#2 How to change the flow of control through your code
Summary
TLDRIn this lesson on Embedded Systems Programming, Miro Samek teaches how to control the flow of code in C by introducing concepts like loops and conditional statements. Using a simple example, he demonstrates how to implement a while-loop and conditional 'if' statements. The lesson also covers key details about how the processor handles instructions, including how branch instructions modify the program counter (PC). Key insights on optimizing code for performance and avoiding unnecessary overhead, especially in time-critical systems, are also explored. The lesson concludes with a focus on decision-making at runtime and the importance of understanding flow control in embedded programming.
Takeaways
- π Backup your projects regularly by making incremental changes to avoid losing progress.
- π The flow of control in a program is typically linear, but it can be modified to include loops or conditionals.
- π The simplest loop in C is a while-loop, which checks a condition and repeats until the condition is false.
- π The Program Counter (PC) register tracks the current instruction being executed by the processor.
- π ARM processors use a pipeline to improve instruction throughput, but branches can cause pipeline stalls, affecting performance.
- π A single C statement like 'while' can generate multiple machine instructions, affecting code execution and performance.
- π The compiler can optimize control flow by rearranging instructions for better speed and efficiency.
- π Loops, when used in time-critical code, can introduce performance issues due to pipeline stalls and overhead.
- π Loop unrolling can be used to minimize the overhead caused by frequent branching in performance-critical code.
- π The 'if' statement in C allows for conditional execution, which can be used to make decisions based on runtime conditions.
- π Control-flow statements like 'if' can be nested inside other loops or conditionals, providing more flexibility in code design.
Q & A
What is the golden rule of software development mentioned in the script?
-The golden rule is to keep the code working at all times by making only small incremental changes. If something works, save it to avoid losing progress when mistakes are made.
Why is making frequent backup copies of your project important?
-Frequent backups ensure that you can easily revert to a previous working version if something goes wrong, saving you time and effort in fixing broken code.
How does the debugger help in understanding control flow?
-The debugger allows you to step through the code one instruction at a time, showing the program counter (PC) and the state of registers, which helps visualize how the processor handles code execution.
What does the Program Counter (PC) register do?
-The Program Counter (PC) register holds the address of the current instruction being executed. It is updated after each instruction is executed, advancing to the next instruction in sequence.
How does a while loop in C function?
-A while loop in C starts by checking a condition. If the condition is true, the body of the loop executes. Afterward, the condition is checked again. The loop repeats until the condition is false.
What is the purpose of the 'CMP' instruction in the ARM processor?
-The 'CMP' instruction compares two values and sets the Application Program Status Register (APSR) based on the result, such as setting the negative (N) bit if the comparison results in a negative difference.
What does the 'BLT' instruction do in ARM assembly?
-The 'BLT' instruction is a conditional branch that modifies the Program Counter (PC) only if the negative (N) bit in the APSR is set, indicating a 'less than' condition.
How can a loop affect the performance of embedded systems?
-Loops can introduce overhead due to the additional condition checks and jumps. These jumps may cause pipeline stalls, reducing processor throughput, especially in time-critical code like interrupt handling.
What is instruction pipelining in modern processors like ARM Cortex-M?
-Instruction pipelining is a technique where multiple stages of instructions are processed simultaneously. It increases throughput but can lead to pipeline stalls if the instruction flow is disrupted by a branch, causing delays.
What is loop unrolling, and when should it be used?
-Loop unrolling is a technique where the number of iterations within a loop is reduced by increasing the number of operations per iteration. It can speed up execution by reducing branch instructions and overhead, particularly in performance-critical code.
How does a bitwise AND operation help in testing if a number is odd or even?
-A bitwise AND operation with the number 1 tests the least significant bit of the number. If the result is 1, the number is odd; if the result is 0, the number is even.
Can control-flow statements in C be nested, and if so, how?
-Yes, control-flow statements like 'if' can be nested within loops like 'while'. This allows for more complex decision-making and control flow in a program.
Outlines
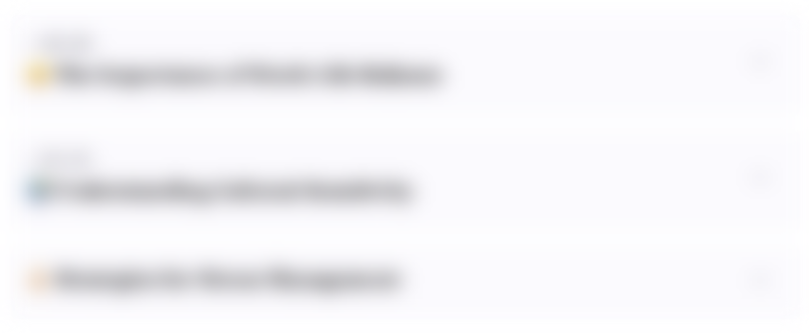
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
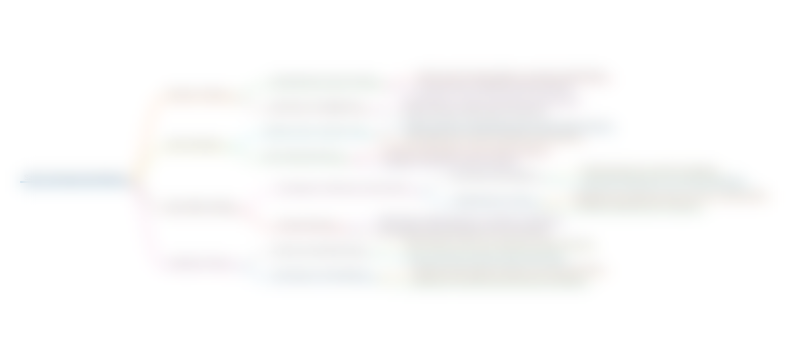
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
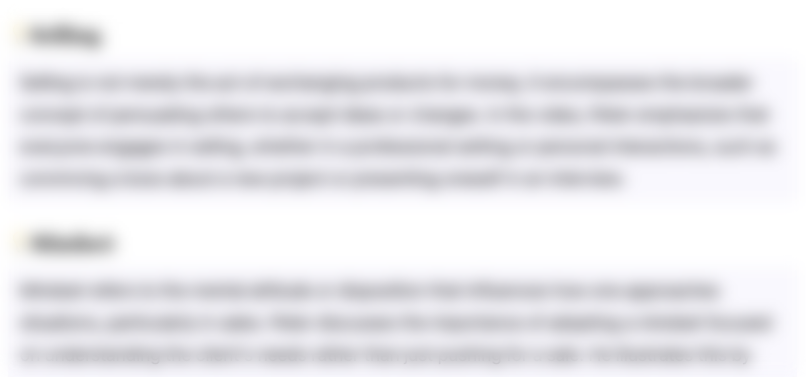
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
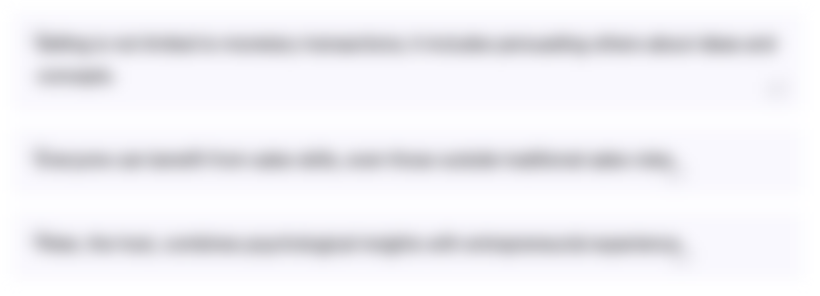
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
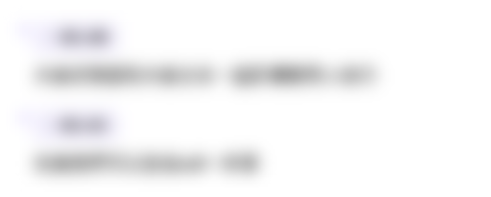
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
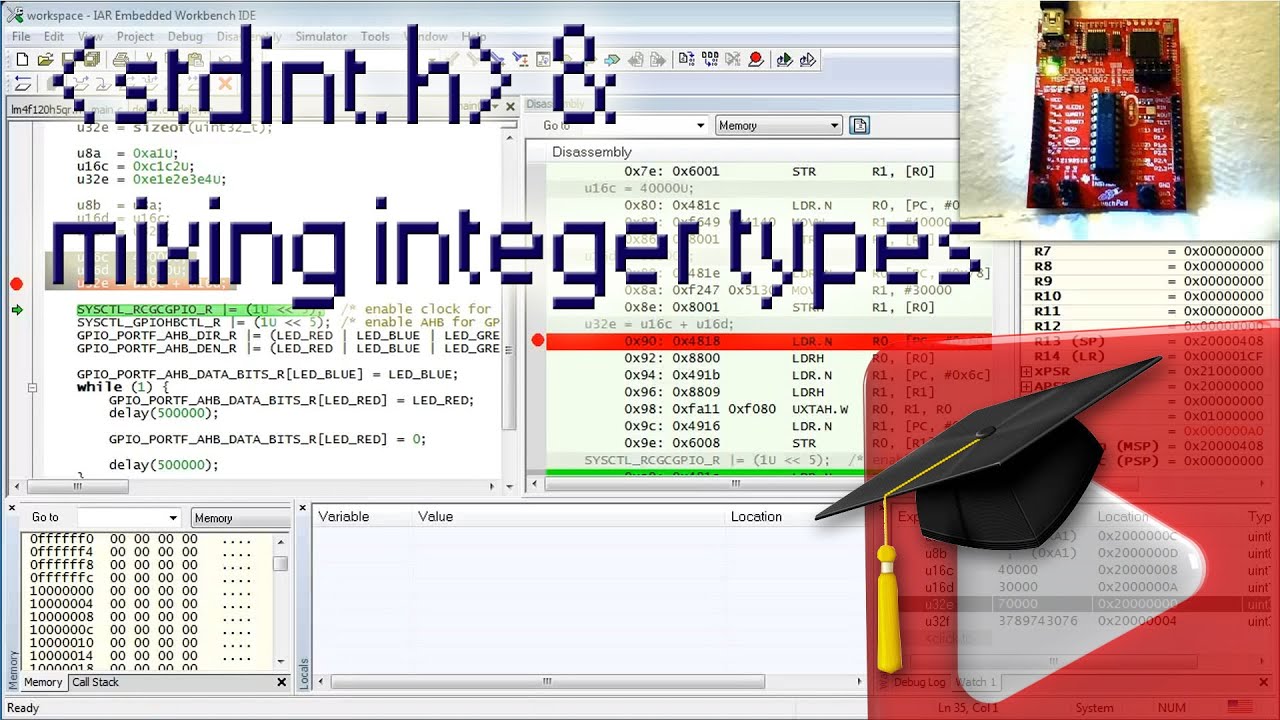
#11 Standard integers (stdint.h) and mixing integer types
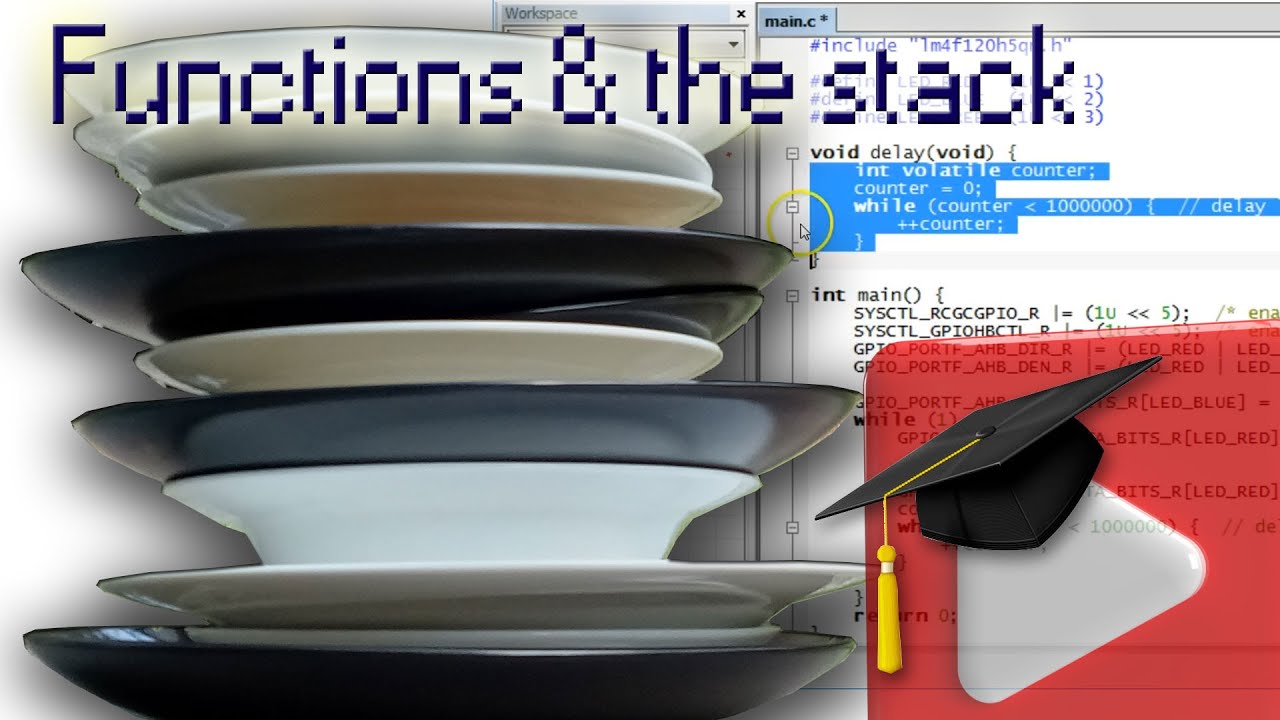
#8 Functions in C and the call stack

#5 Preprocessor and the "volatile" keyword in C

#7 Arrays and Pointer Arithmetic
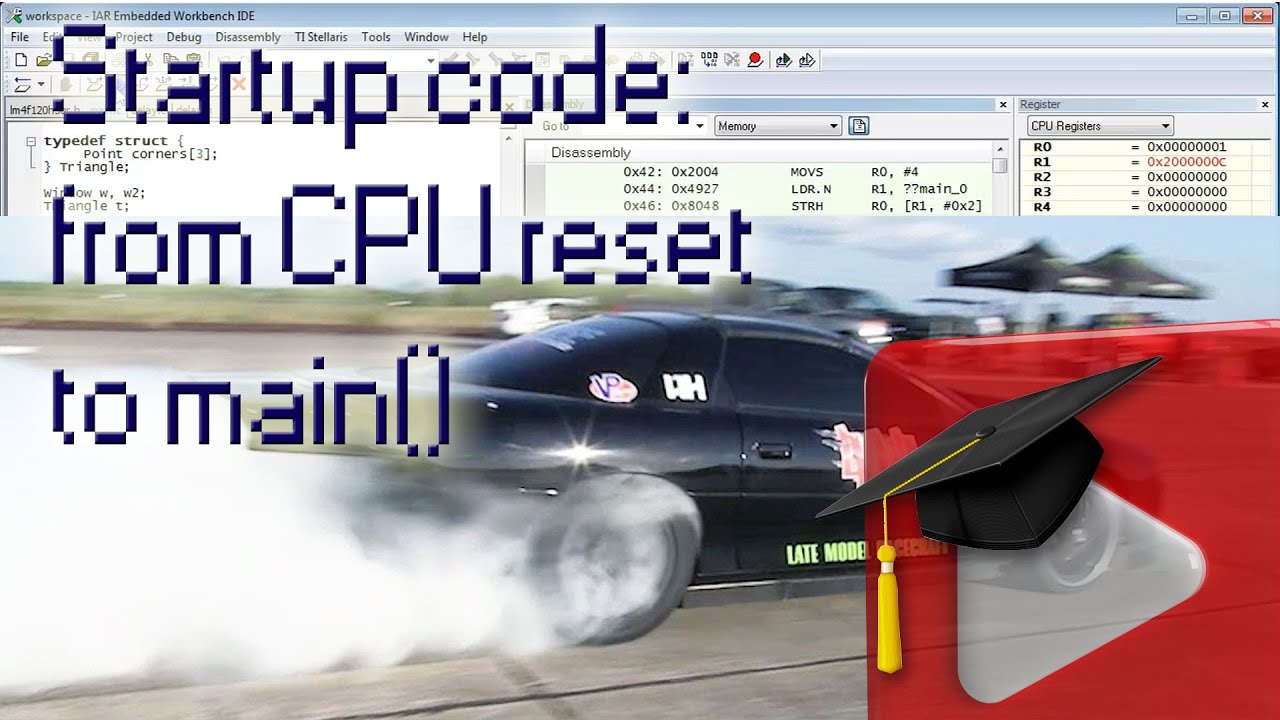
#13 Startup Code Part-1: What is startup code and how the CPU gets from reset to main?
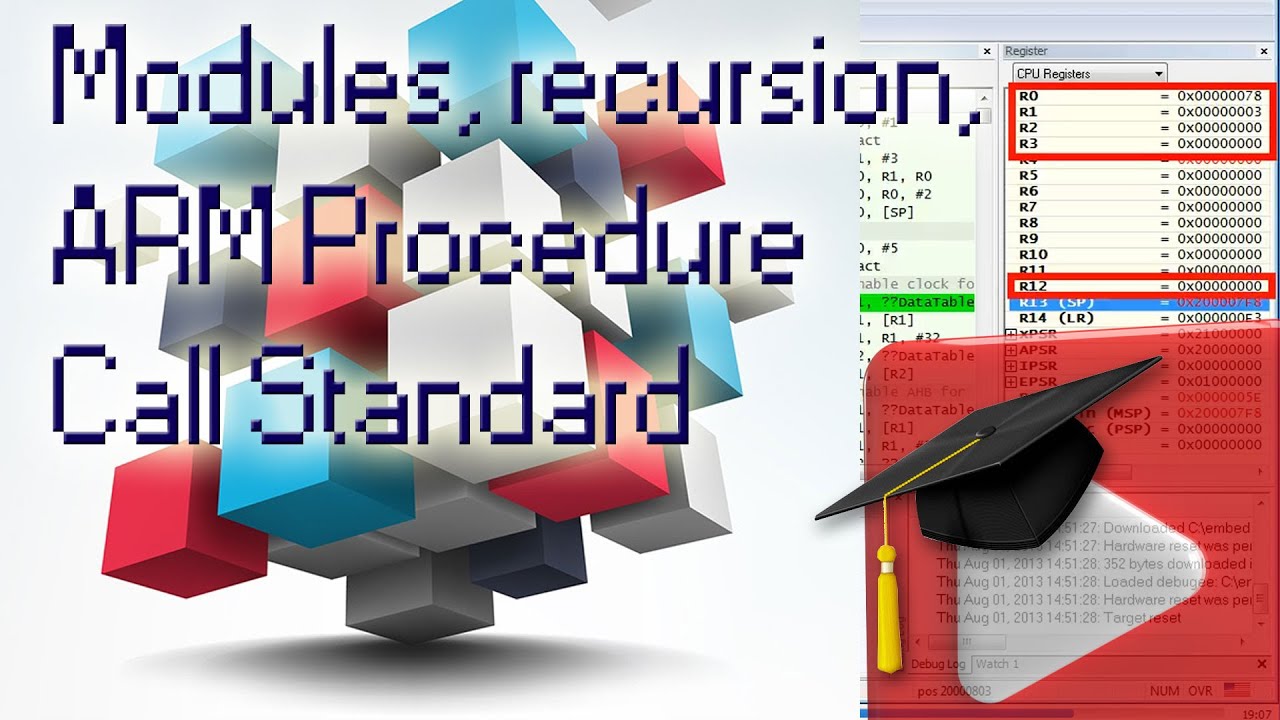
#9 Modules, Recursion, ARM Application Procedure Call Standard (AAPCS)
5.0 / 5 (0 votes)