C++ Super Optimization: 1000X Faster
Summary
TLDRIn this video, Dave, a retired operating systems engineer, explores the powerful C++ feature `constexpr` and its ability to optimize code performance by allowing compile-time evaluation. He demonstrates how this feature can drastically reduce execution time—up to 1,000 times faster—using examples like the Fibonacci algorithm and the Sieve of Eratosthenes. Dave delves into the benefits of `constexpr` for recursive functions, complex algorithms, and large computations, highlighting its potential for improving runtime efficiency and scalability. The video also touches on the limitations and real-world applications of `constexpr`, encouraging developers to rethink optimization strategies in their own code.
Takeaways
- 😀 The 'constexpr' keyword in C++ can significantly increase code speed by evaluating expressions at compile time, potentially improving performance by up to 1,000 times.
- 😀 'constexpr' functions are evaluated by the compiler at compile time, meaning no runtime computation is required, leading to faster execution.
- 😀 While 'constexpr' is often associated with constants, it can also be used for more complex expressions, including recursion, under specific conditions.
- 😀 The Fibonacci sequence, a common problem in computer science, can be implemented in a 'constexpr' function to drastically improve performance compared to a recursive solution.
- 😀 Traditional recursive solutions for Fibonacci have exponential time complexity (O(2^n)), but using 'constexpr' enables linear-time computation at compile time.
- 😀 By adding 'constexpr' to a function, such as the Fibonacci function, the compiler can collapse the entire recursive logic into a constant value, reducing the need for actual function calls.
- 😀 The Fibonacci sum example demonstrated how the compiler can optimize code by calculating results at compile time, turning a recursive function into a single move instruction in the generated assembly.
- 😀 The 'constexpr' approach can be applied to more complex problems, such as the Sieve of Eratosthenes for finding prime numbers, where the entire algorithm can be computed at compile time.
- 😀 Modern compilers, like Clang, are capable of advanced optimizations that allow even complex recursive functions like Fibonacci to be fully evaluated at compile time.
- 😀 While 'constexpr' is a powerful tool, there are practical limitations such as recursion depth and memory usage, which compilers impose to avoid excessive resource consumption during compilation.
Q & A
What is the purpose of the 'constexpr' keyword in C++?
-The 'constexpr' keyword in C++ allows the compiler to evaluate a function or variable at compile time, meaning the value is determined during the compilation process rather than at runtime. It helps in optimizing performance by reducing the amount of work done at runtime.
How can 'constexpr' improve the performance of code?
-By using 'constexpr', the code can be evaluated at compile time, leading to faster execution at runtime. This reduces runtime calculations and can even replace complex functions with pre-calculated results, making the code more efficient.
What is the difference between 'constexpr' and a regular constant in C++?
-A regular constant, defined using 'const', can only be assigned a value at runtime, while 'constexpr' constants are evaluated at compile time. 'constexpr' enables more advanced optimizations as the compiler can compute values ahead of time.
Why did the Fibonacci function with 'constexpr' run significantly faster than the regular recursive one?
-The 'constexpr' version of the Fibonacci function allows the compiler to evaluate the Fibonacci sequence at compile time, avoiding the expensive recursion during runtime. This drastically reduces the computation time from milliseconds to microseconds.
What are some practical limitations of 'constexpr' functions?
-Although 'constexpr' functions can provide significant performance benefits, they have practical limitations, such as recursion depth limits and the memory constraints of the compiler's evaluation environment. If the computations require excessive resources, the compiler may not be able to complete the evaluation.
What is the Fibonacci sequence and how is it used in the script?
-The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, starting from 0 and 1. In the script, it's used as an example to show how 'constexpr' can optimize a recursive function by calculating Fibonacci numbers at compile time.
Why is recursion considered inefficient for calculating Fibonacci numbers?
-Recursion is inefficient for Fibonacci numbers because it leads to redundant calculations. Each function call generates two more calls, resulting in an exponential growth in the number of calls, which increases the time complexity significantly.
What optimization technique can be used to improve recursive Fibonacci calculations?
-One common optimization is memoization, where previously calculated Fibonacci values are stored and reused to avoid redundant calculations. However, the script demonstrates that 'constexpr' can achieve an even more efficient solution by computing values at compile time.
How does the Sieve of Eratosthenes algorithm work to find prime numbers?
-The Sieve of Eratosthenes algorithm works by iteratively marking the multiples of each prime number starting from 2. Numbers that remain unmarked are prime. The algorithm continues until the square root of the maximum number is reached, at which point all primes are identified.
Can complex algorithms like the Sieve of Eratosthenes be implemented using 'constexpr' functions?
-Yes, the script demonstrates that even complex algorithms like the Sieve of Eratosthenes can be implemented using 'constexpr' functions. The compiler can evaluate the entire sieve process at compile time, leading to instant results and efficient prime number generation.
Outlines
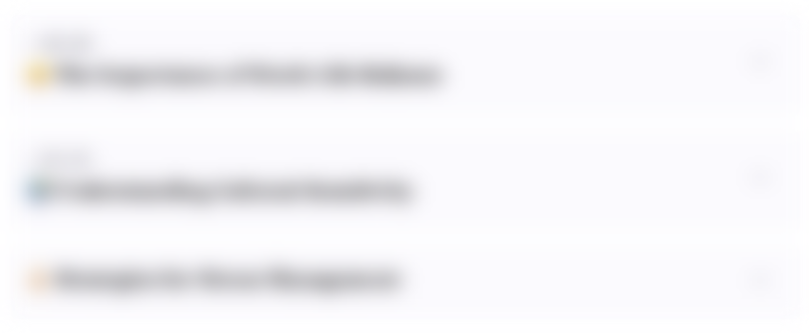
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
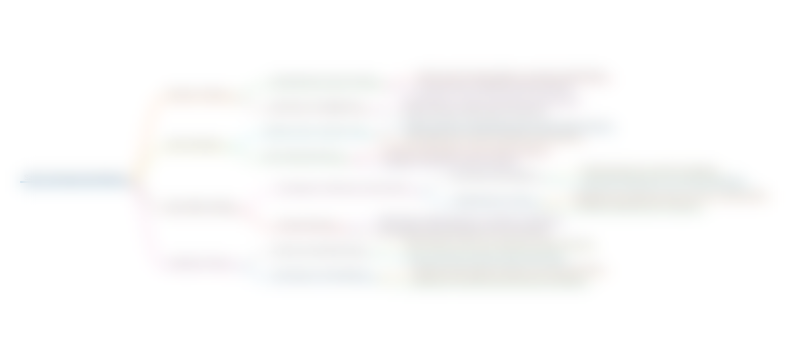
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
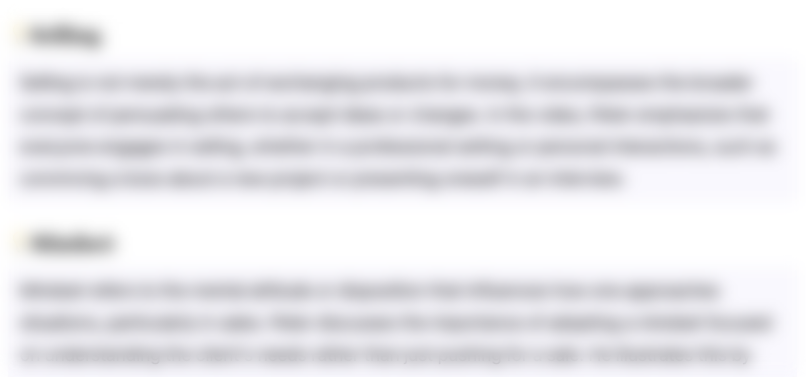
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
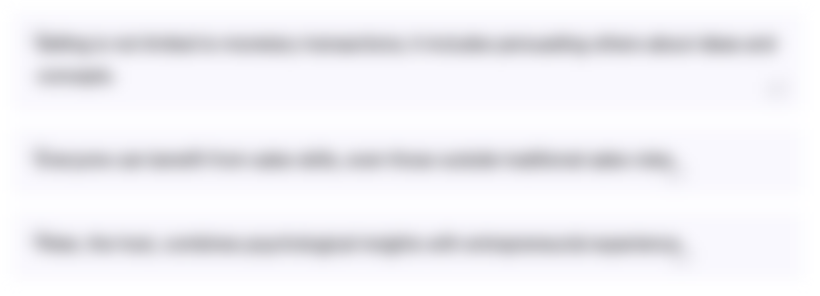
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
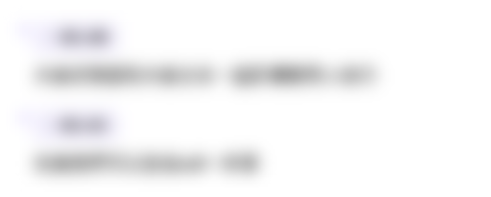
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)