[SER222] M03_02 Implementation (6/10): The DeleteMin Operation
Summary
TLDRThis video tutorial explains the `deleteMin` operation in binary search trees (BSTs). It walks through the process of finding and deleting the minimum node, handling two cases: when the node is a leaf or when it has a right child. The operation involves recursively finding the minimum node, then replacing it with its right child if necessary. The tutorial also covers how recursion works, with a focus on updating the tree structure and the tree size variable `N` during the process. It's a straightforward operation but essential to understanding BST manipulations.
Takeaways
- π The `deleteMin` operation removes the minimum node in a binary search tree (BST), which requires careful handling depending on the structure of the tree.
- π To find the minimum node, start at the root and move left until reaching a node without a left child.
- π If the minimum node has no right child, it is simply removed from the tree.
- π When the minimum node has a right child, the right child replaces the minimum node, maintaining the BST properties.
- π The deletion process involves two main cases: when the node has no children and when it has a right child.
- π The recursion in the deleteMin operation starts from the root, moving down the tree and eventually updating the parent nodeβs values after deletion.
- π The `deleteMin` function is closely related to the `findMin` function, with the key difference being the deletion of the node instead of just finding it.
- π If the left child of a node is `null`, it indicates that the node is the minimum, and the right child (if any) replaces it.
- π As the recursion unwinds, the N value of the nodes visited during the deletion process is updated to reflect the treeβs new structure.
- π In recursive tree manipulations, proper handling of edge cases (like having a right child or no children) ensures the tree remains balanced and the properties of the BST are preserved.
Q & A
What is the main purpose of the deleteMin operation in a binary search tree?
-The main purpose of the deleteMin operation is to remove the minimum element from the binary search tree (BST), which is typically the leftmost node in the tree.
How do you find the minimum node in a binary search tree?
-To find the minimum node, start at the root and continuously move left until you reach a node that has no left child. This node will be the minimum.
What happens when you delete the minimum node in a tree with no children?
-If the minimum node has no children, it can simply be removed from the tree without any further adjustments.
How does the deleteMin operation handle the case where the minimum node has a right child?
-If the minimum node has a right child, the right child is moved up to replace the minimum node, and the original minimum node is removed.
What is the role of recursion in the deleteMin operation?
-Recursion is used to traverse down the tree to find the minimum node. Once the minimum node is found, recursion unwinds to update the tree and adjust the sizes of the nodes.
In the deleteMin method, what happens if the left child of the node is null?
-If the left child of the node is null, the node is considered the minimum, and its right child (if it exists) replaces it in the tree.
What does the 'N' variable represent in the code, and why is it updated during recursion?
-'N' represents the size of the tree or the number of nodes. It is updated during recursion to reflect the new size of the tree after a node is deleted.
What is the significance of updating the N variable as the recursion unwinds?
-Updating the N variable as recursion unwinds ensures that the size of the tree is accurately reflected at each level after the deletion operation.
How does the deleteMin operation differ from the findMin operation?
-The deleteMin operation not only identifies the minimum node (like the findMin operation) but also removes it from the tree, handling edge cases like when the node has a right child.
What happens if the right child of the minimum node is null during the deleteMin operation?
-If the right child of the minimum node is null, the node is simply removed, and no further adjustments are necessary.
Outlines
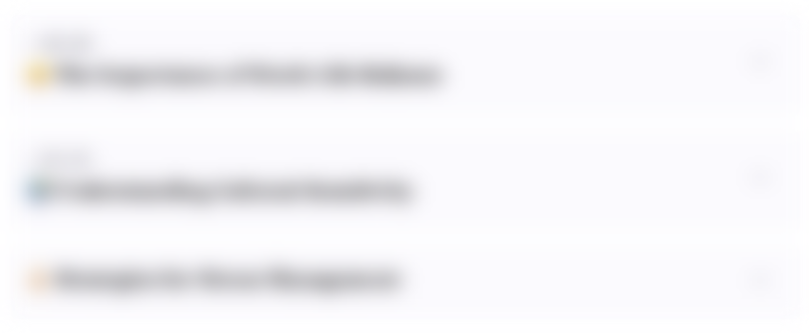
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
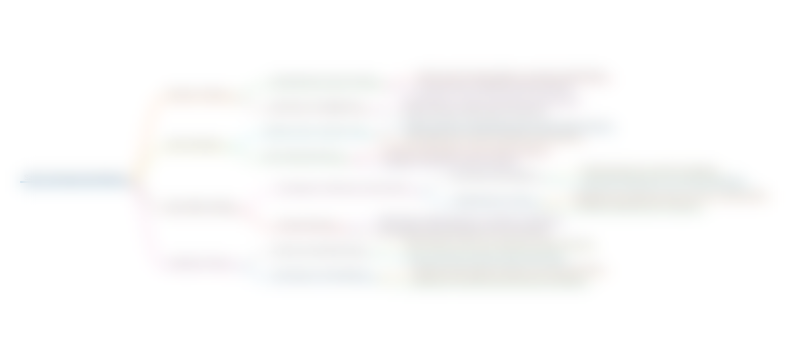
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
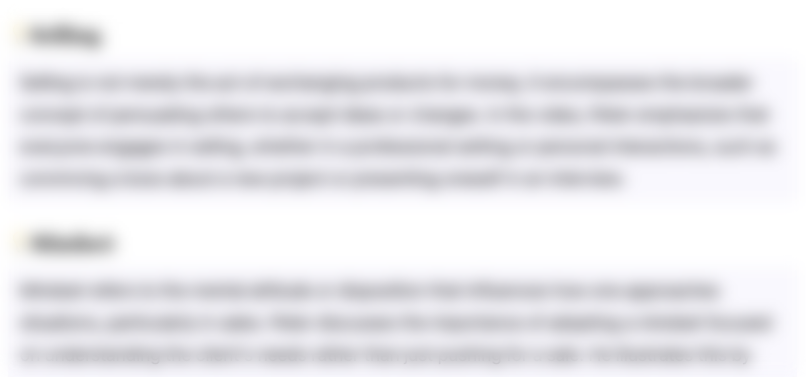
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
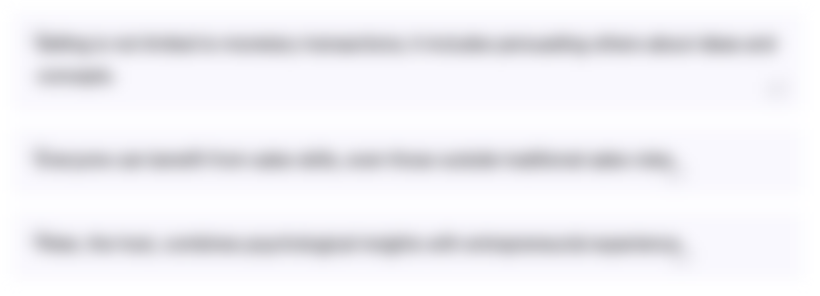
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
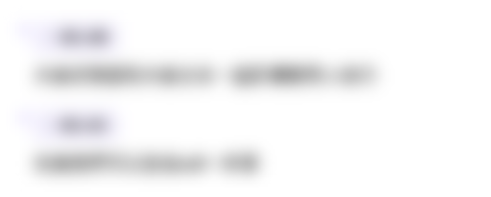
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

[SER222] M03_02 Introduction (1/2): The Concept
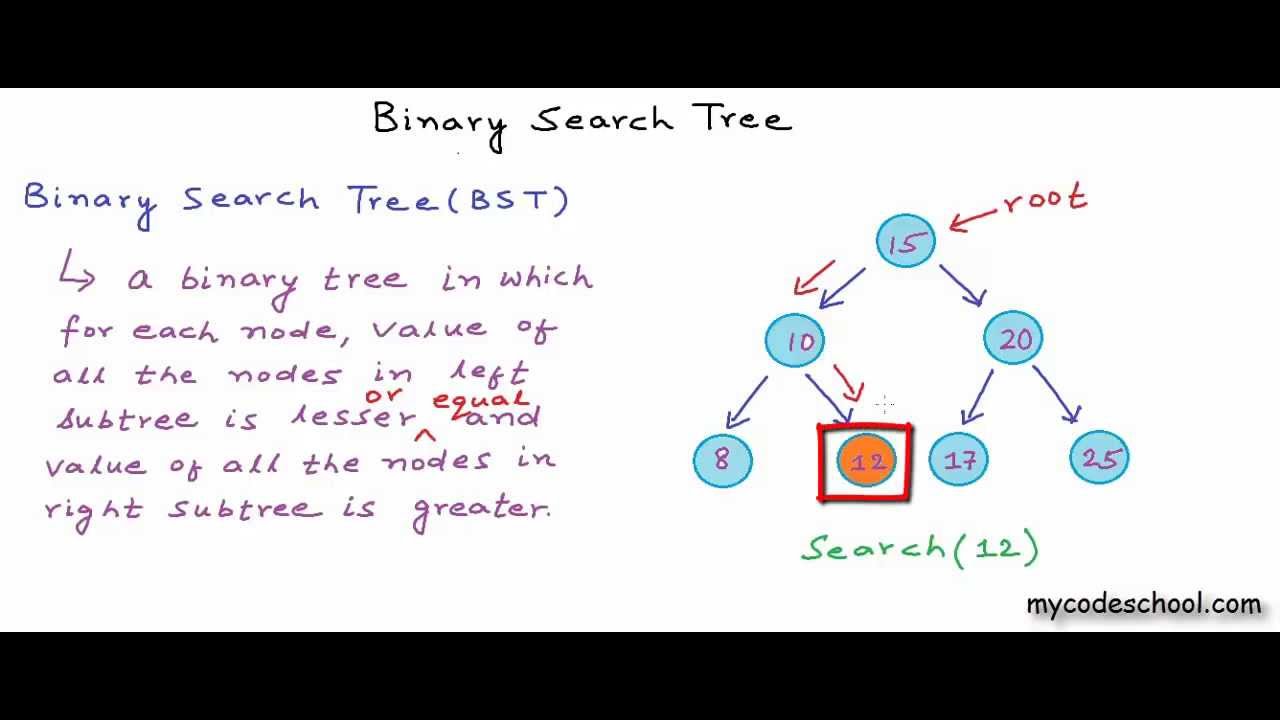
Data structures: Binary Search Tree
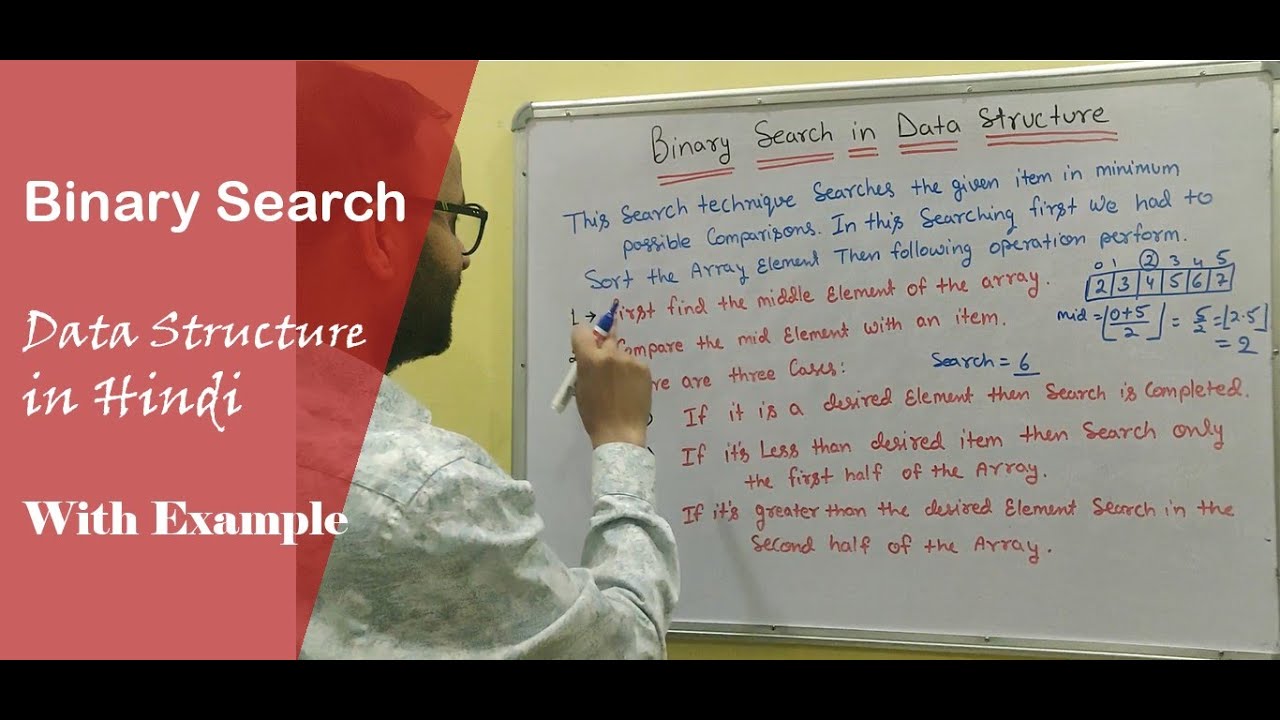
Binary Search in Data structure Hindi | with Algorithm Example | CS gyan

L-3.7: Introduction to Trees (Binary Tree, Almost Complete Binary Tree, Full BT, Complete BT, BST)
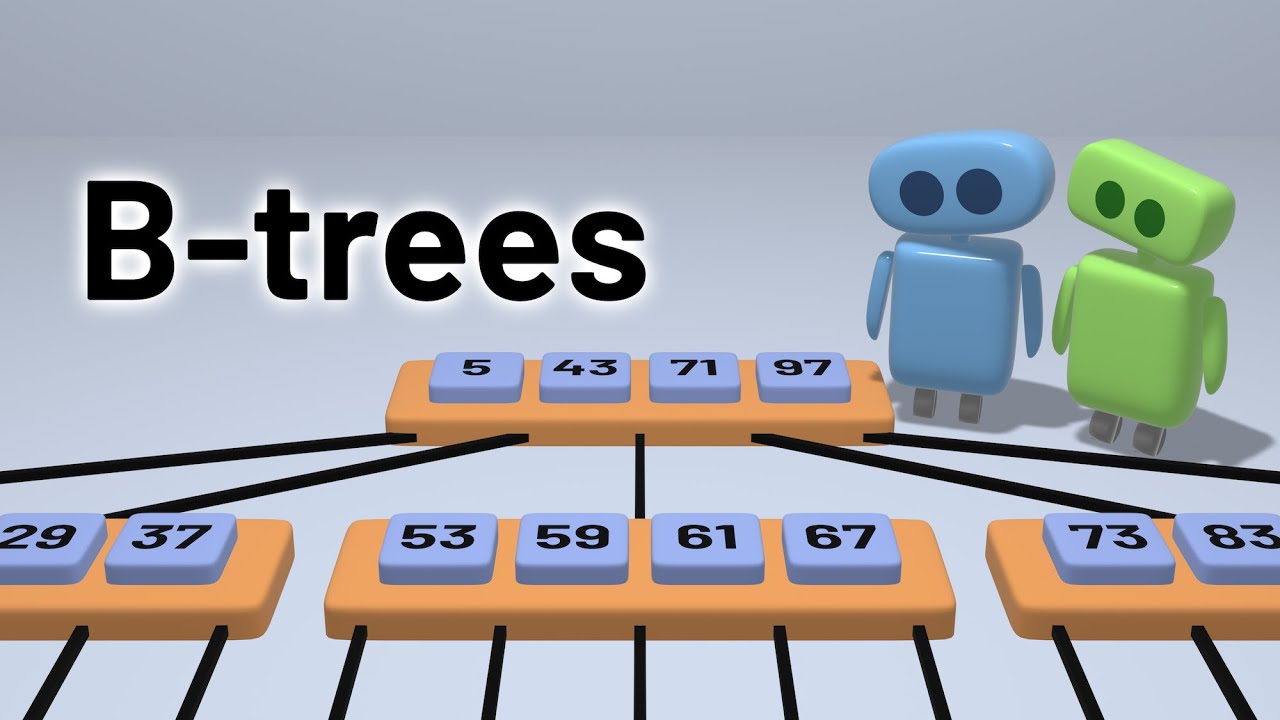
Understanding B-Trees: The Data Structure Behind Modern Databases
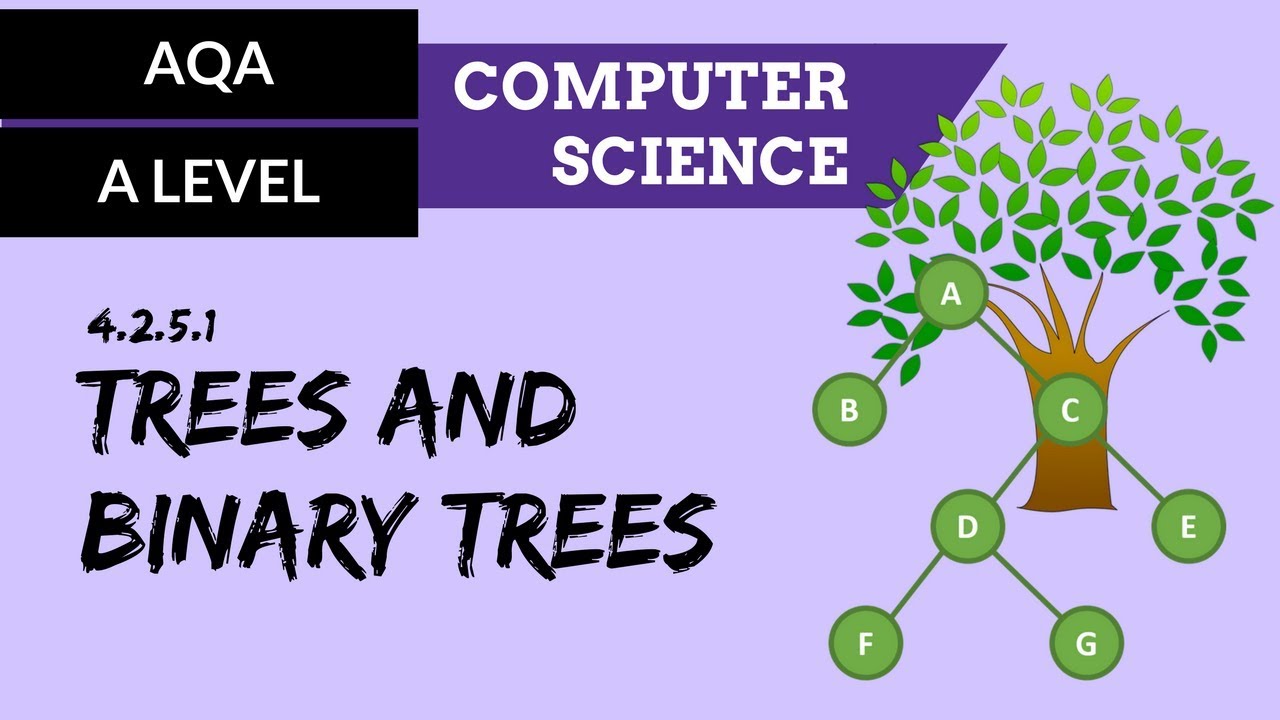
AQA AβLevel Trees & Binary trees
5.0 / 5 (0 votes)