If Else Branching Logic in JavaScript | Learn Basic Coding
Summary
TLDRIn this tutorial, the video introduces JavaScript's if and if-else statements, demonstrating how these control structures help programs make decisions based on conditions. The presenter explains how to use if statements to execute code when a condition is met, using practical examples such as offering free shipping for orders above a certain amount and validating a login form. Additionally, the video covers the use of else to handle cases where conditions are not satisfied, and highlights the importance of comparing both values and data types in conditional checks.
Takeaways
- ๐ The `if` statement in JavaScript allows the program to make decisions based on conditions.
- ๐ The syntax of the `if` statement includes the keyword `if`, followed by a condition in parentheses and a block of code within curly braces.
- ๐ A basic example of an `if` statement is checking if the shopping cart total exceeds a certain amount to apply free shipping.
- ๐ The `else` statement is used to provide alternative actions when the condition in the `if` statement is not met.
- ๐ The comparison operators such as `>` (greater than) and `>=` (greater than or equal to) can be used within `if` statements for numeric comparisons.
- ๐ To compare values in JavaScript, the strict equality operator (`===`) checks both the value and the data type.
- ๐ When a condition is true, the code inside the `if` block is executed, otherwise, the program exits the `if` block.
- ๐ You can combine multiple conditions using logical operators such as `&&` (AND), ensuring that both conditions must be true to execute the code inside the block.
- ๐ Example: The login system requires both the correct username and password, and if either is incorrect, an error message will be displayed using `else`.
- ๐ It is important to ensure that both value and type match when using the strict equality operator, for instance, comparing strings and numbers carefully.
Q & A
What is the purpose of the `if` statement in JavaScript?
-The `if` statement is used to evaluate a condition, and if the condition is true, a block of code inside the statement is executed. It allows programs to make decisions and execute different code based on specific conditions.
How does the `if` statement syntax work in JavaScript?
-The `if` statement syntax consists of the `if` keyword, followed by a condition in parentheses, and a block of code in curly braces. If the condition evaluates to true, the code inside the block is executed.
What is the role of the `else` statement in JavaScript?
-The `else` statement is used to define a block of code that will be executed when the condition in the `if` statement is false. It provides an alternative action to be taken when the initial condition is not met.
Can you explain the example of the online store discount using the `if` statement?
-In the example, the program checks if the total purchase amount (`totalBelanja`) is greater than 100,000. If true, it displays a message for free shipping. If false, a message is shown stating the total is too low for free shipping.
Why is the condition `if (totalBelanja > 100000)` used in the online store example?
-The condition `if (totalBelanja > 100000)` ensures that only customers who have spent more than 100,000 receive free shipping. It checks whether the purchase total meets the specified threshold for the offer.
What is the difference between the `if` and `else` blocks in the login validation example?
-The `if` block checks whether the username is 'admin' and the password is '1234'. If both conditions are true, it displays a success message. The `else` block is executed when either the username or password does not match, showing an error message instead.
What happens when the username or password is incorrect in the login validation example?
-If the username or password is incorrect, the `else` block is triggered, and the message 'Username or password is incorrect' is displayed, indicating a failed login attempt.
Why do we use `===` instead of `==` in the login validation condition?
-The `===` operator checks both the value and the type of the variables. This is important to ensure that the `username` and `password` match exactly, both in value and type. Using `==` would allow type coercion, which could lead to unexpected results.
How can you modify the `if` condition to include a check for `>= 100000` instead of just `> 100000` in the discount example?
-To include purchases exactly equal to 100,000 in the free shipping offer, you can change the condition to `if (totalBelanja >= 100000)`. This ensures that customers with a total purchase of 100,000 or more are also eligible for free shipping.
What would happen if we replaced the `else` block in the examples with another `if` block?
-If we replaced the `else` block with another `if` block, the second condition would be evaluated independently. This could lead to situations where both blocks are executed, even if only one of them should be. The `else` block is specifically meant to handle the case when the initial `if` condition is false, ensuring only one block is executed.
Outlines
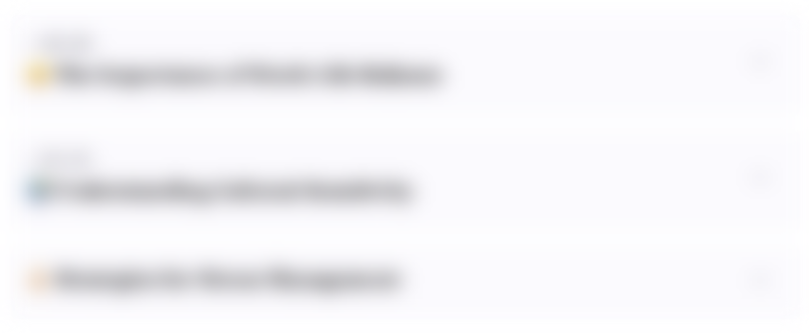
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
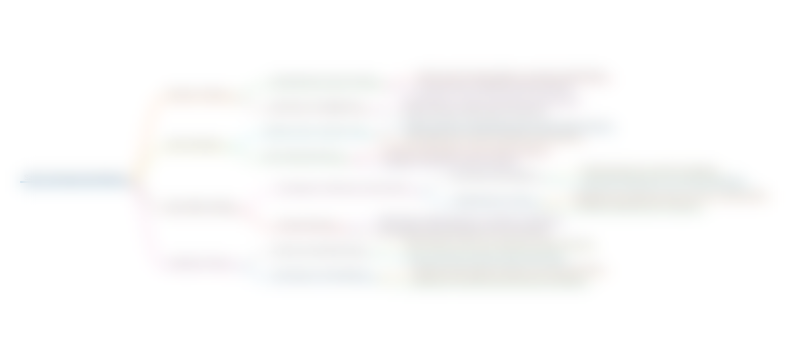
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
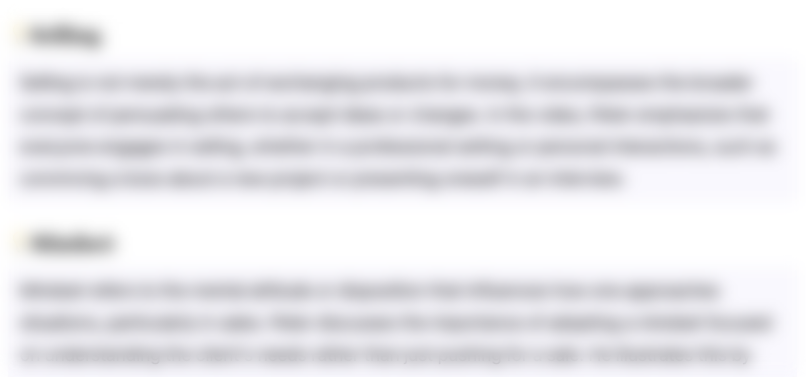
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
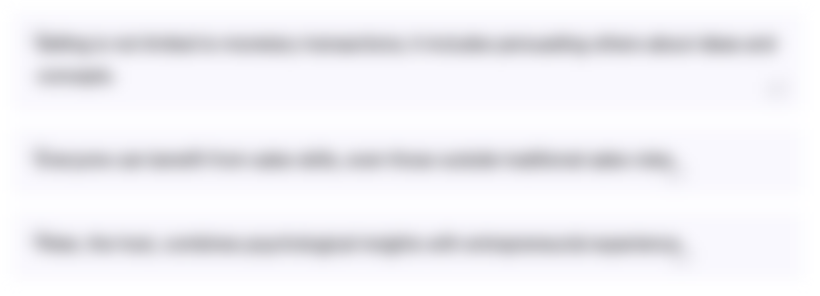
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
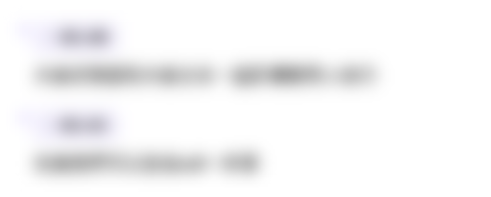
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
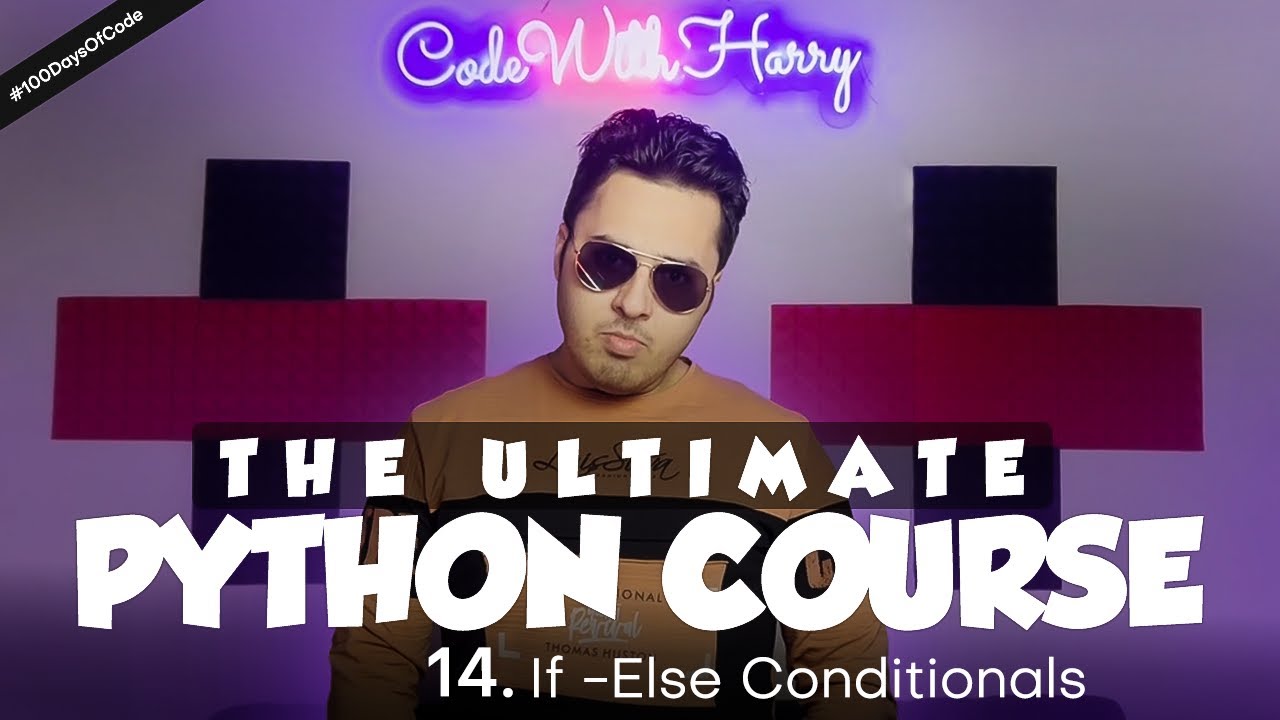
If Else Conditional Statements in Python | Python Tutorial - Day #14
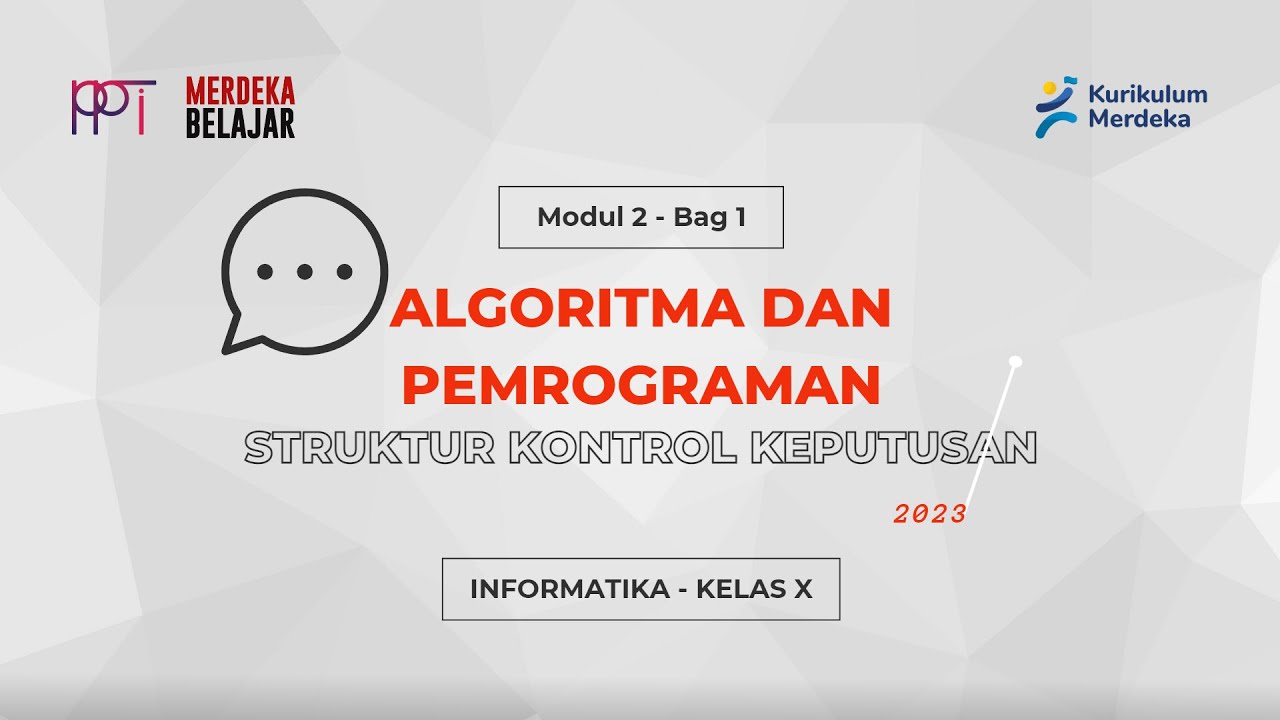
Modul 2 Bag. 1 - Struktur Kontrol Keputusan | Informatika | Kelas X | PPTIKSMAGA
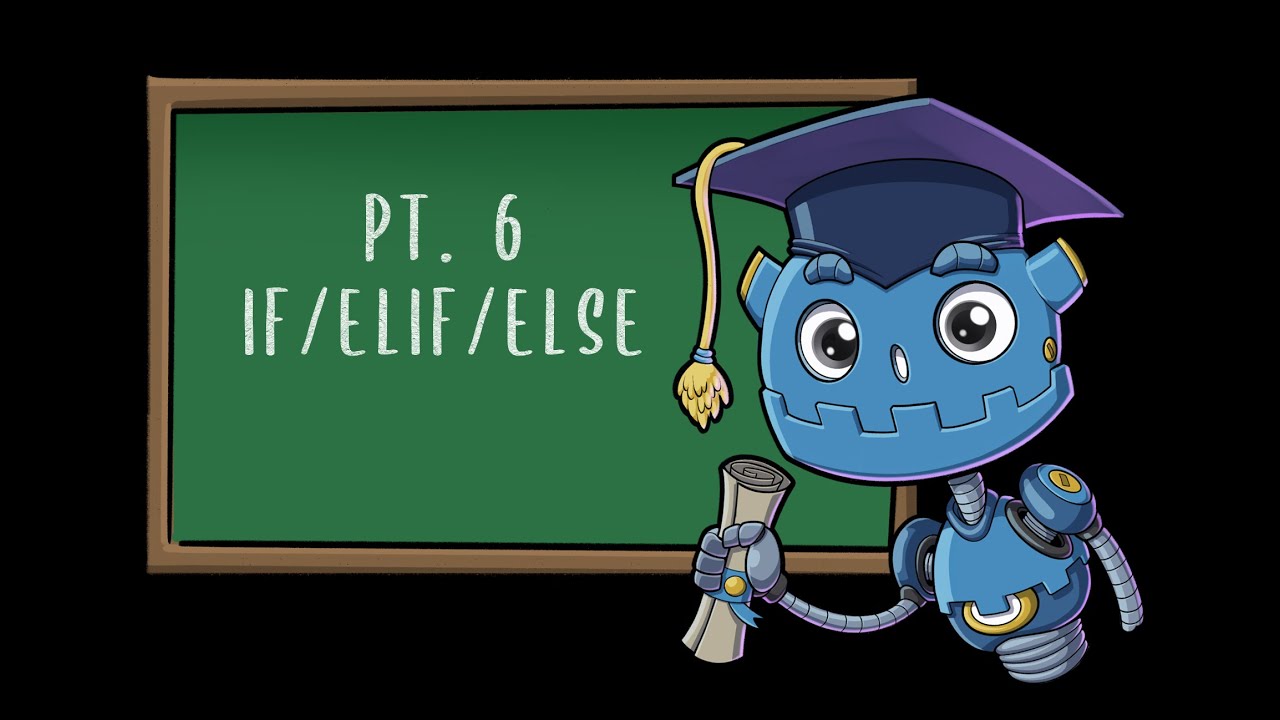
If/Elif/Else Statement Chain | Godot GDScript Tutorial | Ep 06
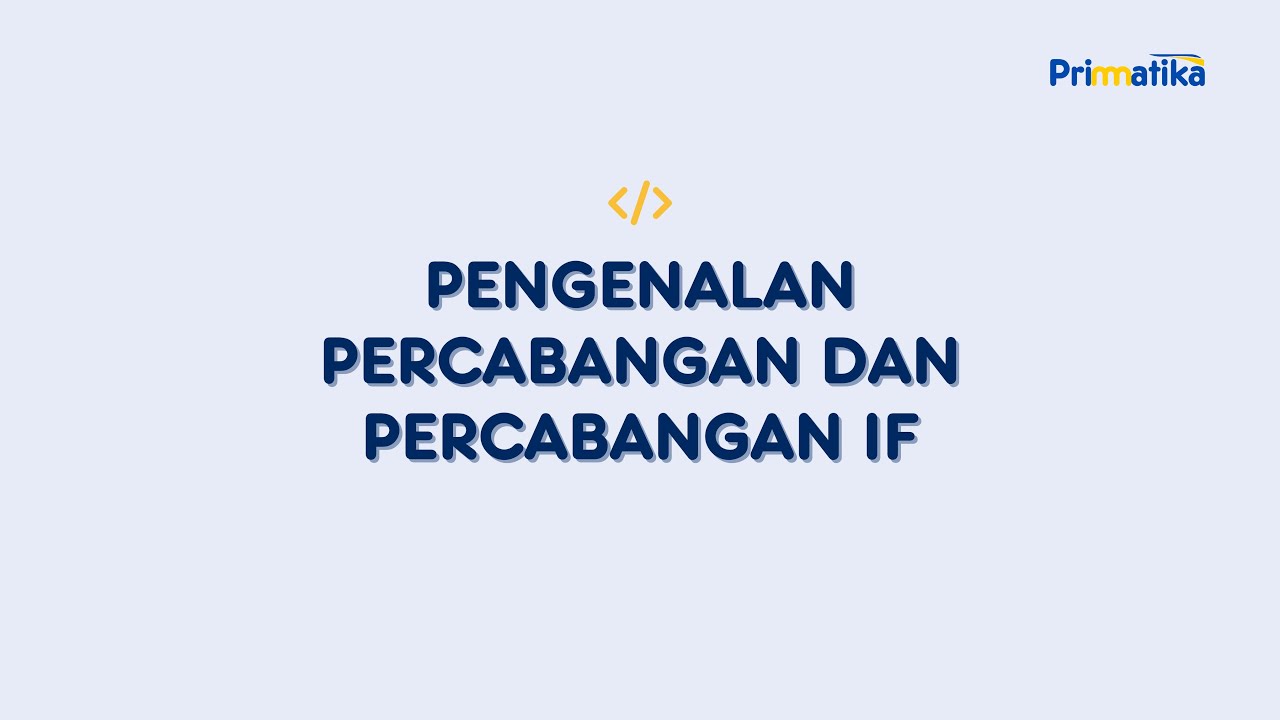
Konsep Dasar Percabangan dan Percabangan IF Bahasa Pemrograman Python
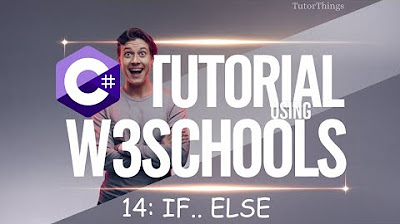
W3Schools | C# Full Course | W3Schools C# | C# Tutorial - Full Course for Beginners | C# Tutorial

Karel Python - if/else
5.0 / 5 (0 votes)