Selenium Browser Automation in Python
Summary
TLDRIn this tutorial, you'll learn how to automate web scraping and browser interactions using Selenium in Python. The video demonstrates how to open a website, navigate through it, click on links, and extract data interactively—ideal for dynamic websites where content appears only after user actions. The example uses the 'neural9.com' site and Amazon to showcase scraping the price of a book. The tutorial covers setting up the necessary tools, using XPath for element selection, handling browser tabs, and interacting with dynamic content, making it a valuable resource for automating web scraping tasks.
Takeaways
- 😀 Selenium allows for interactive web scraping by automating browser actions such as clicking, scrolling, and navigating between tabs.
- 😀 Unlike traditional web scraping with requests, Selenium can interact with dynamic websites that require user actions like scrolling or hovering to load content.
- 😀 The tutorial demonstrates how to install Selenium and the WebDriver Manager using pip, which makes setting up ChromeDriver easier and more reliable.
- 😀 You can use the `driver.get()` function to open a webpage, and `driver.maximize_window()` to automatically maximize the browser window.
- 😀 XPath is a powerful tool in Selenium for locating HTML elements by their attributes, such as anchor tags (`<a>`) or specific class names.
- 😀 To interact with elements like links, you can use `find_elements` with XPath queries to filter elements based on specific criteria, such as text or class.
- 😀 Selenium enables you to automate clicking on elements by finding the correct link using conditional checks, such as searching for specific text within a webpage.
- 😀 When working with multiple browser tabs, `driver.switch_to.window()` allows you to switch between them and continue interacting with the correct tab.
- 😀 After navigating between tabs, ensure that the page is fully loaded before interacting with elements by using time delays or synchronization techniques.
- 😀 The tutorial concludes with a simple example of extracting prices from Amazon using XPath to find specific tags and extracting the relevant data like product price and currency symbol.
- 😀 While this tutorial provides a basic introduction, Selenium can be used for more complex automation tasks, such as scraping dynamic content or automating browser games, with more advanced XPath and interactions.
Q & A
What is the main purpose of the tutorial in the video?
-The main purpose of the tutorial is to teach how to automate website interactions and web scraping using Selenium in Python. The tutorial demonstrates how to open a browser, click on elements, scroll, and extract dynamic content from websites.
What is the difference between traditional web scraping and the approach demonstrated in the video?
-Traditional web scraping involves sending HTTP requests to a website and parsing the raw HTML response. The approach demonstrated in the video uses Selenium to simulate real user interactions, such as clicking and scrolling, to interact with dynamic content that would not be accessible through simple HTTP requests.
What tools and libraries are required to follow the tutorial?
-The tutorial requires the installation of two Python libraries: Selenium (`pip install selenium`) for web automation and WebDriver Manager (`pip install webdriver-manager`) to manage the Chrome driver used by Selenium.
Why is it important to use WebDriver Manager when working with Selenium?
-WebDriver Manager is used to automatically manage the installation and path configuration of the appropriate ChromeDriver version. This avoids compatibility issues between the installed browser version and the WebDriver, ensuring smooth automation.
What does the `driver.maximize_window()` command do?
-The `driver.maximize_window()` command in Selenium automates the process of maximizing the browser window after the website is loaded. This ensures the browser is displayed in full-screen mode.
How is XPath used in the tutorial, and why is it important?
-XPath is used to locate specific HTML elements on a webpage, such as links and div containers. In this tutorial, XPath is crucial for finding elements based on their structure, attributes, or text content (e.g., finding book links with specific text). It provides a precise and flexible way to query the DOM.
What is the role of `driver.switch_to.window()` in the script?
-The `driver.switch_to.window()` method is used to switch between browser tabs. In this tutorial, it allows the script to switch to the new tab (Amazon page) after clicking on a book link, enabling the script to interact with the content of that new tab.
What challenges did the tutorial address when scraping a dynamic website like Amazon?
-The tutorial addressed the challenge of dealing with dynamically loaded content that appears only after interacting with the webpage (e.g., clicking links). It also discussed handling multiple tabs in the browser and switching between them to scrape data from a different webpage (Amazon).
How does the script handle the extraction of the book price from Amazon?
-The script locates the book's price on the Amazon page by using XPath to search for anchor tags (`<a>`) containing specific span tags with the price text. The XPath query filters for spans containing keywords like 'Paperback' and the currency symbol (e.g., Euro), extracting the relevant price information.
What is the significance of `options.add_experimental_option('detach', True)` in the script?
-The line `options.add_experimental_option('detach', True)` is used to keep the browser open after the script completes. By default, Selenium closes the browser once the task is finished, but this option allows the user to view the browser session even after the script has ended.
Outlines
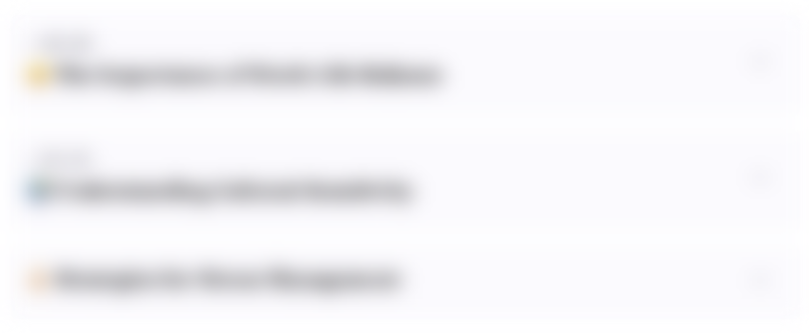
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
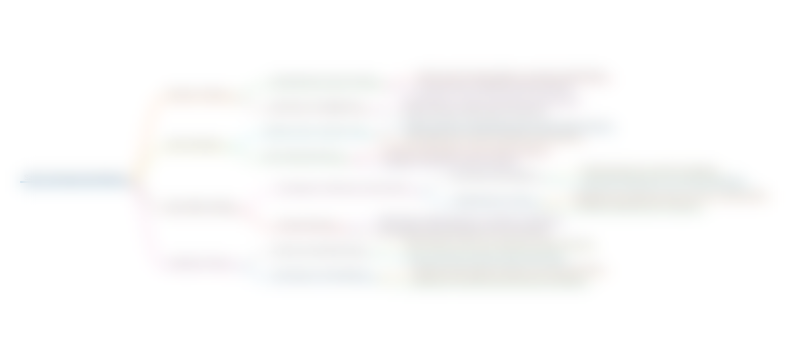
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
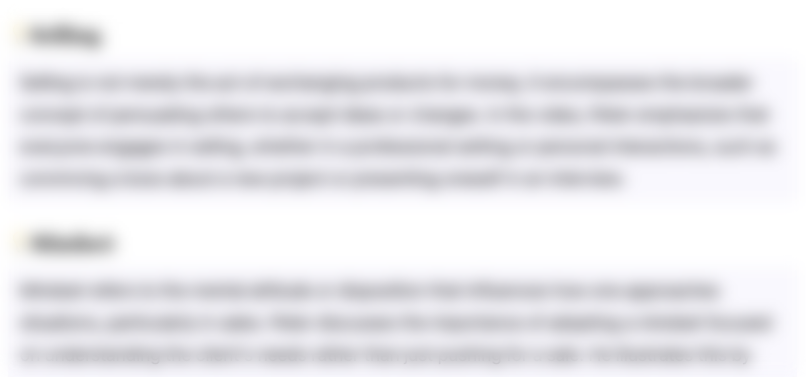
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
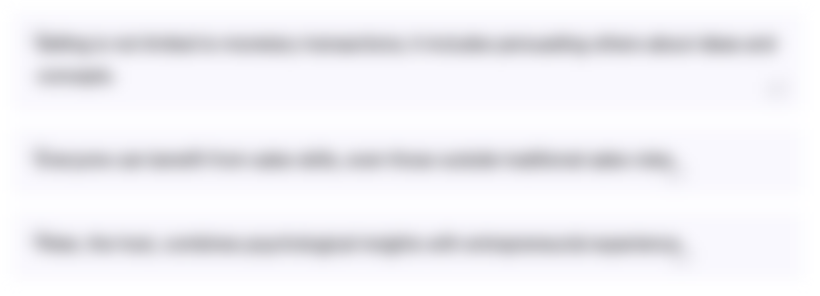
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
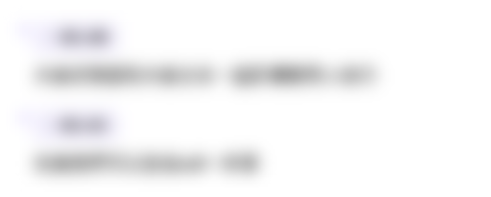
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
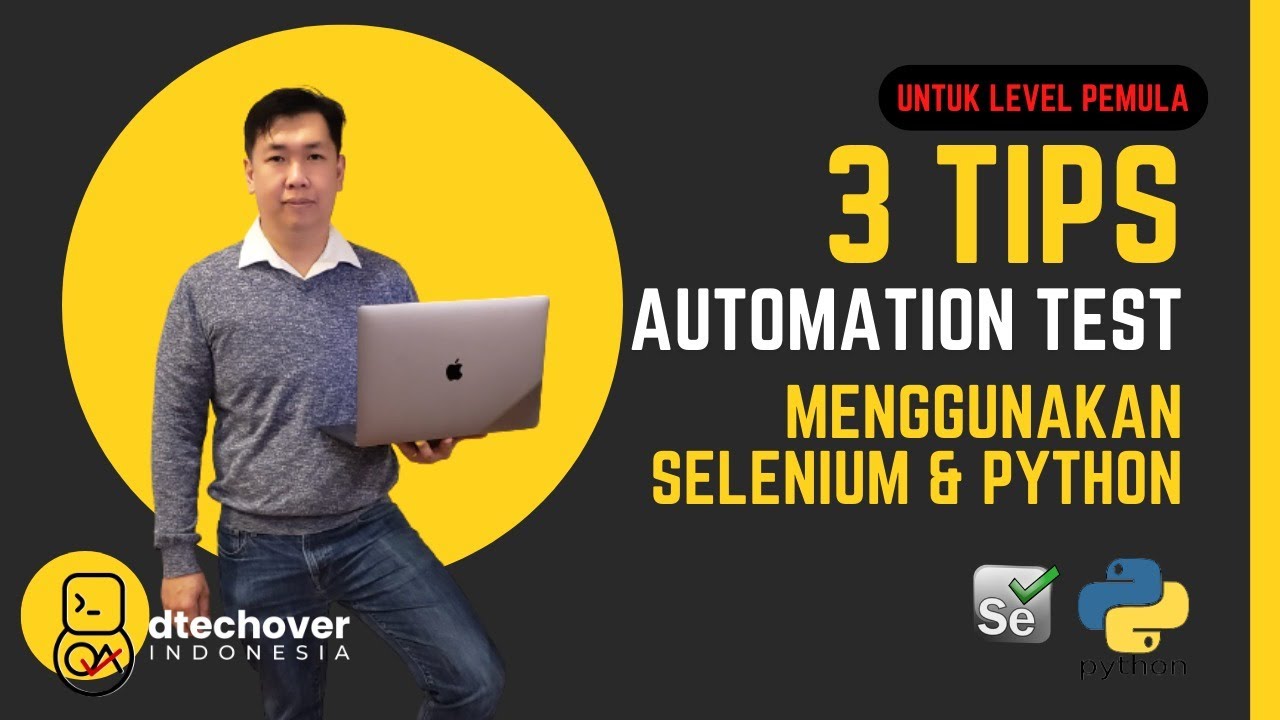
3 Tips Automation Test menggunakan Selenium dan Python
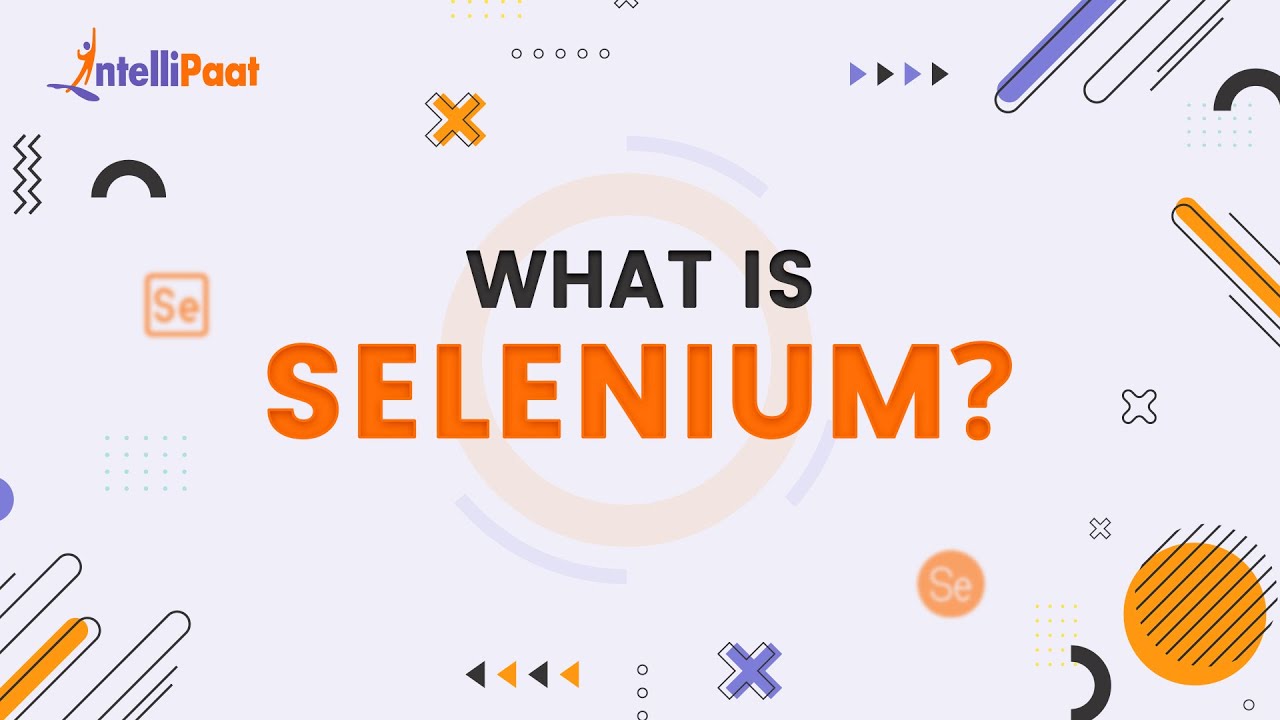
What is Selenium | Selenium Explained in 2-minutes | Introduction to Selenium | Intellipaat
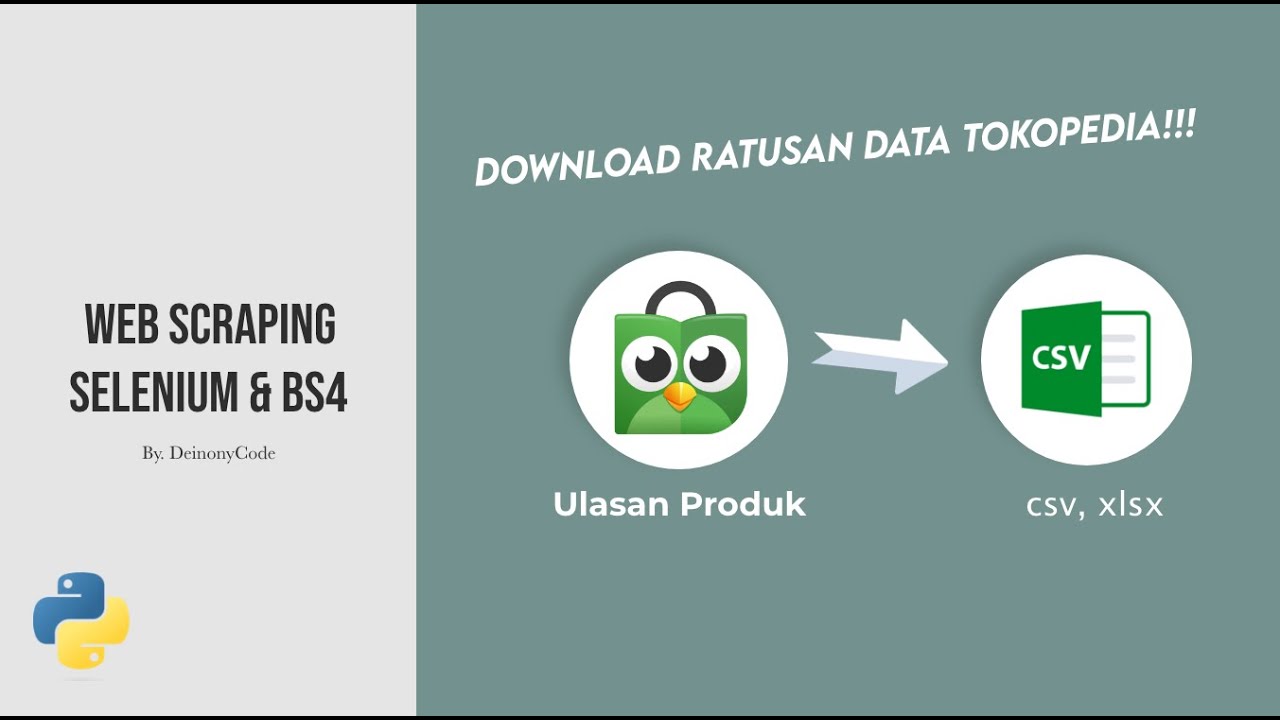
Scraping Data Ulasan Produk Tokopedia Menggunakan SELENIUM & BEAUTIFULSOUP
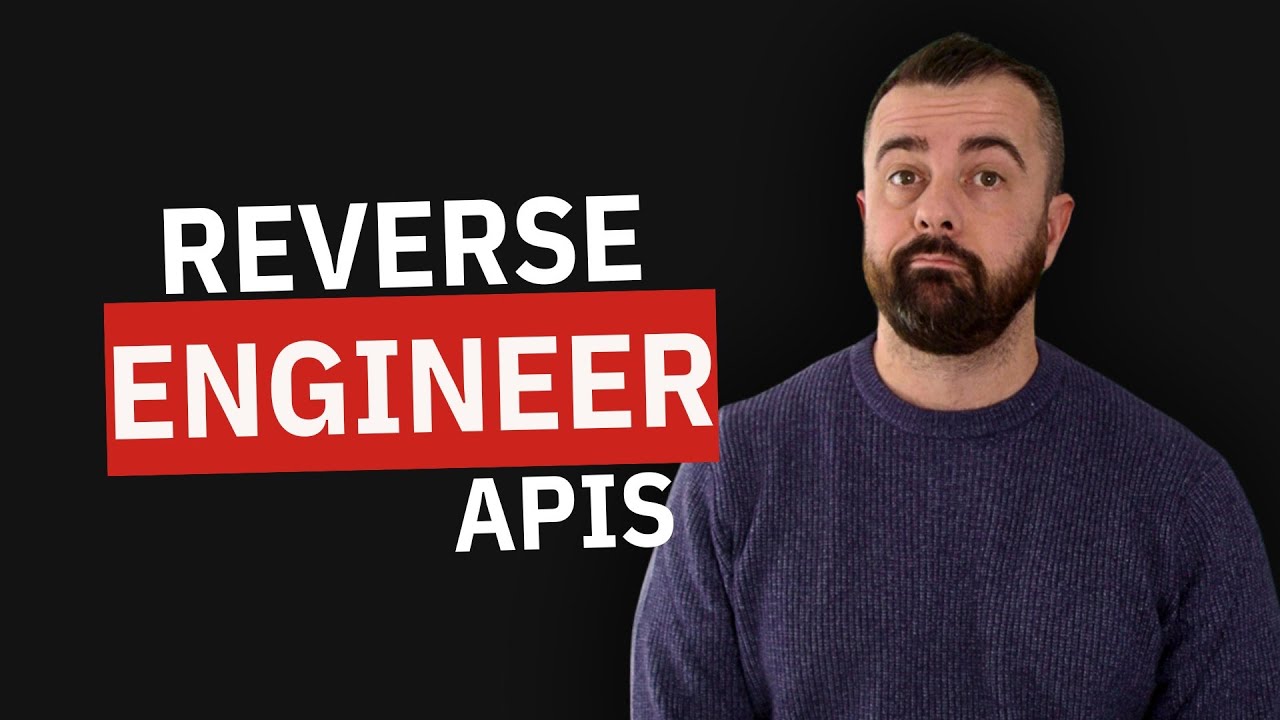
This is How I Scrape 99% of Sites
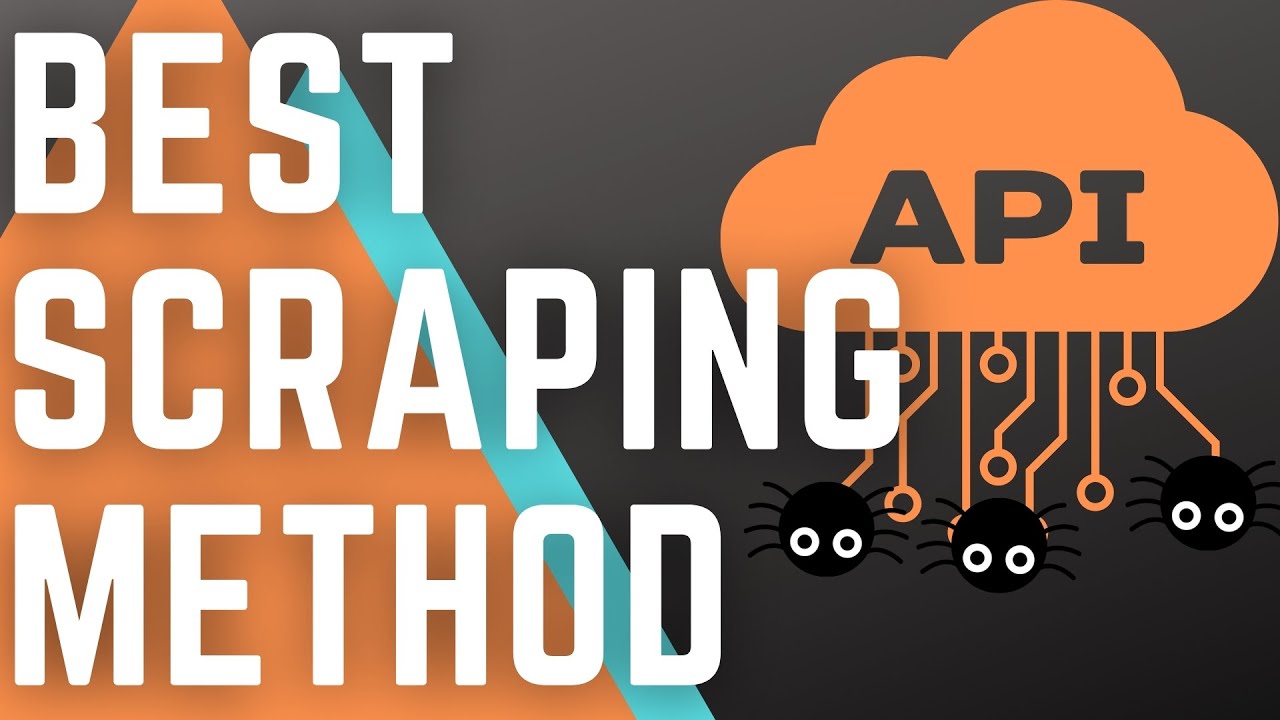
Always Check for the Hidden API when Web Scraping
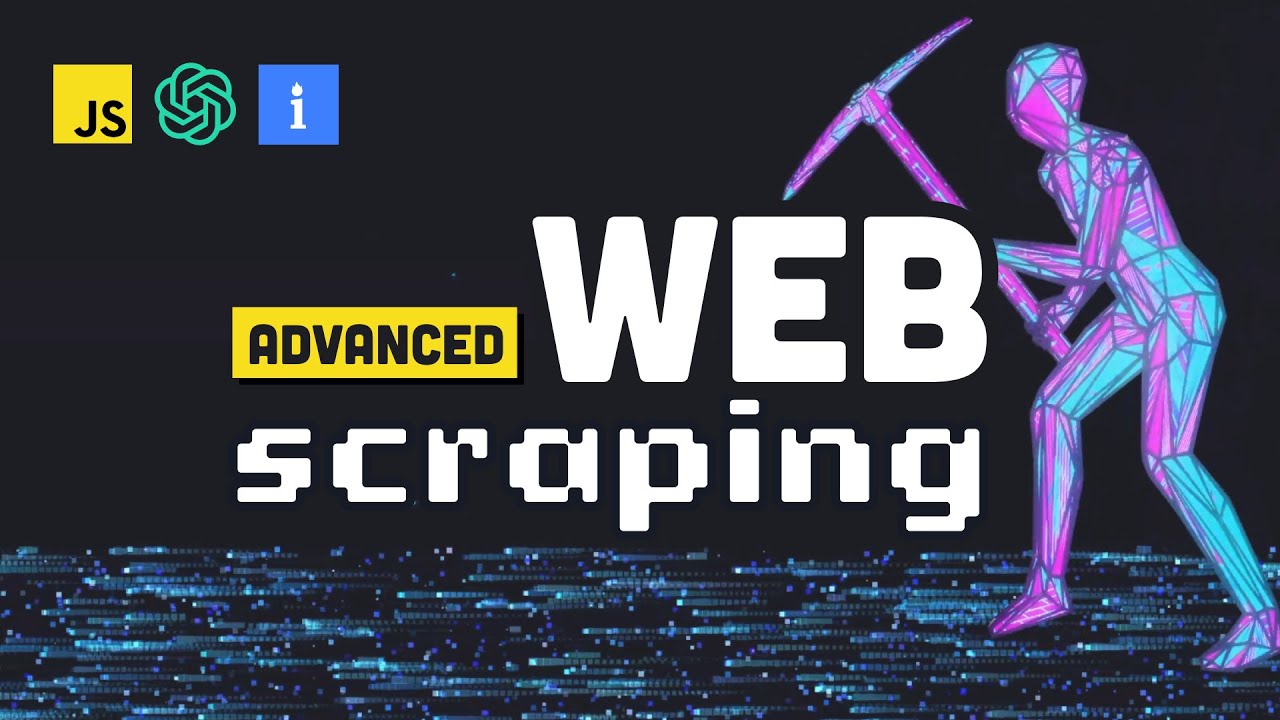
Industrial-scale Web Scraping with AI & Proxy Networks
5.0 / 5 (0 votes)