Learn Assembly Programming - Instructions, Mnemonics, Operands, and Opcodes
Summary
TLDRIn this tutorial, Dan explores assembly language concepts, focusing on instruction mnemonics, opcodes, and operands. He explains how opcodes instruct the processor to perform operations, with mnemonics acting as human-readable representations of these opcodes. Dan also breaks down operands, which are parameters following the mnemonic, and demonstrates how instructions are formed. By using practical examples, he showcases the movement of data across registers in assembly code, along with an introduction to system calls. The tutorial offers a hands-on approach to understanding low-level programming fundamentals, with a focus on 32-bit registers and NASM assembly language.
Takeaways
- 😀 OpCodes, short for operation codes, tell the processor what operations to perform and are followed by arguments that instruct the processor on how to perform those operations.
- 😀 Mnemonics are friendly terms used to describe OpCodes, helping to make machine language more understandable. For example, the mnemonic 'MOV' represents the opcode B8.
- 😀 Operands are arguments that follow the mnemonic in an instruction. They directly affect the behavior of the instruction by specifying what data is being moved or operated on.
- 😀 Instructions are composed of a mnemonic and its operands, and they make up the executable lines of code in an assembly program.
- 😀 The 'MOV' mnemonic is one of the most commonly used in assembly, and it can work with up to three operands, such as a register and a value.
- 😀 In the x86 architecture, the EAX, ECX, EDX, and EBX registers are commonly used with the MOV instruction, with each having a corresponding opcode (e.g., B8 for EAX, B9 for ECX).
- 😀 Assembly language allows working with both 32-bit and 16-bit registers, with the 16-bit registers being prefixed by the hex value '66'.
- 😀 Hexadecimal values represent instructions in assembly code, and the size of each value corresponds to the number of bytes being moved (e.g., 32-bit registers use 4 bytes).
- 😀 The use of a hex editor can help visualize machine code and disassembled instructions, allowing developers to see how instructions are represented in binary form.
- 😀 The tutorial demonstrated how to set up and run assembly code in both NASM (Netwide Assembler) on Linux and Visual Studio on Windows, showing the process of building and linking assembly programs.
Q & A
What is an opcode in assembly language?
-An opcode (short for operation code) tells the processor what operation to perform. It is followed by arguments that instruct the processor on what data to operate on, thus forming the core instructions in machine language.
What is the relationship between mnemonics and opcodes?
-Mnemonics are human-readable representations of opcodes. For example, the mnemonic 'mov' corresponds to the opcode 'b8', which moves data into a register. While opcodes are the actual machine code instructions, mnemonics provide a more accessible way for programmers to work with them.
What are operands in the context of assembly language?
-Operands are the arguments or parameters that follow the mnemonic in an instruction. They define the data to be processed by the operation. Depending on the mnemonic, there can be zero to three operands.
What is an example of an instruction in assembly language?
-An example of an instruction is 'mov eax, 1'. Here, 'mov' is the mnemonic, and 'eax' and '1' are the operands, forming the instruction that moves the value 1 into the EAX register.
What does the 'int' mnemonic do in assembly language?
-'int' is the mnemonic for the opcode 'cd', which triggers an interrupt. This is typically used for system calls or for interacting with the operating system at a low level.
Why does the tutorial focus on 32-bit registers before moving to 64-bit?
-The tutorial focuses on 32-bit registers (i386 or ia32) to ensure a solid understanding of basic assembly concepts before progressing to more complex 64-bit systems, as 32-bit is simpler and more foundational.
What role do registers like EAX, ECX, EDX, and EBX play in the tutorial?
-These are general-purpose registers used in assembly language. The tutorial demonstrates how to move values into these registers using the 'mov' instruction and shows how opcodes like 'b8', 'b9', 'ba', and 'bb' correspond to these registers.
What is the purpose of the '66' prefix in assembly language?
-The '66' prefix indicates that the operation is being performed on 16-bit registers instead of 32-bit registers. For example, 'b8' moves data into the EAX register (32-bit), while '66 b8' moves data into the AX register (16-bit).
What is a system call in the context of assembly programming?
-A system call is a request made by a program to the operating system to perform low-level operations, such as interacting with hardware or managing processes. In the tutorial, system calls are invoked using opcodes like 'cd' for interrupts.
How is NASM used in the tutorial?
-NASM (Netwide Assembler) is used to write and assemble the assembly code in the tutorial. The tutorial guides users through creating assembly files, assembling them into object files, and linking them to generate executable code.
Outlines
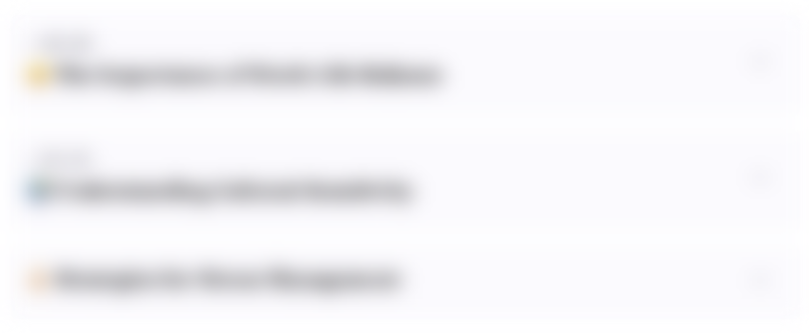
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
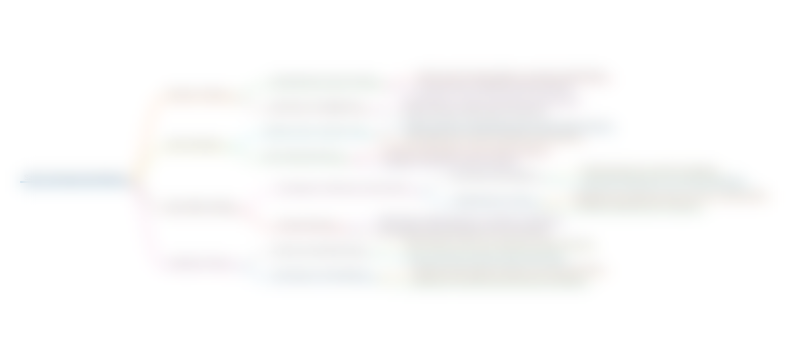
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
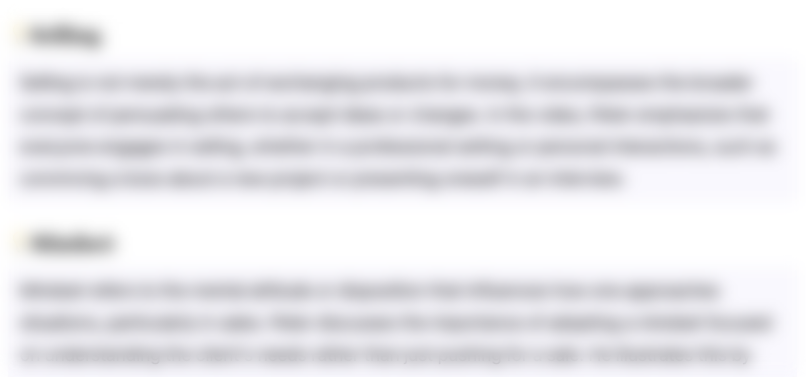
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
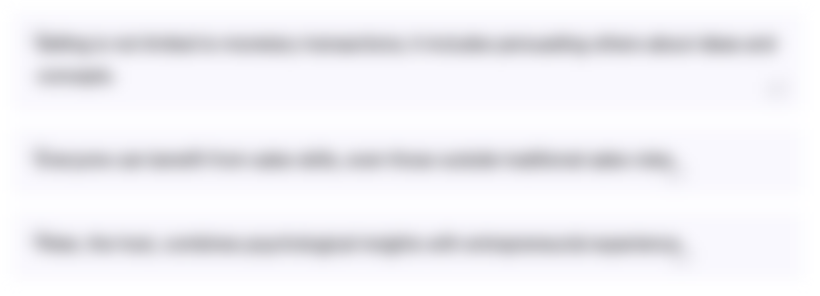
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
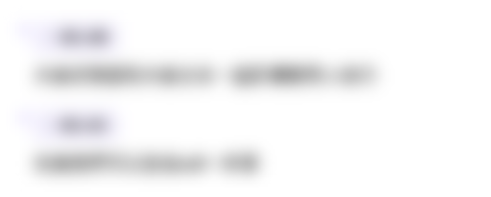
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

8085 instructions
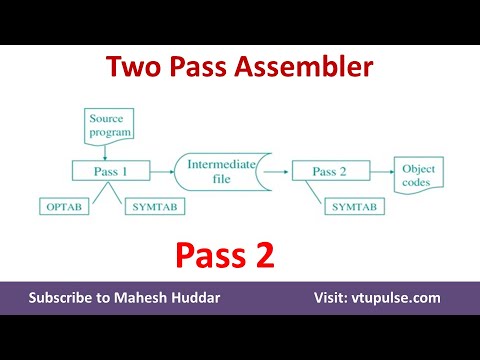
9. Pass - 2 Assembler of Two-pass assembler in System Software by Dr. Mahesh Huddar

IGCSE Computer Science 2023-25 - Topic 3: HARDWARE (2) - Fetch–Decode–Execute Cycle. Cores, Cache
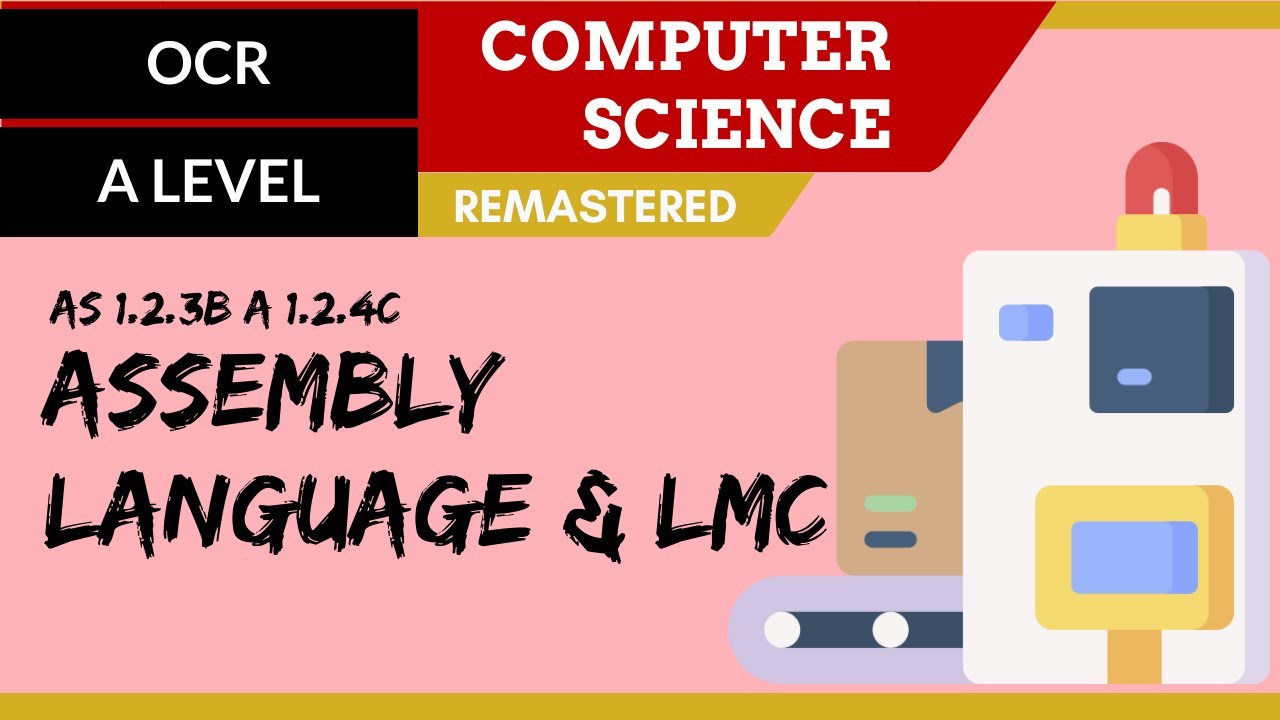
34. OCR A Level (H046-H446) SLR7 - 1.2 Assembly language and LMC language
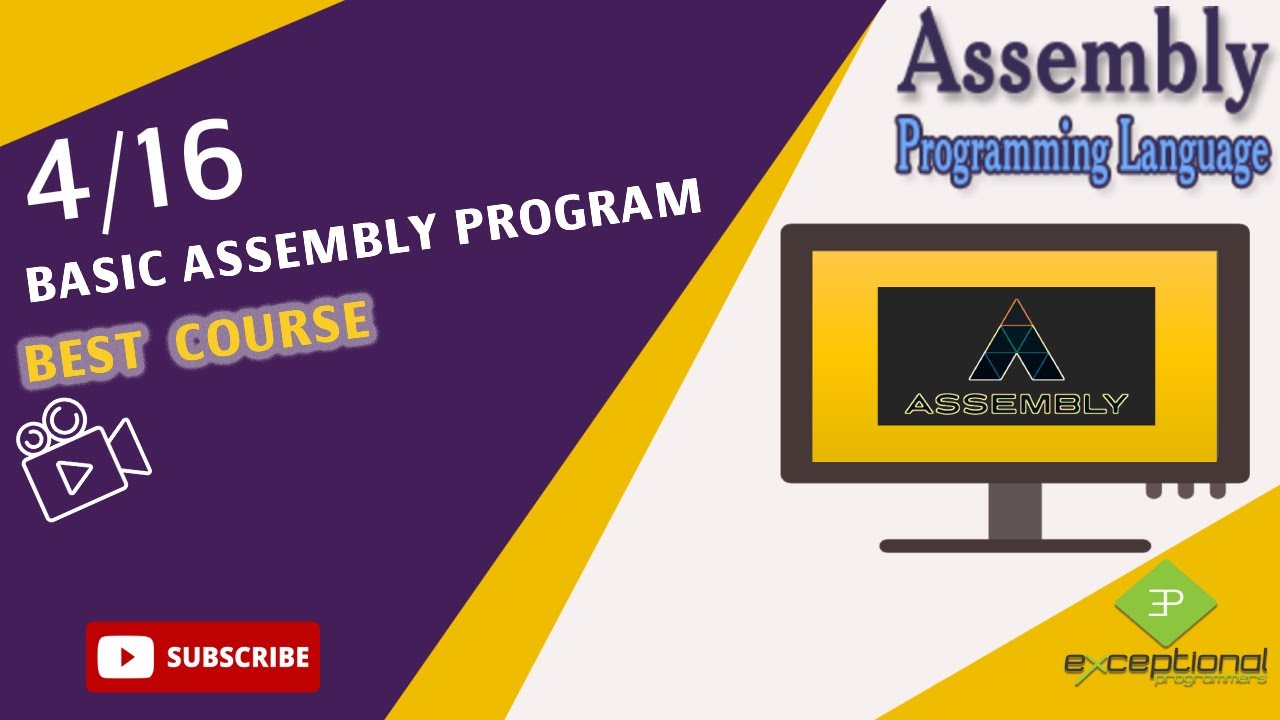
Sections Of The Assembly Program | first assembly program | | Assembly Language in MASM Part 4/16
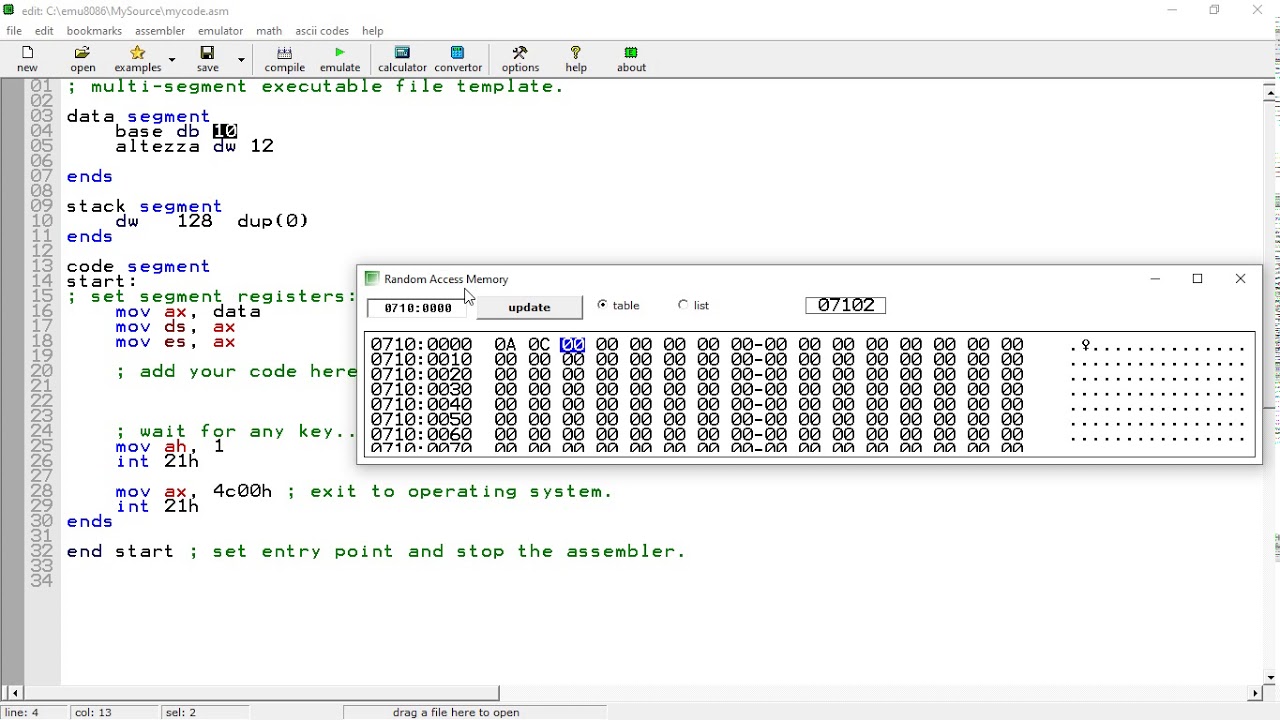
Il DataSegment 8086 Emulatore
5.0 / 5 (0 votes)