Learn JavaScript Programming Basics: String Operators in JavaScript
Summary
TLDRIn this video, the instructor explains how to work with string operators in JavaScript, focusing on string concatenation. Using examples, the instructor demonstrates how to combine multiple strings using the '+' operator and how to add spaces between strings. The tutorial also highlights how to handle multiple variables and simplifies the process by using template literals. The video aims to teach beginners how to efficiently concatenate strings and understand the differences between string and number operations in JavaScript.
Takeaways
- 😀 String operators in JavaScript are used to combine multiple strings together.
- 😀 The `+` operator is used for string concatenation in JavaScript.
- 😀 If no space is added between strings, the result will show them together without any separation.
- 😀 You can insert spaces between strings by adding a space as a string (`' '`) between variables in the concatenation.
- 😀 Concatenating multiple strings requires multiple uses of the `+` operator to combine them.
- 😀 Template literals (backticks) provide a cleaner and more readable way to concatenate strings.
- 😀 Template literals allow you to embed variables directly within the string using `${}`.
- 😀 Using template literals eliminates the need for multiple `+` signs and quotation marks when concatenating strings.
- 😀 Adding variables to strings using template literals is a more efficient way to format strings with dynamic content.
- 😀 This tutorial emphasizes how to properly concatenate strings and use template literals to simplify JavaScript code.
Q & A
What is the purpose of using string operators in JavaScript?
-String operators in JavaScript are used to combine or concatenate strings, allowing multiple string values to be joined together into a single string.
What is the issue with concatenating strings directly using the '+' operator?
-When concatenating strings directly with the '+' operator, no space is inserted between the strings by default, which results in the strings being joined together without any separation.
How can we add a space between two concatenated strings in JavaScript?
-To add a space between two concatenated strings, simply insert a string containing a space between the variables like this: `string1 + ' ' + string2`.
What happens if you try to use the '+' operator on numbers in JavaScript?
-If you use the '+' operator with numbers in JavaScript, it performs addition rather than concatenation. This behavior only applies to numerical data types.
How can we concatenate more than two strings in JavaScript?
-To concatenate more than two strings, you simply continue using the '+' operator between each string. For example: `string1 + ' ' + string2 + ' ' + string3`.
What is the advantage of using template literals over the '+' operator for string concatenation?
-Template literals, which use `${}` syntax, provide a cleaner and more readable way to concatenate strings, especially when dealing with multiple variables, making the code easier to maintain.
What syntax is used to create template literals in JavaScript?
-Template literals are created using backticks (`` ` ``) and the variables are embedded using `${}` syntax, like this: `` `${string1} ${string2}` ``.
Can the '+' operator be used for string concatenation and addition at the same time?
-Yes, the '+' operator in JavaScript behaves differently depending on the data type. If used with strings, it performs concatenation, and if used with numbers, it performs addition.
How do you display the concatenated result of variables in the browser using JavaScript?
-To display the result of concatenated variables in the browser, you can use `document.write()`. For example: `document.write(string1 + ' ' + string2)`.
What happens if you use template literals instead of the '+' operator in the given example?
-If you use template literals instead of the '+' operator, the output will be the same, but the code will be cleaner and easier to read, especially when concatenating multiple variables.
Outlines
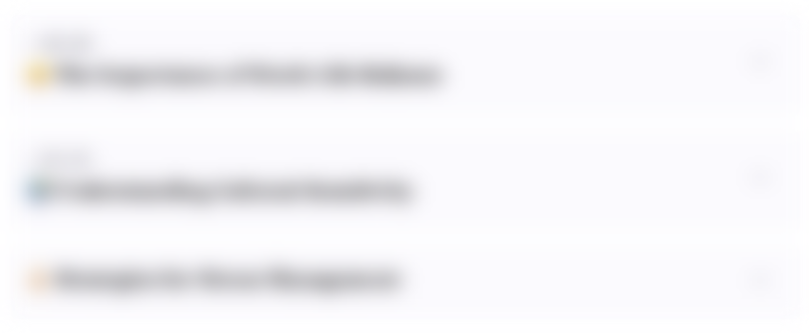
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
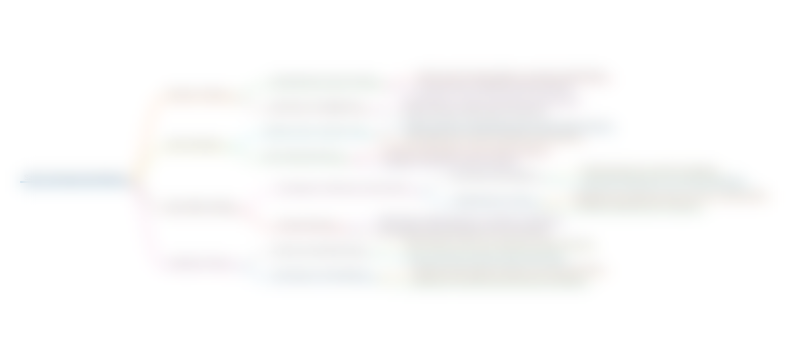
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
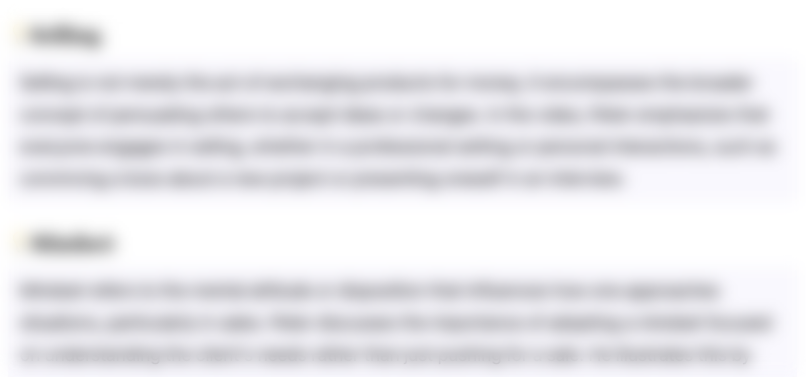
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
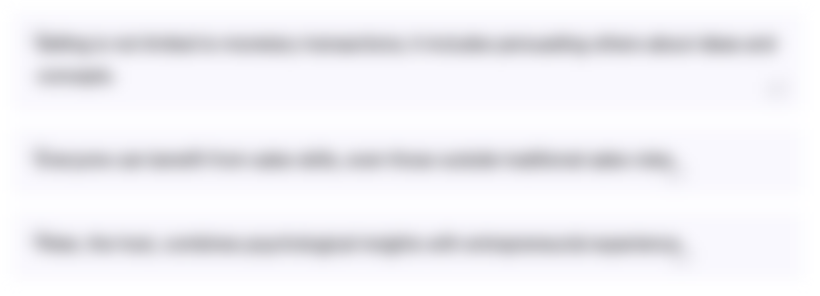
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
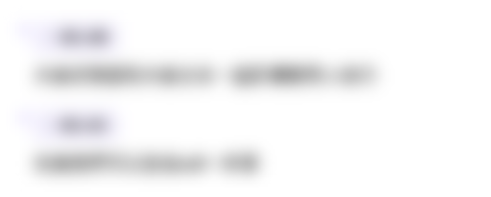
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

☕️ Curso de Java na prática - Operadores Aritméticos - Atribuições - aula 6 - Fundamentos Parte 2/5

Belajar Python [Dasar] - 16 - Operasi dan manipulasi string (part 1)

BAID MK1 P3
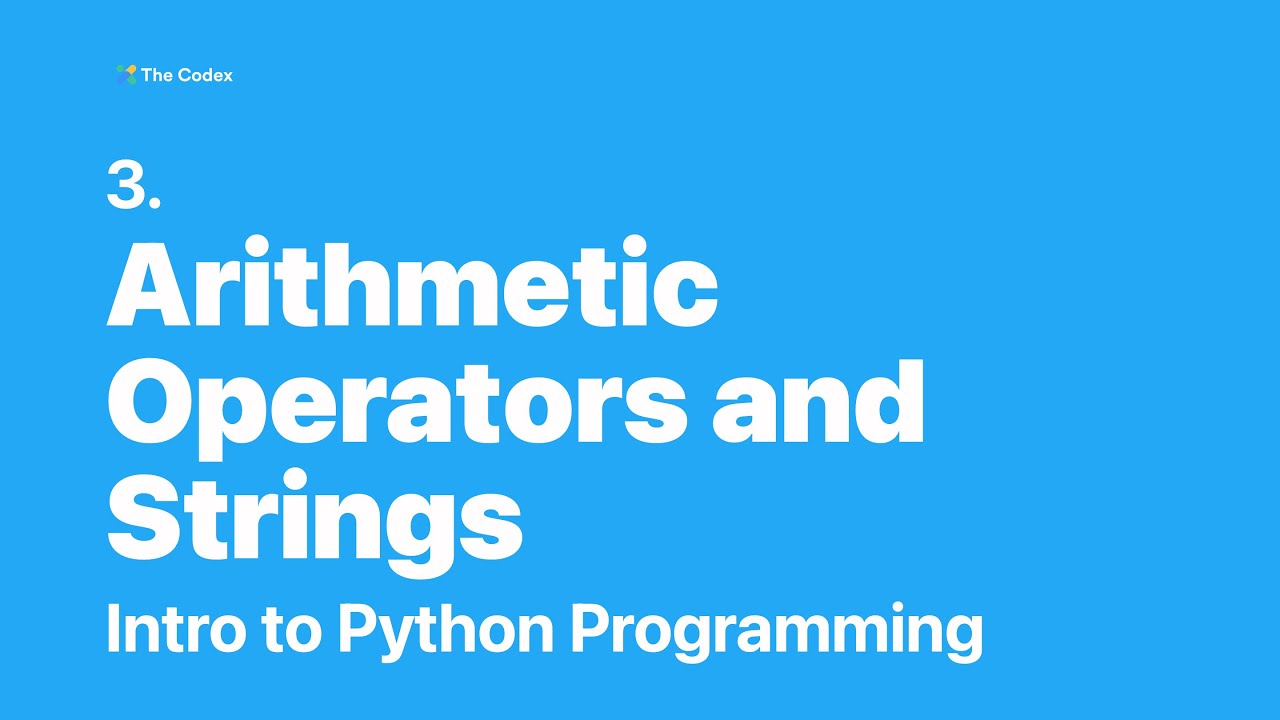
Python Programming #3 - Arithmetic Operators and Strings
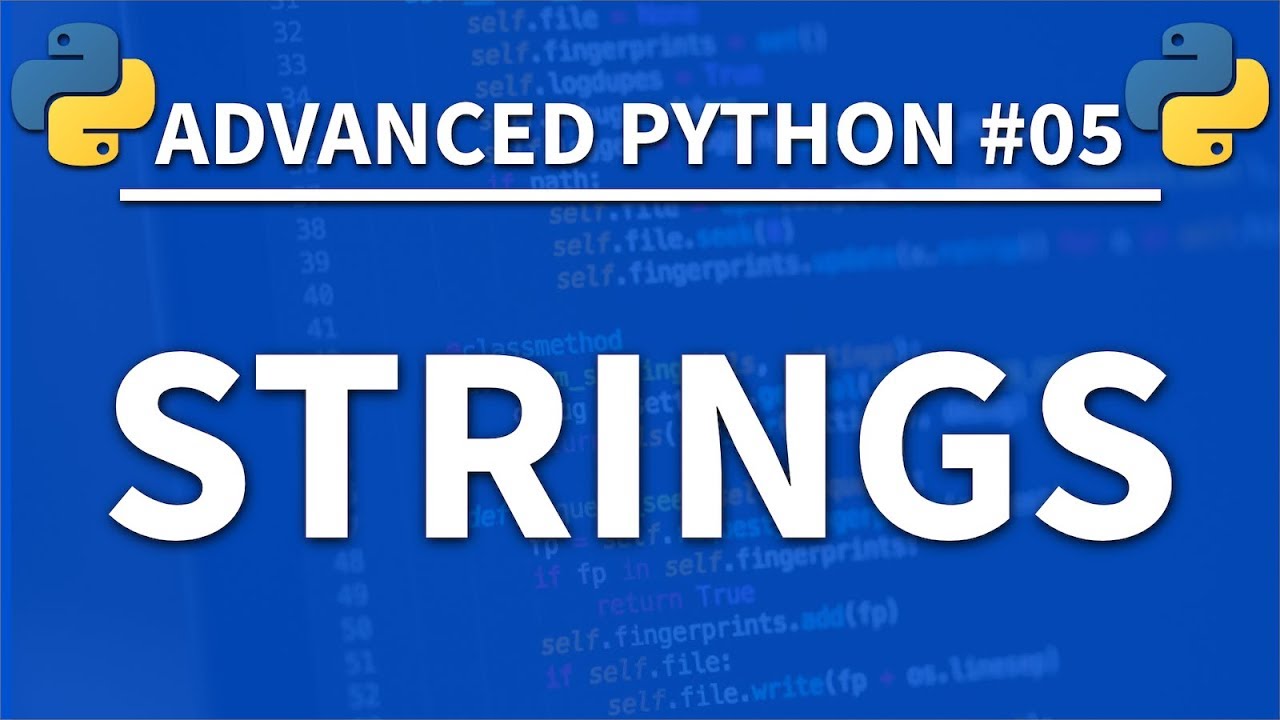
Strings in Python - Advanced Python 05 - Programming Tutorial

Codecademy: "Learn Javascript" Walkthrough | Variables: 1 - 10
5.0 / 5 (0 votes)