Aula 16: Modificadores, autoload e traits
Summary
TLDRThis PHP tutorial dives deep into advanced concepts such as simulating multiple inheritance using traits, working with static properties and methods, handling class inheritance, and resolving method conflicts. It also covers the use of the 'final' keyword to prevent class or method modifications, and introduces SPL Autoloading for automatic class loading. By illustrating practical examples, the video empowers PHP developers to efficiently manage complex code structures and optimize class functionality, making it an essential resource for mastering advanced PHP programming techniques.
Takeaways
- 😀 Traits in PHP simulate multiple inheritance by allowing classes to share functionality without being instantiated.
- 😀 The `final` keyword is used to prevent method overrides or class inheritance, ensuring consistency in the codebase.
- 😀 Static properties and methods are shared across all instances of a class, not specific to individual objects.
- 😀 Constants in PHP are defined using the `const` keyword and can be accessed using `ClassName::CONSTANT_NAME`.
- 😀 Autoloading in PHP is facilitated by `spl_autoload_register()`, dynamically loading classes without the need for `include` or `require` statements.
- 😀 The `use` keyword is used in PHP to include traits within a class, making their methods available without inheritance.
- 😀 When multiple traits define the same method, a conflict can arise, which can be resolved using `insteadof` to specify the preferred method.
- 😀 PHP does not allow direct instantiation of traits; they are only used in classes to share functionality.
- 😀 The `static` keyword makes properties and methods belong to the class itself, rather than to individual class instances.
- 😀 PHP classes and traits cannot be instantiated like objects; instead, they define behavior to be shared across instances or classes.
Q & A
What are Traits in PHP, and how do they help with multiple inheritance?
-In PHP, Traits are a mechanism that allows code to be reused in multiple classes. They simulate multiple inheritance by allowing methods to be shared between classes without needing to create complex inheritance hierarchies. Traits provide a solution for situations where you need the same functionality in multiple classes.
Can you instantiate a Trait in PHP?
-No, you cannot instantiate a Trait in PHP. Traits are not classes, but rather reusable sets of methods that can be included in classes using the 'use' keyword. They are designed to be incorporated into concrete classes, not instantiated on their own.
How do you resolve method name collisions when using multiple Traits in PHP?
-Method name collisions between Traits can be resolved using the 'insteadof' operator to specify which method to use. If you want to rename a method from a Trait, you can use the 'as' keyword to assign a new name to the method and avoid collisions.
What is the purpose of the 'use' keyword when working with Traits?
-The 'use' keyword in PHP is used to include one or more Traits in a class. It allows the class to inherit methods from the specified Traits, making those methods available to the class without the need for full inheritance.
How does the 'insteadof' operator work in PHP when resolving conflicts between Traits?
-The 'insteadof' operator is used to resolve method conflicts between Traits. If two Traits define a method with the same name, you can use 'insteadof' to specify which method should be used by the class. For example, 'Trait1::method insteadof Trait2::method' means Trait1's method will be used.
What is the difference between static and final methods in PHP?
-Static methods belong to a class and can be called without creating an instance of the class, whereas final methods cannot be overridden in any child classes. Static methods are defined with the 'static' keyword, while final methods are defined with the 'final' keyword to prevent further extension or modification.
How does SPL autoloading work in PHP?
-SPL (Standard PHP Library) autoloading automatically loads class files when a class is instantiated without the need to explicitly include the file. The 'spl_autoload_register()' function allows you to register an autoloading function that includes the class file based on the class name, streamlining class management.
Why should you avoid manually including class files in large PHP applications?
-Manually including class files can become cumbersome and error-prone in large PHP applications. Using SPL autoloading ensures that classes are automatically loaded as needed, reducing the risk of forgetting to include necessary files and making the codebase more manageable and efficient.
Can a class directly instantiate a Trait in PHP?
-No, a class cannot directly instantiate a Trait. Traits are designed to be included in classes using the 'use' keyword, allowing their methods to be accessed as if they were part of the class itself. Traits cannot be instantiated because they do not have constructors or instance-specific behavior.
What happens if you try to override a final method in PHP?
-If you attempt to override a final method in a child class, PHP will throw an error. Final methods are meant to prevent modification, ensuring that their behavior remains unchanged, regardless of inheritance.
Outlines
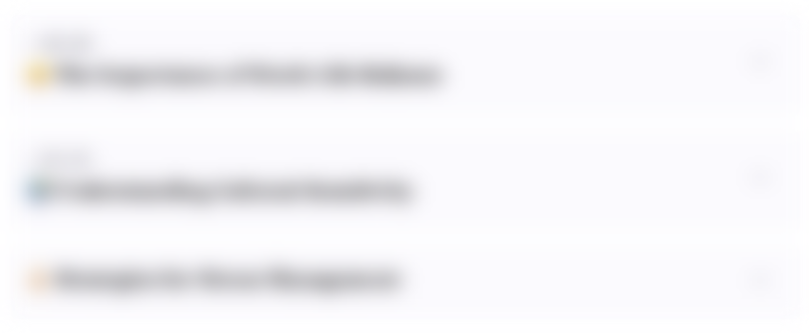
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
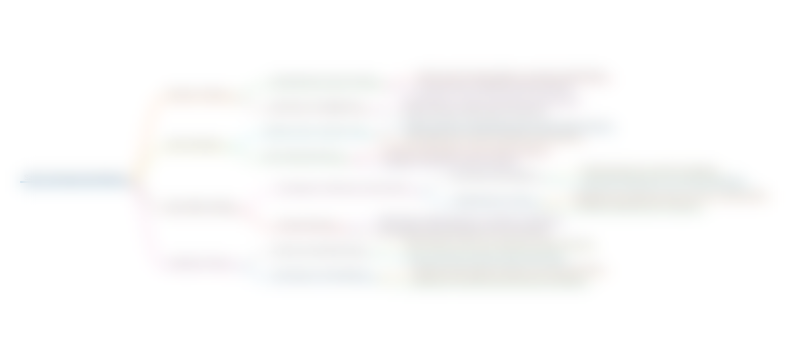
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
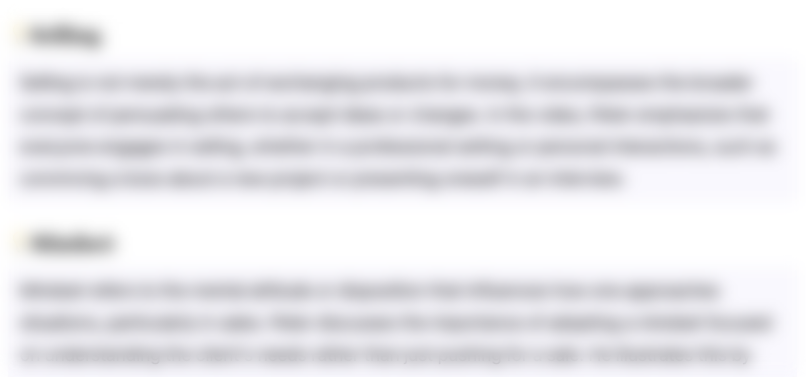
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
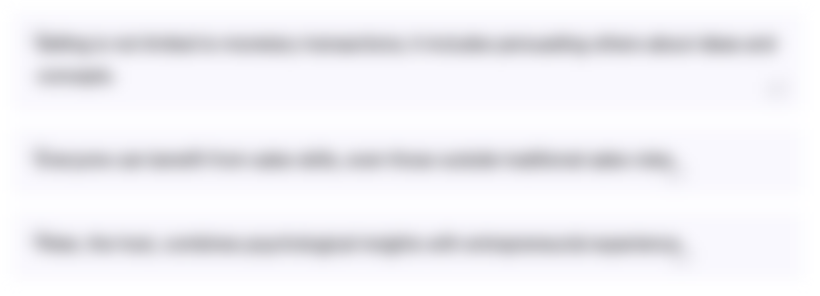
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
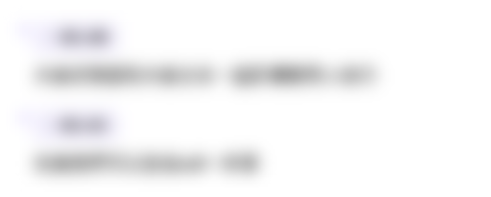
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
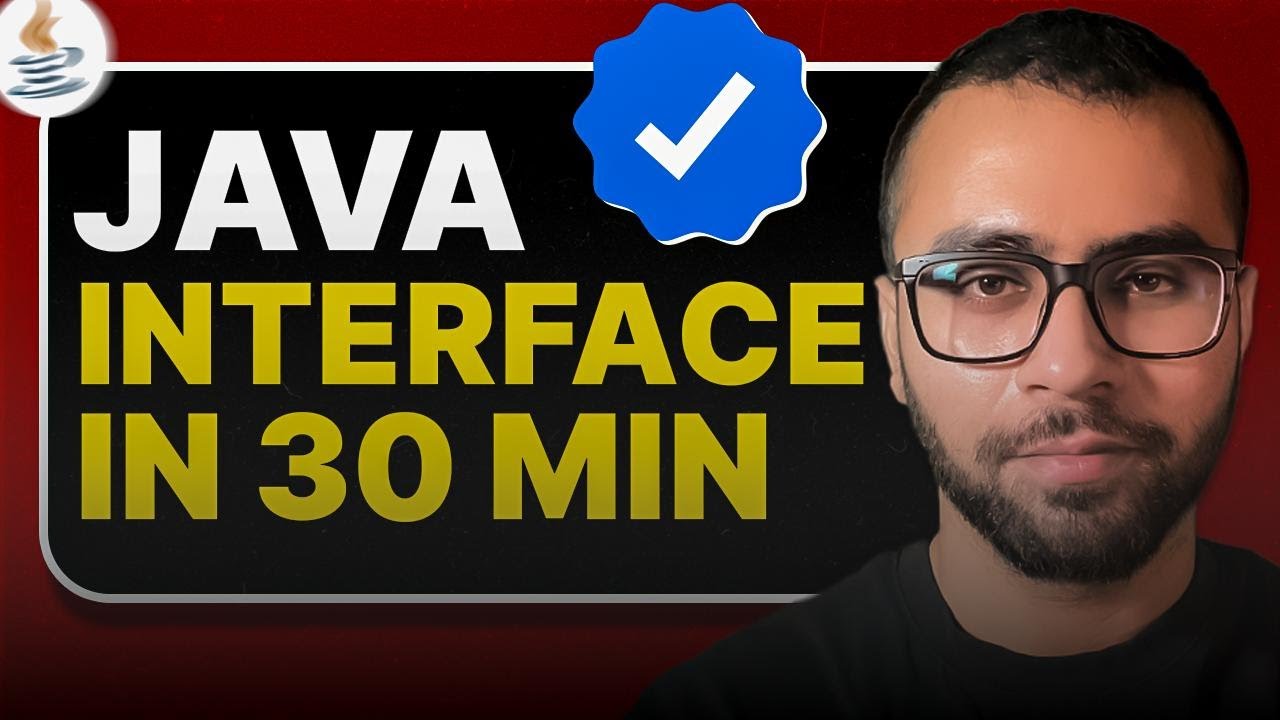
Mastering Java Interfaces: Static & Default Methods, Multiple Inheritance Explained

Belajar PHP Dasar | 15. Belajar OOP PHP Dasar (Inheritance)
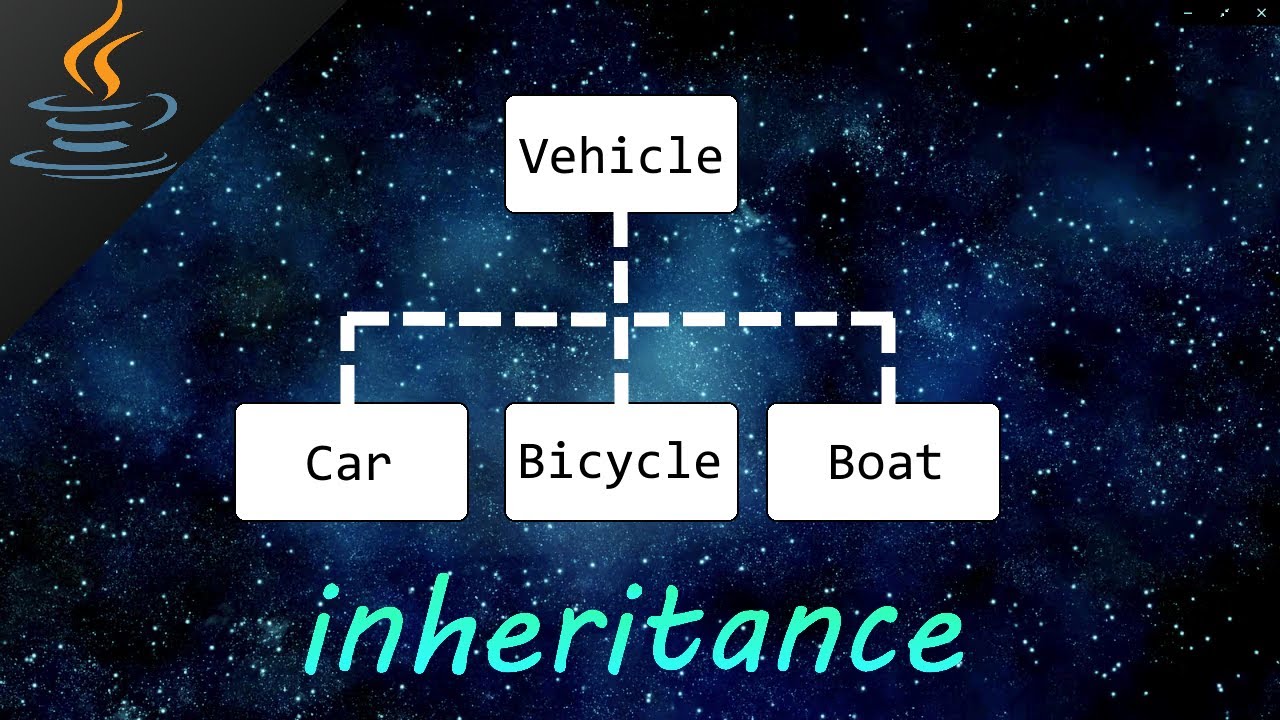
Java inheritance 👪
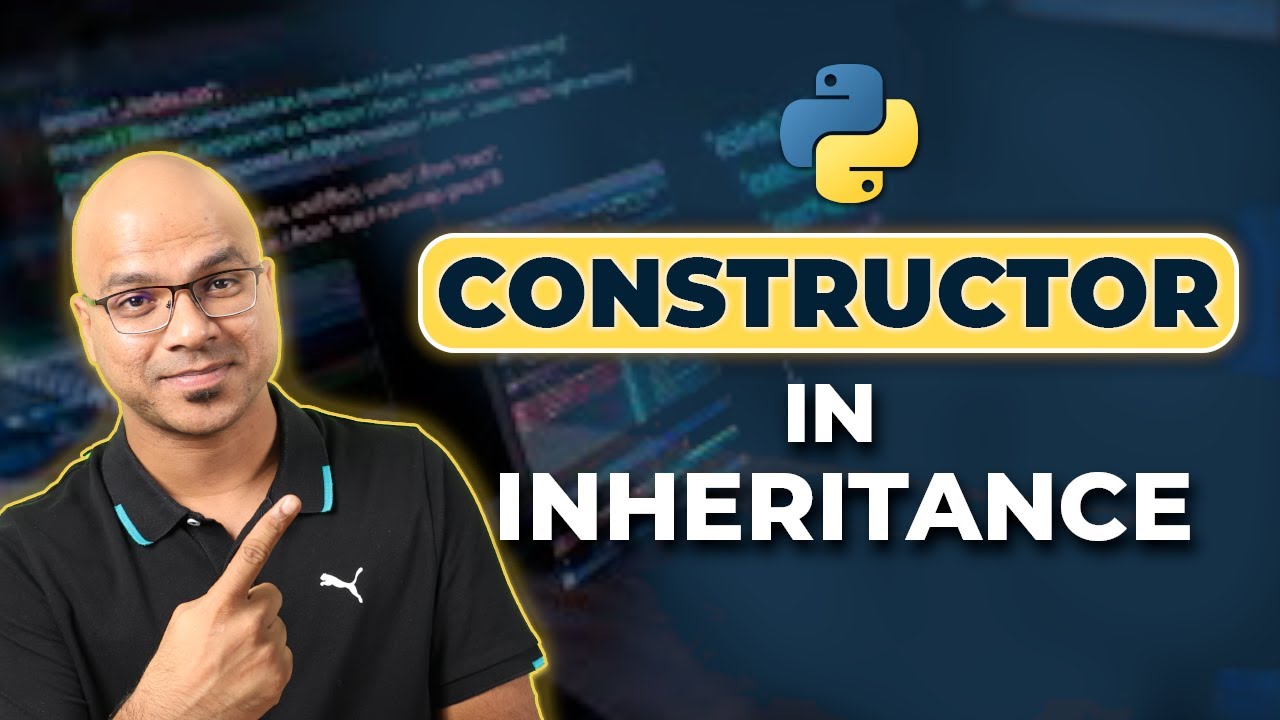
#56 Python Tutorial for Beginners | Constructor in Inheritance
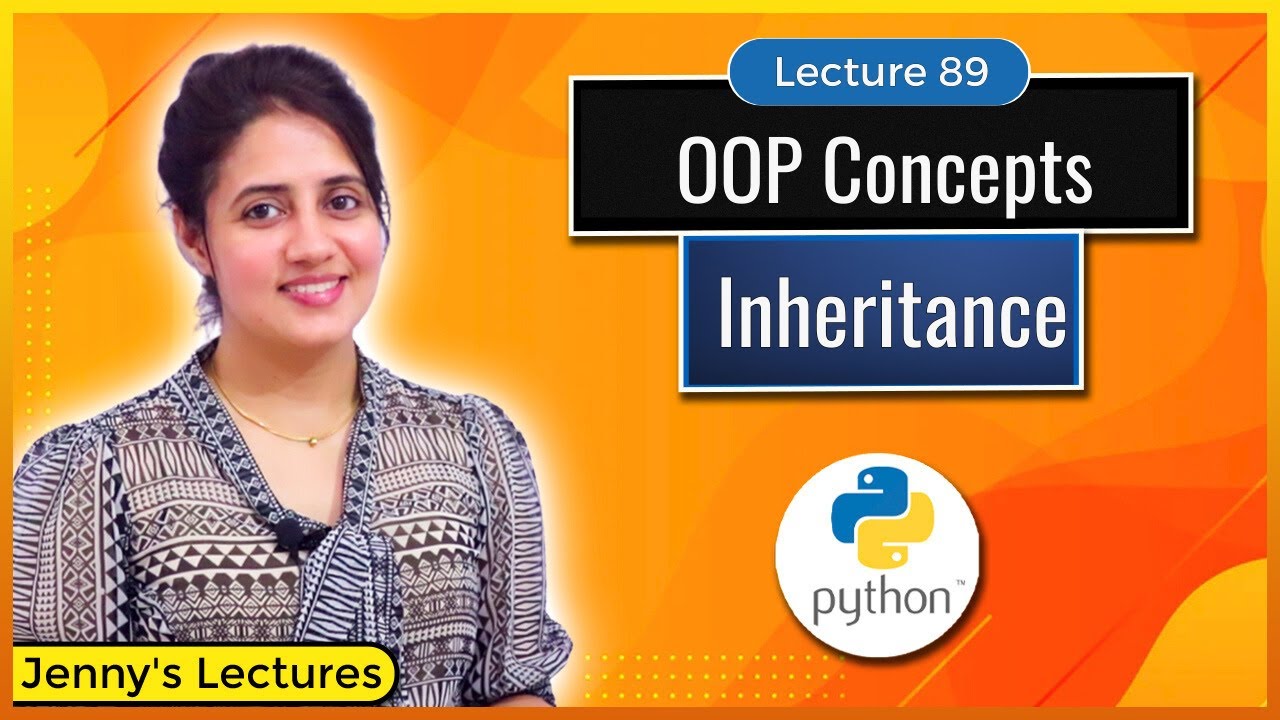
Inheritance in Python | Python Tutorials for Beginners #lec89

Java Inheritance | Java Inheritance Program Example | Java Inheritance Tutorial | Simplilearn
5.0 / 5 (0 votes)