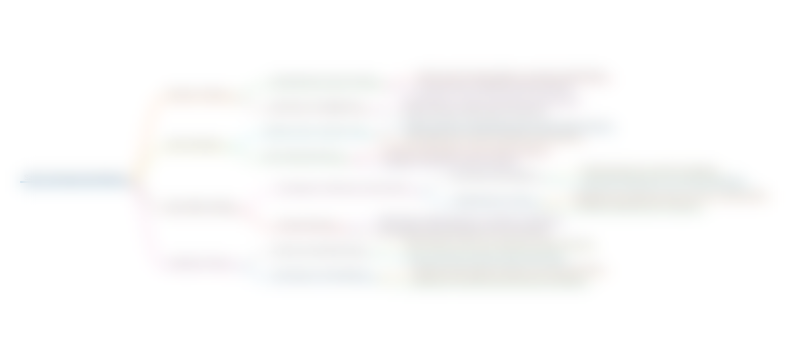
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
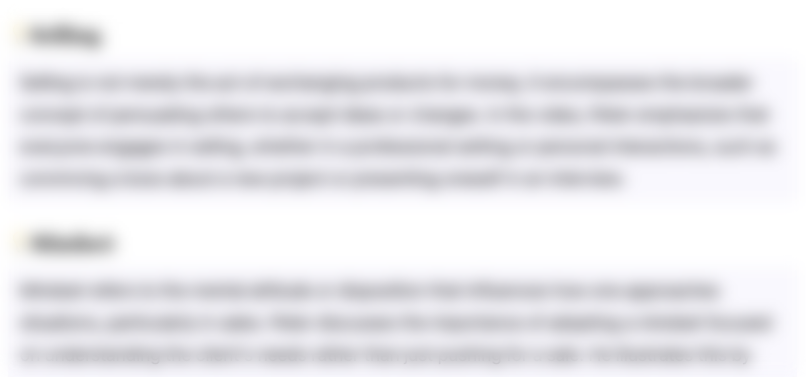
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
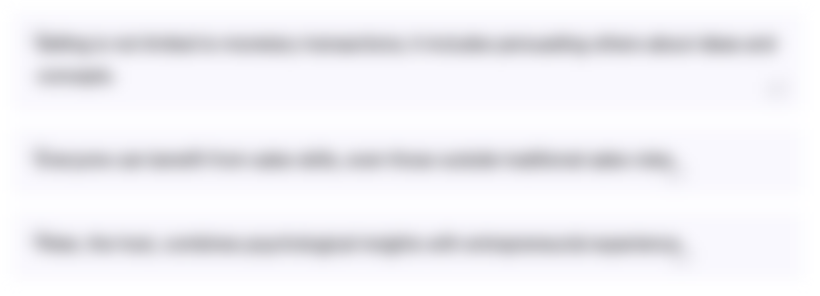
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
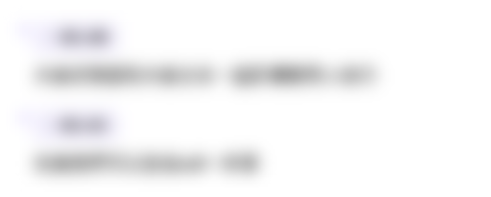
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)