JavaScript performance is weird... Write scientifically faster code with benchmarking
Summary
TLDRIn this video, the legendary programmer Rob Pike's rules of programming are explored, emphasizing the importance of measuring performance before optimizing code. The presenter benchmarks various methods for looping through arrays in JavaScript, revealing that traditional `for` loops outperform others in speed for large datasets. The video also highlights the efficiency of using `Set` for searching values compared to `array.includes`. Finally, it examines sorting algorithms, showcasing quicksort's advantages over built-in methods, and underscores the necessity of building functional code before optimizing based on measured performance.
Takeaways
- 😀 The first rule of programming: do not talk about programming; focus on writing code.
- 📏 Measure performance before optimizing your code to identify true bottlenecks.
- ⚠️ Premature optimization is dangerous; wait until you've measured performance.
- 🔄 There are multiple ways to loop over arrays in JavaScript, each with different performance characteristics.
- 🚀 Traditional `for` loops are generally faster than higher-level functions like `forEach` and `reduce` for large datasets.
- 📊 Use the Doo benchmarking tool to compare performance of different coding methods effectively.
- 📦 Using a Set can significantly improve lookup times compared to using `Array.includes`, especially with large datasets.
- 🔍 The performance of sorting algorithms can vary based on the data type; quicksort is typically the fastest for numbers.
- 🛠️ Simple algorithms are less prone to bugs and easier to implement than complex ones.
- 📈 Built-in JavaScript methods often provide sufficient performance for most applications; prioritize getting your code to work first.
Q & A
What are the five rules of programming mentioned in the script?
-The five rules are: 1) Do not talk about programming, 2) Measure, don't tune for speed until you've measured, 3) Avoid premature optimization, 4) Use simple algorithms over fancy ones, and 5) Understand that you can't predict where bottlenecks will occur.
Why is measuring performance important before optimizing code?
-Measuring performance is crucial because it helps identify actual bottlenecks, allowing programmers to avoid wasting time on optimizations that don't address the real issues.
What is the traditional method of looping over an array in JavaScript?
-The traditional method is using a 'for' loop, where a variable is incremented to iterate through the array's indices.
How does the performance of different array loop methods compare?
-The traditional 'for' loop is generally faster than methods like 'forEach' and 'reduce' because it has less overhead from function calls and abstractions.
What is the significance of using a Set for searching values in an array?
-Using a Set allows for O(1) performance when checking for the existence of a value, as it indexes unique values, making searches significantly faster than the O(n) performance of array includes.
What sorting algorithms were compared in the video, and which performed best?
-The algorithms compared were bubble sort, quick sort, and merge sort. Quick sort generally performed the best, being nearly three times faster than the built-in array sort method for numeric data.
What is the drawback of using complex sorting algorithms in JavaScript?
-Complex algorithms can be buggier and harder to implement than simpler ones, and for most use cases, JavaScript's built-in sorting methods are sufficiently fast.
What tool is recommended for benchmarking JavaScript code?
-Dino, specifically the built-in benchmarking tool Doo, is recommended for benchmarking JavaScript code efficiently.
What lesson about optimization does the video emphasize?
-The video emphasizes that optimization should come after ensuring the code works correctly and should be guided by actual performance measurements rather than assumptions.
Why might performance vary based on the size of the data set when looping or searching?
-Performance can vary significantly with data size due to the differences in algorithmic complexity, as larger datasets often amplify the advantages of more efficient algorithms.
Outlines
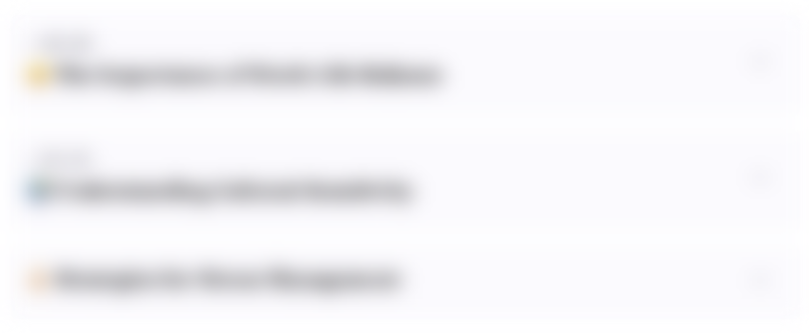
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
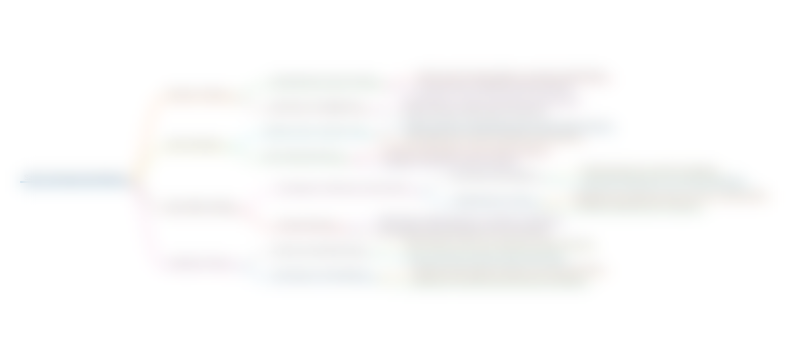
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
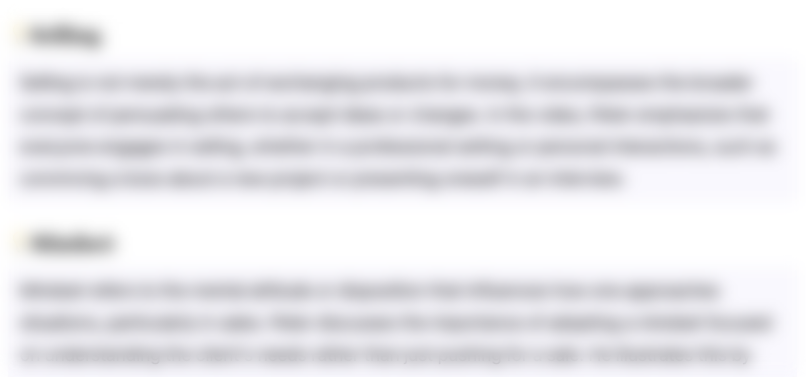
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
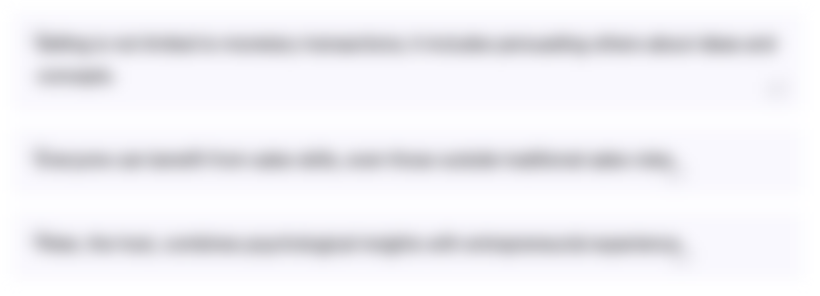
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
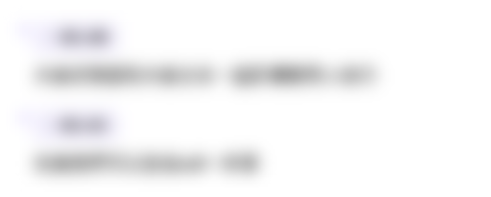
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Make Your Pandas Code Lightning Fast
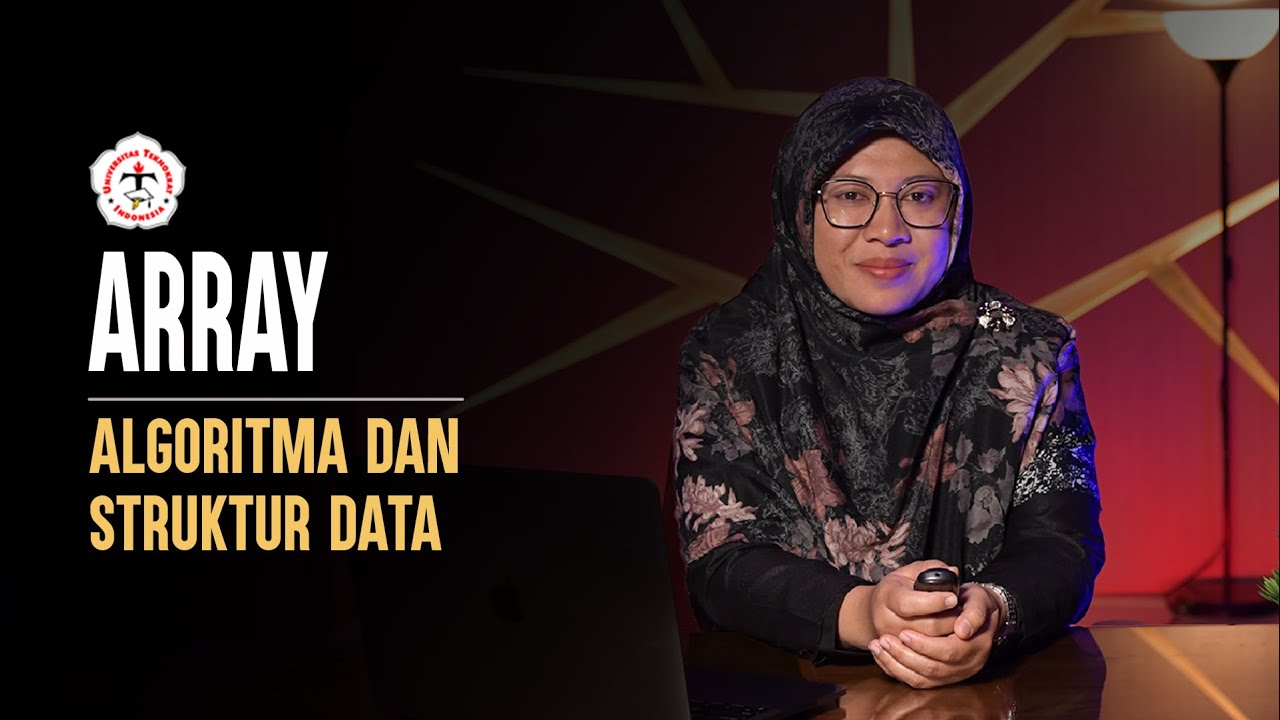
Penerapan ARRAY pada Algoritma dan Struktur Data

Introducción - 1 - Estructuras de Datos en C#
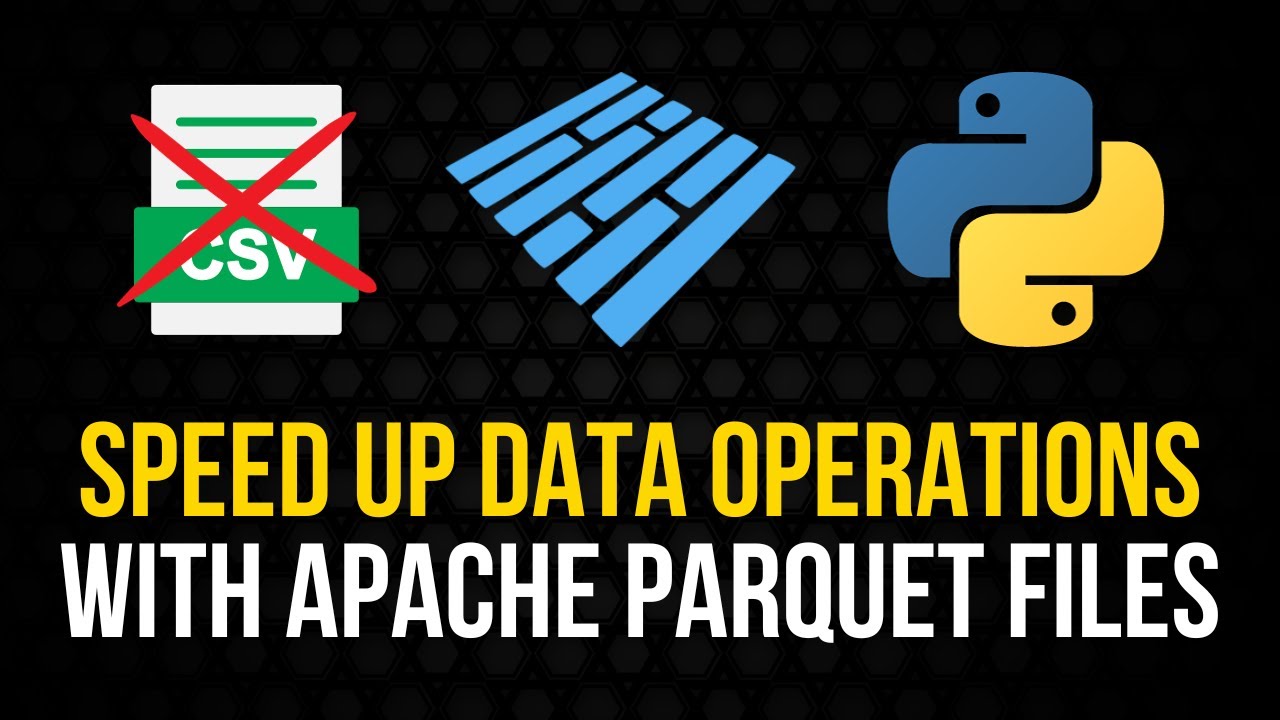
Speed Up Data Processing with Apache Parquet in Python

Basics of Time Complexity and Space Complexity | Java | Complete Placement Course | Lecture 9

8 Data Structures Every Programmer Should Know
5.0 / 5 (0 votes)