C++ programming, use a function to input 2 numbers, calculate and display exponent with functions
Summary
TLDRIn this programming tutorial, Professor Liu walks through the creation of a program that calculates the result of raising a base number to an exponent. The process is broken into three main parts: gathering input, calculating the result, and displaying the output. The program uses functions to handle each task and includes a loop that allows users to repeat the calculation as desired. By explaining the logic behind using references, function calls, and looping, the tutorial demonstrates a clean and efficient way to structure a program that involves user interaction and repeated operations.
Takeaways
- π A clear program structure is essential: The program is divided into three parts: input, calculation, and output.
- π Functions help organize the code: The program uses multiple functions to handle distinct tasks (getting input, calculating, and displaying results).
- π Use of 'void' function for input: The 'getInput' function uses pass-by-reference parameters to directly modify input values.
- π Returning values: The 'calculate' function returns the result of the computation instead of using pass-by-reference for output.
- π Displaying results: The 'display' function outputs the base, exponent, and result in a readable format.
- π Loops improve usability: A loop is used to ask the user if they want to perform another calculation, allowing for repeated calculations.
- π Case-insensitive user input: The program handles both uppercase and lowercase inputs (e.g., 'Y' and 'y') when asking whether to repeat the calculation.
- π Program flow is modular: Each function has a clear responsibility, which makes the code more organized and easier to understand.
- π Use of prototypes: Function prototypes are declared before definitions to inform the compiler about function signatures.
- π User-friendly design: The program ensures clarity by providing prompts and output that are easy for the user to follow (e.g., 'Enter base' and 'Do you want to do it again?').
- π Clear debugging: The program is tested with various inputs to ensure it works as expected (e.g., testing with base 5 and exponent 3).
Q & A
What is the purpose of the program in the transcript?
-The program calculates the result of a base raised to an exponent, such as 3 raised to the power of 2 (3^2).
What are the three main parts of the program as described by the professor?
-The program consists of three main parts: 1) Getting input (base and exponent), 2) Calculating the result, and 3) Displaying the result.
Why does the professor choose to use a void function for the input?
-A void function is used because it allows the professor to pass values by reference, meaning the function can modify the values of the input variables (base and exponent).
What is the role of the prototype in this program?
-The prototype defines the structure of the function (like 'get input', 'calculate', and 'display'), allowing the program to know the type of arguments and return values before their actual implementation.
What is the significance of the 'double' data type in the calculation function?
-The 'double' data type is used in the calculation function to ensure the result is accurate and can handle floating-point numbers.
How does the program pass values back and forth between functions?
-The program uses pass-by-reference for variables like the base and exponent, meaning changes made in the functions directly affect the original values.
What does the professor mean by 'display base, exponent, and result'?
-The program will output the values of the base, exponent, and the result of the base raised to the power of the exponent to the user.
What role does the loop play in the program?
-The loop is used to allow the user to repeatedly calculate powers of different numbers by asking if they want to perform another calculation after each result.
What input does the program expect for continuing after each calculation?
-The program prompts the user with 'Do you want to do it again?' and expects the user to respond with either 'yes' or 'no'. The program will continue if 'yes' is entered.
Why does the professor use the 'toLower' function for user input?
-The 'toLower' function is used to handle both uppercase and lowercase responses ('yes' or 'no'), making the program more user-friendly and less error-prone.
Outlines
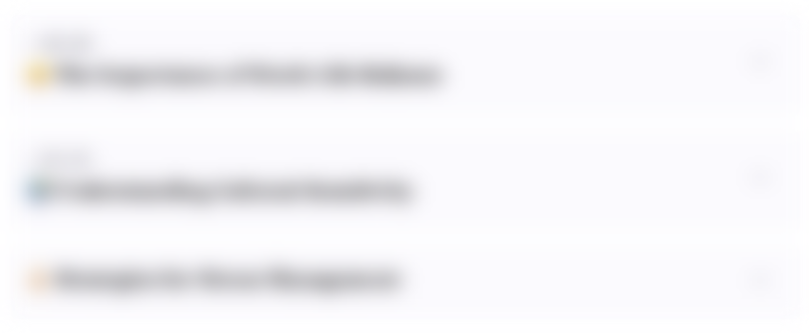
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
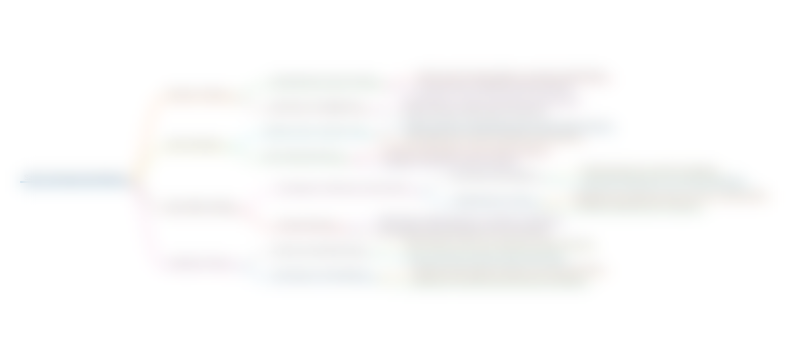
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
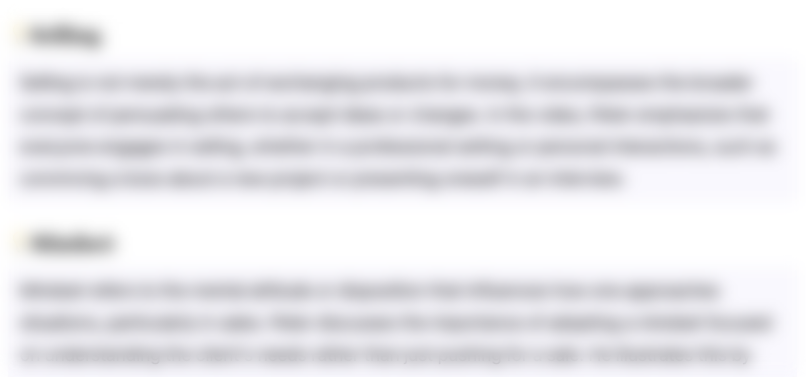
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
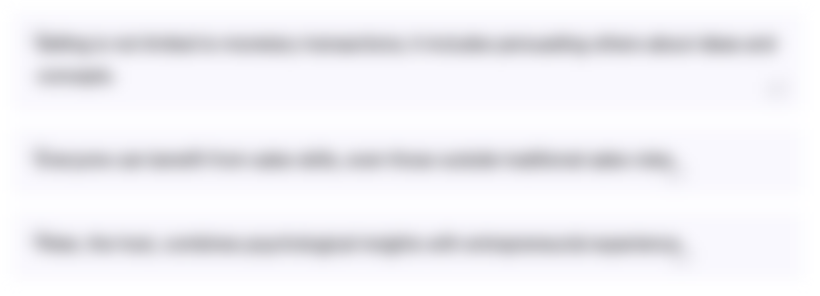
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
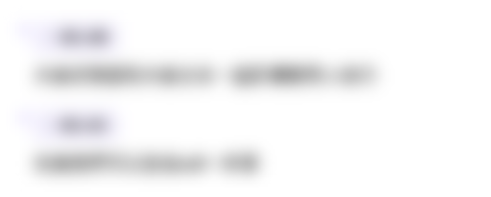
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
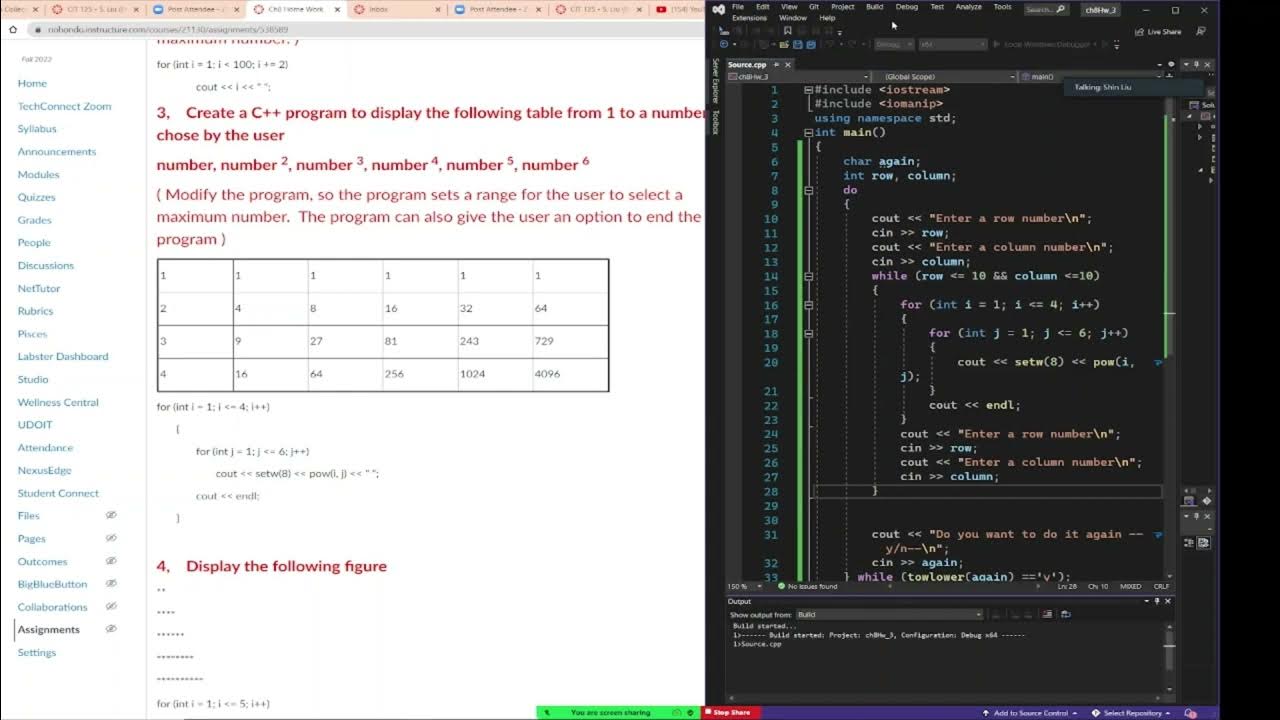
C++ programming, for loop to create a exponential table, do while loop to continue
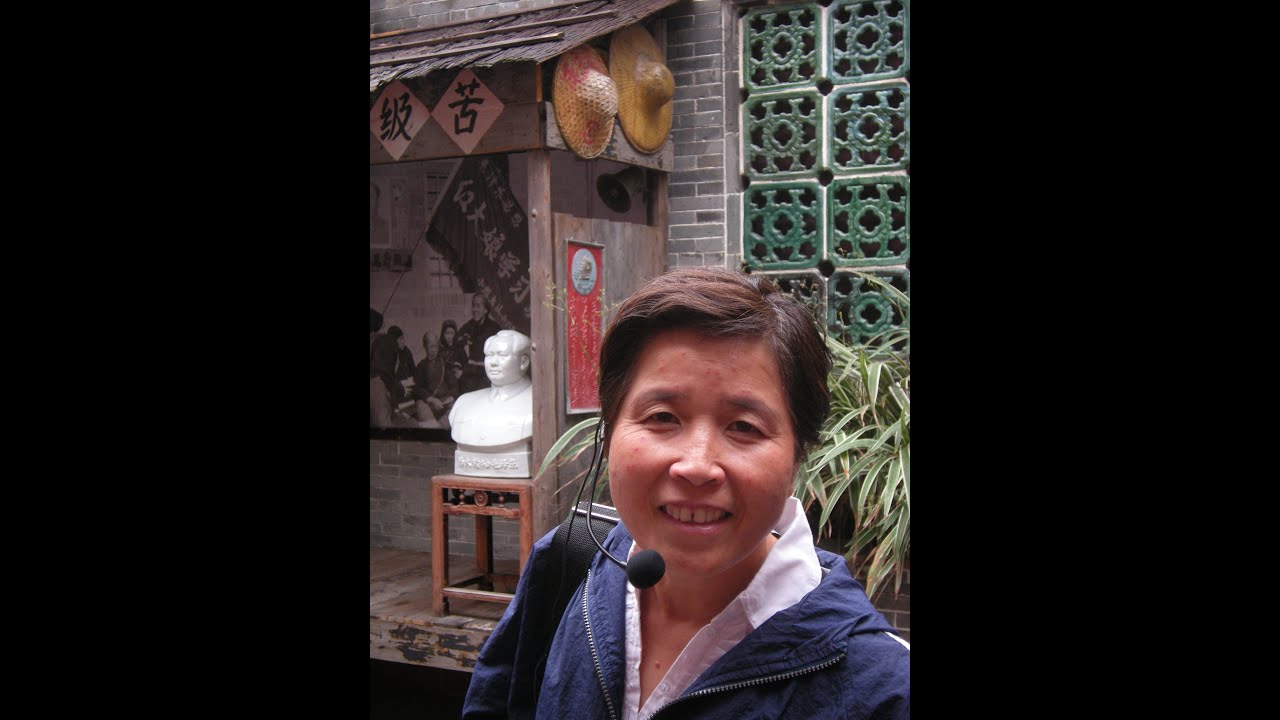
C++ programming call functions to input 2 numbers, calculate and display the sum and differences.
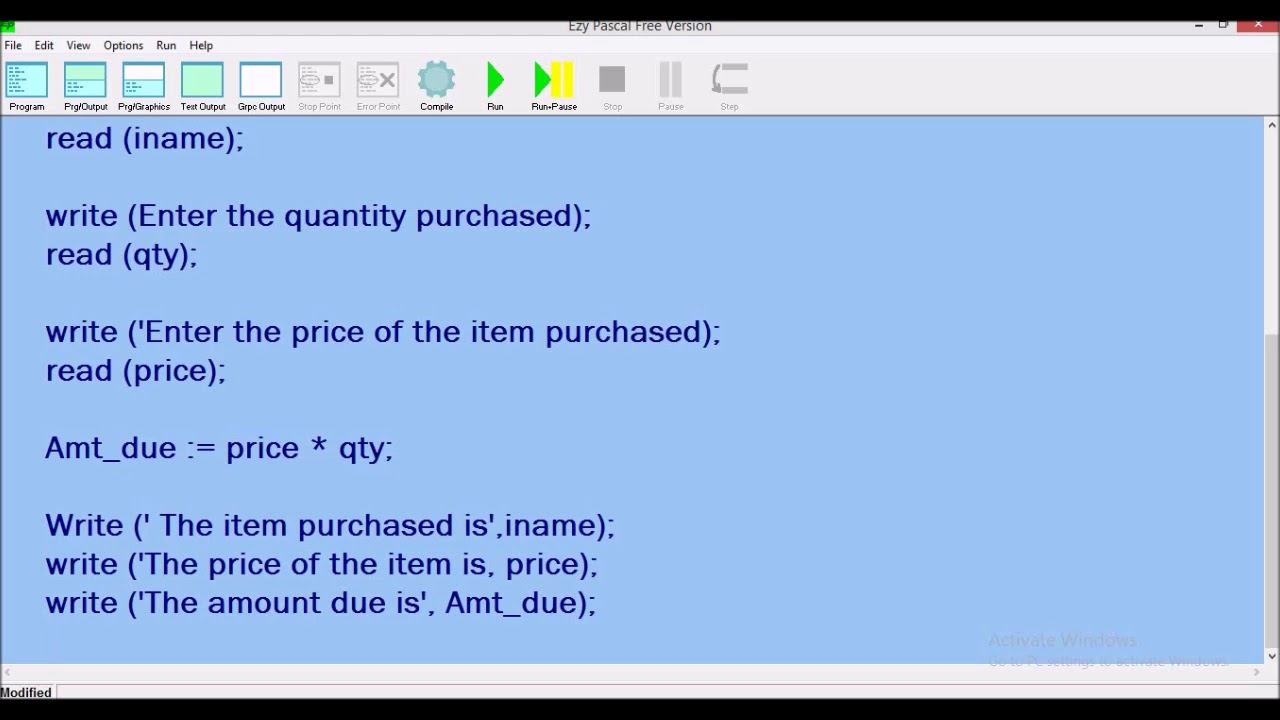
Ezy Pascal Tutorial No 1

Scratch - Kondisi If-Else Sederhana #5

PEMOGRAMAN PYTHON (FUNGSI LOGIKA)
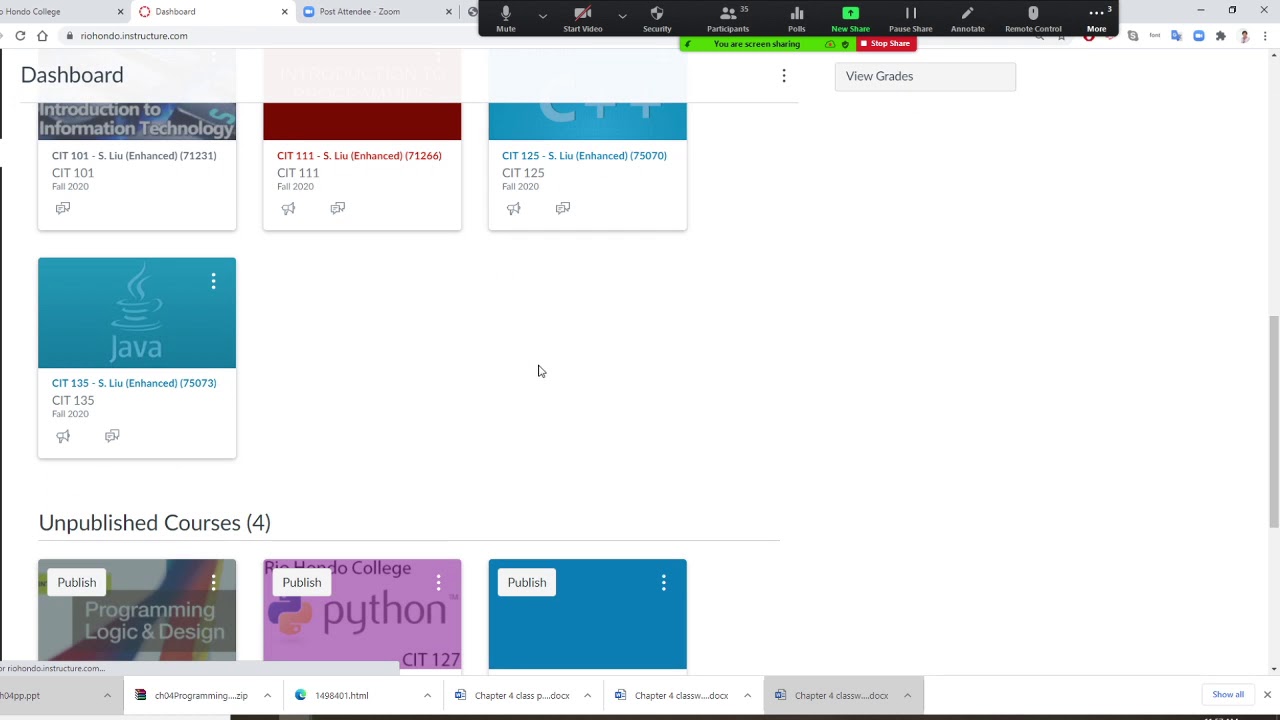
C++ Programming For loop Introduction, setw function
5.0 / 5 (0 votes)