Python Syntax - Everything you need to know!
Summary
TLDRThis video tutorial offers an in-depth introduction to Python syntax, focusing on indentation with spaces or tabs, emphasizing readability and uniformity. It covers the significance of using snake case for variables and functions, pascal case for classes, and capitalized snake case for constants, as per PEP 8 guidelines. The tutorial also explains the use of comments with hashtags, string literals with different quote types, and techniques for managing long statements with backslashes. Aimed at beginners, the video simplifies complex concepts and encourages interactive learning through a provided repository for hands-on practice.
Takeaways
- 😀 Python uses indentation with spaces or tabs for code structuring, unlike languages like JavaScript that use semicolons.
- 📏 The PEP 8 style guide recommends using four spaces for indentation if spaces are chosen, and one tab if tabs are used.
- 👀 Consistency is key: never mix spaces and tabs in Python code.
- 🔑 Functions in Python are defined using the `def` keyword, followed by the function name and colon.
- 📝 Comments in Python are made using the `#` symbol, which is essential for code readability and collaboration.
- 🔍 String literals in Python can be declared using single quotes, double quotes, or triple quotes for multi-line strings.
- 📑 Multi-line strings are useful for preserving formatting, such as line breaks, in the output.
- 🔗 Long statements in Python can be split into multiple lines using a backslash for better readability.
- 📝 Casing conventions in Python are important for readability: snake_case for variables, functions, methods, and modules; PascalCase for classes; and UPPER_CASE for constants.
- 🌐 For further learning, the video suggests checking out the Python category on cosec.com for tutorials, written articles, and coding repositories.
Q & A
What is the primary focus of the video?
-The primary focus of the video is to teach the basics of Python syntax, including code structuring, indentation, and commenting.
Why is indentation important in Python?
-Indentation is important in Python because it is used for code structuring, distinguishing blocks of code such as functions, loops, and conditionals.
What is the recommended number of spaces for indentation according to PEP 8?
-According to PEP 8, the recommended number of spaces for indentation is four.
Can you use tabs for indentation in Python?
-Yes, you can use tabs for indentation in Python, but it is recommended to use spaces for consistency, and mixing spaces and tabs is discouraged.
How does Python handle the end of statements compared to other languages like JavaScript?
-Python does not require a semicolon at the end of statements like JavaScript does. Instead, Python uses indentation to define the end of a statement.
What is the purpose of comments in Python code?
-Comments in Python code serve to explain the code, making it easier to understand for both the developer and others who may read the code. They are also useful for temporarily disabling code during testing.
How do you create a comment in Python?
-In Python, you create a comment by starting the line with a hashtag (#), and the rest of the line is treated as a comment and not executed.
What are the different ways to declare strings in Python?
-In Python, you can declare strings using single quotes ('string'), double quotes ("string"), triple single quotes ('''string'''), or triple double quotes ("""string""") for multi-line strings.
Why would you use multi-line strings in Python?
-Multi-line strings are used in Python when you want to preserve whitespace and line breaks in the output, which is not possible with single-line strings.
How can you separate long statements in Python for better readability?
-You can separate long statements in Python for better readability by using a backslash (\) at the end of the line before the line break.
What are the casing conventions for different elements in Python according to PEP 8?
-According to PEP 8, variables, functions, methods, and modules should be in snake_case, classes should be in PascalCase, and constants should be in UPPER_SNAKE_CASE.
Outlines
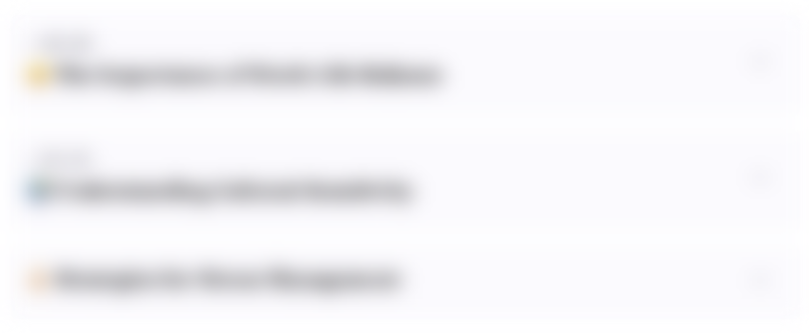
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
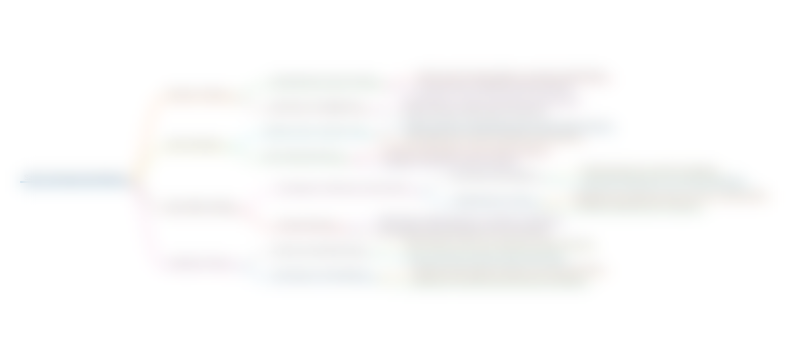
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
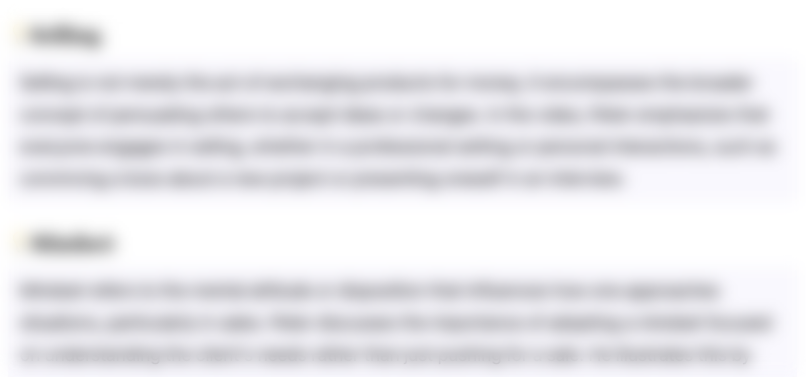
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
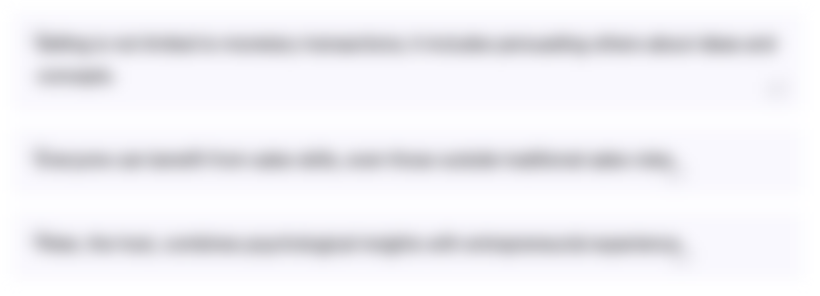
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
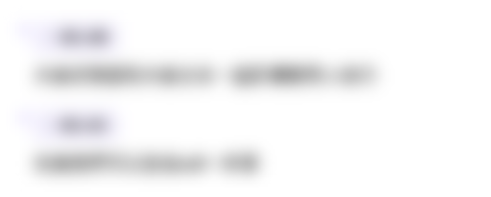
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
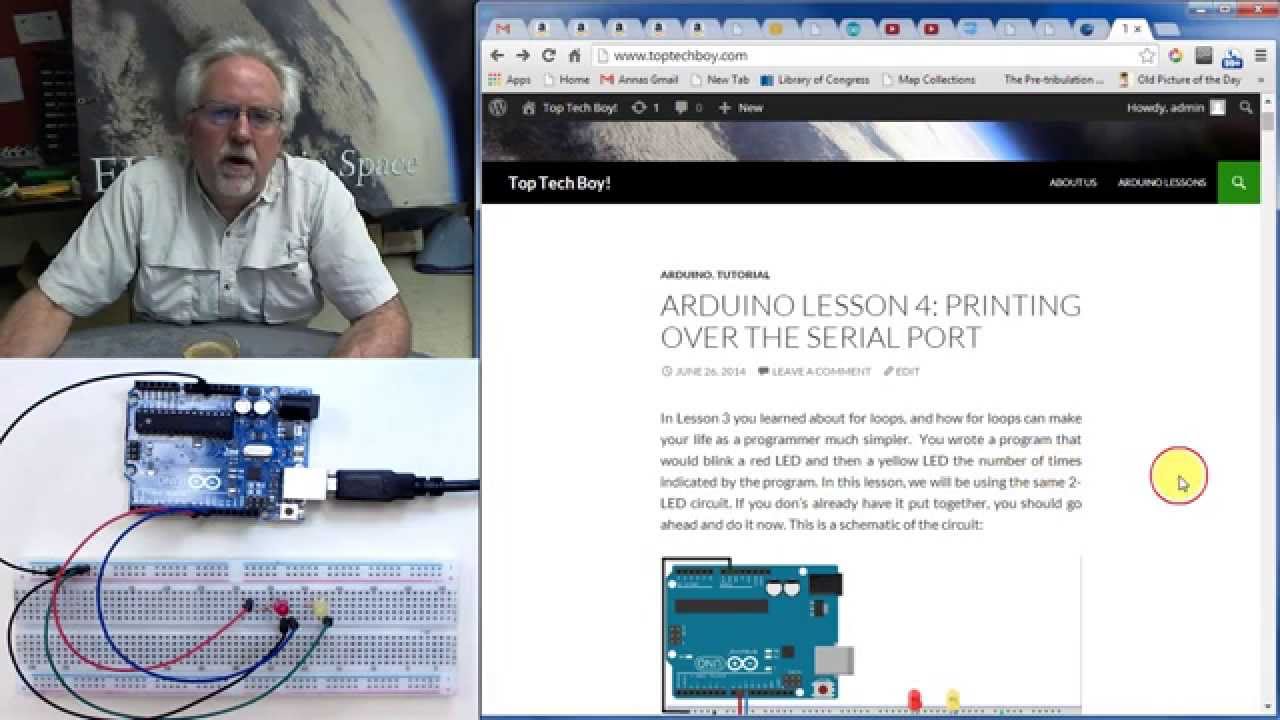
LESSON 4: Printing Over the Arduino Serial Port

Cara Menuliskan Kode Python di Google Colab [EPISODE-2]
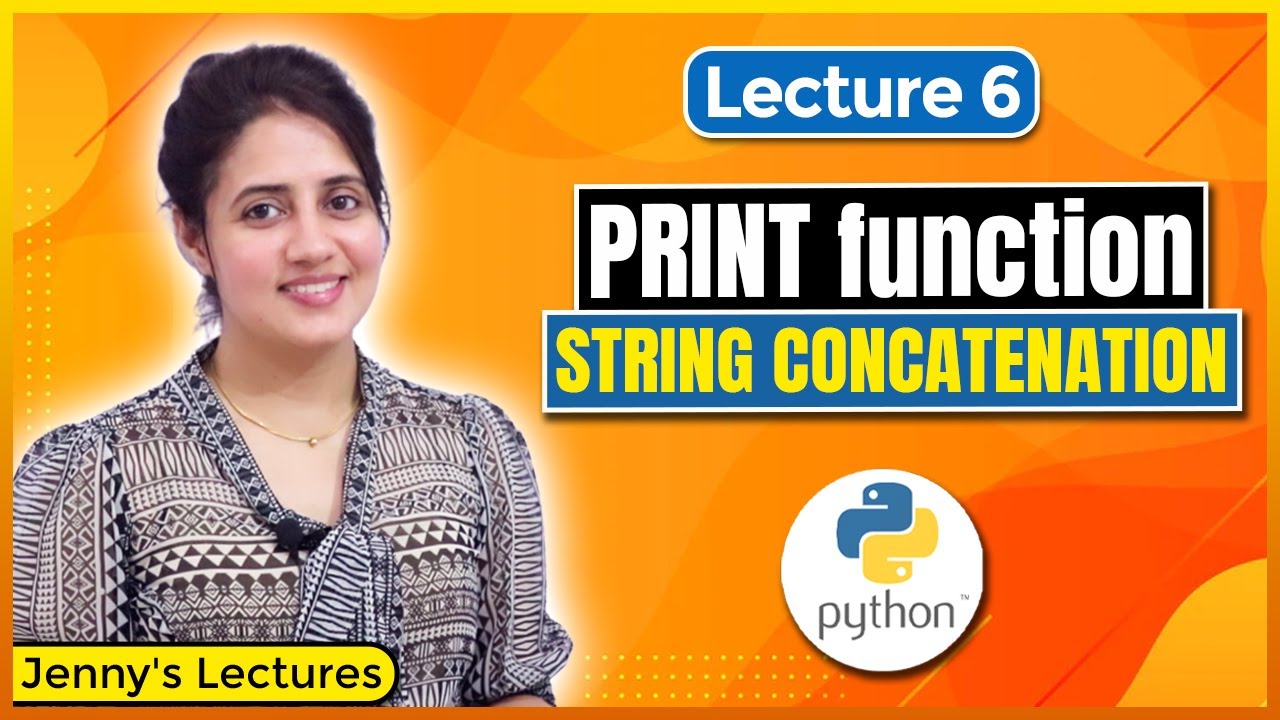
P_06 print() function & String concatenation(using +) in Python | Python Tutorials for Beginners
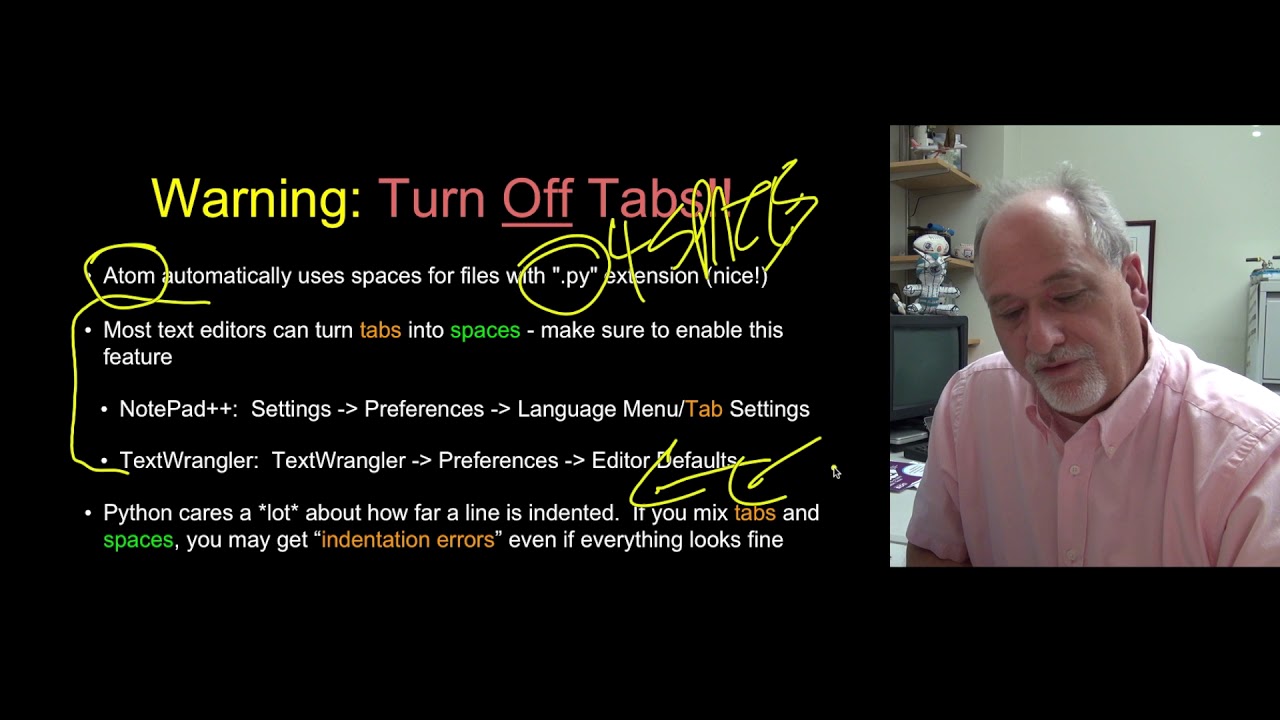
03 - Conditional A - Python for Everybody Course
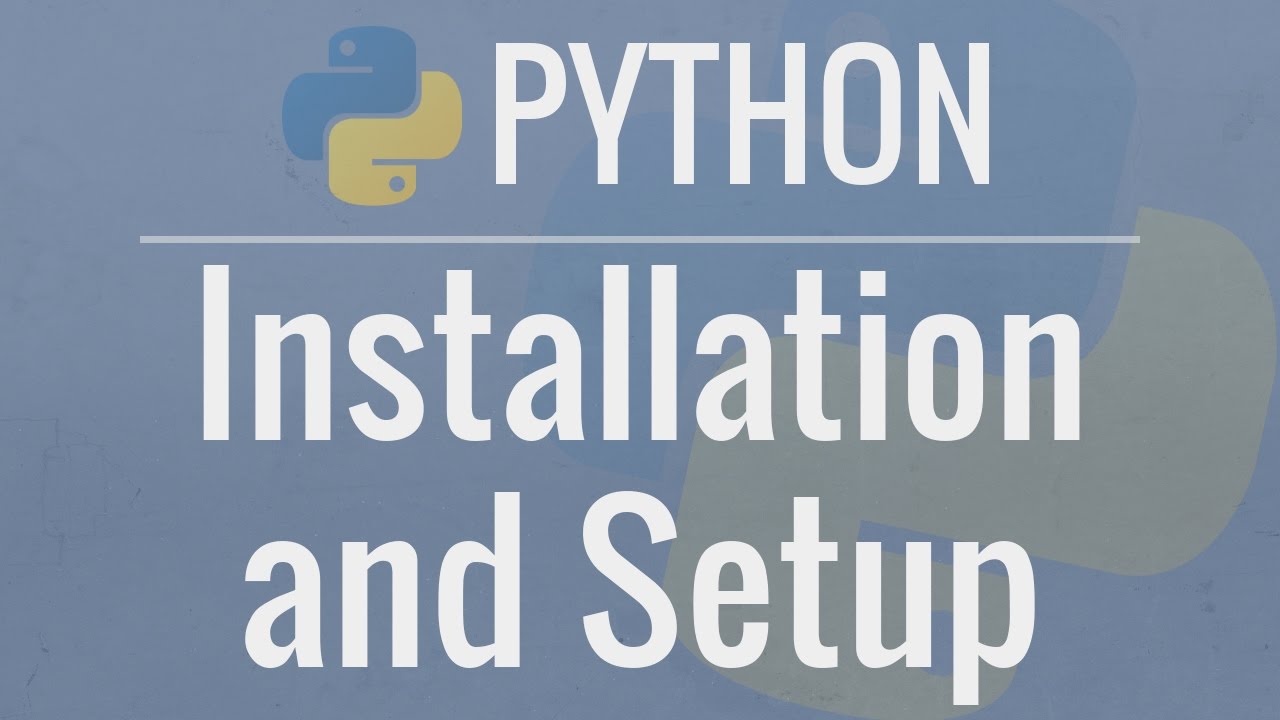
Python Tutorial for Beginners 1: Install and Setup for Mac and Windows

Python - Introduction - W3Schools.com
5.0 / 5 (0 votes)