= vs == vs === in App Lab (Code.org)
Summary
TLDRIn this tutorial, we explore the differences between the assignment operator (`=`) and the equality operators (`==` and `===`) in JavaScript. The single equal sign is used for assignment, setting a value to a variable. The double equal sign checks if two values are the same, without considering their data types, while the triple equal sign compares both the value and data type. The tutorial uses various examples to demonstrate how these operators work, highlighting the importance of understanding when to use each one for accurate comparisons.
Takeaways
- ๐ The single equal sign (`=`) is used for variable assignment in JavaScript.
- ๐ The double equal sign (`==`) checks if two values are equal, but it doesn't consider their data types.
- ๐ The triple equal sign (`===`) checks both the value and the data type for equality.
- ๐ The value `15` assigned to variable `a` and variable `b` are the same, but their comparison using `==` will depend on data type.
- ๐ A string like `"15"` is treated differently from a number `15` even though the values appear the same.
- ๐ The double equal sign (`==`) will return `true` for `a == b` and `a == c`, but for different data types like a number and a string, `==` still returns `true` when the values match.
- ๐ The triple equal sign (`===`) is stricter and returns `false` if the data types differ, even if the values are the same.
- ๐ In the case of comparing `a = 15` and `d = "hello"`, both `==` and `===` will return `false` due to mismatched values and types.
- ๐ Modifying variable `b` to `20` and comparing `a == b` shows that `==` checks for value equality and returns `false` when values differ.
- ๐ The key distinction between `==` and `===` is that `===` does not allow type coercion, requiring both values and types to match exactly.
Q & A
What does the single equal sign (=) do in JavaScript?
-The single equal sign (=) is an assignment operator. It is used to assign a value to a variable.
What does the double equal sign (==) do in JavaScript?
-The double equal sign (==) checks if the values of two variables are the same, without considering their data types.
What is the purpose of the triple equal sign (===) in JavaScript?
-The triple equal sign (===) checks if both the value and the data type of two variables are the same.
How does JavaScript treat the comparison between a number and a string with the same value using the double equal sign?
-Using the double equal sign, JavaScript checks if the values are the same, but it does not consider the data type, so a number and a string with the same value would be considered equal.
Why does the triple equal sign return false when comparing a number and a string with the same value?
-The triple equal sign returns false because it checks both the value and the data type. A number and a string with the same value have different data types, so they are not considered equal.
What happens when you compare a variable with a different value using the double equal sign?
-When comparing variables with different values using the double equal sign, it will return false, regardless of their data types.
What role do quotation marks play when declaring a variable with a string in JavaScript?
-Quotation marks are used to indicate that the value is a string, meaning the data type is text, not a number.
What happens when a number and a string with different values are compared using the double equal sign?
-When a number and a string with different values are compared using the double equal sign, the result will be false because their values do not match.
What is the difference between using a single equal sign and a double equal sign in JavaScript?
-The single equal sign (=) is used for assignment, while the double equal sign (==) is used for comparison of values.
Can the double equal sign be used to compare both the value and data type in JavaScript?
-No, the double equal sign only compares the values of variables, not their data types. For data type comparison, the triple equal sign (===) should be used.
Outlines
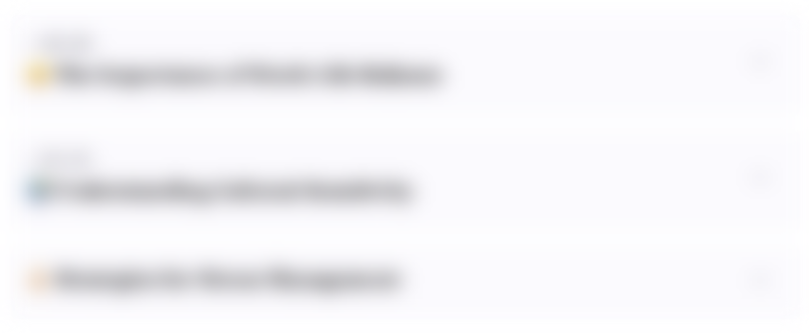
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
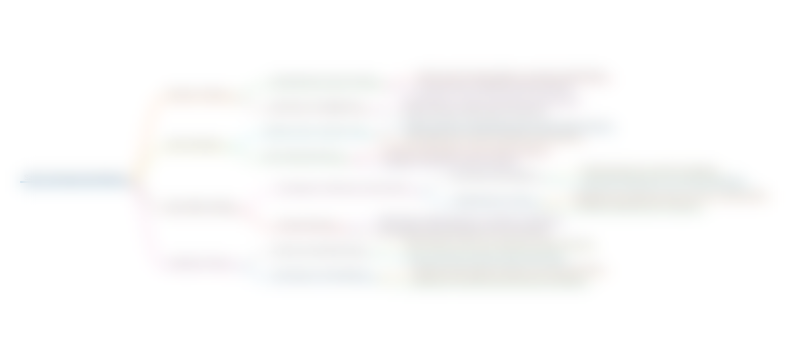
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
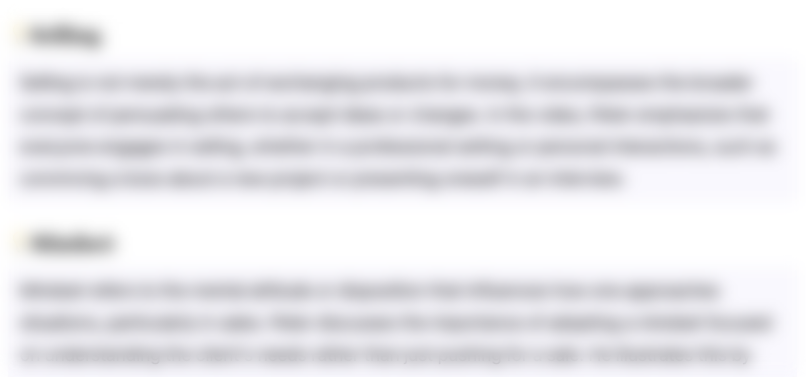
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
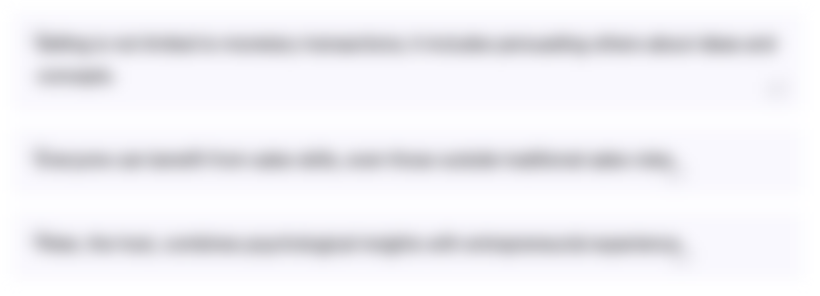
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
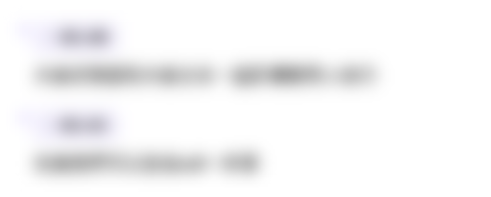
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
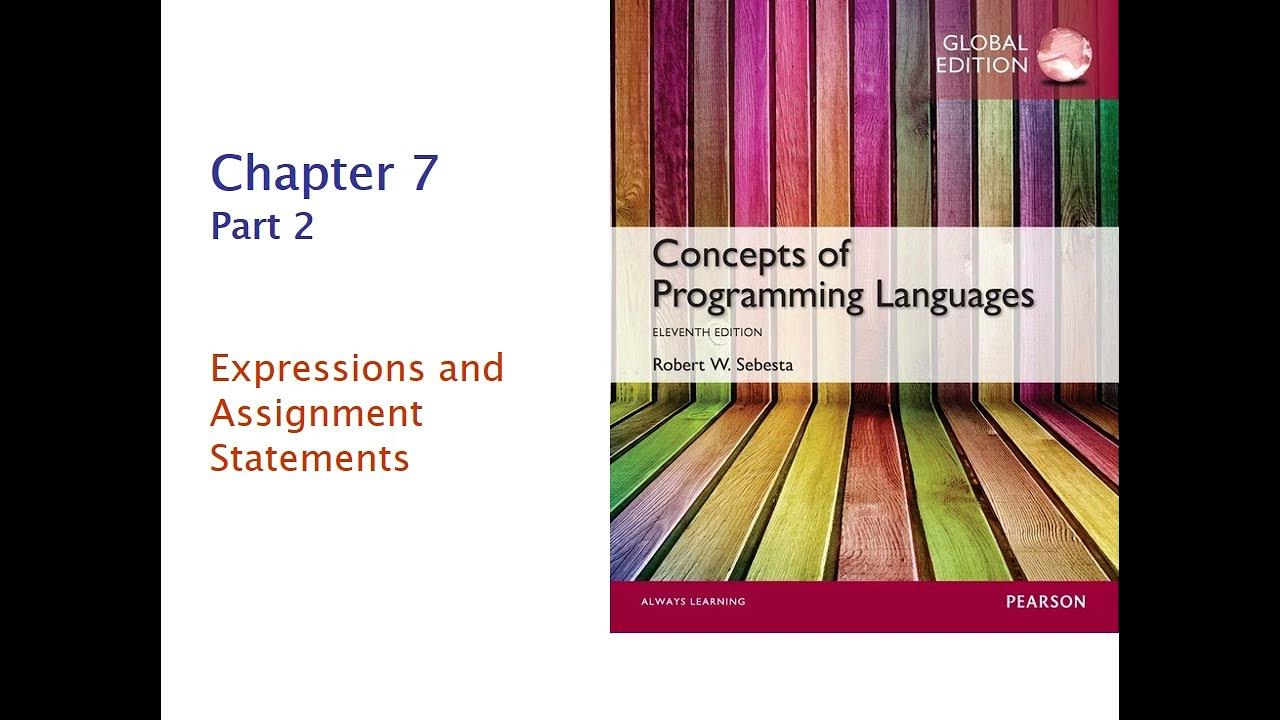
COS 333: Chapter 7, Part 2

std::move and the Move Assignment Operator in C++
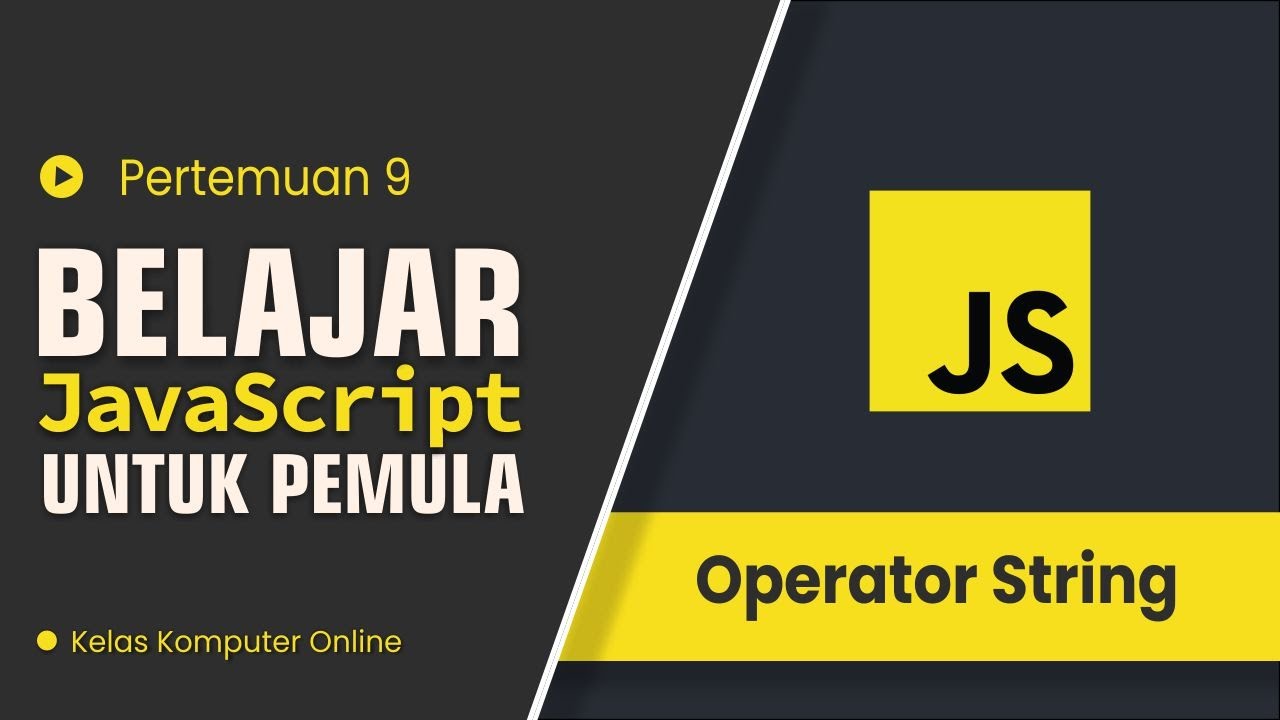
Learn JavaScript Programming Basics: String Operators in JavaScript
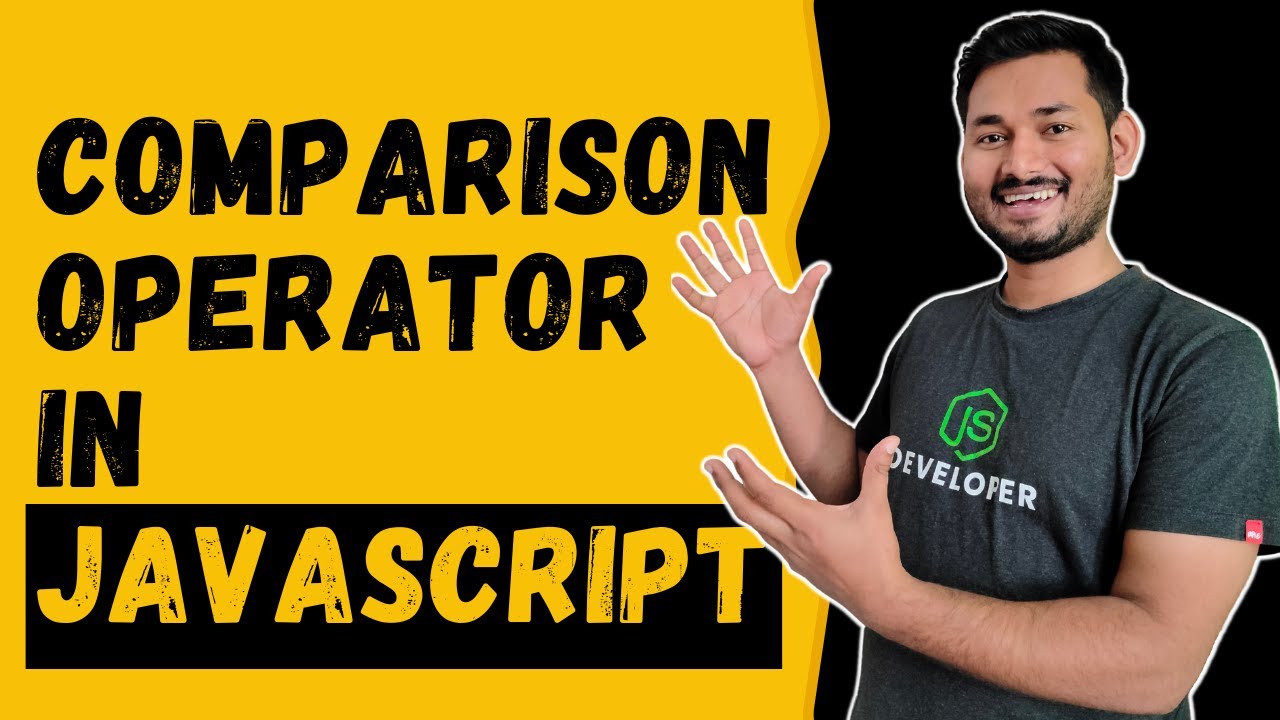
Comparison Operators in JavaScript Explained in Hindi | The Complete JavaScript Course | Ep.10
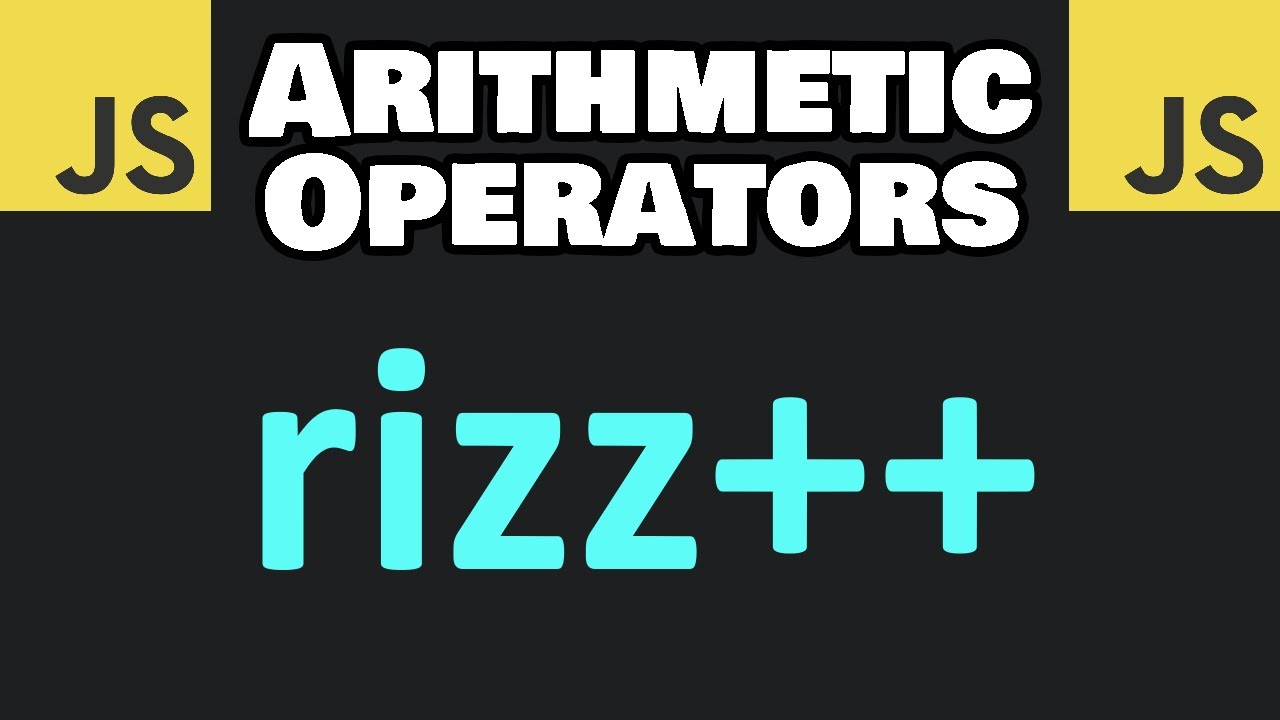
JavaScript ARITHMETIC OPERATORS in 8 minutes! โ
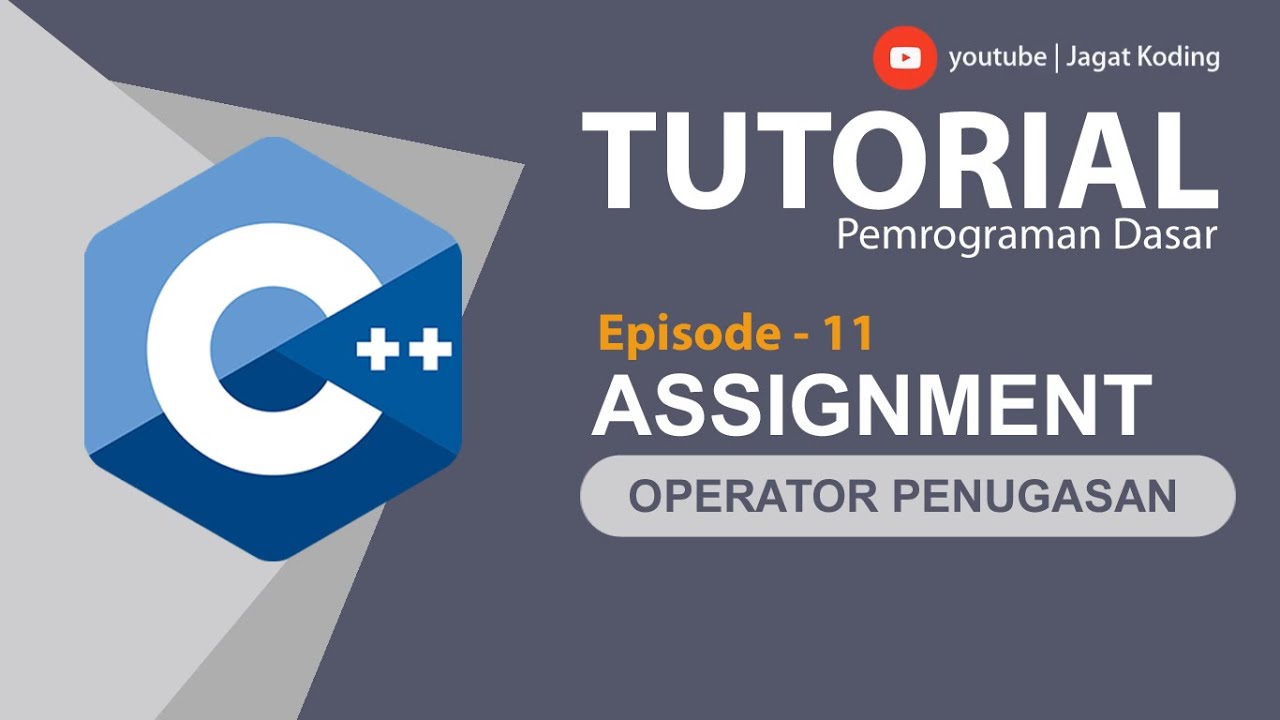
C++ 11 | Operator Assignment | Tutorial Pemrograman C++
5.0 / 5 (0 votes)