#6 Bit-wise operators in C
Summary
TLDRIn this lesson on Embedded Systems Programming, Miro Samek introduces the use of bit-wise operators in C to control a composite LED on the Launchpad board. The lesson explores various bit-wise operations, including OR, AND, XOR, NOT, and bit-shifting, and how they can be applied to manipulate GPIO registers efficiently. By using these operators, programmers can set, clear, and toggle individual bits in a register without affecting others. The lesson also covers important coding idioms and shows how to improve program readability, with practical demonstrations on the behavior of signed versus unsigned integers during shifts.
Takeaways
- π Bitwise operators in C are essential for manipulating individual bits in embedded systems, such as controlling LEDs on hardware like the Launchpad board.
- π The bitwise OR operator (`|`) allows you to set a specific bit without affecting others, which is useful for turning LEDs on without disturbing the state of other LEDs.
- π The bitwise AND operator (`&`), combined with the complement (`~`), can be used to clear a specific bit, turning LEDs off without interfering with the others.
- π The XOR operator (`^`) allows you to toggle bits, flipping their state, which can be useful for changing the LED state between on and off.
- π The bitwise NOT operator (`~`) inverts all bits, changing 1s to 0s and 0s to 1s, which is useful for creating masks in bit manipulation.
- π The shift operators (`<<` for left shift and `>>` for right shift) are used to move bits, with the left shift corresponding to multiplication by powers of 2, and the right shift corresponding to division by powers of 2.
- π Right shifts behave differently for signed and unsigned integers. For unsigned integers, zeros are shifted in, but for signed integers, sign extension occurs to preserve the negative value.
- π Using bitwise operators on registers allows for efficient manipulation of hardware control registers (like GPIO), improving the performance of embedded applications.
- π Bitwise operations are compiled into highly optimized machine code, such as the `BIC` instruction for clearing a bit, making them efficient for real-time systems.
- π Defining bit masks using bitwise shifts (e.g., `1 << n`) is a more readable and maintainable method compared to using hexadecimal constants, especially for high-order bits.
- π Understanding bitwise operators and how they interact with hardware is crucial for embedded systems programming, particularly for tasks like setting, clearing, and toggling bits in GPIO registers.
Q & A
What is the primary focus of this lesson?
-The primary focus of this lesson is to teach how to use bit-wise operators in C to control the LEDs on the Launchpad board, particularly to manipulate individual bits in hardware registers without disturbing other bits.
What issue arises when attempting to control the blue and red LEDs simultaneously?
-The issue is that when you set the bit for the red LED, it also clears the bit for the blue LED, as both LEDs share the same GPIO register. This can be avoided by using bit-wise operators.
Why are bit-wise operators important for embedded systems programming?
-Bit-wise operators allow precise manipulation of individual bits in hardware registers, which is essential for controlling hardware like LEDs, sensors, and other peripherals in embedded systems.
What are the six bit-wise operators available in C?
-The six bit-wise operators in C are bit-wise OR, AND, XOR, NOT (one's complement), right shift, and left shift.
How does the bit-wise OR operator work?
-The bit-wise OR operator performs a logical OR operation between corresponding bits of two operands. If either bit is 1, the result is 1; otherwise, it's 0.
What happens when you use the bit-wise AND operator?
-The bit-wise AND operator performs a logical AND operation between corresponding bits of two operands. The result is 1 only when both bits are 1, otherwise the result is 0.
What is the difference between right-shifting signed and unsigned integers?
-When right-shifting signed integers, the shift operation preserves the sign by shifting 1s into the most significant bits if the number is negative (sign-extension). For unsigned integers, zeros are shifted into the most significant bits.
What is the significance of using bit-shift operators to define GPIO bits?
-Using bit-shift operators to define GPIO bits makes the code more readable and prevents errors, especially when working with higher-order bits, as it allows you to directly specify bit positions.
How does the C assignment operator (operator-equals) work with bit-wise operations?
-The operator-equals (e.g., |= or &=) is shorthand for performing a bit-wise operation on a variable and then storing the result back into the variable, simplifying the code.
What is a key takeaway about compiler optimization when using bit-wise operations?
-The compiler can optimize bit-wise operations to generate more efficient machine code, such as using a single machine instruction for clearing a bit, rather than performing a full AND operation with a bit mask.
Outlines
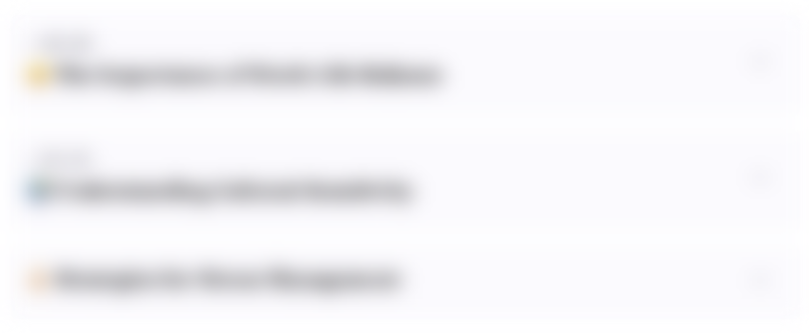
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
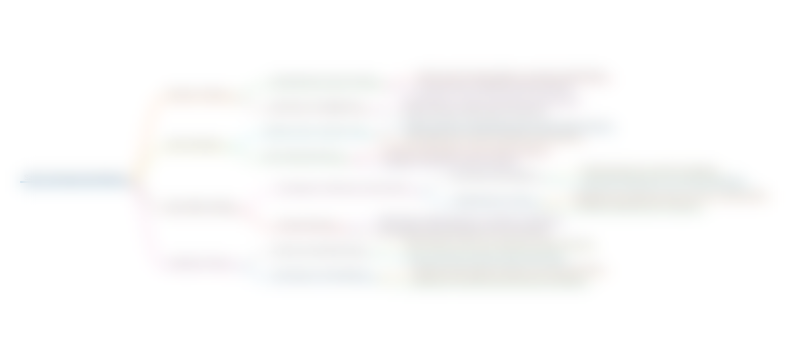
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
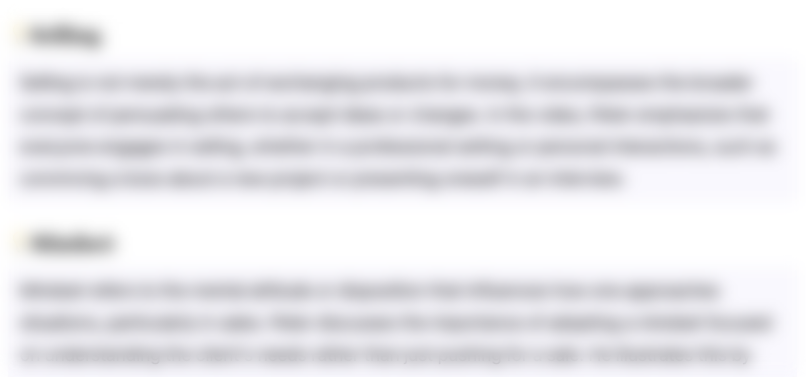
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
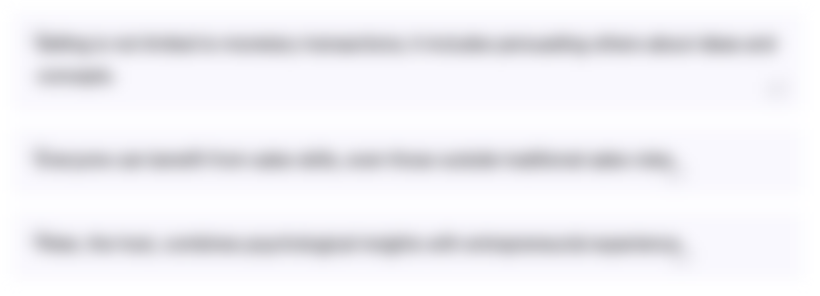
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
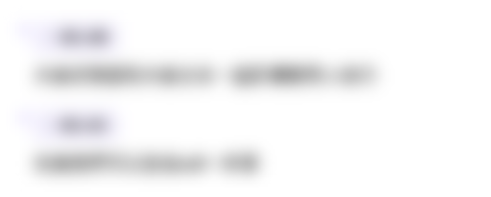
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)