I gave 127 interviews. Top 5 Algorithms they asked me.
Summary
TLDRThis video script by Sahil offers insights into the top 5 algorithms frequently asked in coding interviews: the 'top k elements', 'sliding window', 'backtracking', 'dynamic programming', and graph traversal techniques like 'Breadth First Search' and 'Depth First Search'. It emphasizes that mastering a few key algorithms can significantly improve interview performance, as they are often the basis for solving a variety of problems. The script also touches on the importance of understanding data structures and provides links to related code examples for further learning.
Takeaways
- 📘 The speaker emphasizes that knowing every algorithm is not necessary to succeed in coding interviews, highlighting the 80-20 rule where 20% of algorithms are asked in 80% of interviews.
- 🔍 The top 5 algorithms frequently asked in interviews are to be discussed, with specific problems where they apply, aiming to help viewers identify the most important algorithms.
- 🔑 The 'top k elements' algorithm is crucial for various problems like finding the k largest/smallest numbers in an array or the k most frequent numbers, and can be efficiently solved using a heap data structure.
- 🔠 The 'Sliding window' algorithm is applicable to problems involving substrings or subarrays with certain constraints, and involves expanding and contracting a window while tracking characters or elements.
- 🌐 Backtracking is a method for exploring all possible solutions by building them step by step and is often implemented using recursion, as demonstrated by the Combination sum problem.
- 💡 Dynamic Programming is an approach that breaks a problem into smaller subproblems and utilizes the solutions of these subproblems to efficiently solve the original problem, as opposed to recalculating them.
- 🌟 Breadth First Search (BFS) and Depth First Search (DFS) are fundamental graph traversal algorithms, with BFS using a queue and DFS using a stack to explore vertices systematically.
- 🛣️ BFS is particularly useful for finding the shortest path between two vertices in a graph, while Dijkstra’s algorithm and Topological sort are related concepts worth exploring.
- 📚 Mastering Data Structures is essential for understanding and effectively applying algorithms, as they form the foundation for solving complex problems in coding interviews.
- 🎓 The speaker, Sahil, suggests that viewers watch another video for insights on mastering Data Structures and Algorithms, indicating a broader learning resource.
- 📈 The script suggests that while the top 5 algorithms are important, a comprehensive understanding of algorithms and data structures is necessary to excel in coding interviews.
Q & A
What is the 80-20 rule or Pareto’s principle in the context of coding interviews?
-The 80-20 rule, or Pareto’s principle, as mentioned in the script, suggests that 20% of algorithms are asked in 80% of coding interviews. This principle is used to emphasize that mastering a smaller subset of algorithms can significantly increase one's chances of success in interviews.
Why is the 'top k elements' algorithm important in coding interviews?
-The 'top k elements' algorithm is important because it appears in various types of problems such as finding the k largest or smallest numbers in an array or the k most frequent numbers. It's also used in 'Sliding Window' problems, making it a versatile and commonly asked algorithm in interviews.
What is the time complexity of finding the top k largest numbers in an array using sorting?
-Sorting the array and then taking the k largest elements has a time complexity of O(nlogn), where n is the number of elements in the array. This is because the sorting operation itself typically takes O(nlogn) time.
How does the 'top k elements' algorithm improve upon the time complexity of sorting?
-The 'top k elements' algorithm uses a heap data structure to maintain the top k largest numbers, which has a time complexity of O(nlogK). This is more efficient than sorting the entire array, especially when k is much smaller than n.
Can you explain the 'Sliding window' algorithm using the Largest Substring without repeating characters problem?
-The 'Sliding window' algorithm for the Largest Substring without repeating characters problem involves using two pointers to expand and contract a window within the string. The algorithm expands the window by moving the right pointer until a repeating character is found, then contracts the window by moving the left pointer, ensuring the maximum window size without repeating characters.
What is Backtracking and how is it implemented?
-Backtracking is a technique used to explore all possible solutions by building them step by step. It involves recursion and is implemented by going back (or 'backtracking') to previous steps and exploring other options when an invalid state is reached.
How does the Combination Sum problem illustrate the concept of Backtracking?
-The Combination Sum problem demonstrates Backtracking by starting with an empty combination and recursively adding numbers to the combination, updating the sum, and the index. If the sum exceeds the target or a valid combination is found, the algorithm backtracks to explore other combinations.
What is the main difference between Dynamic Programming and Backtracking?
-While Backtracking explores all possible solutions from scratch, Dynamic Programming is more thoughtful about the solutions it explores. It breaks a problem into smaller subproblems and uses the solutions to these subproblems to build up to the solution of the original problem, often using memoization to avoid redundant calculations.
How does the script suggest solving the Combination Sum problem using Dynamic Programming?
-The script suggests a Dynamic Programming approach where you start with the combinations that add up to the target sum minus the last number and then use the last number to generate new combinations that add up to the target sum.
What are the main differences between Depth First Search (DFS) and Breadth First Search (BFS)?
-DFS explores as far as possible along each branch before backtracking, using a stack to implement Last In First Out (LIFO) order. BFS, on the other hand, explores all neighboring vertices at the present depth prior to moving on to vertices at the next depth level, using a queue for First In First Out (FIFO) order.
Why are Breadth First Search (BFS) and Depth First Search (DFS) important for coding interviews?
-BFS and DFS are important for coding interviews because they are fundamental graph traversal algorithms used to solve a variety of problems, including finding the shortest path and exploring all possible paths in a graph.
What is the relationship between Topological Sort and Depth First Search (DFS)?
-Topological Sort is closely related to DFS as it is a linear ordering of vertices such that for every directed edge uv, vertex u comes before v in the ordering. Topological Sort can be implemented using DFS to visit all vertices in a directed acyclic graph.
Outlines
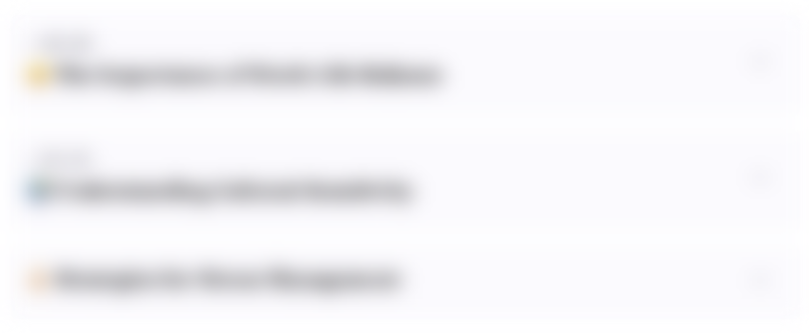
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
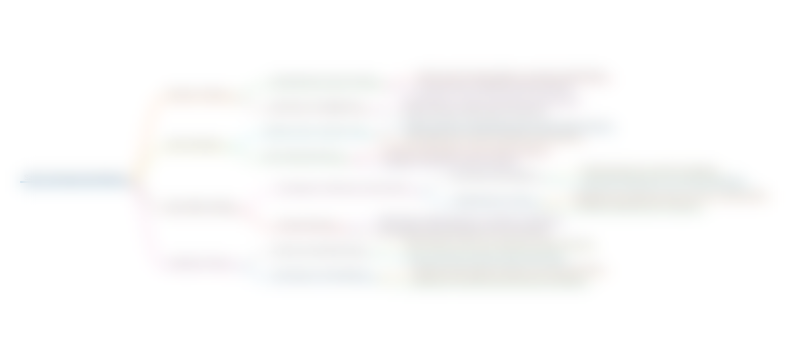
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
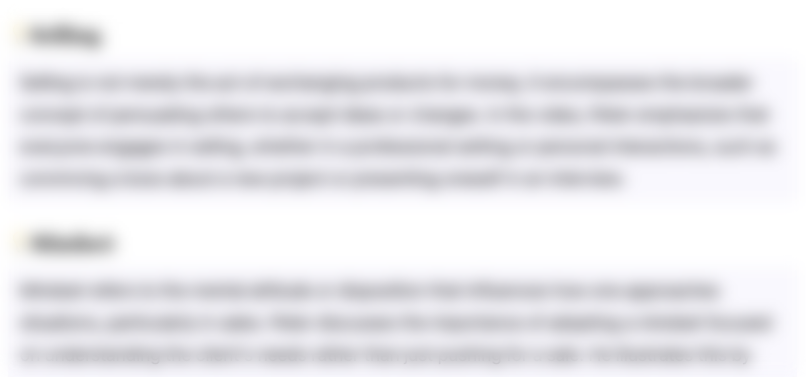
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
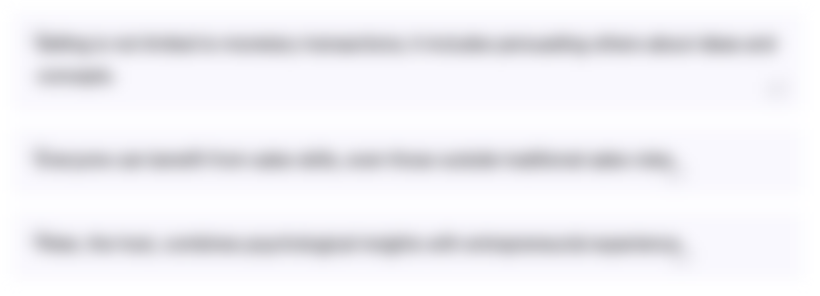
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
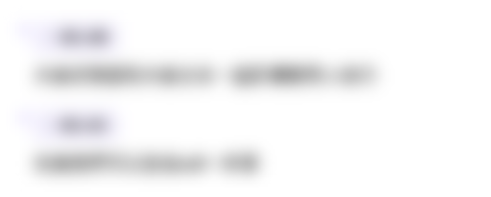
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
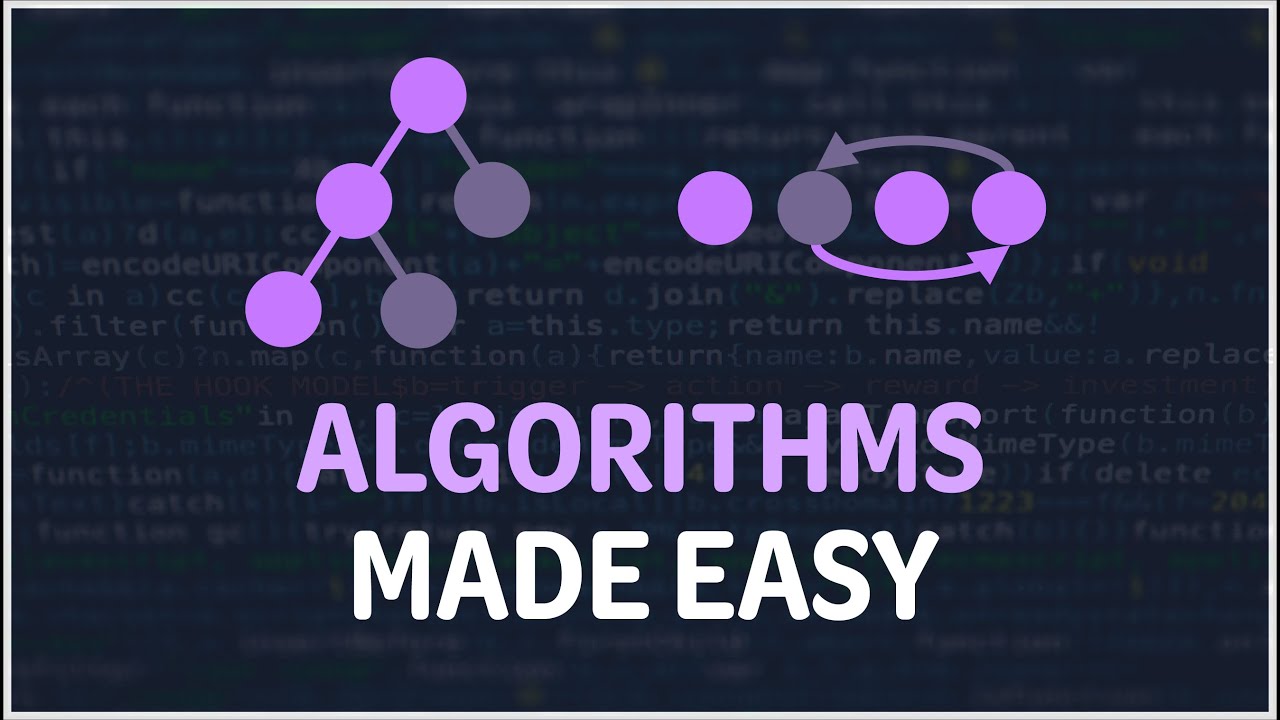
Top 7 Algorithms for Coding Interviews Explained SIMPLY
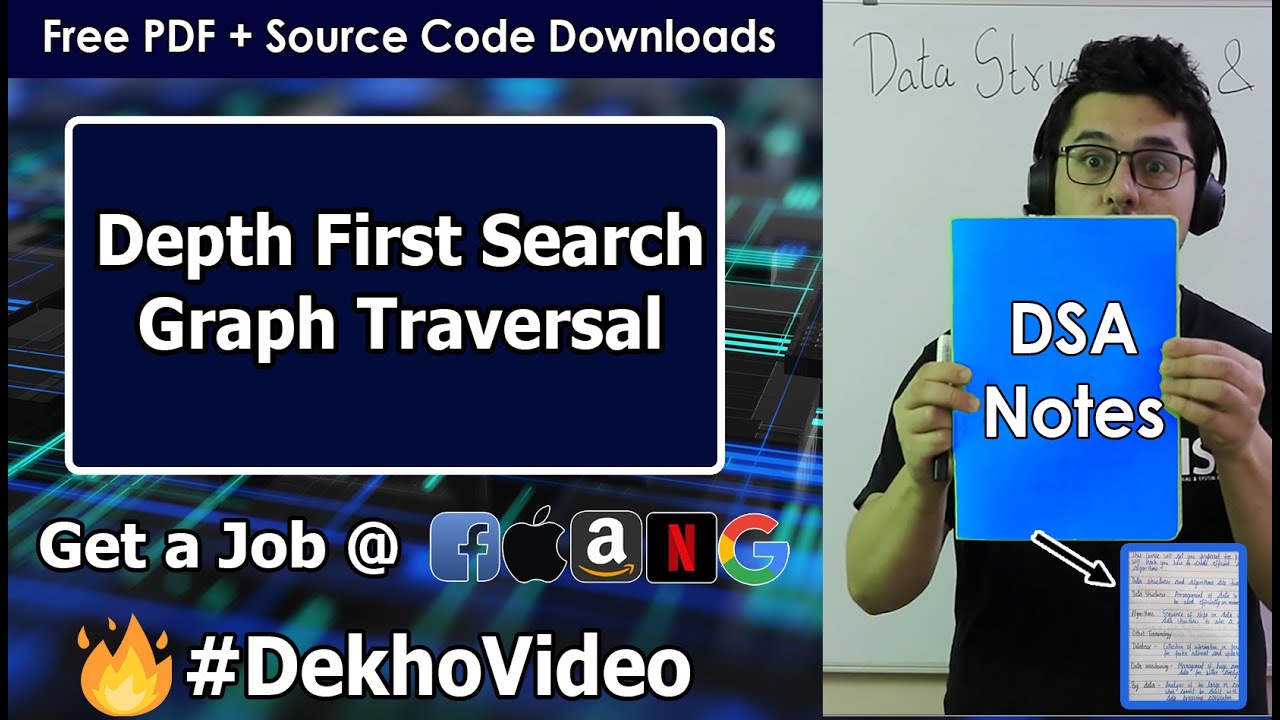
Depth First Search (DFS) Graph Traversal in Data Structures

93. OCR A Level (H446) SLR14 - 1.4 Data structures part 2 - Graphs (operations)

Breadth First Search BFS
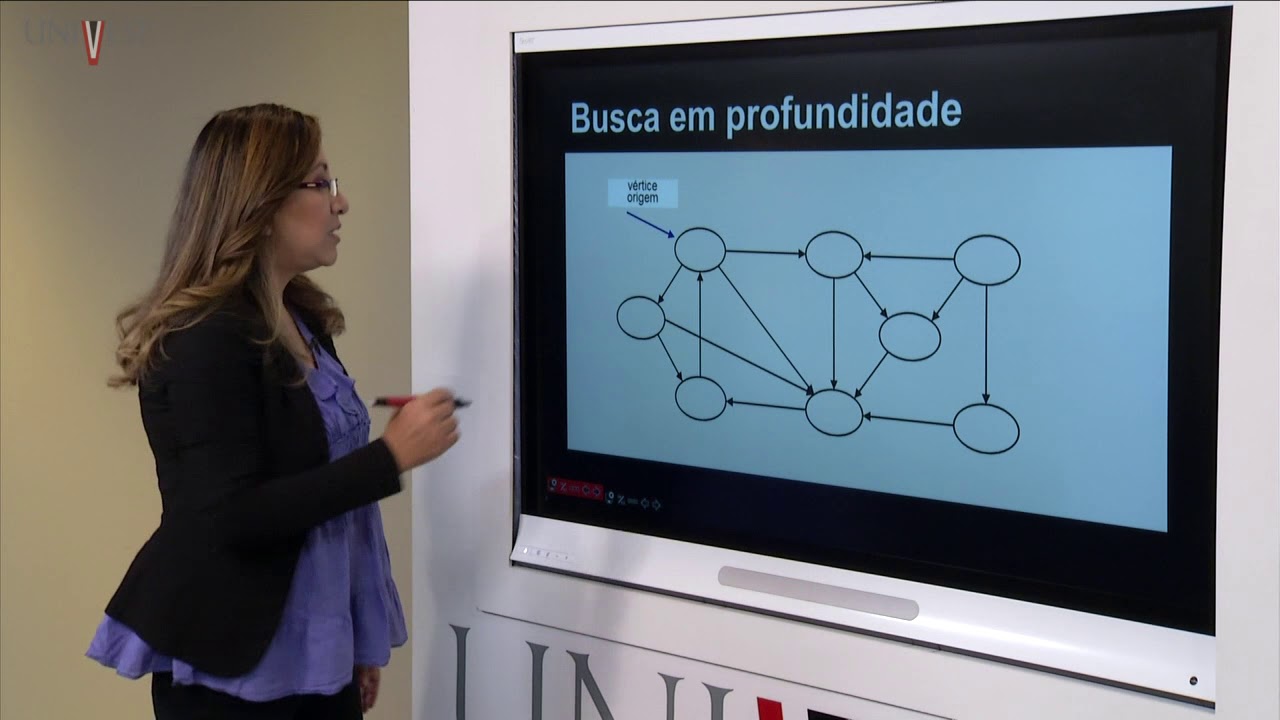
Projeto e Análise de Algoritmos - Aula 12 - Algoritmos de busca em largura e profundidade em grafos
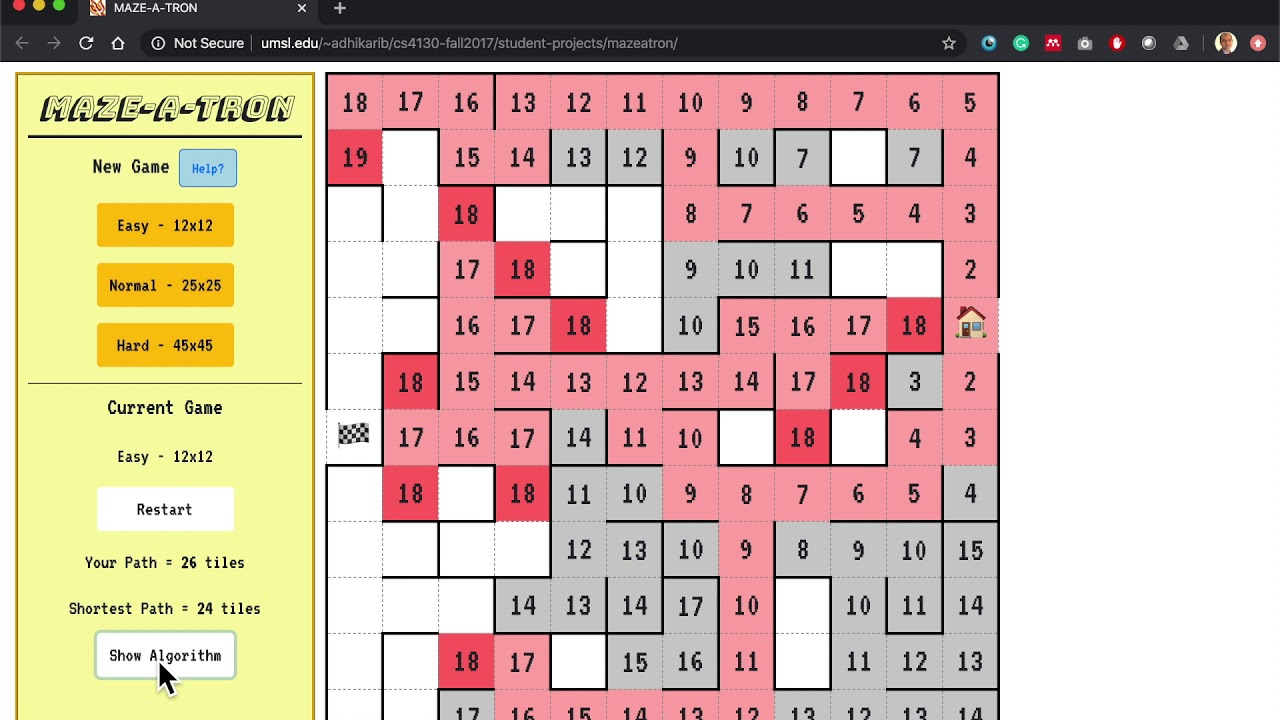
AI - Ch03 - Uninformed search algorithms
5.0 / 5 (44 votes)