System and Strings Alex and Chris are learning to infiltrate a secure system Starting with a string
Summary
TLDRThis video script outlines a Python programming task in which the user creates a menu-driven interface for a series of word games. The program reads words from a dictionary file and allows the user to select games that find the longest word, words without specific letters, words with single or all vowels, and palindromes. The user input drives the selection of different game options, and the program continues to run until the user chooses to quit. It emphasizes a structured approach to implementing various word games and interacting with files in Python.
Takeaways
- π The task is to create a Python program offering multiple word games in a menu-driven format for the user to choose from.
- π The program reads a dictionary file (dictionary.txt) and uses its content for various word-related games.
- π Each game has its own functionality, such as finding the longest word, words with maximum length, palindromes, and words with specific vowel constraints.
- π The user interacts with the program by selecting a game from a menu, and the input is processed based on their choice.
- π The program must handle both lowercase and uppercase inputs by converting them to lowercase using the 'lower()' method.
- π Functions should be defined for each game, but initially, the functions are left empty and need to be implemented.
- π The dictionary file is opened in 'read' mode and should be properly closed at the end of the program.
- π The program should loop indefinitely until the user chooses 'Q' to quit, after which the dictionary file will be closed.
- π If the user selects an invalid option, the program should print an error message and prompt the user to try again.
- π Each game option will require specific user input, such as letters to exclude, word length, or the list of vowels, depending on the game.
- π The core of the program involves iterating through the dictionary file to check words against the criteria specified by each game.
Q & A
What is the main goal of the Python program described in the transcript?
-The main goal of the program is to create a menu-driven word game system where users can select different word games. The program interacts with a dictionary file and implements various word-based challenges such as finding the longest word, words with specific letters, and palindromes.
How is the dictionary file handled in the program?
-The dictionary file (`dictionary.txt`) is opened at the beginning of the program using the `open()` function in read mode. The file is read and its contents are split into a list of words, which are then used for the word game functionalities.
What is the purpose of the `find_longest_word` function?
-The `find_longest_word` function is intended to find the longest word in the dictionary that contains the letters specified by the user. This function will be implemented with logic to search through the dictionary and identify the appropriate word.
What does the `find_words_without_letters` function do?
-The `find_words_without_letters` function is designed to find words in the dictionary that have the maximum length but do not contain any of the letters specified by the user. The user can exclude certain letters, and the program will filter the words accordingly.
What is the role of the `input()` function in the program?
-The `input()` function is used to prompt the user for input, such as their choice of game and any required letters or criteria. The input is then processed to trigger the corresponding game function or perform actions like filtering words.
How does the program handle user input for game selection?
-The program uses a `while True` loop to continuously display the menu of game options to the user. Based on the user's input (e.g., 'a', 'b', 'q'), the program calls the corresponding function for the selected game. If an invalid choice is entered, the user is prompted to try again.
Why is the dictionary file closed at the end of the program?
-The dictionary file is closed at the end of the program using the `close()` method to free up system resources. This is important for maintaining proper file handling and preventing potential memory leaks or file access issues.
What does the program do if the user chooses 'q'?
-If the user chooses 'q', the program breaks out of the loop, effectively ending the program. The dictionary file is then closed, and the program concludes.
What are some of the word games listed in the menu of the program?
-The word games listed in the menu include finding the longest word containing specified letters, finding words with maximum length excluding specific letters, finding words with exactly one vowel, finding words containing all vowels, and finding palindromes.
What is the significance of using the `lower()` method on user input?
-The `lower()` method is used to convert the user's input to lowercase, which ensures that the program can handle both uppercase and lowercase inputs in a case-insensitive manner. This helps avoid errors due to case mismatches in user input.
Outlines
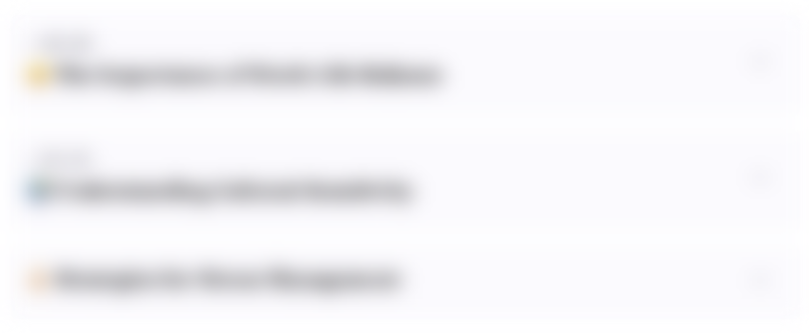
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
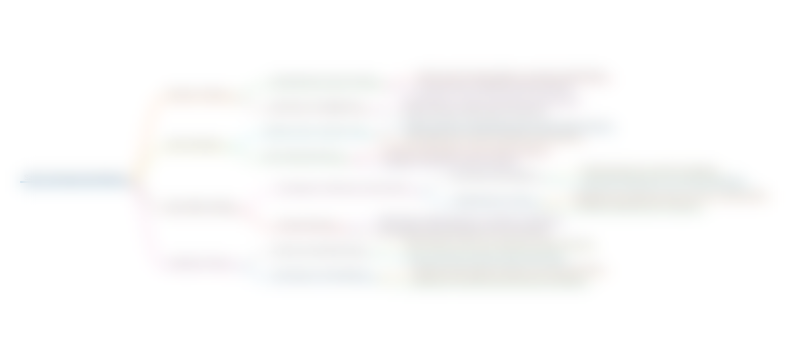
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
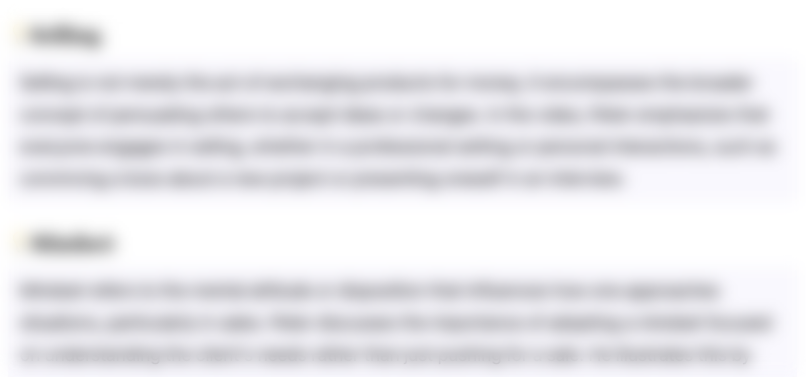
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
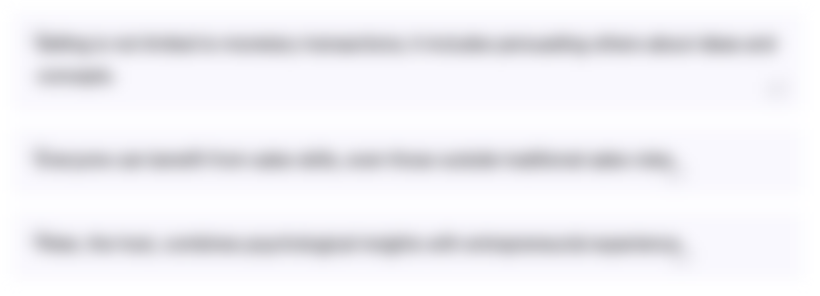
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
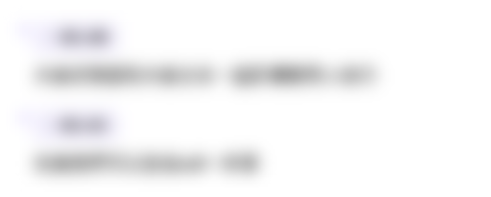
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
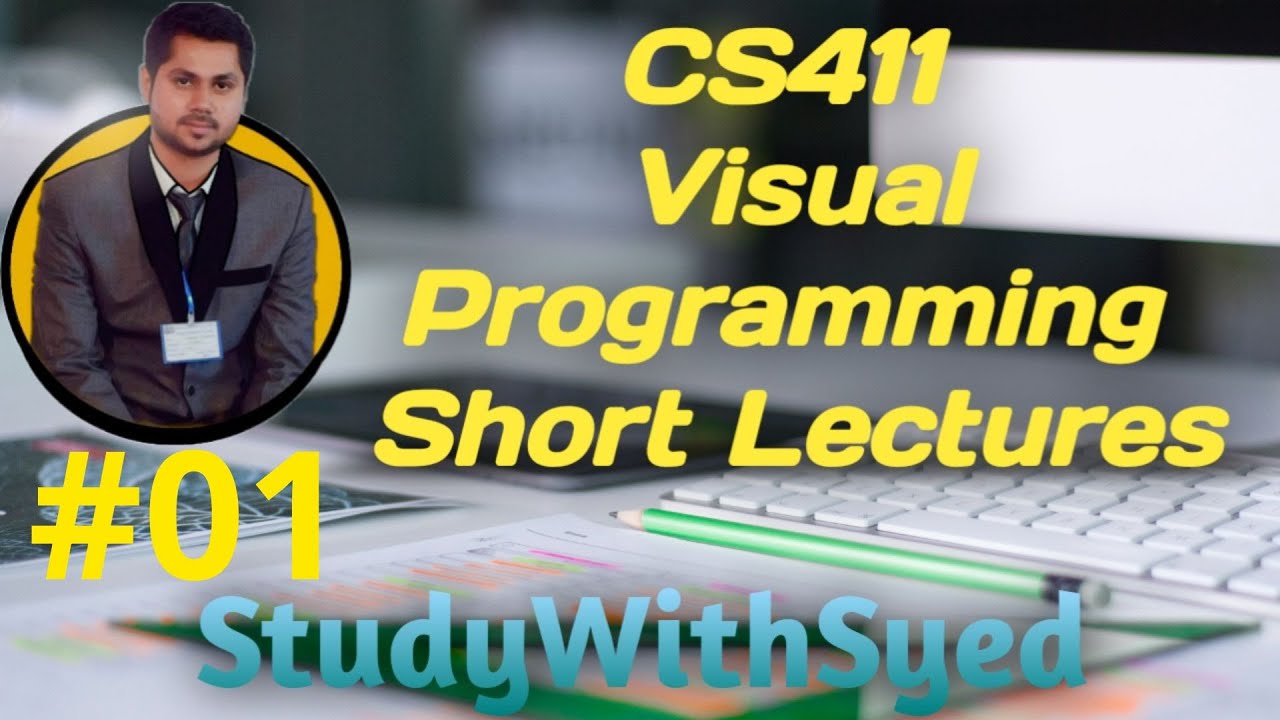
CS411 Lecture 1, Visual Programming short lecture 1
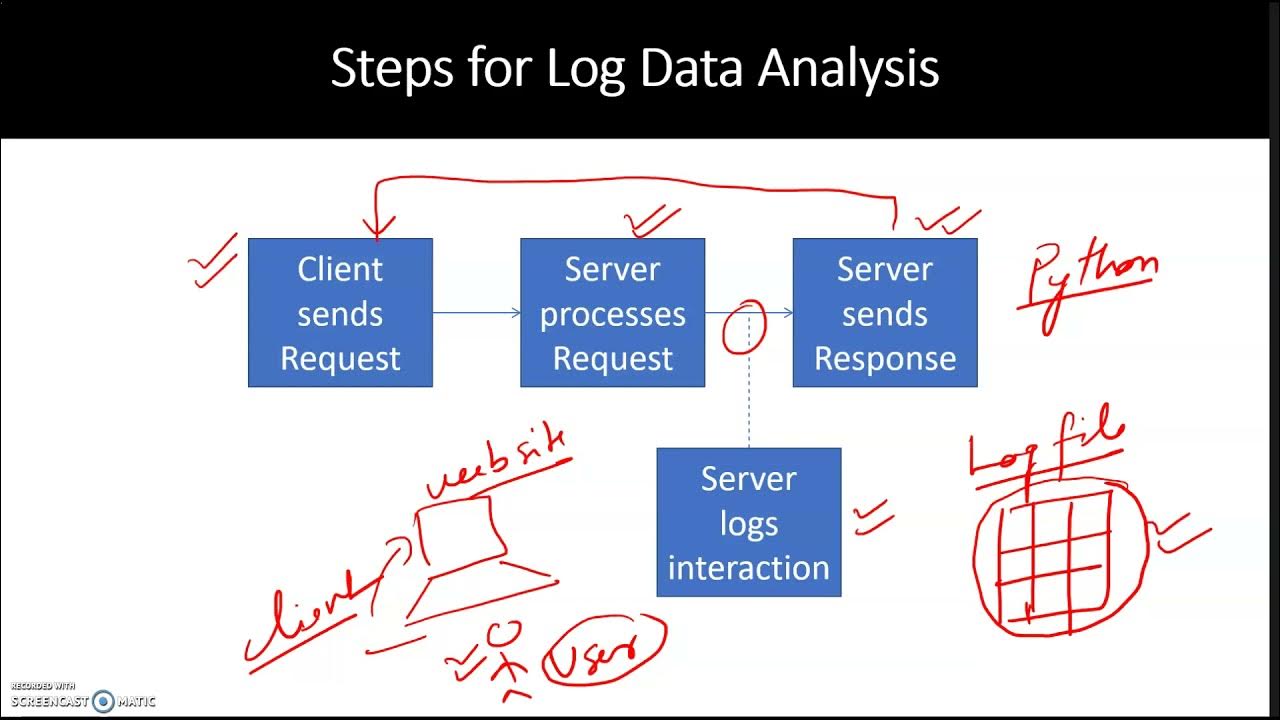
Data Analysis Processing Web Log Data
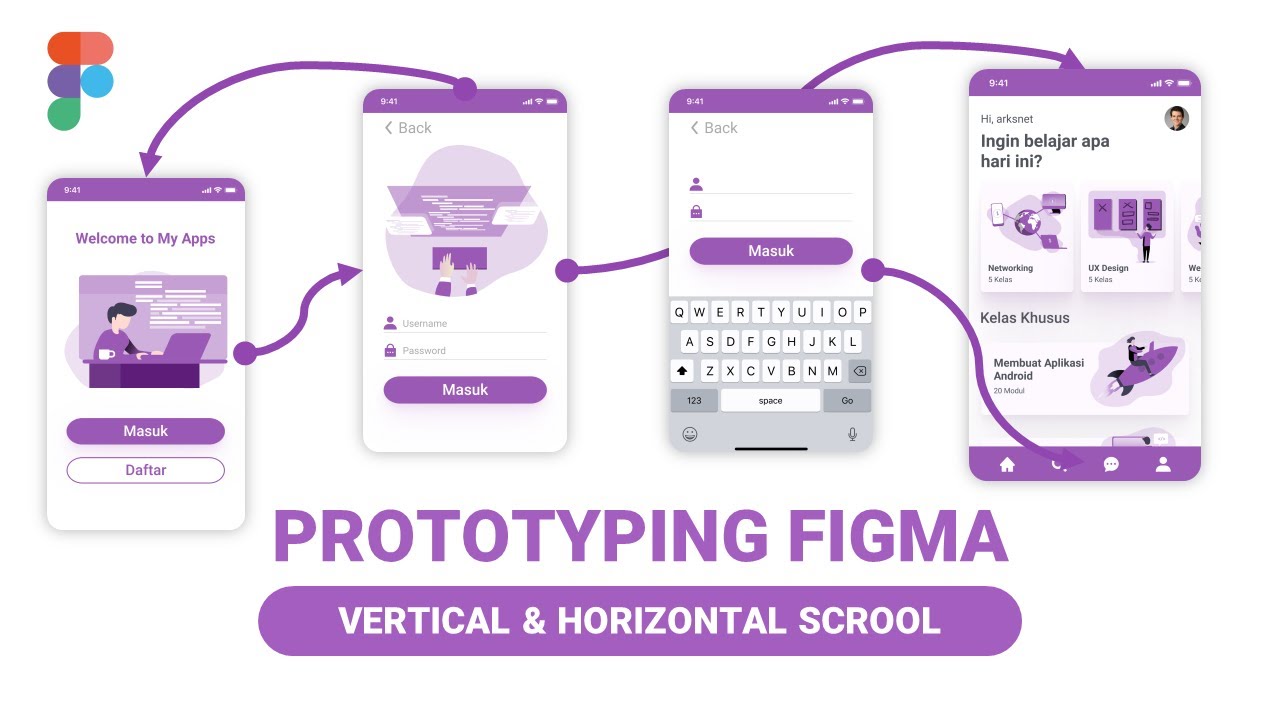
Membuat Prototyping & Interactions di Figma - Belajar Figma Bareng (3/3)
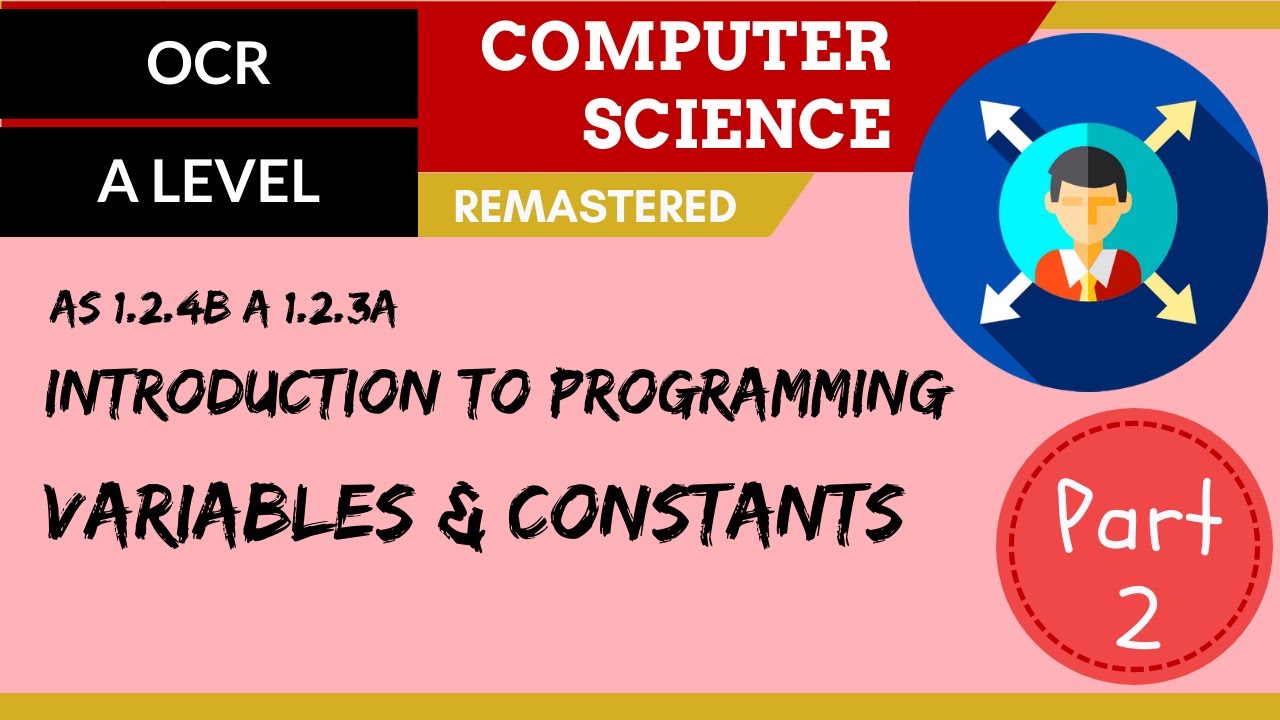
41. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 2 variables & constants
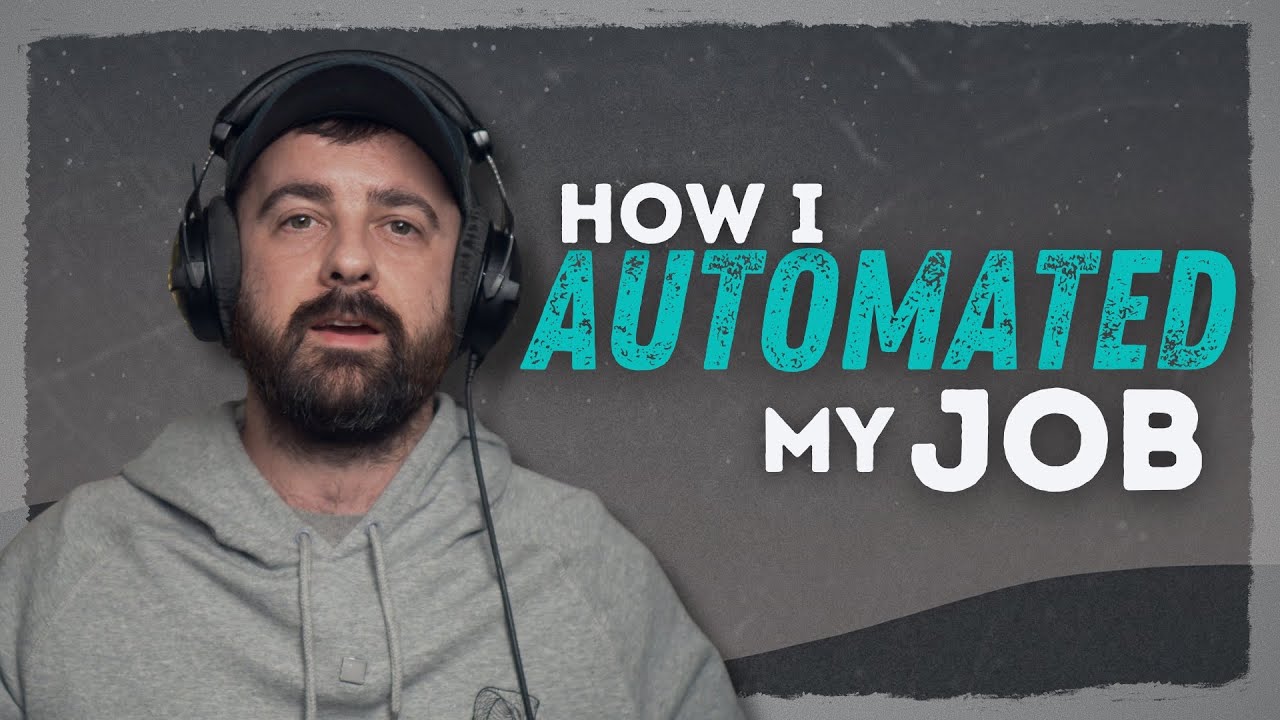
The most important Python script I ever wrote
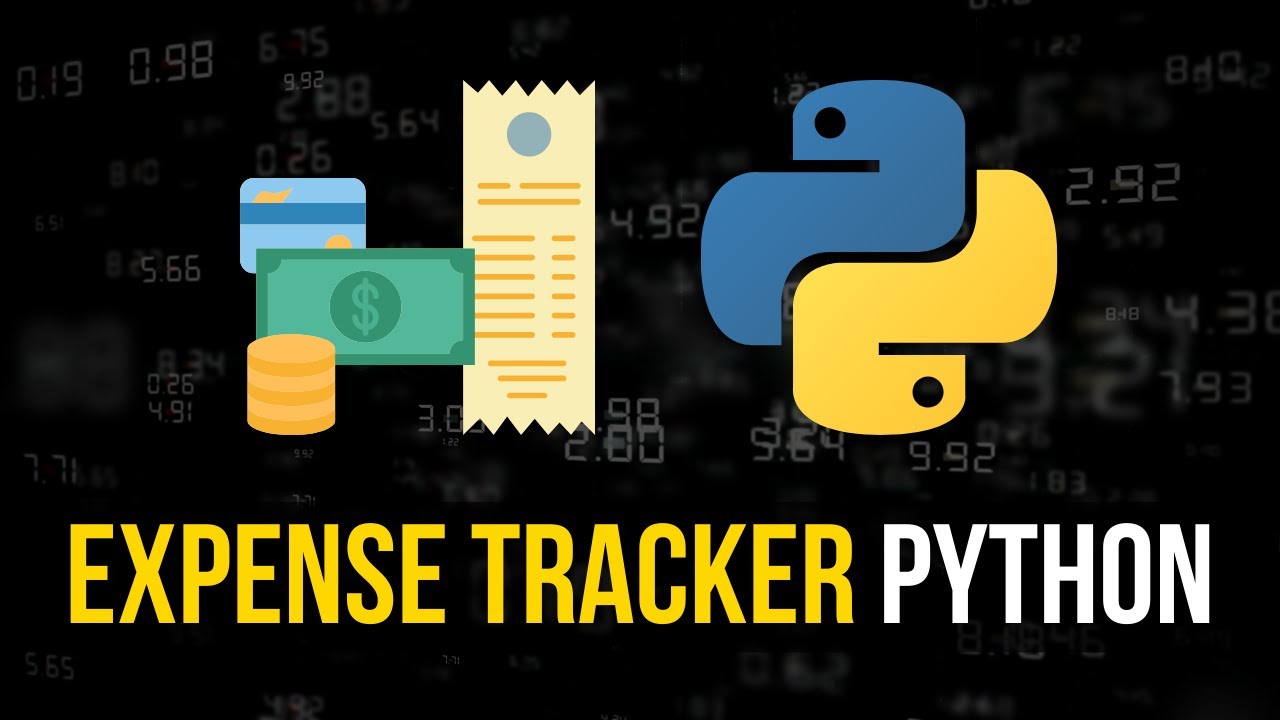
Professional Expense Tracker in Python
5.0 / 5 (0 votes)