COS 333: Chapter 1, Part 2
Summary
TLDRThis script delves into the foundational concepts of programming languages, exploring the influences shaping their design, such as computer architecture and methodologies. It outlines various paradigms including imperative, functional, and logic programming, each with unique approaches to computation. The discussion also covers implementation methods like compilation, interpretation, and hybrid systems, highlighting their trade-offs in efficiency and portability. Finally, it touches on programming environments and tools that aid developers in coding, debugging, and profiling software.
Takeaways
- 🌟 The lecture discusses preliminary concepts in programming languages, including influences on language design, language categories (paradigms), implementation methods, and programming environments.
- 🔍 Influences on programming language design are primarily computer architecture and programming methodologies, with von Neumann architecture significantly impacting the nature of programming languages.
- 🔧 Imperative programming languages, such as C, C++, and Java, are dominant due to their close modeling of the von Neumann computer architecture, focusing on variables, assignments, and efficient iteration.
- 📚 The evolution of programming languages has been driven by the development of new software methodologies, leading to the introduction of features like functions, modules, data abstraction, and object-oriented programming.
- 🛠️ Programming language implementation methods include compilation, interpretation, and hybrid systems, each with their advantages and disadvantages in terms of execution speed, error reporting, and portability.
- 🔄 The compilation process involves several phases: lexical analysis, syntax analysis, semantic analysis, and code generation, resulting in efficient machine code execution.
- 🔍 Pure interpretation offers advantages like better error reporting and portability but suffers from slower execution speeds due to the need to decode high-level statements at runtime.
- 🤝 Hybrid implementation systems, like the Java Virtual Machine, balance compilation and interpretation, offering improved error reporting and execution speeds while maintaining portability.
- 🚀 Just-In-Time (JIT) systems are a type of hybrid implementation that compiles sub-programs into machine code on-the-fly, improving execution speed for subsequent calls.
- 🛠️ Programming environments encompass a collection of tools that support software development, ranging from simple command-line tools in Unix-like systems to integrated development environments (IDEs) like Visual Studio and NetBeans.
- 📈 The course will continue to explore the history and evolution of major programming languages in subsequent chapters, providing a deeper understanding of language development over time.
Q & A
What are the main factors that influence the design of a programming language?
-The main factors that influence the design of a programming language are computer architecture and programming methodologies. Computer architecture refers to the prevalent computing hardware at the time of language development, while programming methodologies involve the software development processes and paradigms that shape the features and capabilities of the language.
Why is the von Neumann architecture significant to the development of programming languages?
-The von Neumann architecture is significant because it is the most prevalent computer architecture, and it influences the nature of programming languages by dictating how data and instructions are stored and processed. Most modern computers follow this architecture, which is why many programming languages are designed to work closely with it.
What is imperative programming and how does it relate to the von Neumann architecture?
-Imperative programming is a paradigm where programs are written as a sequence of commands for the computer to perform. It closely relates to the von Neumann architecture by modeling the use of variables as memory cells and assignments as the data transfer between memory and CPU, reflecting the architecture's design.
Why are iteration structures efficient in imperative programming languages?
-Iteration structures are efficient in imperative programming languages because they can leverage the sequential storage of values in memory. By using a counter and incrementing it, programs can easily move through memory locations, making iteration a fast and straightforward process.
How has the evolution of programming methodologies led to the development of new programming languages?
-As new programming methodologies are developed, they introduce new ways of thinking about software development. This evolution necessitates the creation of new programming languages that support these paradigms, leading to the development of languages that better accommodate the methodologies' requirements and features.
What are the three main programming language paradigms discussed in the script?
-The three main programming language paradigms discussed are imperative programming, functional programming, and logic programming. Each paradigm has its own approach to computation and problem-solving, catering to different aspects of software development.
How does functional programming differ from imperative programming?
-Functional programming differs from imperative programming in that it relies on the application of mathematical functions to parameters, without the use of variables, assignments, or iteration. It focuses on pure functions and avoids side effects, leading to a different programming style that is more declarative in nature.
What is logic programming and how does it operate?
-Logic programming is a paradigm where programs are expressed as a set of facts and rules. The programming language uses an inference engine to reason about these facts and rules to answer queries. It is a declarative approach where the focus is on the relationships between data and the logic used to derive conclusions.
What are the three main implementation methods for high-level programming languages?
-The three main implementation methods for high-level programming languages are compilation, pure interpretation, and hybrid implementation systems. Each method has its own advantages and disadvantages in terms of execution speed, portability, and ease of use.
Why is pure interpretation less efficient than compilation?
-Pure interpretation is less efficient than compilation because it involves decoding high-level statements at runtime, which is more time-consuming than executing pre-translated machine code. Additionally, the interpreter has to handle the full source program and symbol table during execution, leading to higher memory and storage requirements.
How does a hybrid implementation system, like the Java Virtual Machine, improve upon pure interpretation?
-A hybrid implementation system, such as the Java Virtual Machine, improves upon pure interpretation by translating the high-level code into an intermediate bytecode, which is then interpreted by a virtual machine. This approach allows for better error reporting and potentially faster execution than pure interpretation, while still maintaining portability across different platforms.
What is the role of programming environments in supporting software development?
-Programming environments provide a collection of tools that support various aspects of software development, such as code formatting, debugging, and performance profiling. They can range from simple command-line tools in Unix-like systems to complex visual environments like Microsoft's Visual Studio, which integrate multiple development tools into a unified interface.
Outlines
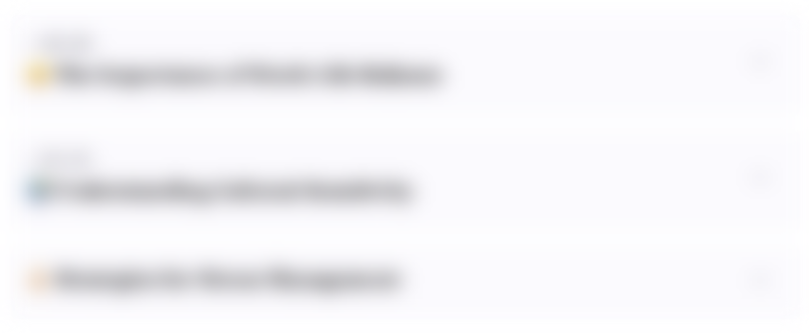
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
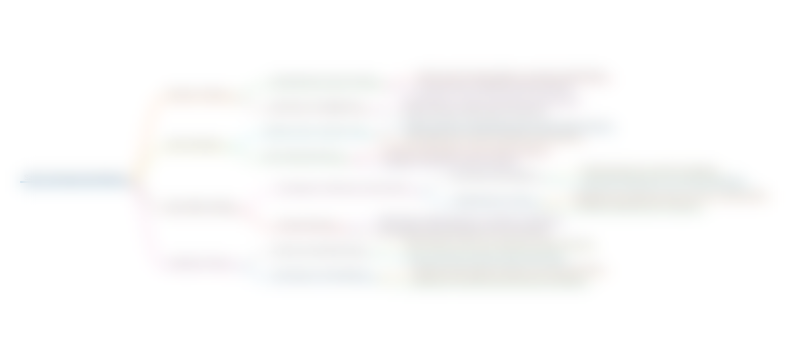
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
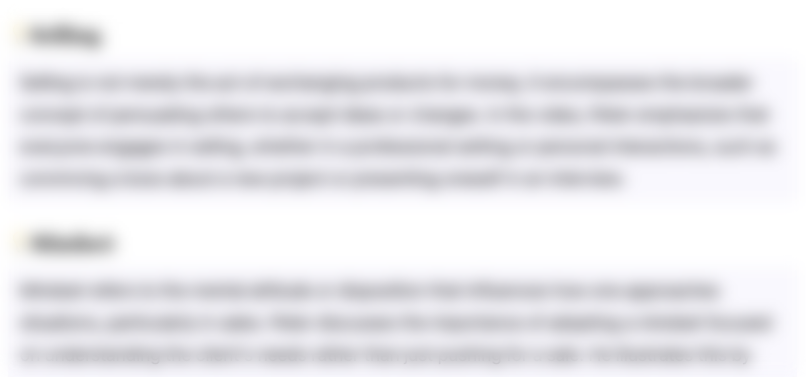
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
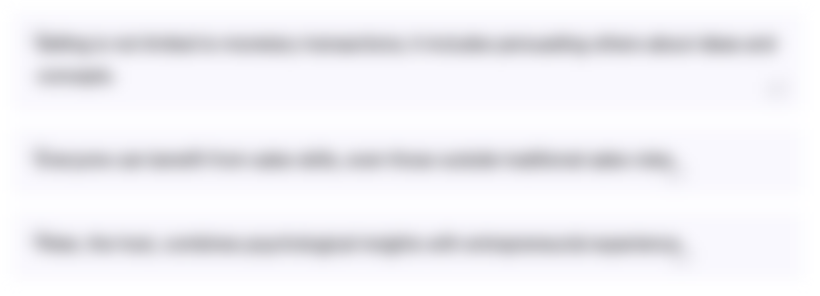
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
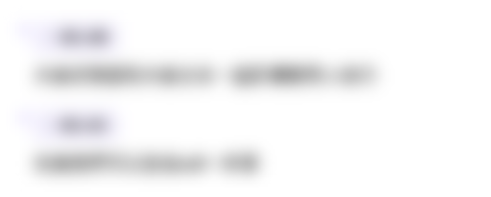
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
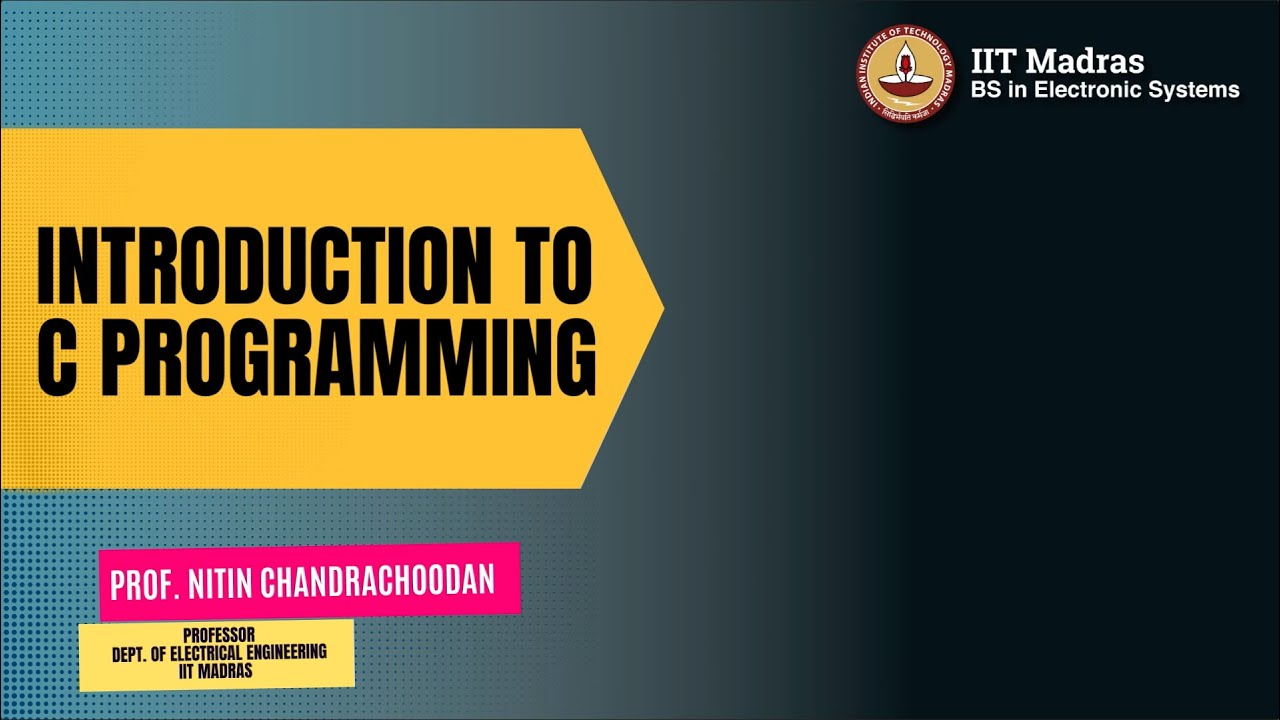
1. How a Computer works

Introduction to Computer Programming | What is it? Programming Language Types

Backend Developer Roadmap 2024 | خارطة تعلم تطوير تطبيقات الباك اند
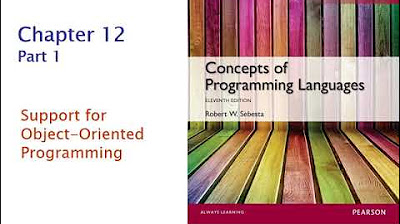
COS 333: Chapter 12, Part 1
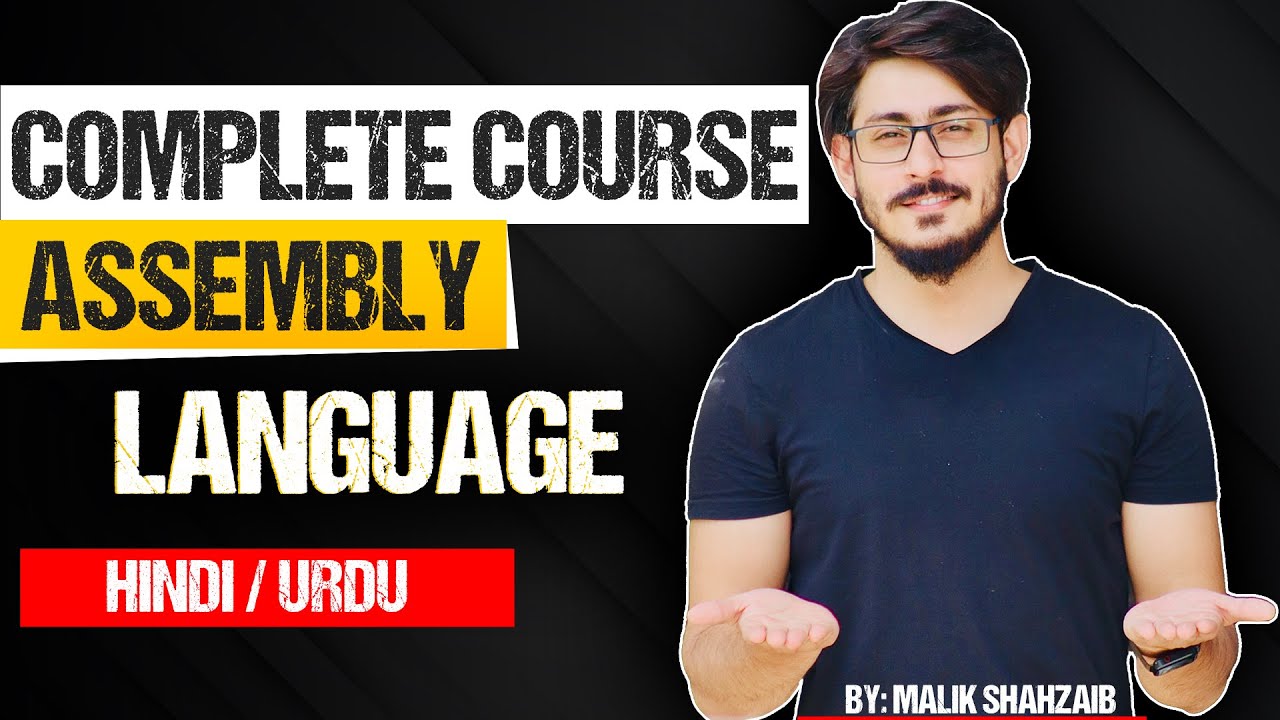
Introduction to Assembly Language Programming Tutorial in Urdu Hindi | best intro
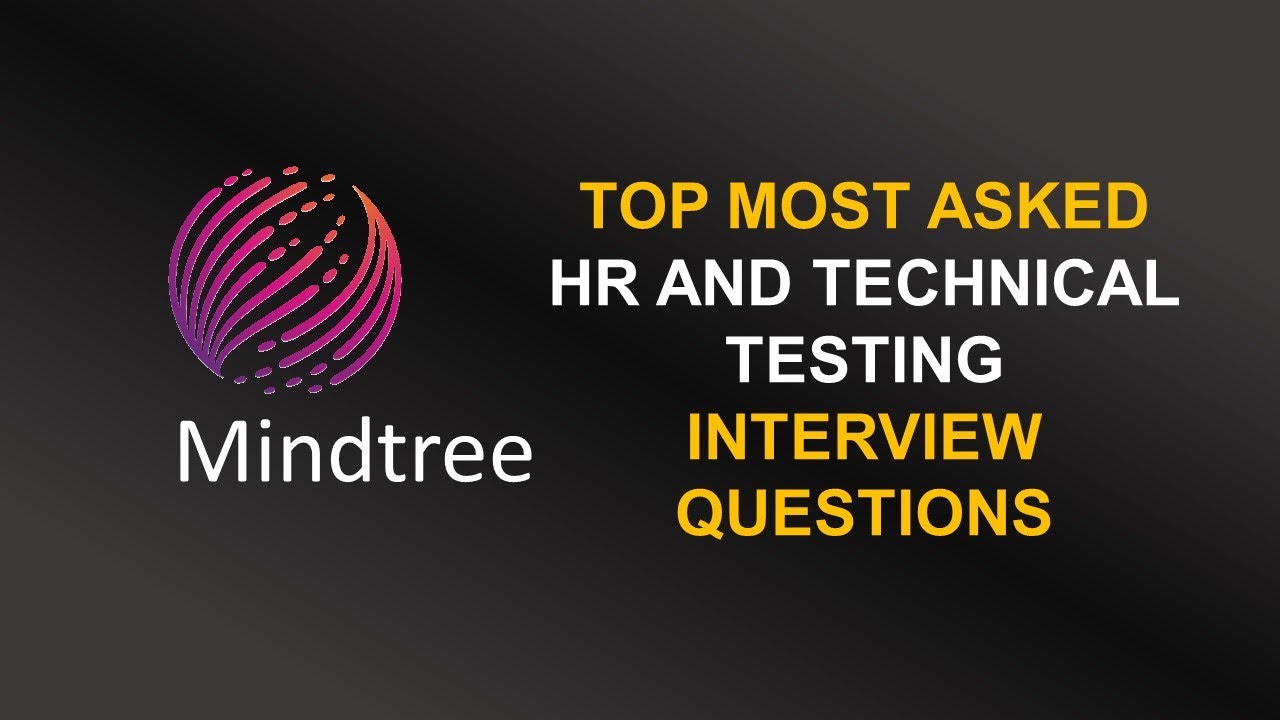
Top Mindtree Interview Questions for Freshers | Most Asked Mindtree Interview 2022
5.0 / 5 (0 votes)