ABAP OO Refresher - Module 1B: From functions to objects - sample solution
Summary
TLDRThis video presents a detailed sample solution to a programming problem involving car objects. The solution demonstrates how to define a class with methods for refueling, driving, and calculating the remaining fuel in the tank. Key concepts include constructor initialization, method implementation, and using assertions to verify correctness. The tutorial also walks through object creation and interactions, such as refueling and driving distances, highlighting debugging techniques and practical testing with assertions. The sample solution is a hands-on guide to understanding object-oriented principles, focusing on class behavior, fuel management, and error handling in a real-world scenario.
Takeaways
- 😀 The script explains how to design and implement a basic car class in object-oriented programming, with methods for refueling, driving, and tracking fuel consumption.
- 😀 The car class includes key attributes: `name`, `tank capacity`, `fuel consumption`, and `current tank level`, which define the state of the car object.
- 😀 The constructor method initializes these attributes, setting the tank level to 0% and converting fuel consumption to a per-liter rate for easier calculations.
- 😀 The `refuel` method returns the amount of fuel added to the tank, ensuring the current tank level reflects the refueled quantity.
- 😀 The `drive distance` method calculates the fuel consumption for a given distance and adjusts the current tank level accordingly. It also includes a safeguard to prevent the car from running out of fuel.
- 😀 The `get current tank level` method calculates and returns the percentage of fuel remaining in the tank, making it easy to monitor fuel status.
- 😀 The main program flow involves creating instances of the car class for different cars (VW Golf, Porsche, Hummer) and simulating real-world actions like refueling and driving.
- 😀 Assertions are used to ensure that the program behaves correctly, such as verifying that the tank levels decrease after driving and remain at 100% after refueling.
- 😀 Debugging is demonstrated by stepping through the code in the debugger, showing how object attributes are set during object creation and how methods are called during runtime.
- 😀 The script introduces basic error handling using assertions to detect and prevent invalid operations, like attempting to drive when the car doesn't have enough fuel.
- 😀 The solution demonstrates a simple yet effective way to model real-world behavior (fuel consumption, refueling, driving distances) using object-oriented principles and methods.
Q & A
What is the main objective of the example code provided in the transcript?
-The main objective is to demonstrate how to implement a car class that supports operations such as refueling, driving a distance, and getting the current fuel level, using object-oriented programming principles.
What are the key methods defined in the public section of the car class?
-The key methods in the public section are the constructor, refuel, drive distance, and get current tank level. These methods handle initializing the car, refueling, simulating driving, and calculating the current fuel level.
How does the refuel method in the car class work?
-The refuel method calculates how much fuel can be added to the car's tank based on the current fuel level and the tank's capacity, then returns the amount of fuel added.
What is the purpose of the 'drive distance' method?
-The 'drive distance' method calculates the amount of fuel required to drive a given distance based on the car's fuel consumption rate and the distance in kilometers. It then updates the fuel level accordingly.
Why are assertions used in the example code?
-Assertions are used to verify the correctness of the program's logic. For example, after refueling or driving, assertions ensure that the tank level is updated correctly, either confirming that the fuel consumption happened or that the car's tank is full.
What does the get current tank level method return?
-The get current tank level method returns the current fuel level in the tank as a percentage of the tank's total capacity.
Why is the constructor of the car class important?
-The constructor initializes the car object with essential attributes, including the car's name, tank capacity, and fuel consumption rate, ensuring that these values are set when the car object is created.
How does the 'drive distance' method handle situations where there isn't enough fuel?
-If the car doesn't have enough fuel to drive the specified distance, the method will throw an assertion error, indicating that the car has run out of fuel and cannot complete the drive.
What is the role of the debugger in the example code?
-The debugger is used to step through the code, allowing the programmer to observe the initialization of car objects, the behavior of methods like refuel and drive distance, and the proper updating of the fuel levels. It helps ensure that the logic works as intended.
Why is the fuel consumption rate divided by 100 in the code?
-The fuel consumption rate is divided by 100 to simplify the calculation of how much fuel is needed per kilometer. This allows the program to handle fuel consumption in terms of liters per 100 kilometers and perform the necessary calculations more easily.
Outlines
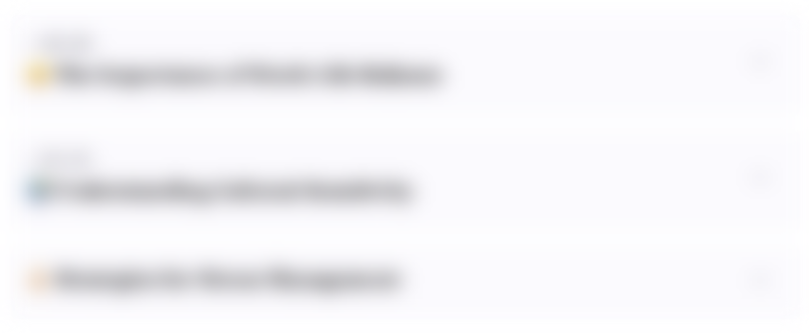
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
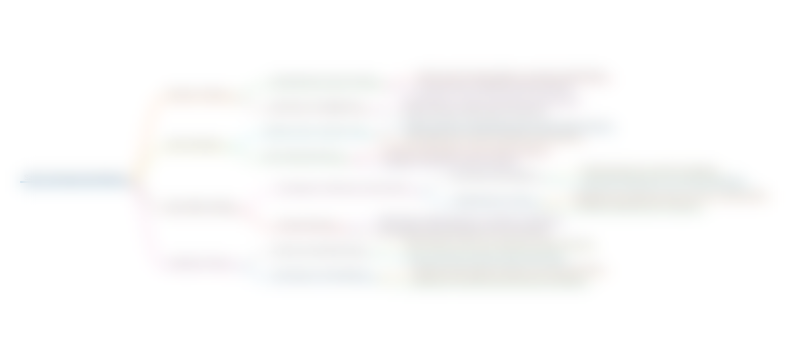
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
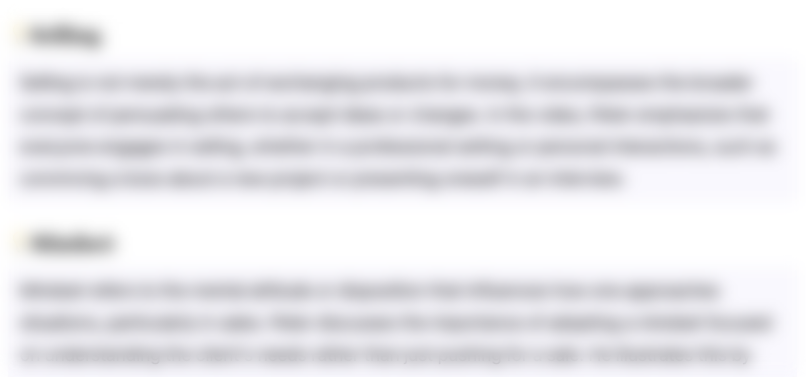
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
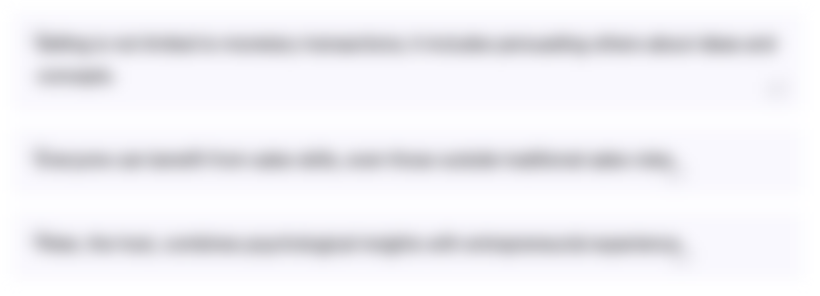
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
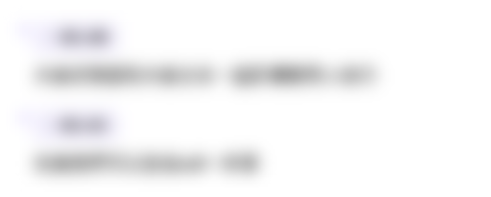
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
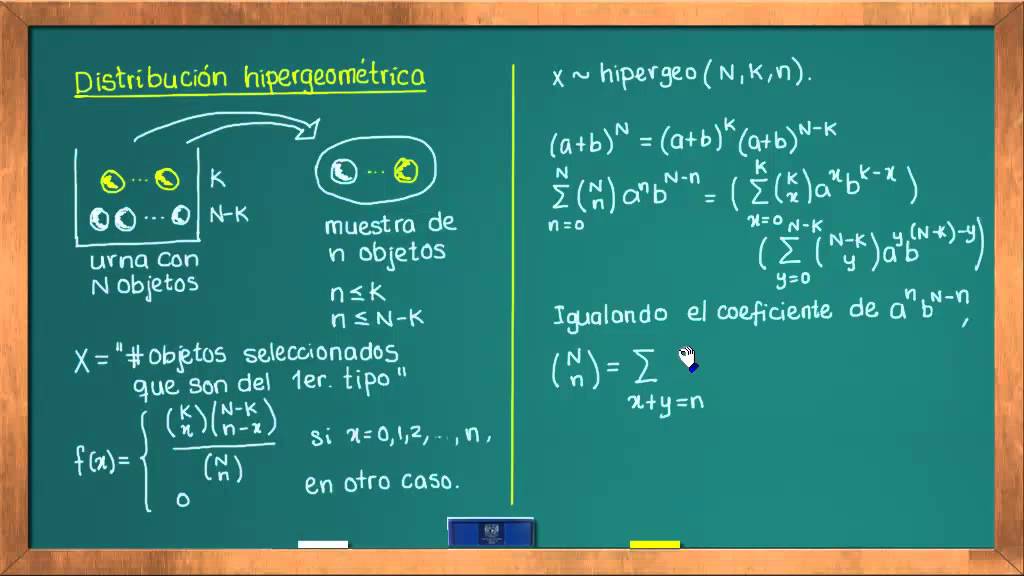
0625 Distribución hipergeométrica

Metode linear programing | Metode Simplex | Matematika Bisnis | OR 2022
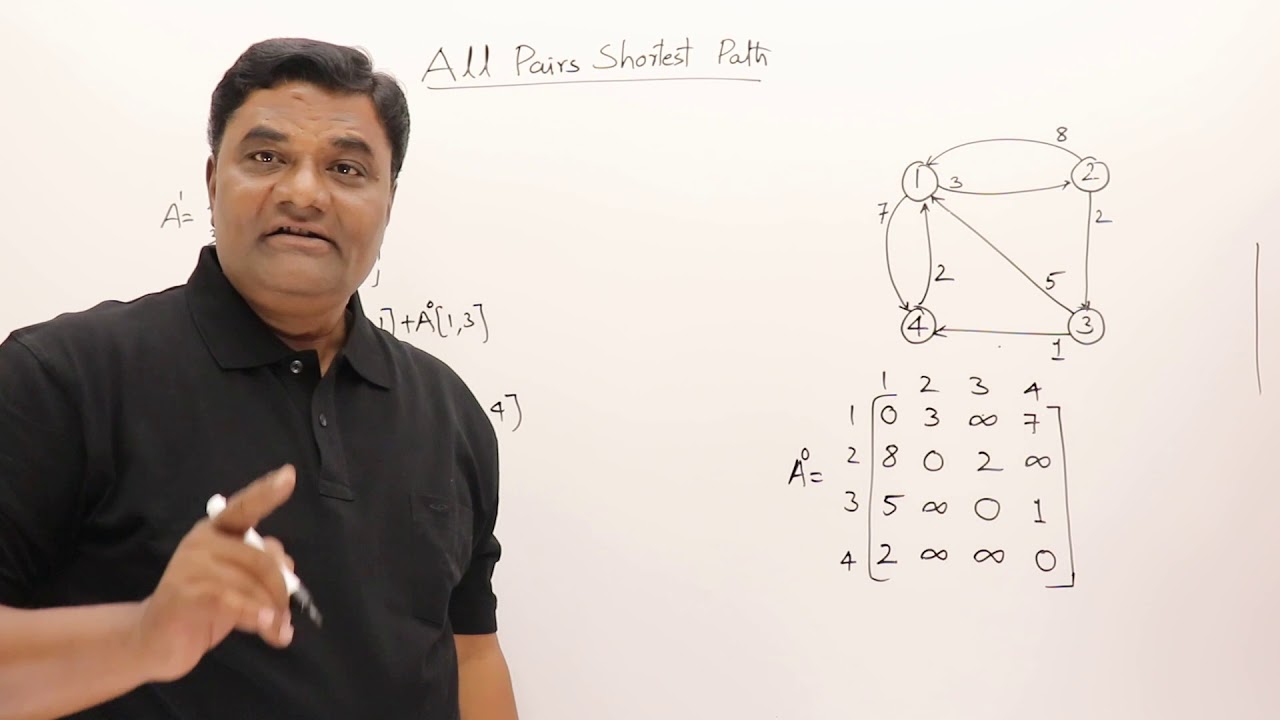
4.2 All Pairs Shortest Path (Floyd-Warshall) - Dynamic Programming
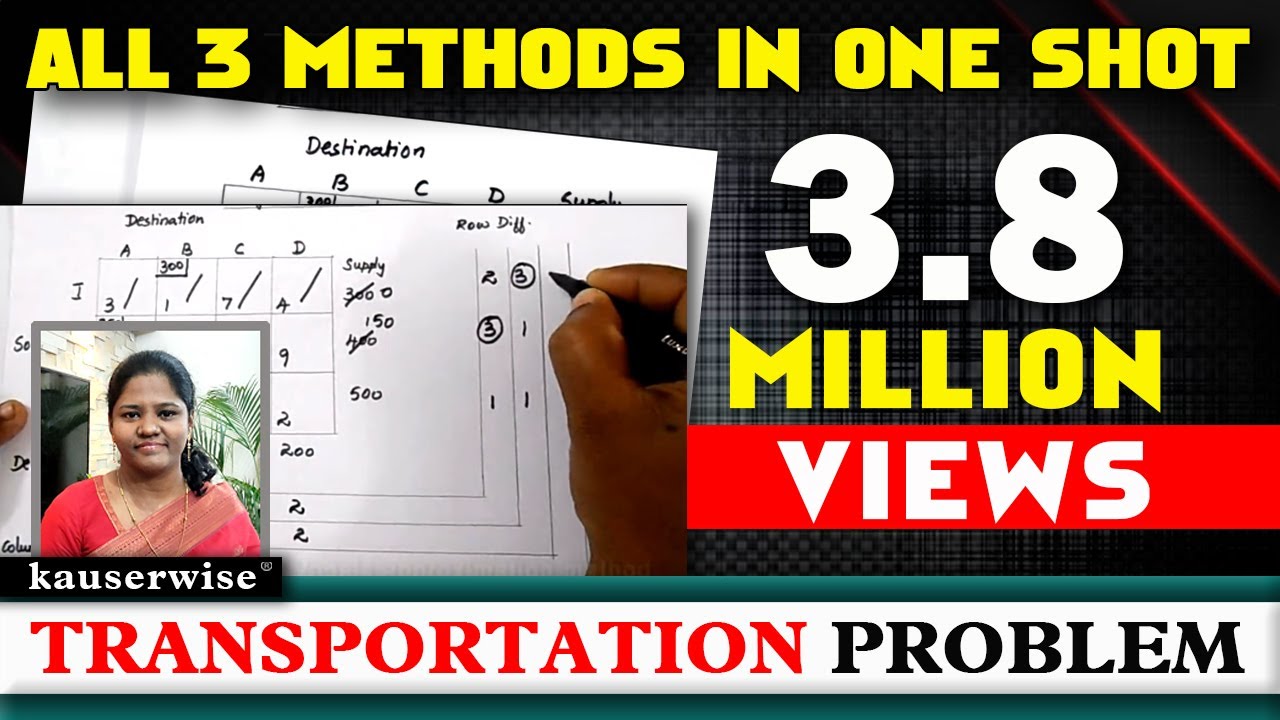
Transportation problem||vogel's approximation[VAM]|Northwest corner||Least cost | by Kauserwise
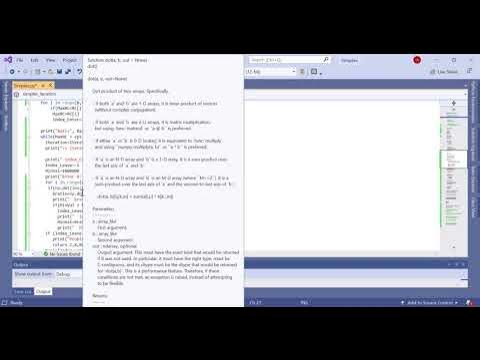
How To Implement the Simplex Algorithm with Python?
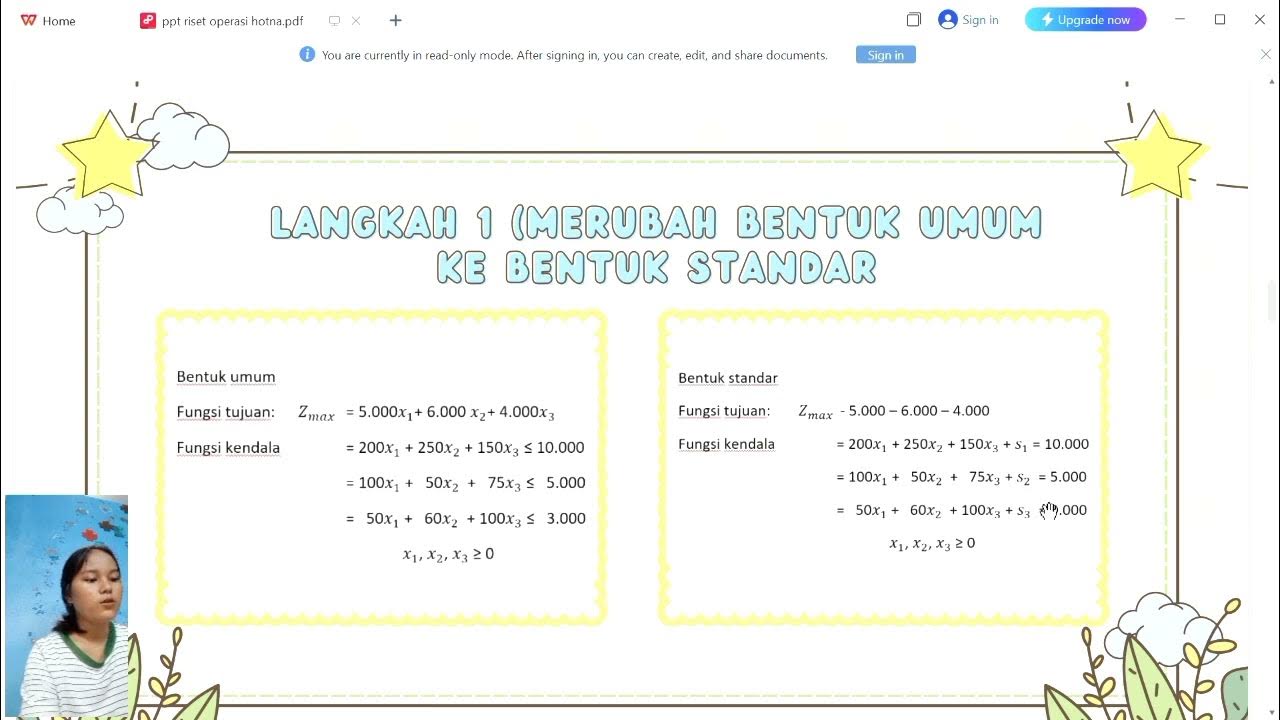
Program Linear Metode Simpleks Soal Cerita 3 Variabel
5.0 / 5 (0 votes)