AQA A’Level Trees & Binary trees
Summary
TLDRThis video explores trees and binary trees, essential data structures for managing hierarchical data. It explains tree terminology like root, children, branches, and leaf nodes. The binary search tree is highlighted for its efficiency in sorting, searching, and retrieving data. The video demonstrates constructing a binary tree with alphabetical comparison, resulting in a structured hierarchy. It also shows how binary trees can be represented in arrays with left, value, and right pointers, allowing easy reconstruction of the tree.
Takeaways
- 🌳 **Trees and Binary Trees**: Trees are a popular data structure for representing hierarchical data, and binary trees are a specific type of tree where each node has at most two children.
- 🔍 **Binary Search Trees**: Binary search trees are dynamic data structures that allow for efficient sorting, searching, and retrieval of data.
- 📚 **Hierarchical Nature**: Many real-world data sets are hierarchical, such as organizational charts, which can be effectively represented by trees.
- 🌟 **Root Node**: The topmost node in a tree is known as the root node, which serves as the starting point for the tree structure.
- 👨👩👧👦 **Children Nodes**: Nodes directly below the root or any other node are called children nodes.
- 🔗 **Branches**: The lines connecting nodes in a tree are referred to as branches.
- 🍃 **Leaf Nodes**: Nodes at the bottom of the tree with no children are called leaf nodes or terminal nodes.
- 📝 **Constructing a Binary Tree**: A binary tree is constructed by inserting elements in a sorted manner, comparing each new element to the current node and placing it to the left or right accordingly.
- 📊 **Array Representation**: Binary trees can be represented in arrays, where each element has three parts: a left pointer, the value, and a right pointer.
- 🔄 **Dynamic Nature**: Binary trees can grow and shrink as needed, adapting to the data as it changes during program execution.
- 🔎 **Traversal and Search**: Searching in a binary tree is efficient because each comparison allows the search to eliminate half of the remaining nodes.
Q & A
What is a tree data structure?
-A tree data structure is a hierarchical data structure that organizes data in a way that each item, called a node, is connected to other items by at most one parent and multiple children.
What is the root node in a tree structure?
-The root node is the topmost node in a tree structure, from which all other nodes descend.
What do you call the nodes that are children in a tree structure?
-In a tree structure, the nodes that are directly connected to another node are called children of that node.
What are the branches in a tree structure?
-The branches in a tree structure refer to the lines or paths that connect nodes to each other.
What is a leaf node or terminal node in a tree?
-A leaf node or terminal node is a node in a tree that does not have any children, meaning it is at the bottom of the tree and has no further sub-trees.
What is a binary tree?
-A binary tree is a specific type of tree where each node has at most two children, typically referred to as the left child and the right child.
What is the significance of a binary search tree?
-A binary search tree is significant because it allows for efficient sorting, searching, and retrieval of data. It maintains a specific order, typically alphabetical or numerical, which enables faster operations compared to other data structures.
How does a binary search tree reflect structural relationships?
-A binary search tree reflects structural relationships by maintaining a hierarchy where each node's children are ordered in a way that the left child is less than the parent and the right child is greater, facilitating quick lookups.
How is a binary tree constructed from a set of data?
-A binary tree is constructed by starting with a root node and then adding subsequent nodes by comparing each new item with the current node and placing it to the left if it is smaller or to the right if it is larger, recursively.
What does it mean for a binary tree to be dynamic?
-A binary tree being dynamic means that it can grow and shrink as needed during the execution of a program, allowing for the addition and removal of nodes as required.
How can a binary tree be represented in an array?
-A binary tree can be represented in an array where each index corresponds to a node, and the left and right child pointers are represented by the indices of the left and right children in the array, respectively.
Outlines
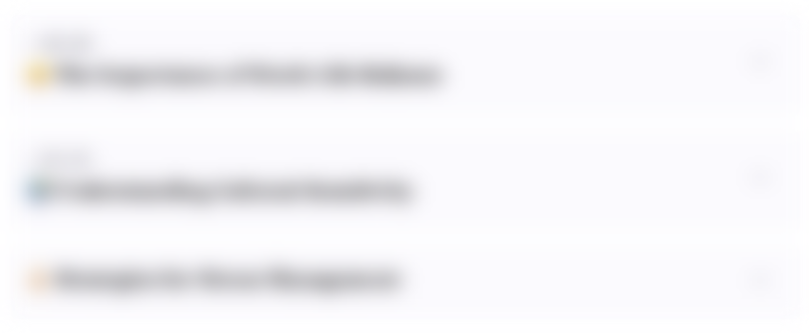
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
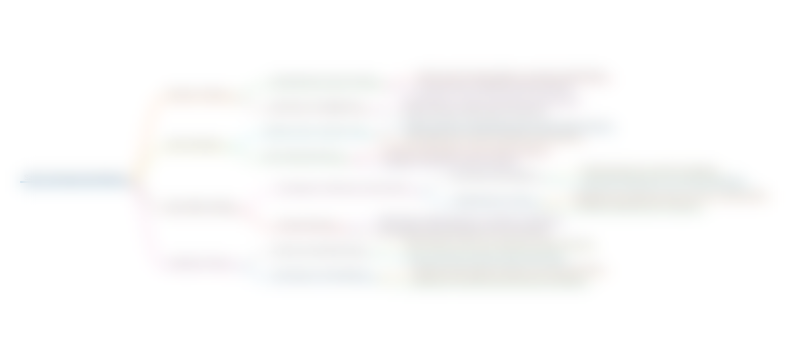
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
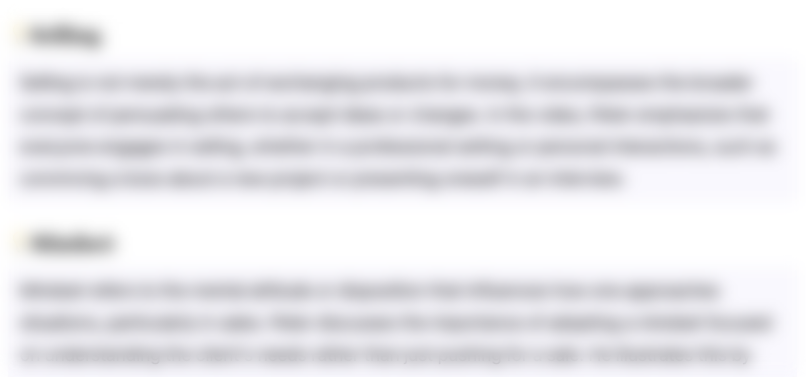
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
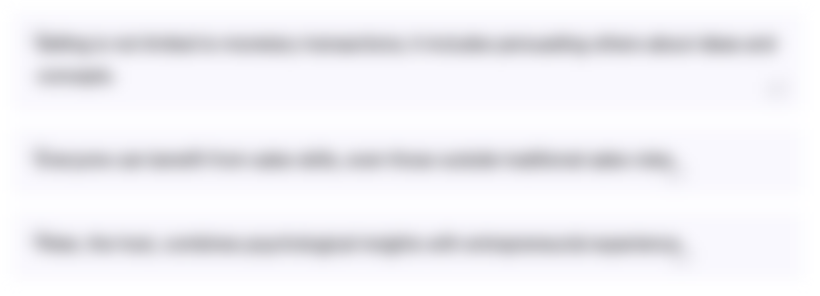
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
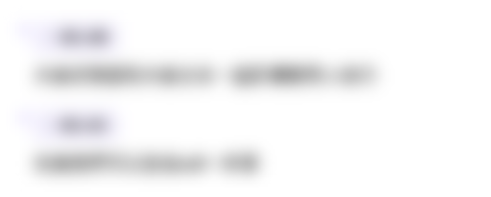
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
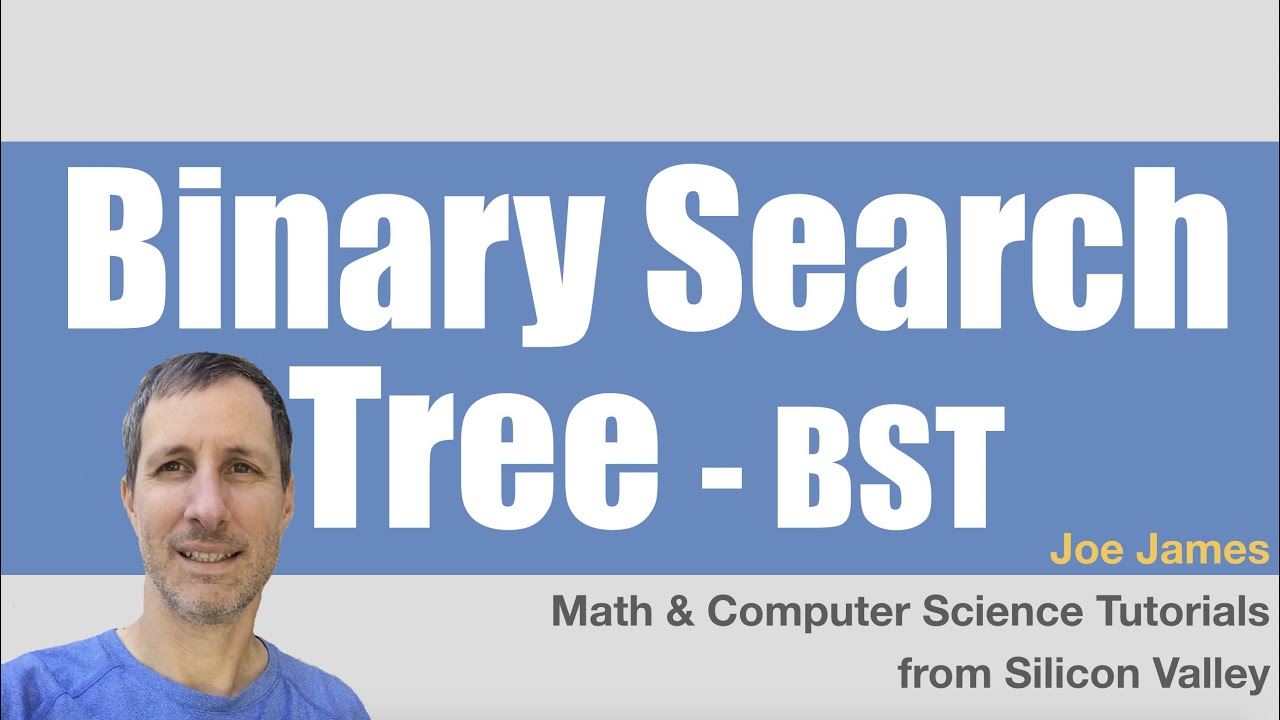
Binary Search Trees (BST) Explained in Animated Demo

L-3.7: Introduction to Trees (Binary Tree, Almost Complete Binary Tree, Full BT, Complete BT, BST)
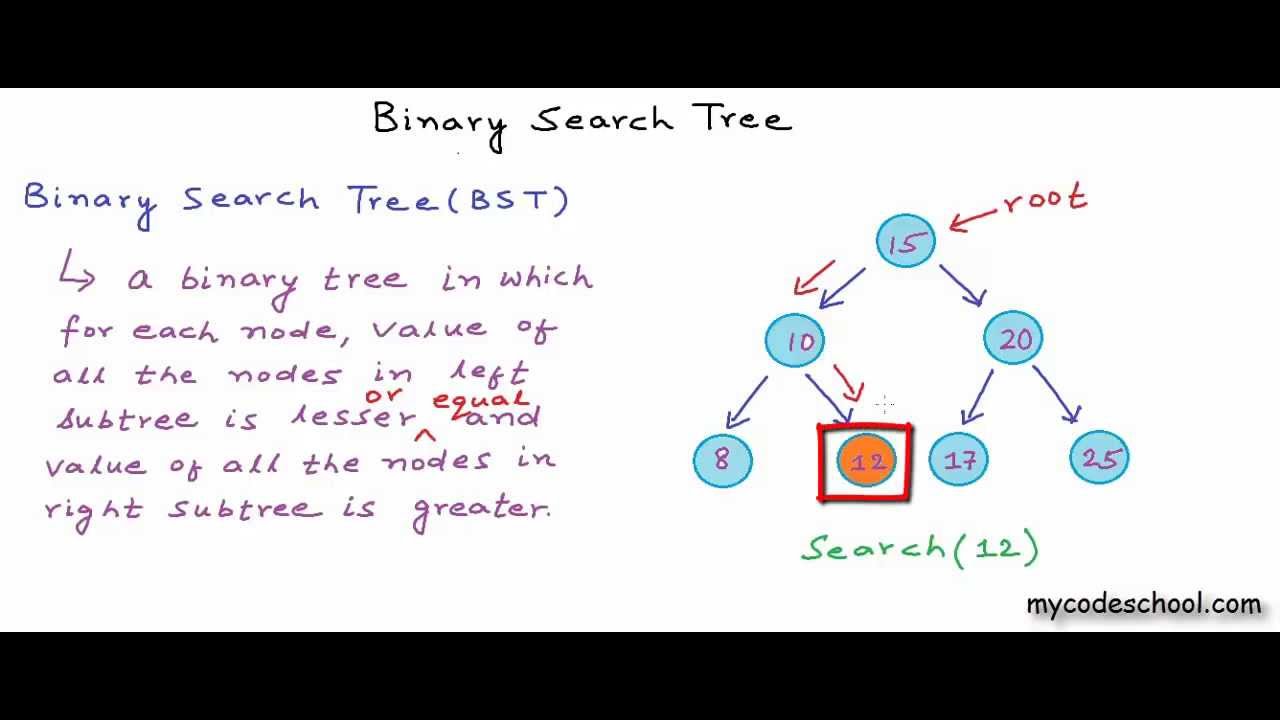
Data structures: Binary Search Tree

LeetCode Problem: 226. Invert Binary Tree | Java Solution
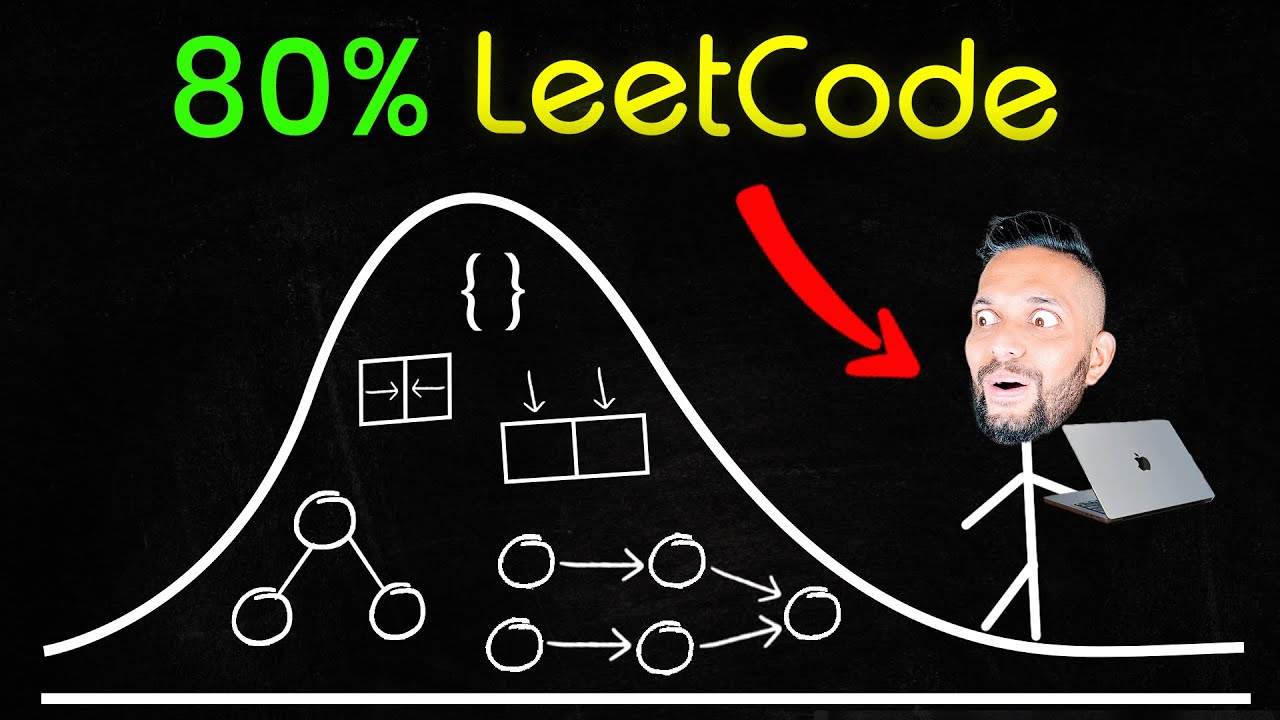
8 patterns to solve 80% Leetcode problems
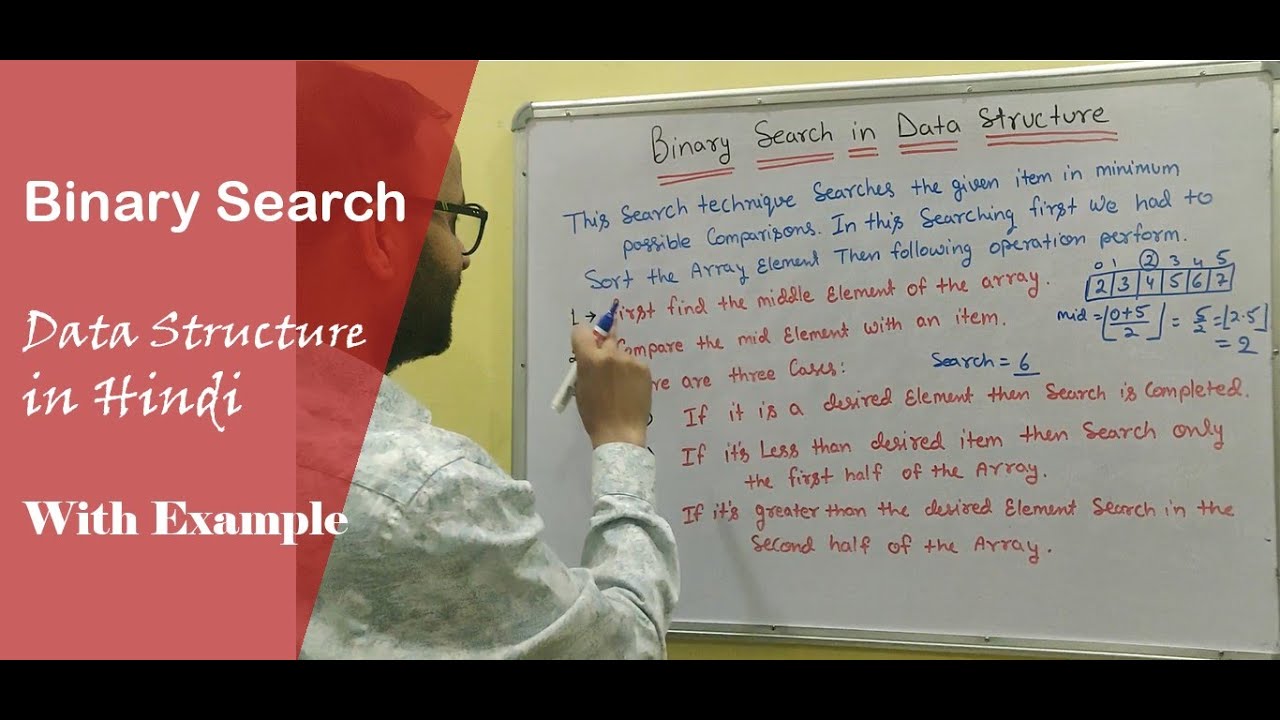
Binary Search in Data structure Hindi | with Algorithm Example | CS gyan
5.0 / 5 (0 votes)