C++ programming call functions to input 2 numbers, calculate and display the sum and differences.
Summary
TLDRIn this tutorial, Professor Liu guides students through the process of improving a basic program that performs addition and subtraction on two numbers. The focus is on modularizing the program by creating reusable functions for input, calculation, and display. By passing data using pointers and employing a loop for user interaction, the program becomes more structured, clear, and maintainable. The session emphasizes the importance of modularity for reusability, scalability, and clarity in programming, encouraging students to create well-organized, functional code that can be easily modified or extended.
Takeaways
- 😀 Modularizing a program helps in organizing code, making it more readable and reusable.
- 😀 Passing variables by address (using pointers) allows changes in functions to reflect in the main program.
- 😀 Functions can be used to encapsulate logic, like input, calculation, and output, making the program more maintainable.
- 😀 The `void` type can be used for functions that do not return values but modify passed variables via references.
- 😀 Refactoring a program into functions reduces code duplication and enhances its clarity.
- 😀 Modularizing the code allows for easy extension, such as adding more operations or features later on.
- 😀 The `calculate` function computes both the sum and difference of two numbers, demonstrating how multiple values can be returned via reference.
- 😀 A loop can be added to allow users to perform calculations repeatedly, making the program more interactive.
- 😀 User input can be handled in a flexible way to prompt the user for repeated actions (e.g., asking whether they want to continue).
- 😀 Using `char` for user choice input (like 'y' or 'n') is an effective way to control the flow of repetitive actions in the program.
Q & A
What is the purpose of modularizing the program in this tutorial?
-Modularizing the program helps break it into smaller, reusable functions, making the code more maintainable, readable, and easier to debug. It also allows functions to be reused in other parts of the program or in different projects.
What does passing variables by reference mean in this context?
-Passing variables by reference means that the function receives the memory address of the variables, allowing the function to modify the original variables directly, rather than working with a copy of them.
Why is the 'void' return type used in some functions?
-The 'void' return type is used in functions when no value is returned. These functions perform actions like displaying results or modifying variables via reference parameters, without returning any value.
What is the advantage of using the 'getInput' function in the program?
-The 'getInput' function allows the program to handle user input in one place, making the code cleaner and more organized. By modularizing input, the program becomes easier to manage and extend.
How does the 'calculate' function work in this program?
-The 'calculate' function takes two input numbers, computes their sum and difference, and then returns these results via reference parameters, which allows the calling function to access the computed values.
What is the role of the 'display' function?
-The 'display' function is responsible for showing the results of the calculations (sum and difference) along with the input numbers. It organizes the output presentation, making the program output more structured and readable.
Why does the program use a loop to ask the user if they want to perform the operation again?
-The loop allows the program to repeat the process of input, calculation, and display as long as the user wants to continue. This is done through a 'do-while' loop that checks if the user input is 'y' (yes).
What happens when the user inputs 'y' or 'Y' when asked if they want to perform the operation again?
-If the user inputs 'y' or 'Y', the program will repeat the steps of asking for two numbers, calculating their sum and difference, and displaying the results. This continues until the user enters something other than 'y'.
What would happen if the 'do-while' loop did not check for 'y' or 'Y' to repeat the process?
-If the loop did not check for 'y' or 'Y', the program would run only once and not give the user the option to perform additional calculations, limiting the program's functionality.
How can the 'calculate' function be modified to return multiple values without using reference parameters?
-The 'calculate' function could be modified to return a structure or a tuple containing both the sum and difference. This way, instead of passing values by reference, the function would return an object containing both results.
Outlines
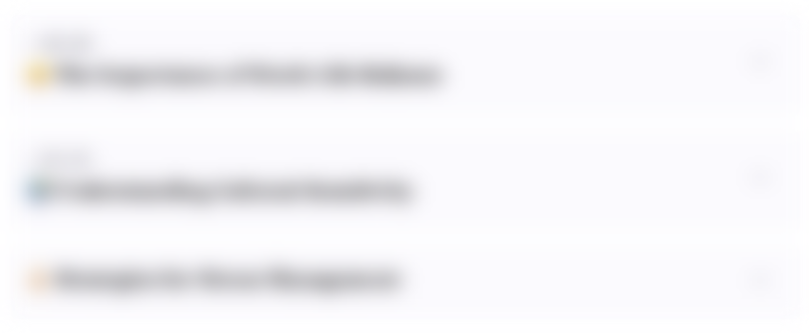
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
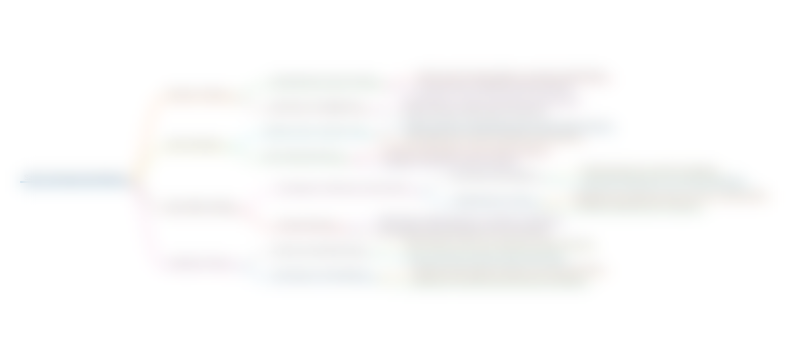
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
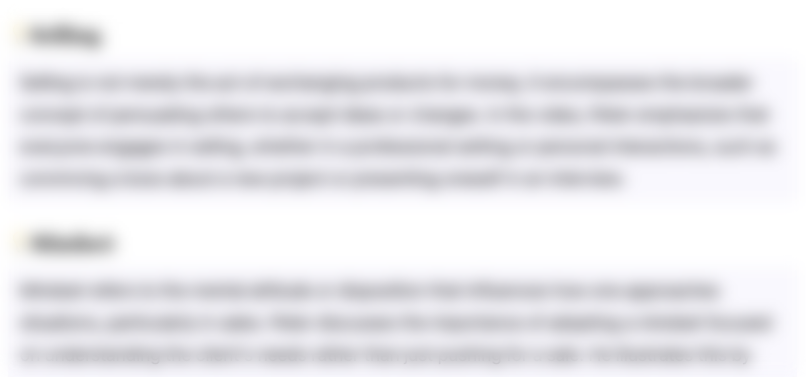
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
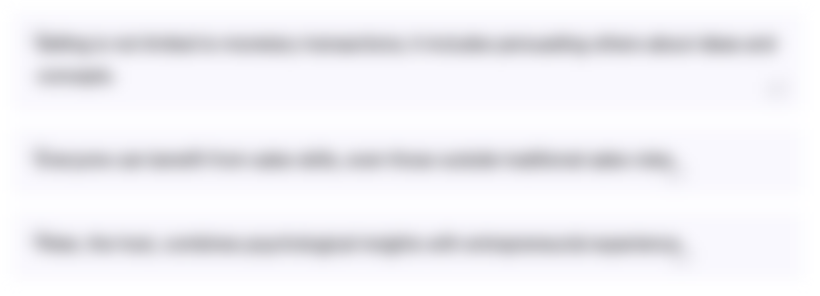
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
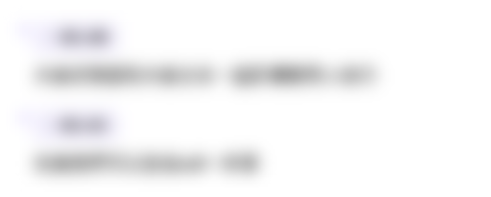
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
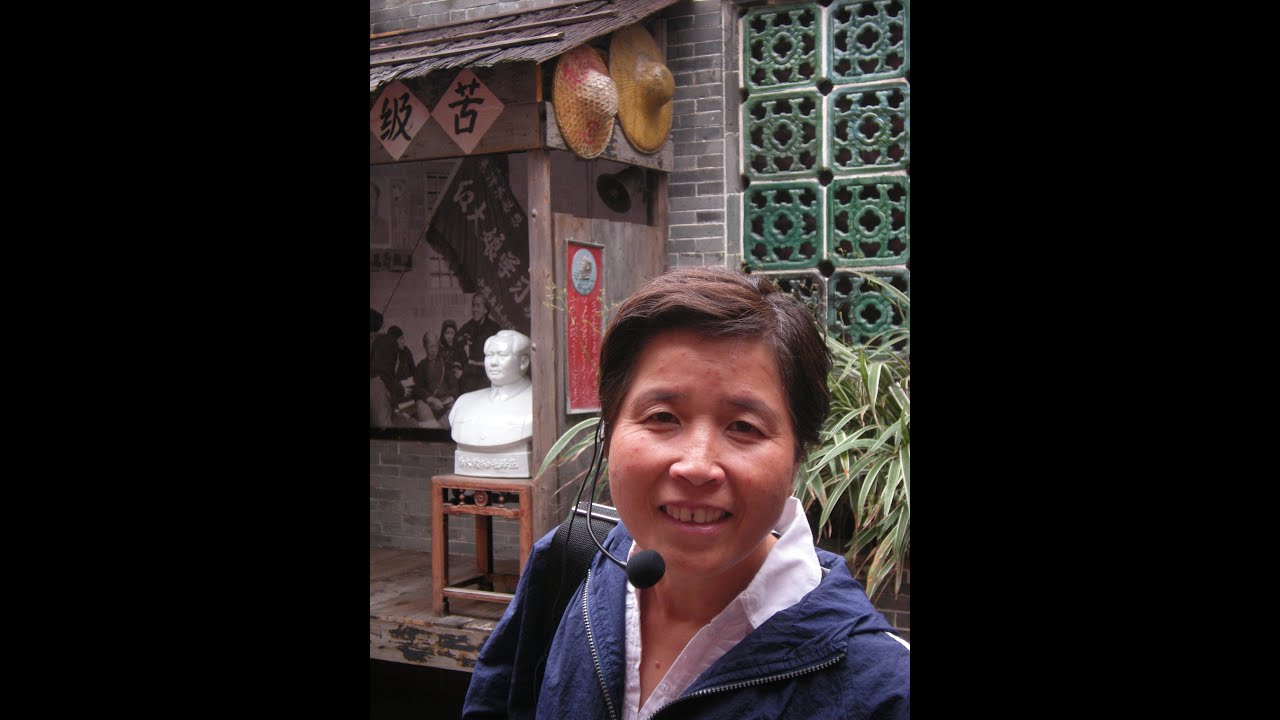
C++ programming, use a function to input 2 numbers, calculate and display exponent with functions
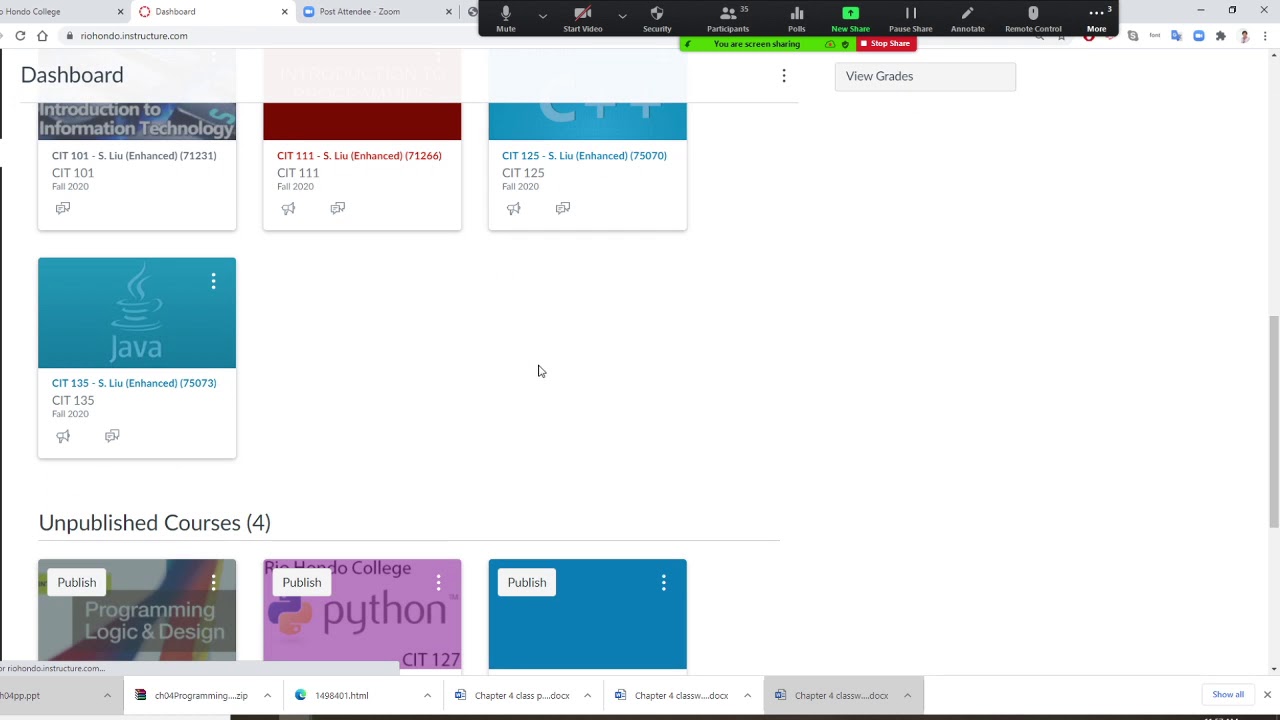
C++ Programming For loop Introduction, setw function
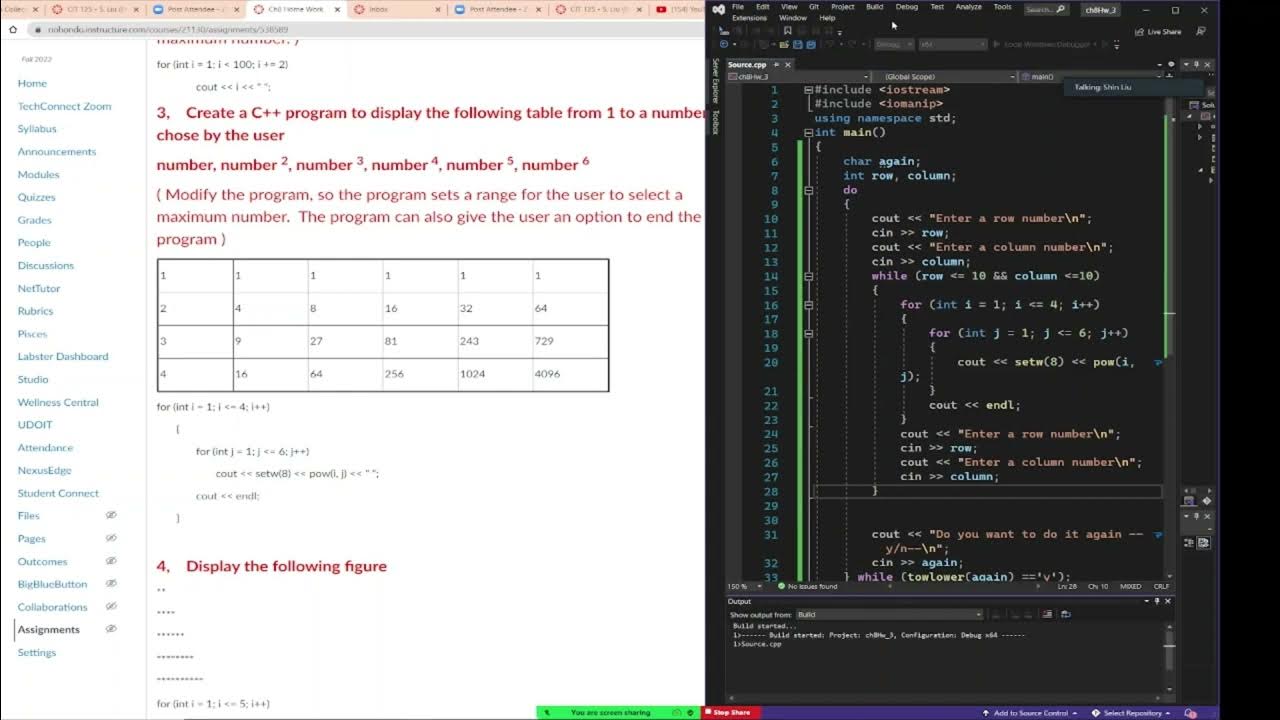
C++ programming, for loop to create a exponential table, do while loop to continue
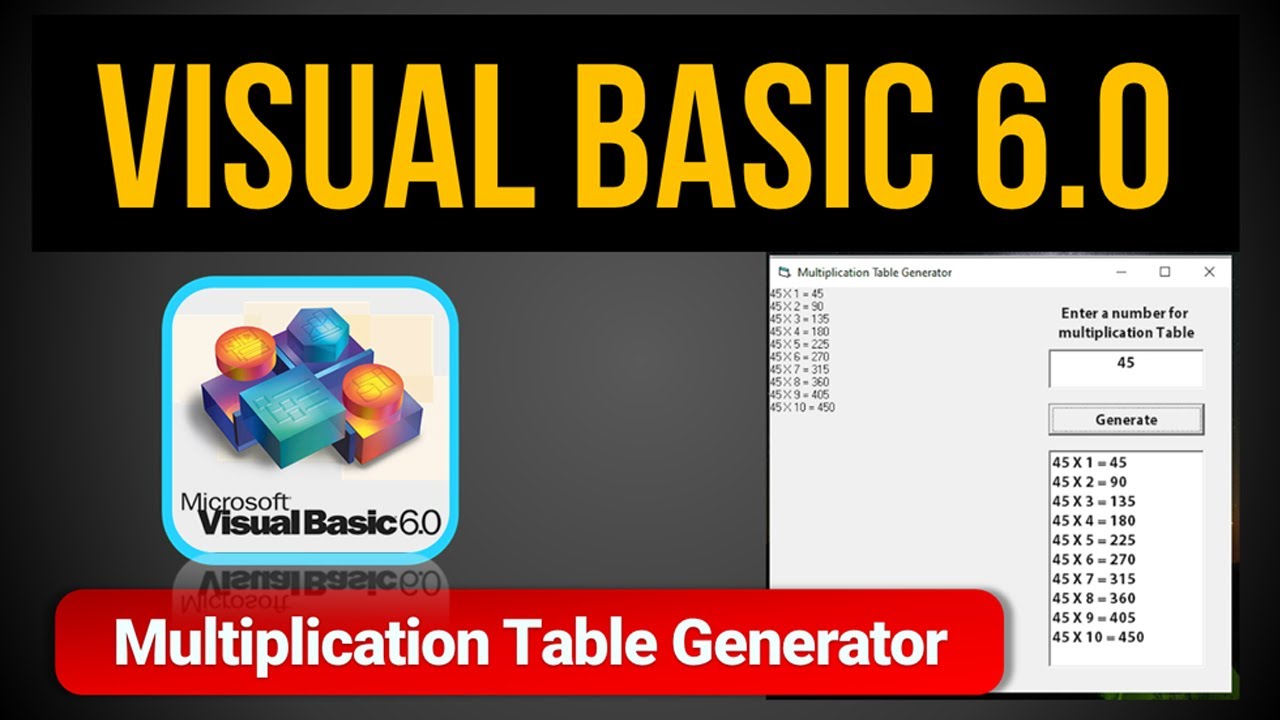
Visual Basic 6.0 | Multiplication Table Generator | For Loop
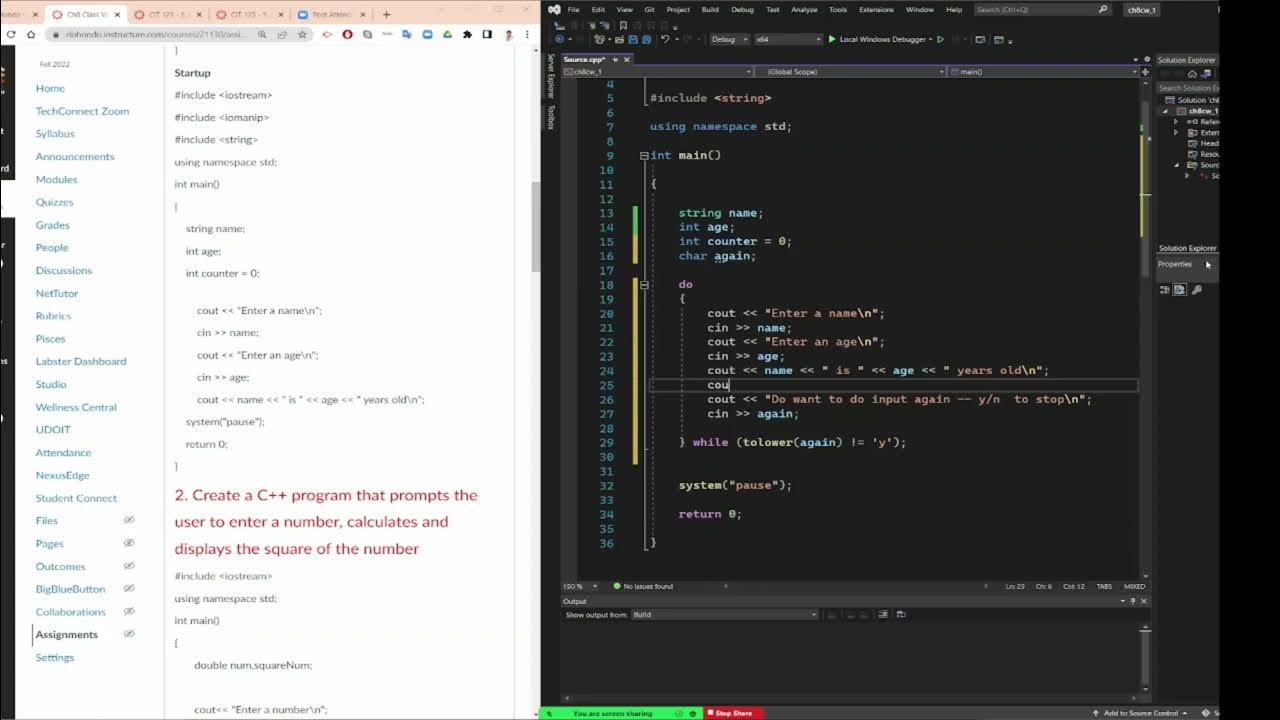
C++ programming , read a name and an age repeatedly with do while loop
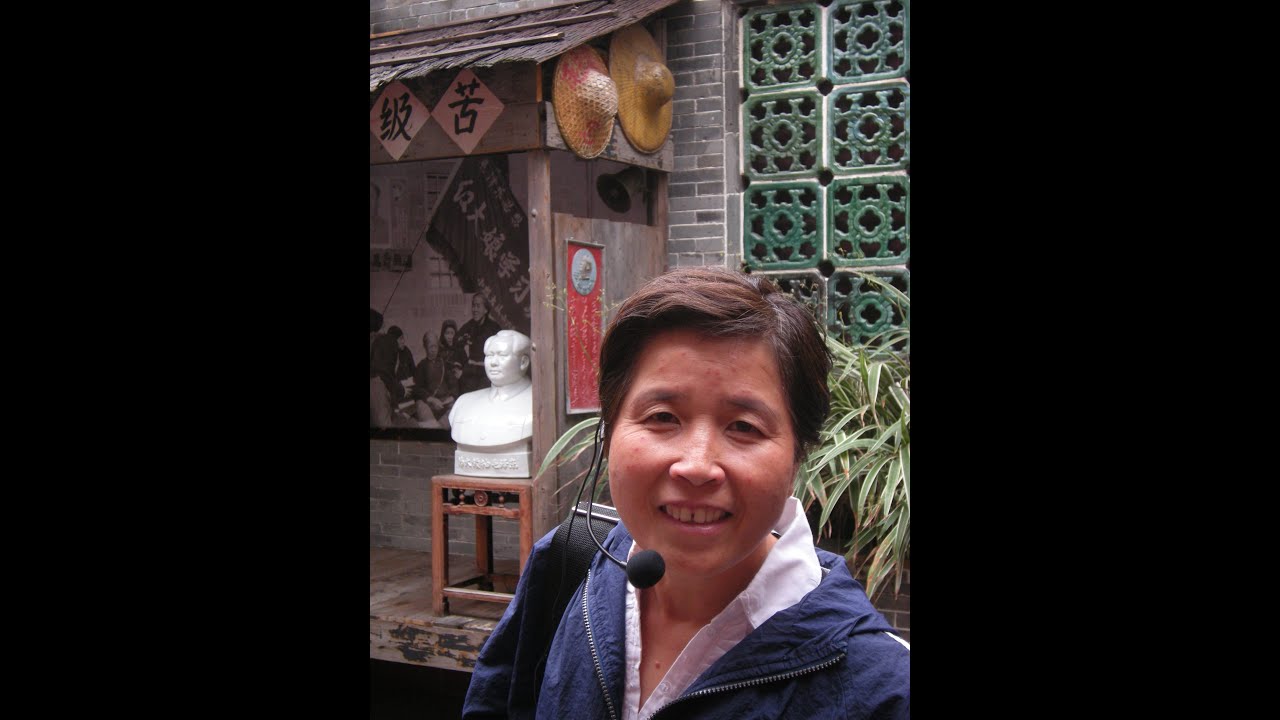
C++ programming to calculate area and perimeter, with functions.
5.0 / 5 (0 votes)