#63 Python Tutorial for Beginners | Exception Handling
Summary
TLDRIn this video, Debbie explains the three main types of errors in Python: compile-time errors, logical errors, and runtime errors. She demonstrates how to handle these errors using try, except, and finally blocks, emphasizing the importance of ensuring smooth software operation even when errors occur. Through examples, she shows how to manage errors like division by zero and invalid inputs, making sure the program doesn't halt unexpectedly. She also highlights the importance of closing resources like files or database connections, and offers practical tips for developers to handle exceptions effectively, ensuring robust and reliable code.
Takeaways
- ๐ There are three types of errors in Python: compile-time errors, logical errors, and runtime errors.
- ๐ Compile-time errors occur when there is a syntax issue in the code, like missing punctuation or misspelled keywords.
- ๐ Logical errors happen when the code runs but produces incorrect output, like incorrect calculations or results.
- ๐ Runtime errors occur during the program's execution, often due to unexpected user inputs or missing resources.
- ๐ Compile-time errors are easy to find since they are detected when the code is compiled.
- ๐ Logical errors can be harder to detect because they don't stop the code from running, but the output is wrong.
- ๐ Runtime errors are difficult to predict and usually happen when the user provides bad input or external resources are missing.
- ๐ To prevent runtime errors, developers need to anticipate potential user mistakes or resource failures and handle them appropriately.
- ๐ Exception handling in Python is done using try, except, and finally blocks to prevent program crashes during errors.
- ๐ The `try` block contains potentially risky code, while the `except` block handles specific errors, and the `finally` block ensures clean-up actions (like closing files or database connections).
- ๐ You can be specific with exception handling, targeting different types of errors (e.g., ZeroDivisionError, ValueError), or use a general 'except' to catch all exceptions.
- ๐ Using `finally` ensures that certain code, like closing resources, is executed regardless of whether an error occurred.
Q & A
What are the three types of errors in Python discussed in the video?
-The three types of errors in Python discussed are compile-time errors, logical errors, and runtime errors.
What is a compile-time error?
-A compile-time error occurs when there is a syntax issue in the code, such as missing a colon or using incorrect spelling in function names. These errors are detected at the time of compiling the code.
Can you explain logical errors in Python?
-Logical errors occur when the syntax of the code is correct, but the output produced is incorrect. For example, if a user expects a result of '5' but the code returns '4' instead, it is a logical error.
What is a runtime error and how does it differ from compile-time or logical errors?
-A runtime error occurs during the execution of the program, when the code runs fine but fails due to specific conditions such as invalid user input (e.g., dividing by zero). Unlike compile-time errors, these are not detected during code compilation, and unlike logical errors, the code runs until the issue arises at runtime.
Why is it important to handle runtime errors?
-Handling runtime errors is crucial because they can cause the program to crash, affecting user experience and potentially causing significant issues, like financial loss in banking software or loss of data in critical applications.
What is the purpose of a 'try' block in error handling?
-A 'try' block is used to attempt executing a piece of code that might cause an error. If an error occurs, it is caught by the 'except' block, allowing the program to handle the exception gracefully instead of crashing.
How do 'except' and 'finally' blocks work in Python?
-The 'except' block handles specific errors that occur during execution, allowing custom error messages or actions. The 'finally' block, on the other hand, executes code regardless of whether an error occurs or not, ensuring that important tasks, like closing resources, are completed.
What happens if an exception is raised inside a 'try' block but not handled?
-If an exception is raised inside the 'try' block and is not handled by an 'except' block, the program will terminate with an error message. To prevent this, it's important to catch and handle potential exceptions.
How can Python handle multiple types of exceptions?
-Python allows handling different types of exceptions by using multiple 'except' blocks, each specifying a particular type of error. For errors not specifically caught, a generic 'except' block can be used to catch all exceptions.
What is the advantage of using the 'finally' block in resource management?
-The 'finally' block ensures that resources, like files or database connections, are properly closed, regardless of whether an exception occurs. This helps prevent resource leaks and ensures the program runs smoothly even in error scenarios.
Outlines
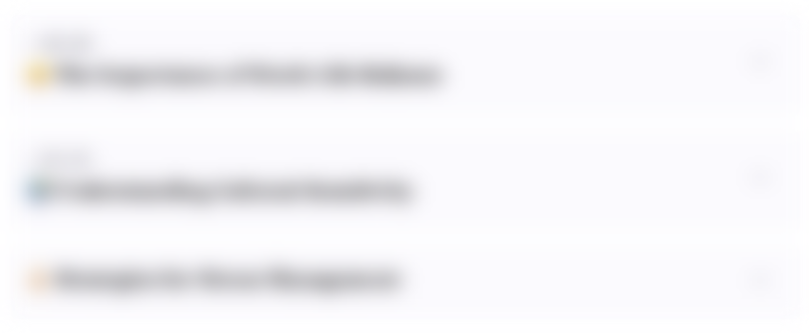
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
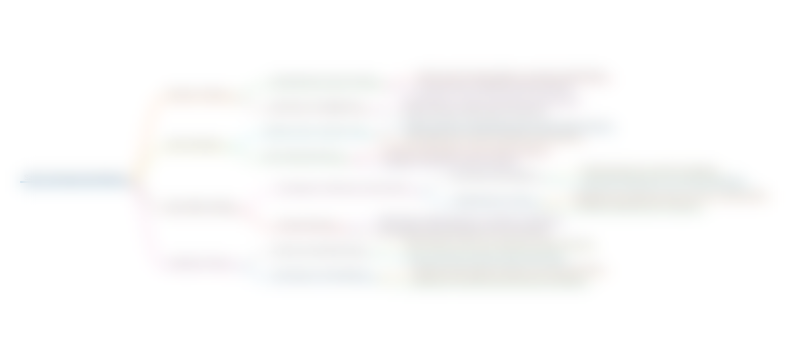
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
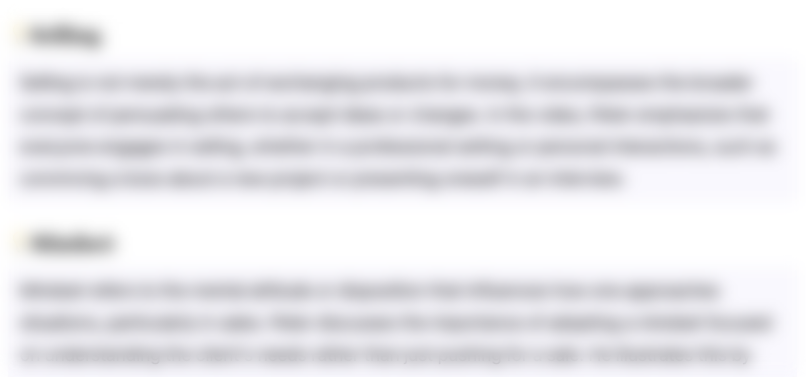
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
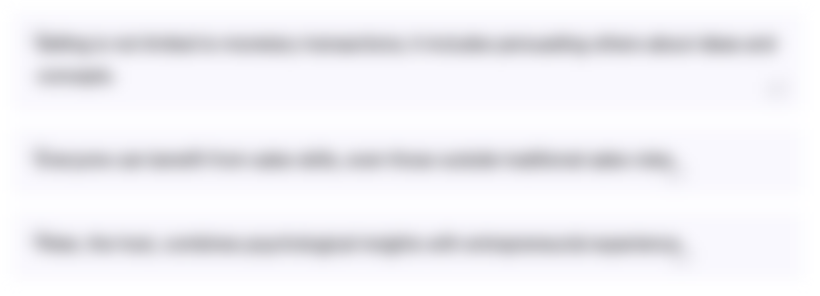
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
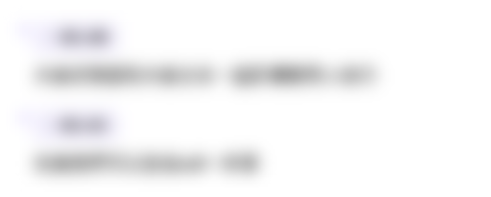
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)