Find Median from Data Stream - Heap & Priority Queue - Leetcode 295
Summary
TLDRIn this coding tutorial, the presenter tackles the problem of finding the median from a data stream, a common challenge in data structures. The solution involves using two heaps—a max heap for the lower half and a min heap for the upper half of the data. The video explains how to maintain these heaps to ensure the median can be found efficiently, with insertions and median retrieval operations having time complexities of O(log n) and O(1), respectively. The presenter guides viewers through the algorithm and provides a Python code implementation, highlighting the importance of balancing the heaps and correcting for Python's lack of a built-in max heap.
Takeaways
- 😀 The video discusses a method to find the median from a data stream, which is a challenging problem but manageable with the right data structure.
- 🔍 The median is defined as the middle value in a sorted list of integers; for even-sized lists, it's the average of the two middle values.
- 💡 The solution involves designing a data structure that supports two operations: adding a number to the data stream and finding the median from the existing numbers.
- 📈 The naive approach to solving this problem is to insert elements in order within a sorted array, which is inefficient with a time complexity of O(n) per insertion.
- 🚀 An improved approach uses two heaps to maintain the data stream, allowing for more efficient median finding and number addition.
- 📚 The two heaps are a max heap (for the smaller half of numbers) and a min heap (for the larger half), with the heaps balancing each other in size.
- 🔄 To maintain the heaps, when a number is added, it's first added to the max heap, and then the heaps are rebalanced if necessary, ensuring all elements in the max heap are less than or equal to those in the min heap.
- 📉 The rebalancing involves moving elements between the heaps to keep their sizes approximately equal and to maintain the ordering property.
- ⏱ The time complexity for adding a number is improved to O(log n) using heaps, whereas finding the median remains an O(1) operation.
- 👨💻 The video provides a walkthrough of the algorithm with examples, demonstrating the process of adding numbers to the heaps and finding the median.
- 🛠️ The final part of the video presents the actual code implementation in Python, highlighting the use of negative values to simulate a max heap, which is not natively supported in Python's standard library.
Q & A
What is the definition of the median in a sorted integer list?
-The median is defined as the middle value in a sorted integer list. If the list has an even number of elements, the median is the average of the two middle values.
What are the two main operations required in the data structure discussed in the video?
-The two main operations are adding a number to the data stream and finding the median of the list.
Why is maintaining a sorted array for finding the median not efficient?
-Maintaining a sorted array requires O(n) time to insert each new element, where n is the number of elements in the array. This makes the operation inefficient for large data streams.
How does the speaker propose to optimize the process of finding the median?
-The speaker proposes using two heaps: a max heap for the smaller half of the numbers and a min heap for the larger half. This allows for efficient insertion and median retrieval.
What is the role of the max heap and the min heap in this solution?
-The max heap (small heap) stores the smaller half of the numbers, and the min heap (large heap) stores the larger half. The max heap allows for quick access to the largest element of the smaller half, and the min heap allows for quick access to the smallest element of the larger half.
How is the balance between the two heaps maintained?
-The balance is maintained by ensuring that the sizes of the two heaps are approximately equal. If the difference in sizes becomes greater than one, elements are moved between the heaps to balance them.
What is the time complexity of adding a number to the heaps?
-The time complexity of adding a number to the heaps is O(log n), where n is the number of elements in the heaps.
How is the median found in constant time using the two-heap method?
-The median is found by accessing the maximum element from the max heap and the minimum element from the min heap. This access is done in O(1) time due to the properties of heaps.
Why does the speaker multiply numbers by negative one when using heaps in Python?
-In Python, the heapq module only implements min heaps. To simulate a max heap, numbers are multiplied by negative one so that the smallest element in the heapq min heap corresponds to the largest element in the original list.
What is the final code structure for the solution in Python?
-The final code structure includes initializing two heaps (small and large), defining the addNum function to handle insertion and balancing, and defining the findMedian function to retrieve the median in constant time.
Outlines
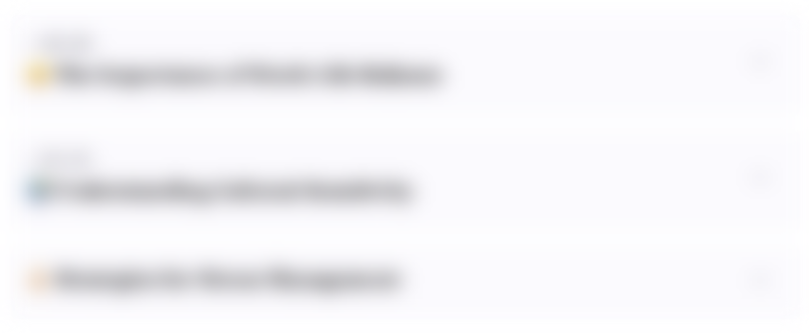
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
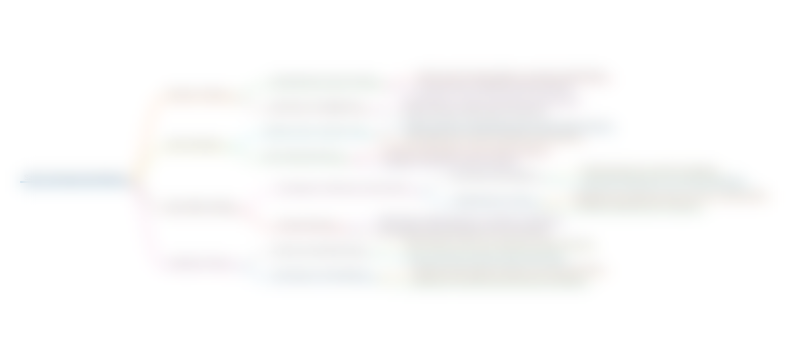
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
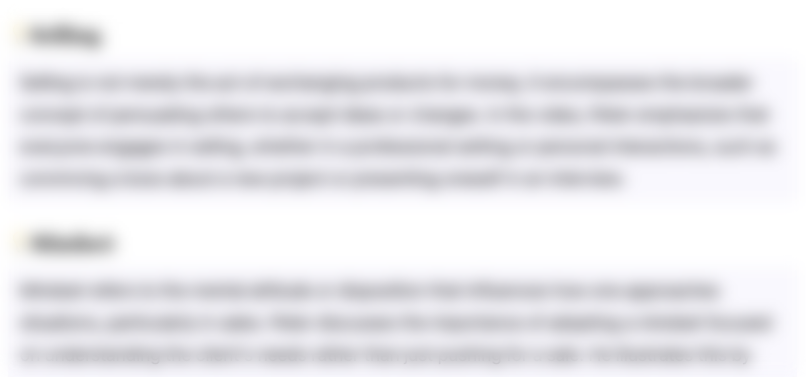
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
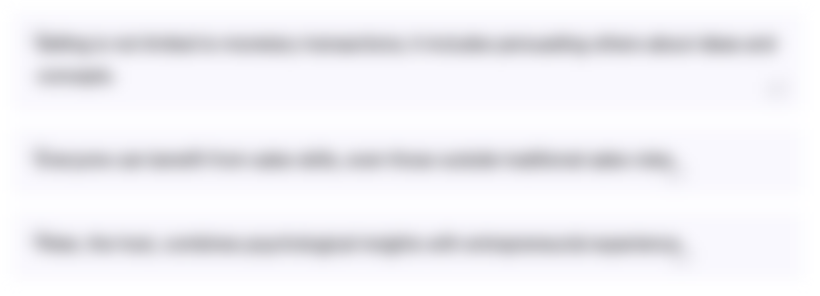
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
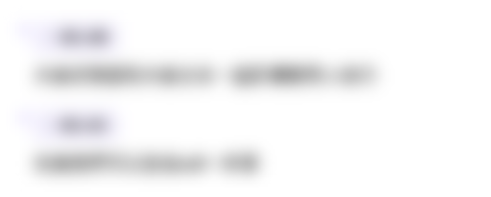
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
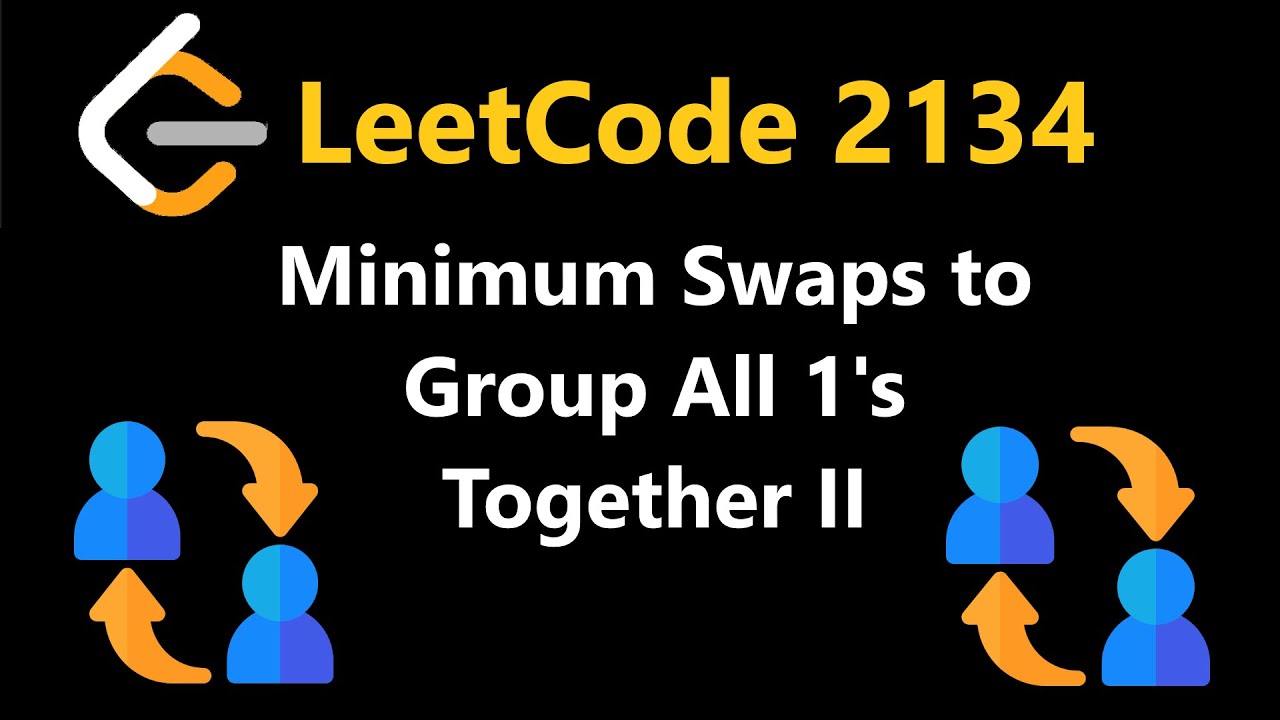
Minimum Swaps to Group All 1's Together II - Leetcode 2134 - Python
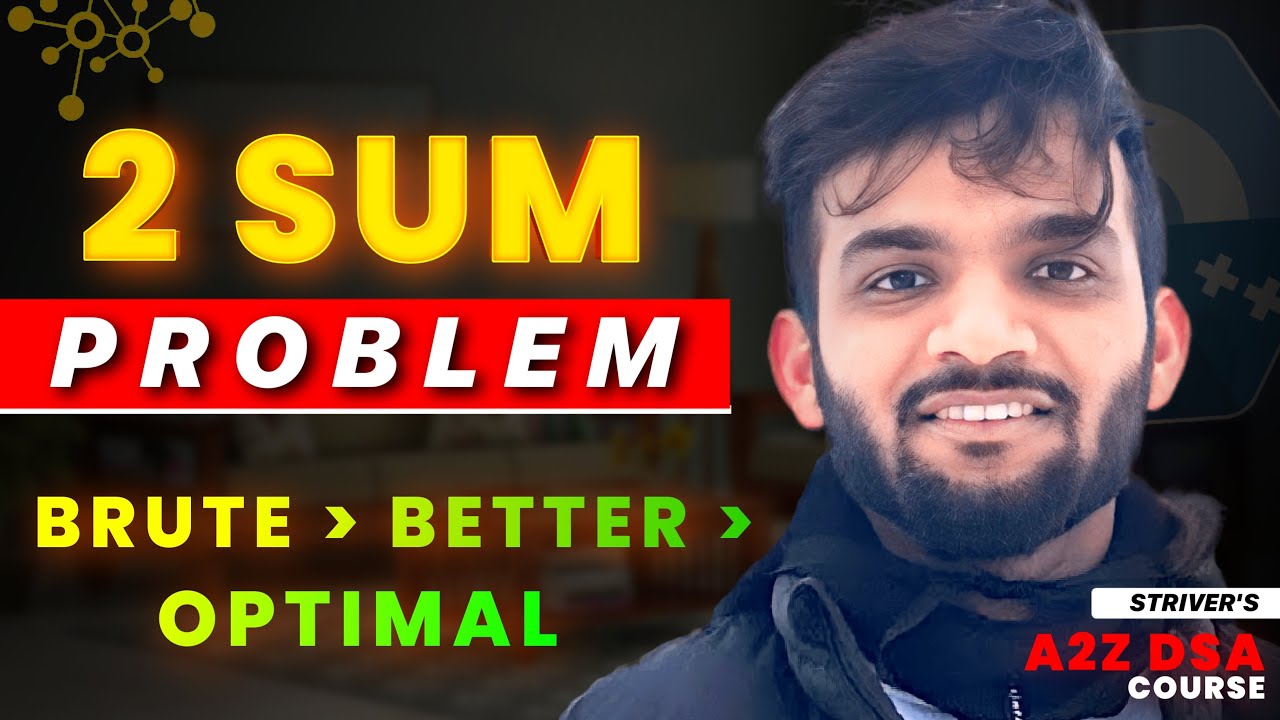
2 Sum Problem | 2 types of the same problem for Interviews | Brute-Better-Optimal

STATISTIKA || Mean, median dan Modus data tunggal || Data ganjil dan data genap

Interquartile Range (IQR) | Math with Mr. J
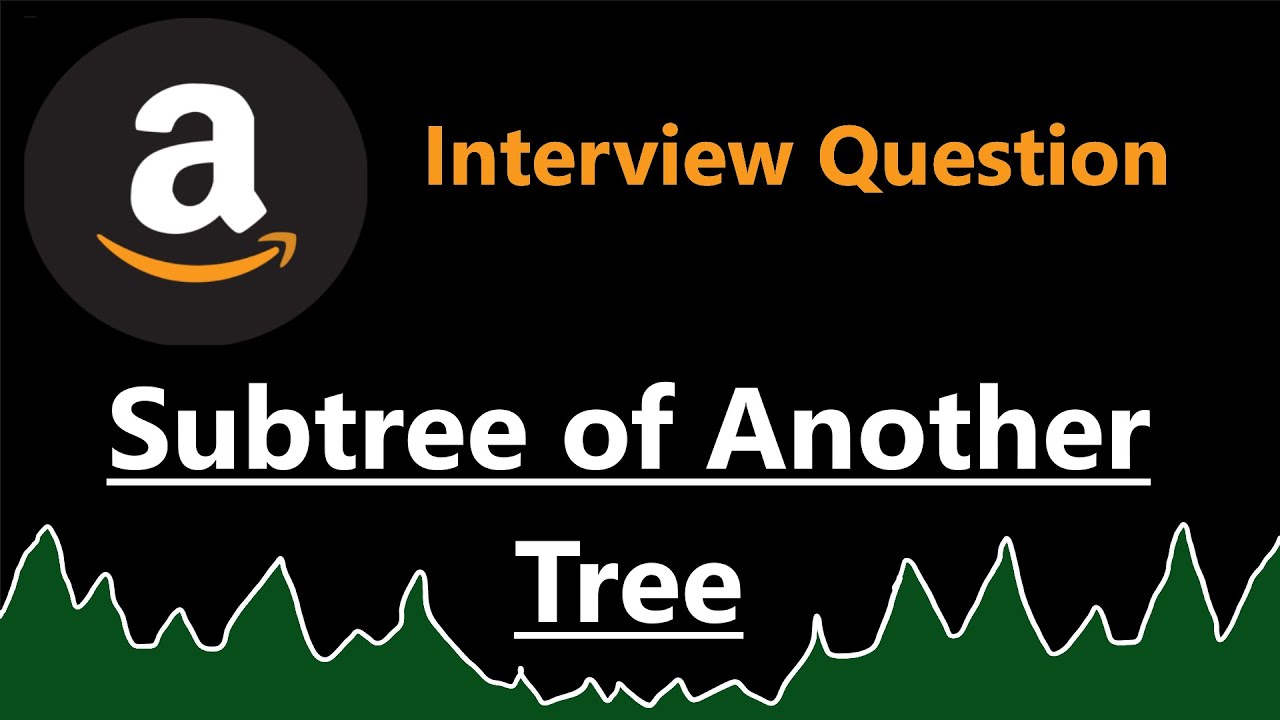
Subtree of Another Tree - Leetcode 572 - Python
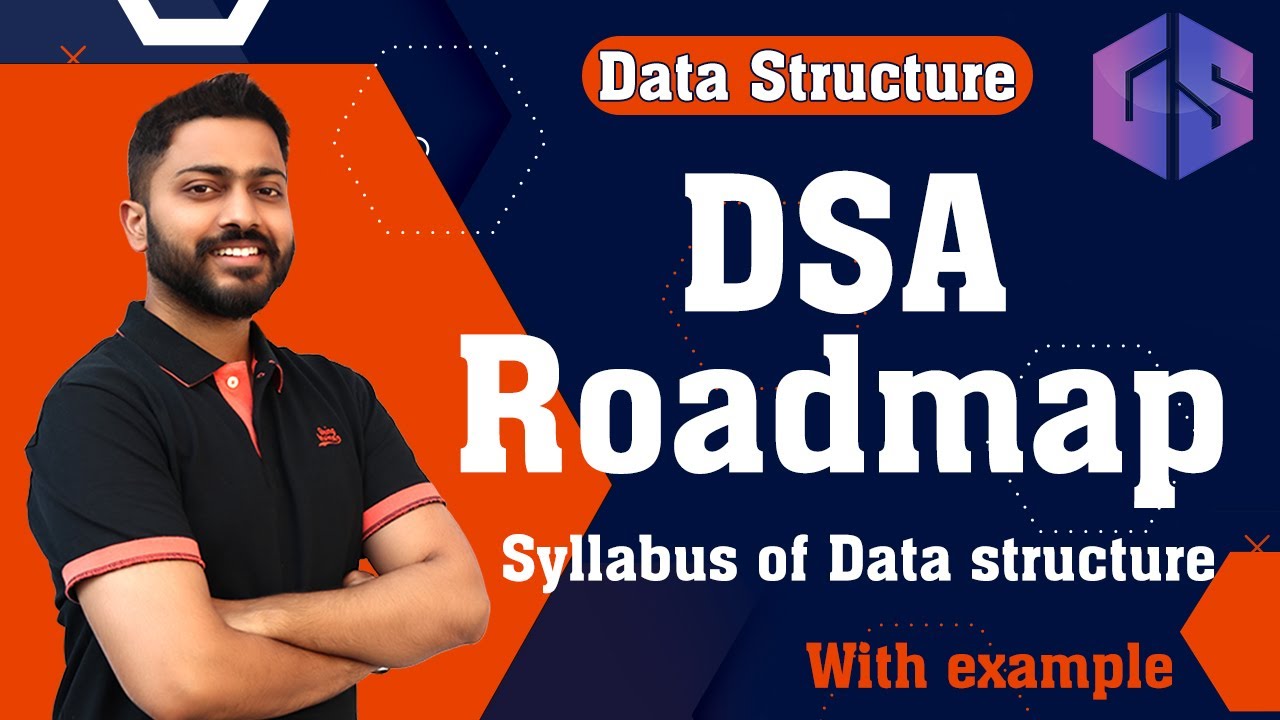
Roadmap 🛣️ of DSA | Syllabus of Data structure | Data Structure for Beginners
5.0 / 5 (0 votes)