Python Tutorial: Working with JSON Data using the json Module
Summary
TLDRIn this tutorial, we explore how to work with JSON data in Python, starting with loading JSON from strings and files into Python objects. The video demonstrates how to manipulate the data, such as removing keys and formatting JSON outputs with indentation. It also covers real-world examples like fetching JSON data from APIs, parsing it, and performing currency conversions using exchange rates from Yahoo Finance. By the end of the tutorial, viewers will have a solid understanding of how to handle JSON data in Python, making it easy to work with APIs and process data for various applications.
Takeaways
- π JSON is a common data format used for storing and exchanging information, often found in APIs and configuration files.
- π JSON stands for JavaScript Object Notation, but it is language-independent and used in many programming languages, including Python.
- π To work with JSON in Python, you can use the built-in `json` library to parse and generate JSON data.
- π The `json.loads()` method allows you to load a JSON string into a Python object, converting JSON objects into Python dictionaries and arrays into lists.
- π JSON data types convert into their Python equivalents, such as strings, integers, booleans (True/False), and null values (None).
- π After converting JSON to a Python object, you can easily loop through data, access specific values, and manipulate them (e.g., deleting keys or modifying values).
- π The `json.dumps()` method allows you to convert Python objects back into a JSON string, and you can use the `indent` parameter to format the output for readability.
- π Sorting JSON keys is possible with the `sort_keys=True` argument in the `json.dumps()` method, which can help organize the output alphabetically.
- π To work with JSON files, you can use `json.load()` to read from a file and `json.dump()` to write data to a file, including the option to format and sort keys.
- π Real-world examples of using JSON include fetching data from APIs, such as converting currency values using a public finance API, which can be parsed and used in Python for calculations.
Q & A
What is JSON and where is it commonly used?
-JSON (JavaScript Object Notation) is a lightweight data format used for storing and transporting data. It is commonly used for fetching data from online APIs, configuration files, and saving data locally.
How does Python handle JSON data when it is loaded?
-In Python, JSON data is loaded using the `json.loads()` method. This converts JSON objects to Python dictionaries, arrays to lists, strings to strings, and boolean and null values to Python's `True`, `False`, and `None`.
What Python method can be used to convert a Python object to a JSON string?
-The `json.dumps()` method is used to convert a Python object back into a JSON string.
How can we make JSON output more readable in Python?
-You can make JSON output more readable by using the `indent` argument in `json.dumps()`. This allows you to format the JSON string with a specified number of spaces for indentation, making it easier to read.
What does the `sort_keys` argument do in `json.dumps()`?
-The `sort_keys` argument, when set to `True`, sorts the keys of the JSON objects alphabetically. This helps organize the data in a predictable order.
How can JSON data be read from and written to files in Python?
-JSON data can be read from a file using the `json.load()` method, which parses the file content into a Python object. To write Python objects to a JSON file, use the `json.dump()` method.
What is the difference between `json.load()` and `json.loads()`?
-`json.load()` is used to read and parse JSON data directly from a file, while `json.loads()` is used to parse JSON data from a string.
How can you access a specific key-value pair within a loaded JSON object?
-Once JSON is loaded into a Python object (such as a dictionary or list), you can access specific values by referencing their keys, like `data['key_name']` for dictionaries, or using indexing for lists.
What is an example of using JSON data from an external API in Python?
-The script demonstrates using JSON data from a Yahoo Finance API to convert USD to other currencies. The data is fetched using `urllib`, parsed into a Python object, and then processed for specific currency conversion rates.
How can Python handle complex nested JSON data structures?
-Python allows you to dig into complex nested JSON structures by accessing each level of the data using dictionary keys and list indexes. For example, `data['list']['resources'][0]['fields']['name']` can be used to access deeply nested values.
Outlines
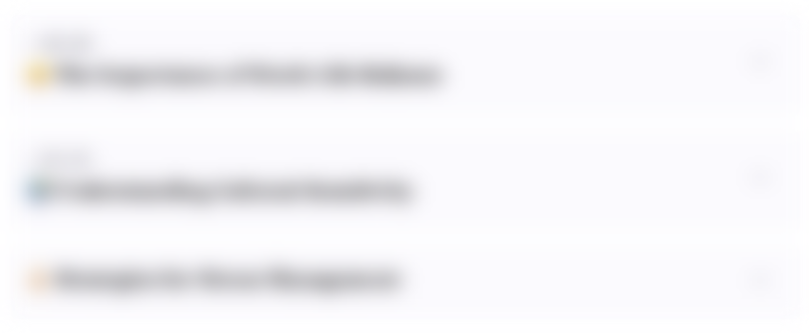
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
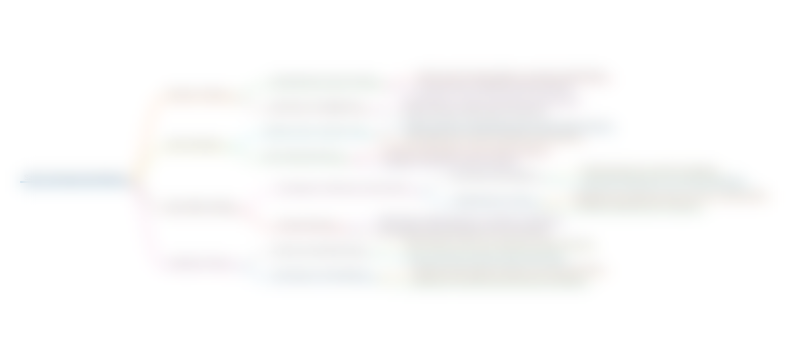
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
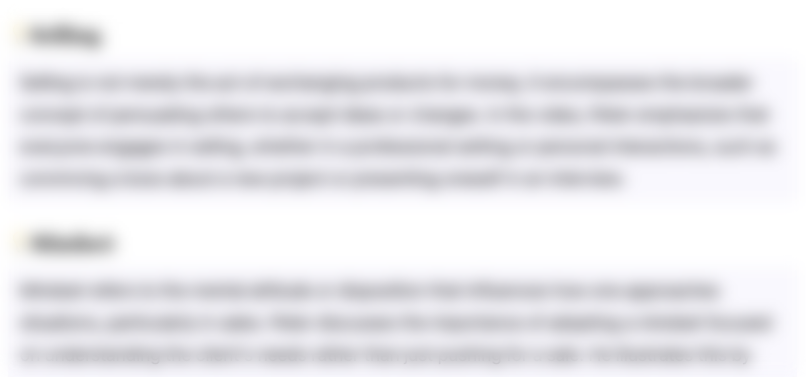
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
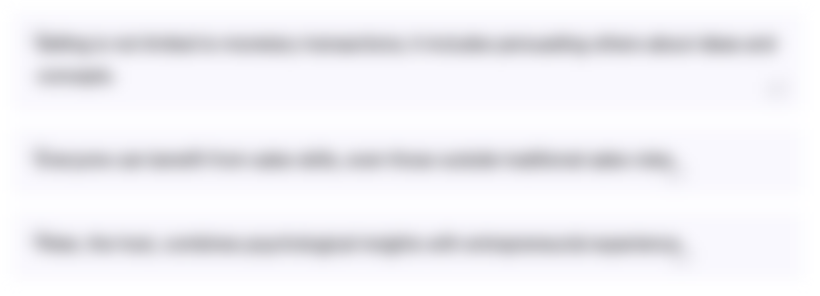
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
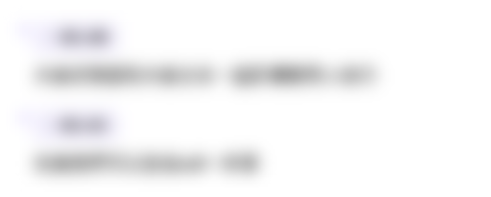
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
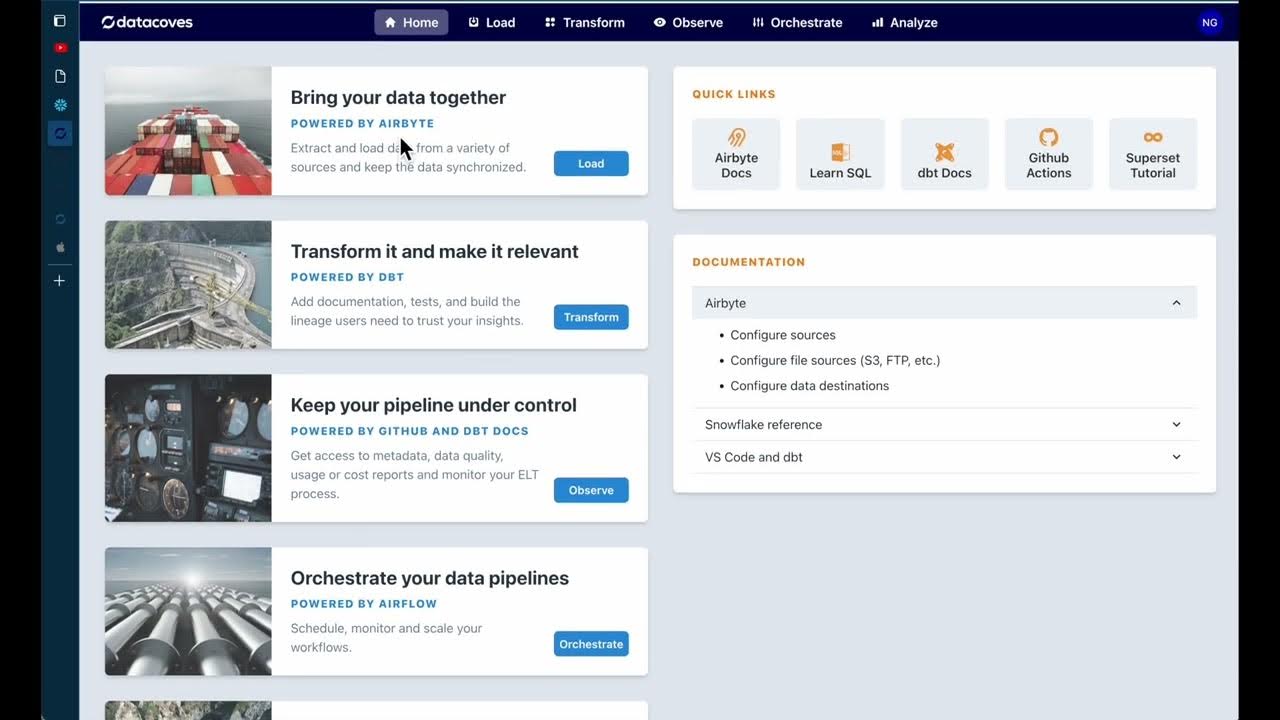
dbt model Automation compared to WH Automation framework
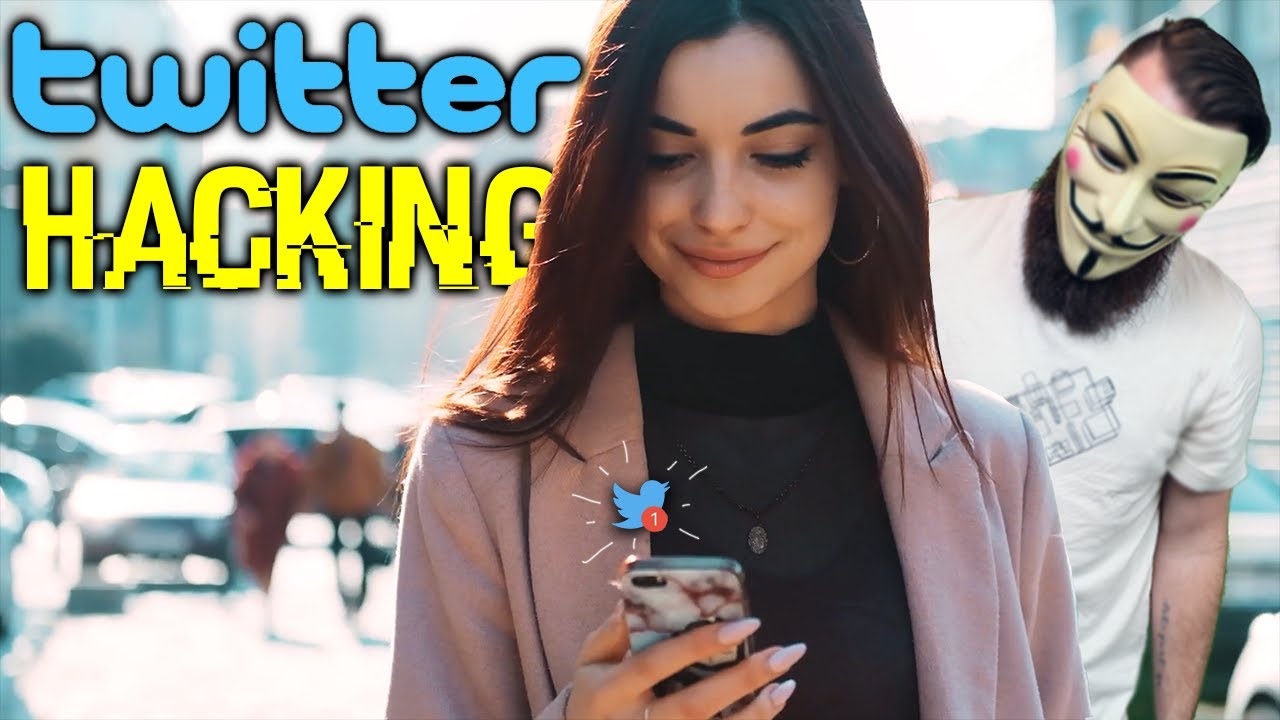
Twitter OSiNT (Ethical Hacking)
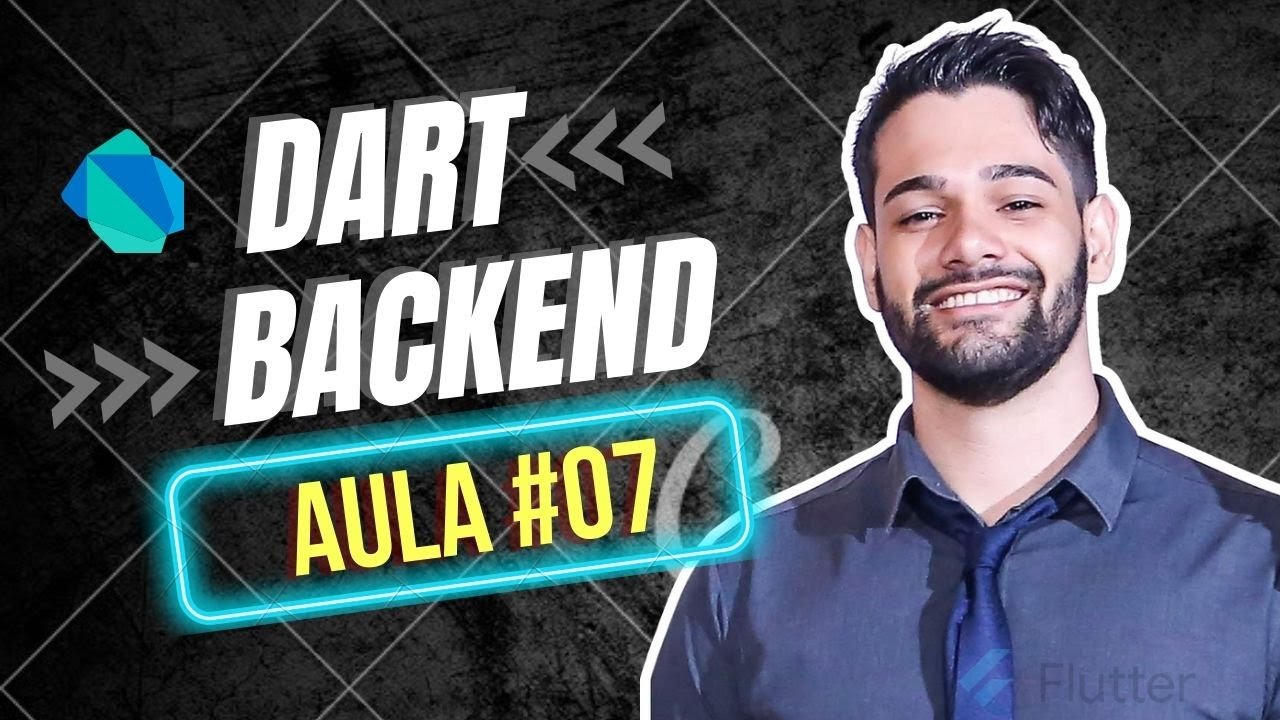
Curso Backend #07 - Mime Type, Trabalhando com retornos correto
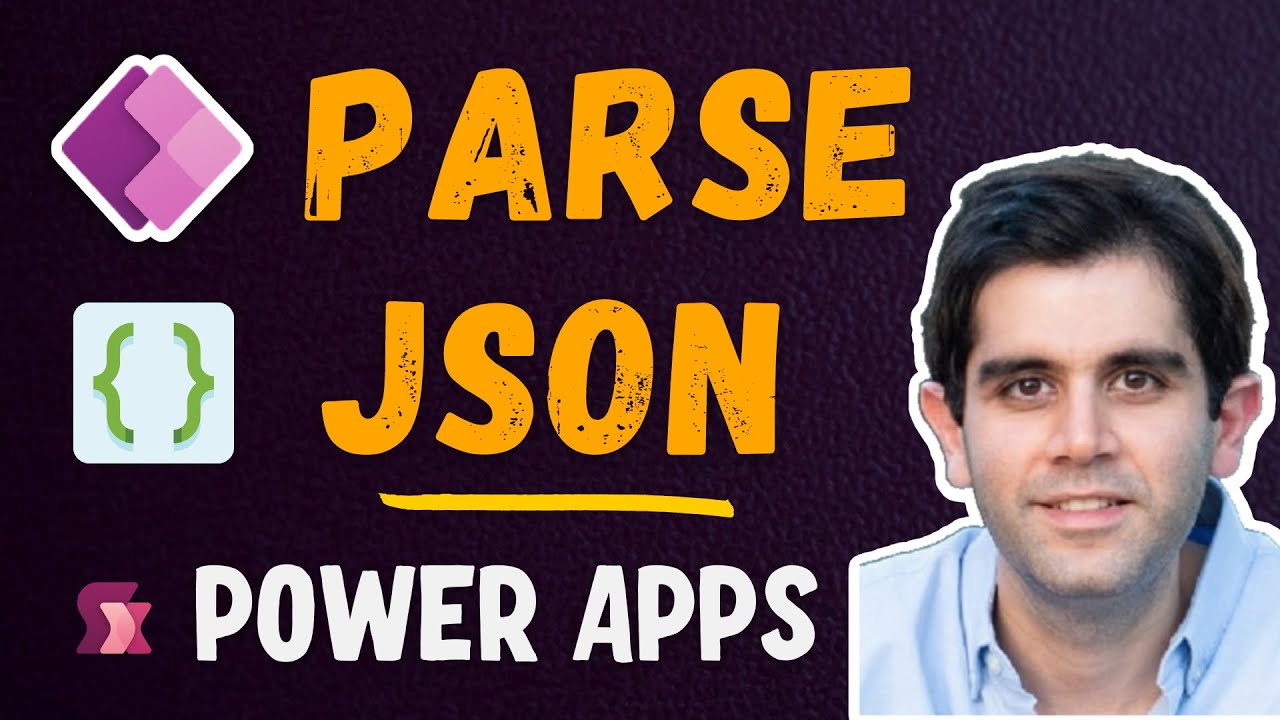
Introduction to Parse JSON in Power Apps | ParseJSON Arrays as Table; Return Array from flow
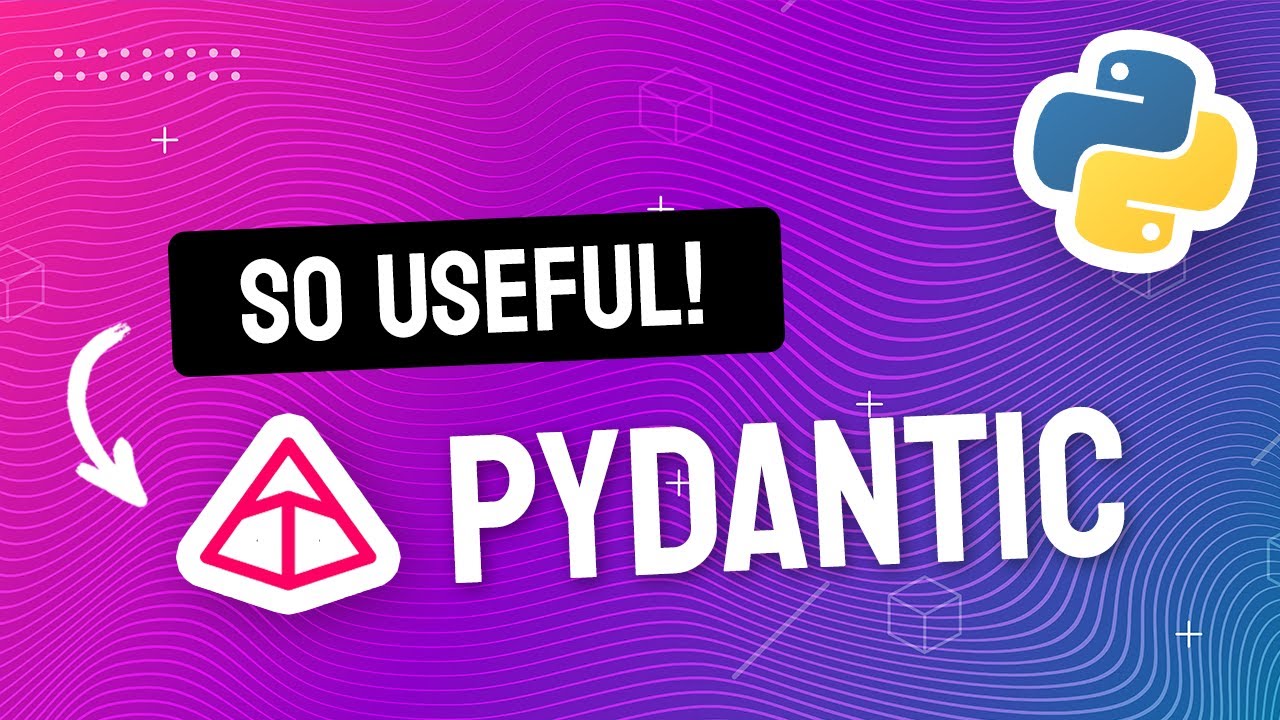
Pydantic Tutorial β’ Solving Python's Biggest Problem
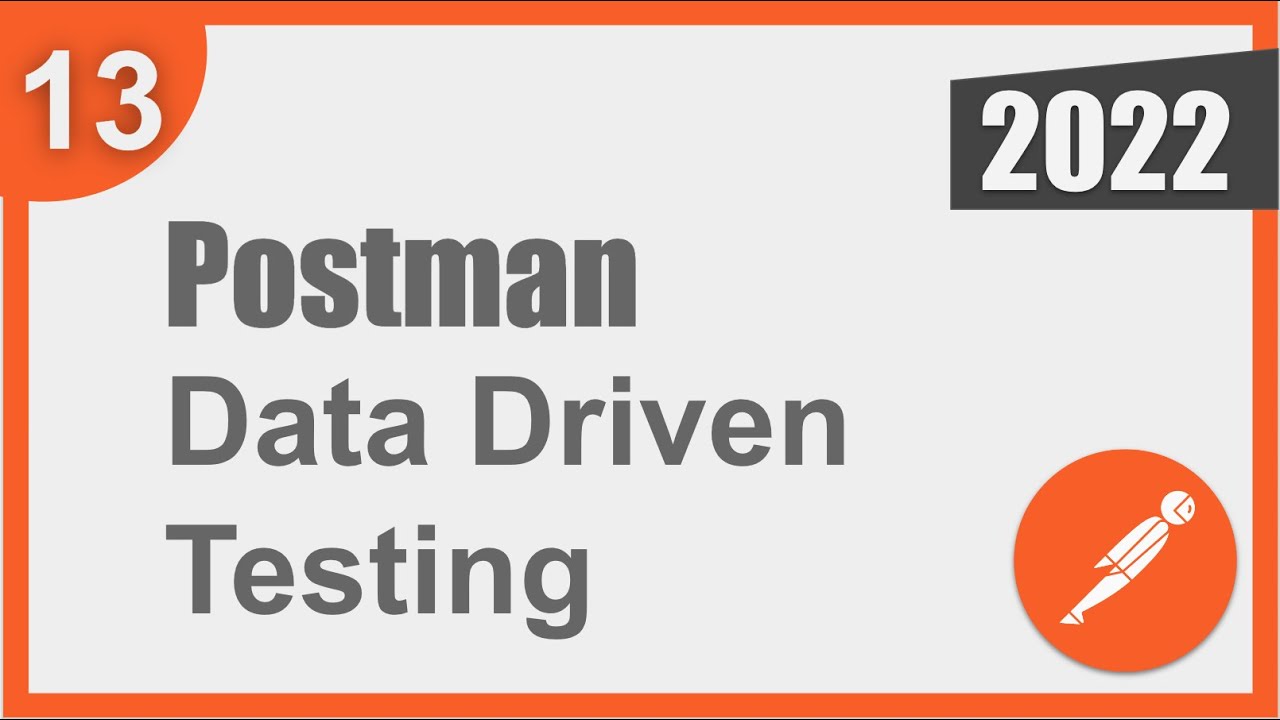
Postman Beginner Tutorial 13 | Data Driven Testing | How to get data from CSV and JSON files
5.0 / 5 (0 votes)