Ezy Pascal Tutorial No 1
Summary
TLDRThis Pascal tutorial walks through writing a program that calculates the total price of an item based on user input. The script guides users in declaring variables, accepting inputs for the item name, quantity, and price, and performing the necessary calculations to determine the total amount due. It also covers error handling, formatted output with two decimal places, and incorporating tax calculations using constants. The tutorial emphasizes basic Pascal programming concepts, such as input/output functions, variable declarations, and debugging, to ensure the program works correctly and displays the final results neatly.
Takeaways
- 😀 The script introduces a Pascal program that asks users to input an item's name, quantity, and price, and then calculates the total amount due.
- 😀 The program begins by declaring necessary variables: item name, price (real), quantity (integer), and amount due (real).
- 😀 The user inputs are captured using `ReadLn` for item name, quantity, and price, while output is displayed using `WriteLn`.
- 😀 The calculated amount due is the result of multiplying the price by the quantity and is stored in the `amountDue` variable.
- 😀 To ensure proper formatting, the output for prices and amounts is displayed to two decimal places using the `:0:2` format.
- 😀 To avoid data overflow, the script uses a constant tax rate of 16.5%, represented as 0.165 in the program.
- 😀 A new `tax` calculation is introduced, which computes the amount of tax based on the total price before tax.
- 😀 The program ensures clarity by labeling each output: item name, price, quantity, amount due, and tax amount.
- 😀 The program demonstrates the importance of proper input validation and error handling during real-world coding exercises.
- 😀 The tutorial emphasizes the importance of good programming practices, such as proper indentation and correct variable naming conventions.
- 😀 The script includes small fixes during testing, such as switching `Write` to `WriteLn` for better output formatting and adjusting the tax calculation for accuracy.
Q & A
What is the main objective of the Pascal program in the script?
-The main objective of the Pascal program is to take user inputs for the name, price, and quantity of an item, calculate the total amount due, and display the information to the user.
What are the key inputs required for the program?
-The key inputs required for the program are the item name, price, and quantity purchased.
Why is the 'real' data type used for the price and amount due variables?
-'real' is used for the price and amount due variables because these values can include decimal places, representing monetary amounts accurately.
What does the statement 'amountDue := price * quantity' accomplish in the program?
-This statement calculates the total cost by multiplying the price of the item by the quantity purchased and stores the result in the 'amountDue' variable.
What is the purpose of the 'write' and 'readln' statements in the program?
-'write' is used to prompt the user for input, and 'readln' is used to read the user's input and store it in the corresponding variable.
How is the output formatted in the program, especially for the price and amount due?
-The output is formatted using 'writeln' to display the item name, price, and amount due, with the price and amount due rounded to two decimal places using the '0:2' format.
What is the significance of the tax calculation section added to the program?
-The tax calculation section demonstrates how to calculate and display tax on the purchase based on a fixed tax rate (16.5%) and adds it to the total amount due.
How does the program handle errors such as missing semicolons or incorrect variable declarations?
-The program addresses common errors by ensuring proper syntax, such as adding semicolons at the end of statements and ensuring variables are declared before use.
Why is 'const' used in the tax calculation, and how is the tax rate expressed?
-'const' is used to define a constant value for the tax rate, which ensures that the rate does not change during the program execution. The tax rate is expressed as a decimal (0.165 for 16.5%).
What changes were made to fix the issue of information being displayed on the same line?
-The program uses 'writeln' instead of 'write' to ensure that each piece of information is displayed on a new line, preventing the output from running across the screen.
Outlines
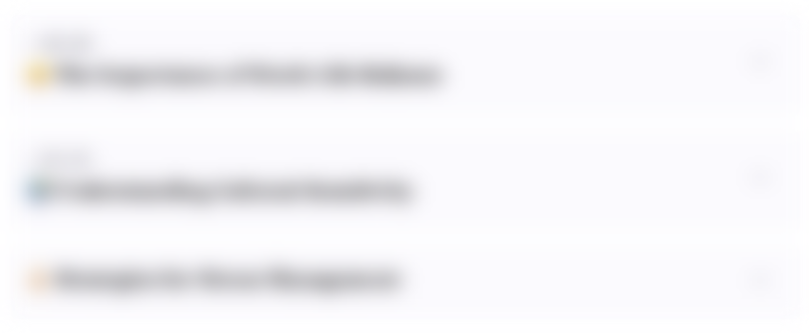
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
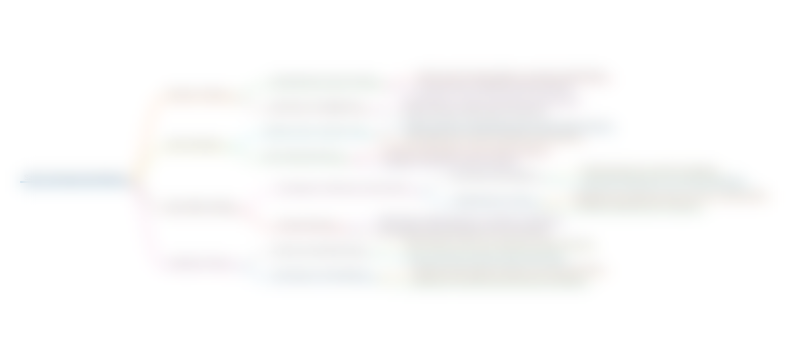
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
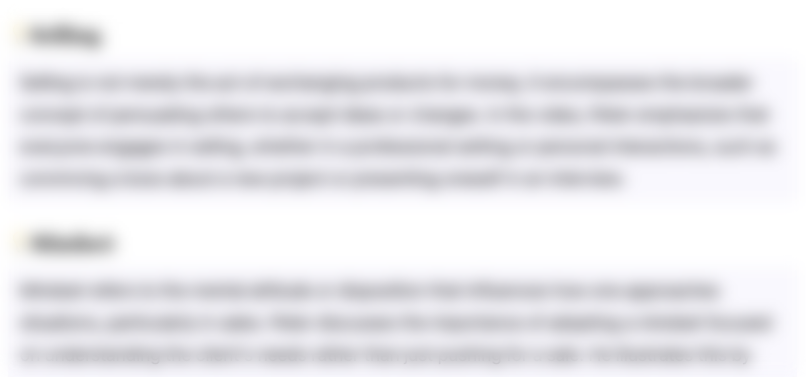
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
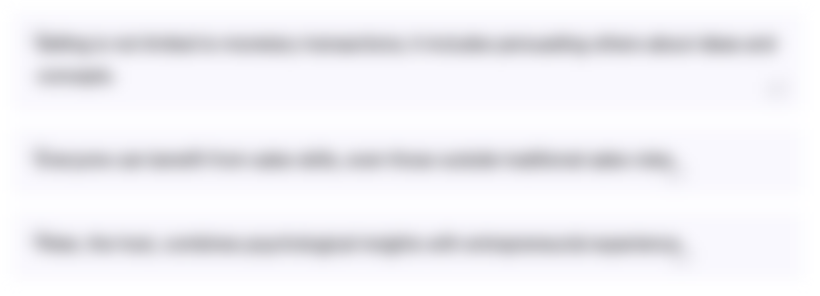
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
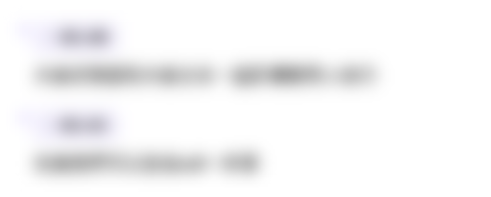
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Program Sederhana Aplikasi Toko Bunga Mengggunakan Bahasa Pemrograman C++ dengan DEV++
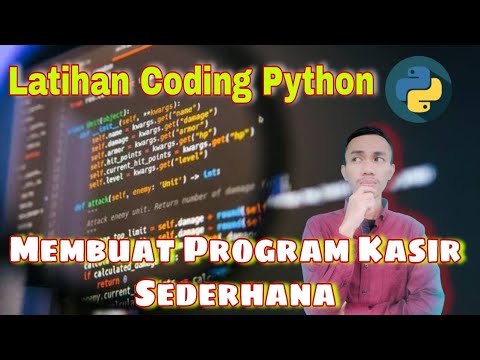
Membuat Program Kasir Sederhana di Python | Algoritma Kasir Menggunakan Python Program Diskon Python
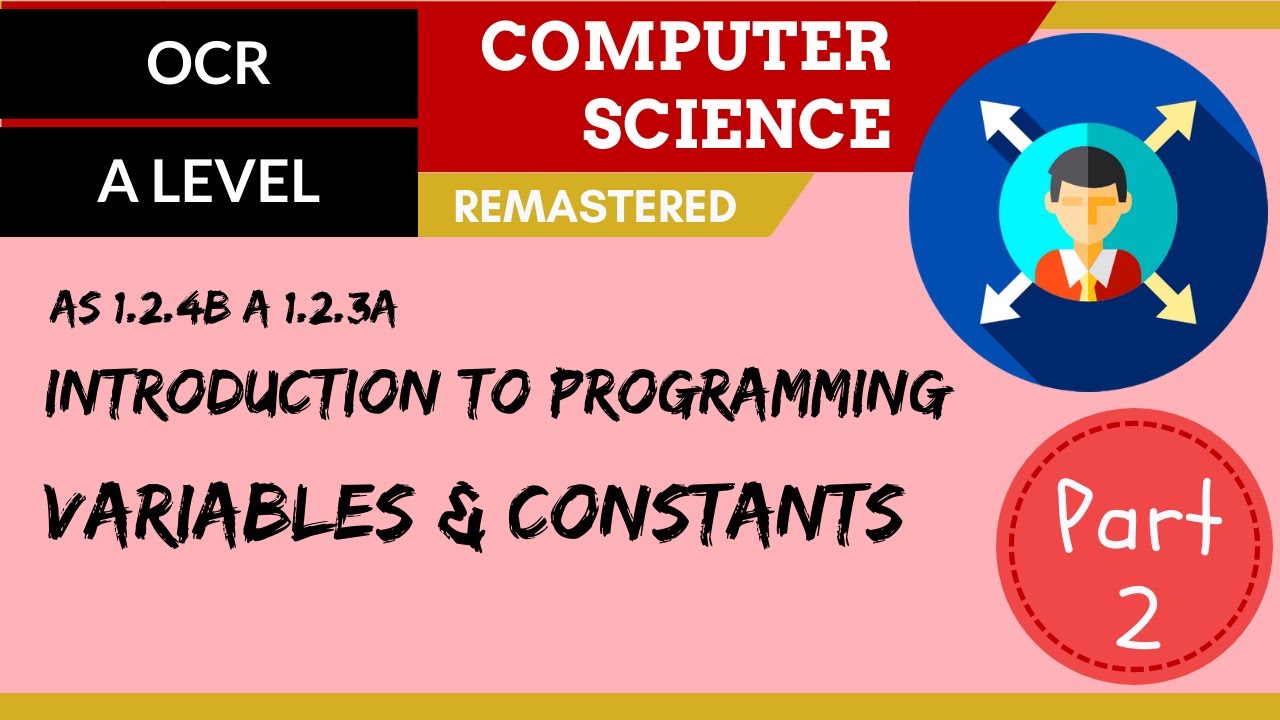
41. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 2 variables & constants
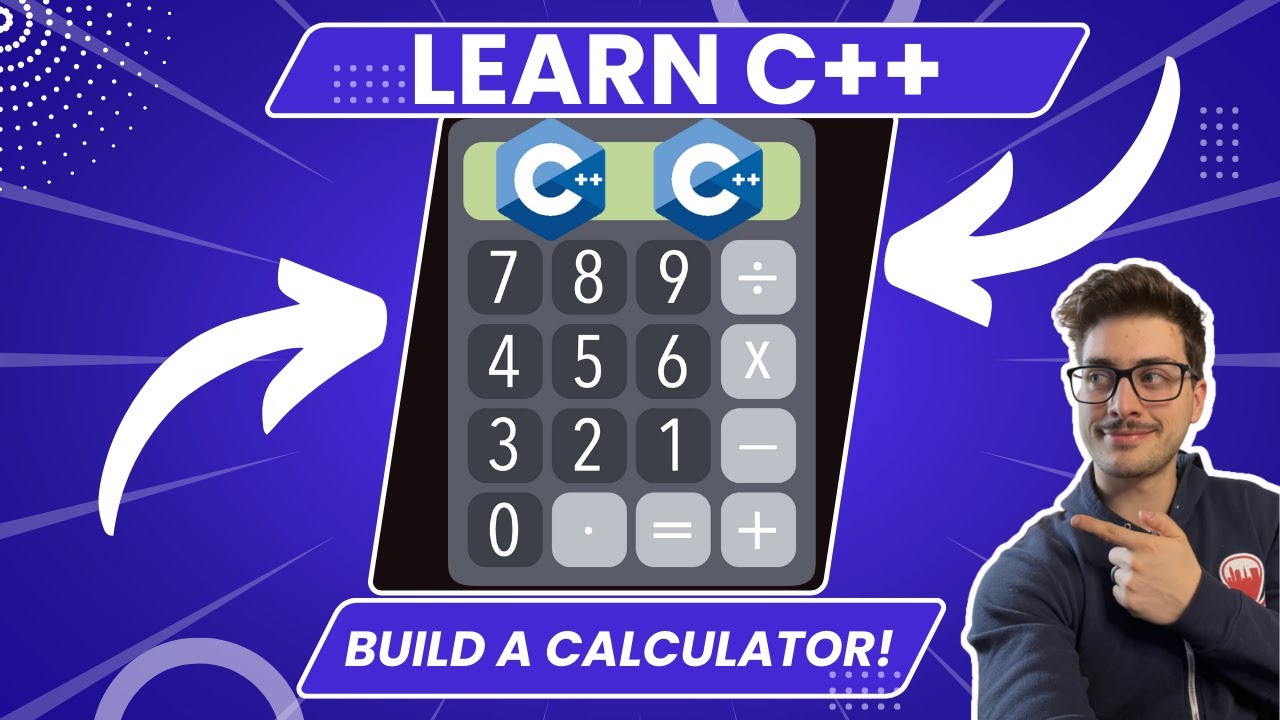
How to Program A Calculator in C++! (Great C++ Microsoft Visual Studio Beginner Project)
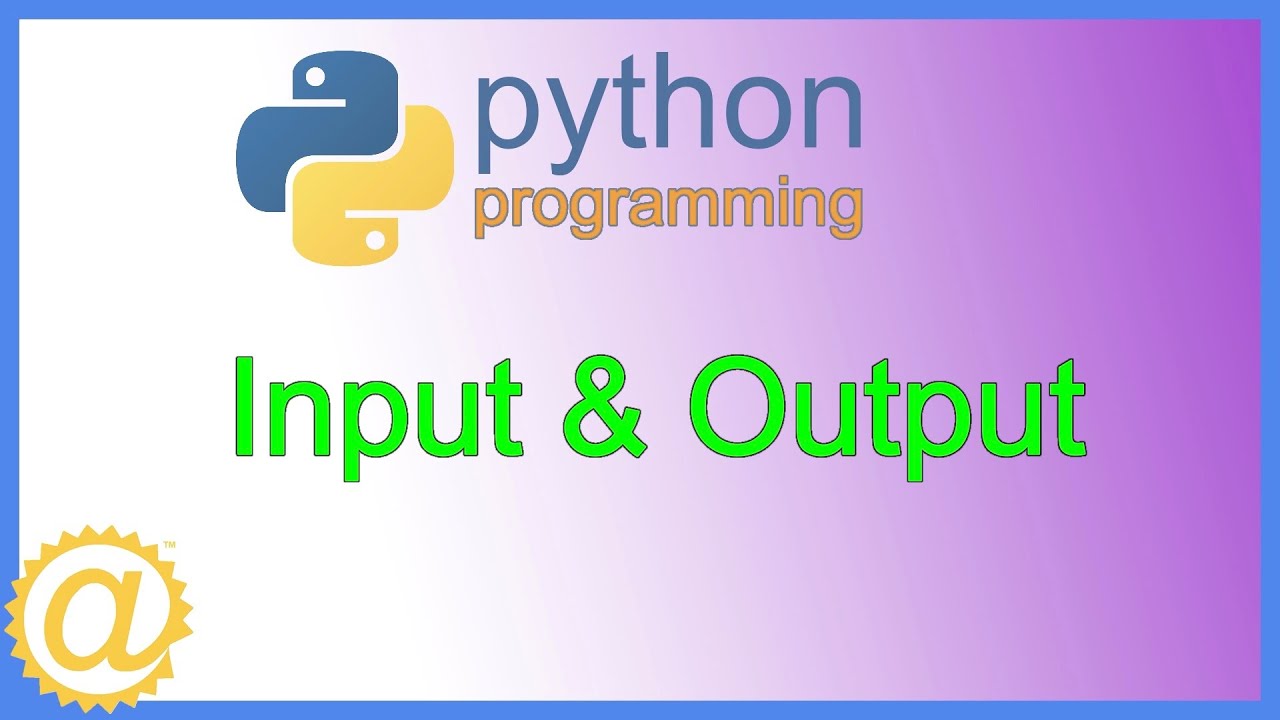
Python Programming - Basic Input and Output - print and input functions
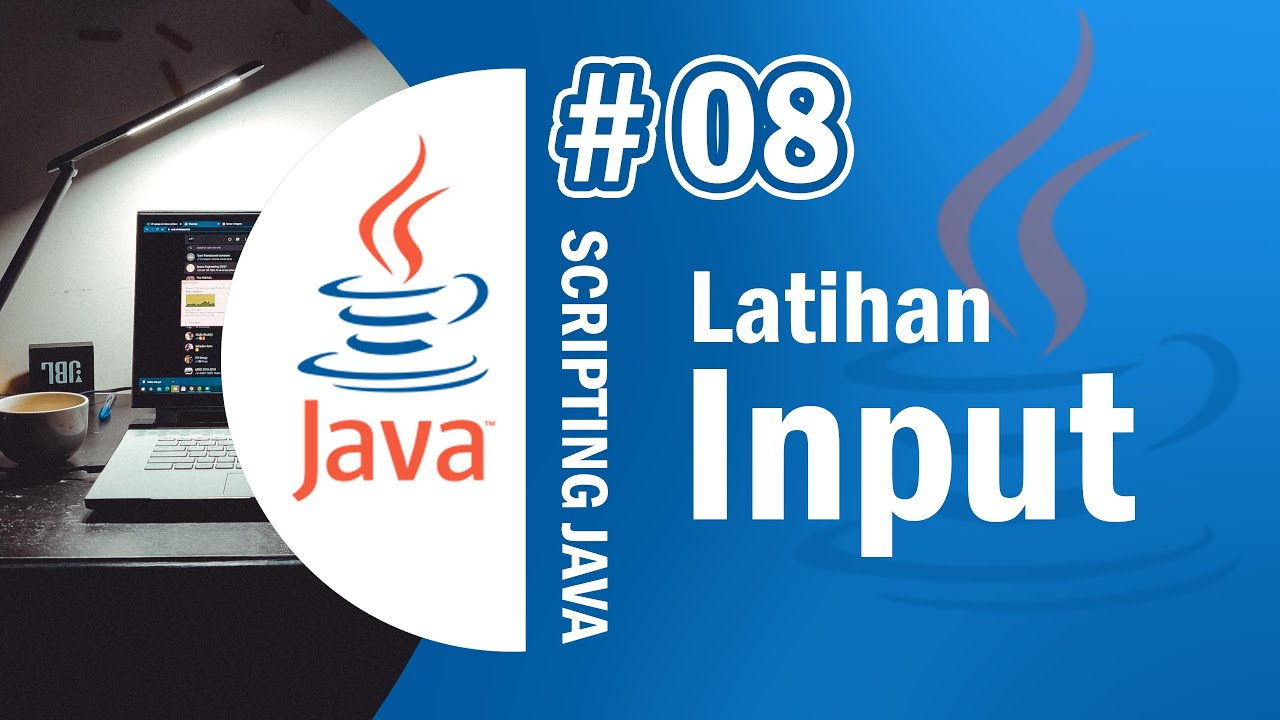
Java 08 - Latihan Input (Membuat Program Sederhana dengan Java) - Tutorial Java Netbeans Indonesia
5.0 / 5 (0 votes)