Raspberry Pi LED Blinking || Interfacing raspberry pi with basic peripherals || FIOT || CSE || JNTUH
Summary
TLDRIn this tutorial, you will learn how to blink an LED using a Raspberry Pi. The instructor walks through the process of setting up the Raspberry Pi, opening the Python IDE, and writing the necessary Python code to control the GPIO pins. The code includes importing libraries, setting pin modes, and writing logic to turn the LED on and off with a one-second delay. The lesson also covers hardware connections, including the use of a resistor to protect the LED. By the end of the tutorial, users will have a working LED blink circuit controlled by Python code on the Raspberry Pi.
Takeaways
- ๐ The lesson covers how to blink an LED using a Raspberry Pi.
- ๐ The Python IDE on Raspberry Pi is used to write the program for LED control.
- ๐ To begin, you need to import the necessary libraries: RPi.GPIO and time.
- ๐ The GPIO pin mode must be set to BOARD mode, which uses physical pin numbers.
- ๐ Pin 3 (physical pin 3) is configured as the output pin for the LED.
- ๐ A 1kฮฉ resistor is used to protect the LED from excessive current.
- ๐ The program repeatedly turns the LED on and off with a 1-second delay using a while loop.
- ๐ The GPIO.output() function controls the LED's state, with HIGH turning it on and LOW turning it off.
- ๐ The time.sleep(1) function is used to delay the LED state change for 1 second.
- ๐ Once the program is written, save the file and run it in the Python IDE to test the setup.
Q & A
What is the first step in blinking an LED using Raspberry Pi?
-The first step is to connect the Raspberry Pi and open the Raspberry Pi desktop. Then, open the Python IDE from the programming menu to start writing the code.
Which programming language is used to blink the LED in this tutorial?
-Python is used to write the code that controls the blinking of the LED on the Raspberry Pi.
How do you import the necessary libraries for controlling GPIO in the Python code?
-To control the GPIO pins, you import the RPi.GPIO library with the command: `import RPi.GPIO as GPIO`, and the time library using `import time`.
What does the 'GPIO.setmode(GPIO.BOARD)' function do?
-The `GPIO.setmode(GPIO.BOARD)` function sets the pin numbering system to BOARD mode, meaning it refers to the physical pin numbers on the Raspberry Pi's GPIO header.
What is the purpose of setting up a pin with 'GPIO.setup(3, GPIO.OUT)'?
-The `GPIO.setup(3, GPIO.OUT)` command configures pin 3 (physical pin 3 on the Raspberry Pi) as an output pin, which allows you to control it, such as turning an LED on or off.
What does the `while True` loop in the Python code do?
-The `while True` loop runs the code indefinitely, repeatedly turning the LED on and off with a delay, causing it to blink continuously.
How is the LED turned on and off in the program?
-The LED is turned on by setting the output of pin 3 to high with `GPIO.output(3, GPIO.HIGH)`, and turned off by setting it to low with `GPIO.output(3, GPIO.LOW)`.
Why is the `time.sleep(1)` function used in the code?
-The `time.sleep(1)` function adds a delay of 1 second between the changes in the LED's state, allowing the LED to stay on or off for a noticeable duration before switching again.
What hardware components are needed to blink the LED?
-You will need an LED, a 1 kฮฉ resistor to limit the current, jumper wires, and a Raspberry Pi to control the LED.
Why is a resistor used in the circuit with the LED?
-A resistor is used to limit the current flowing through the LED, preventing it from burning out by ensuring it receives the correct amount of power.
Outlines
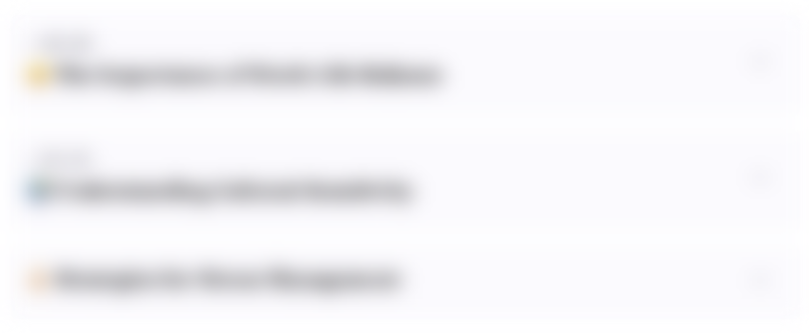
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
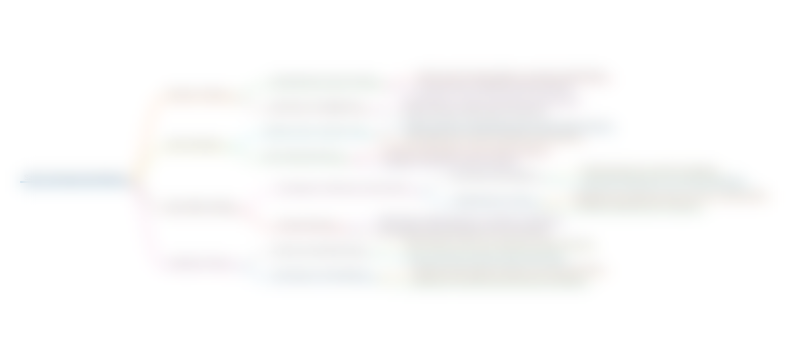
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
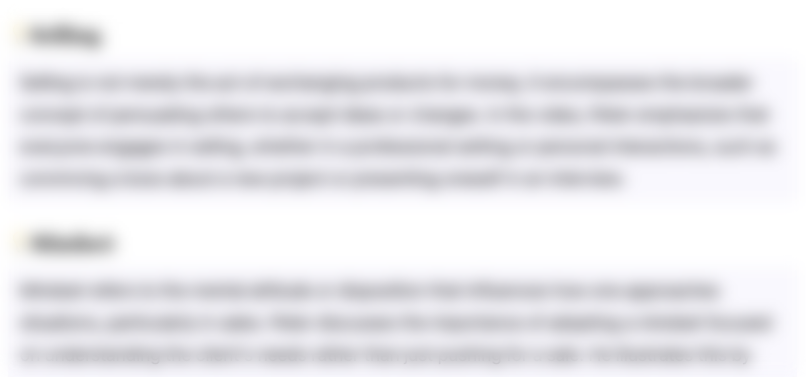
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
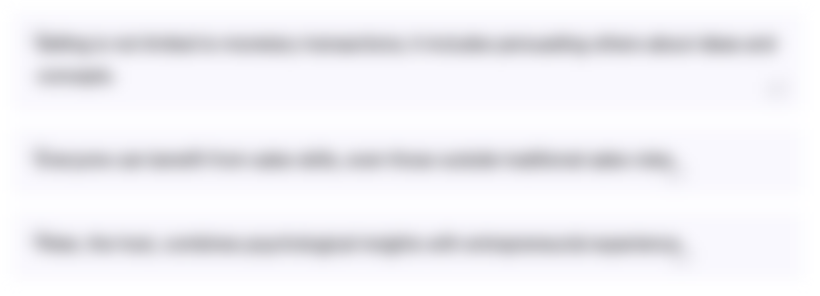
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
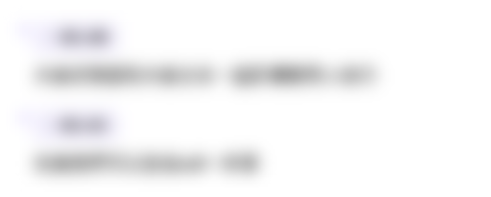
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Blinking LED Raspberry Pi Tutorial
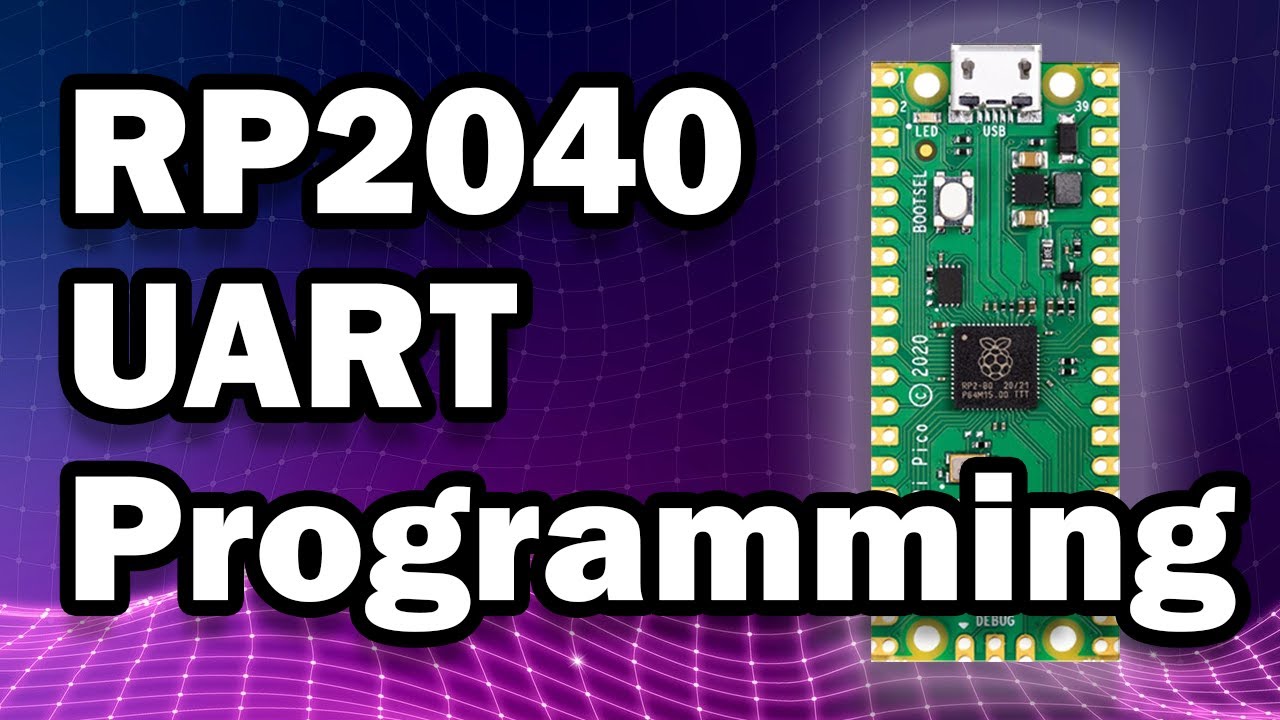
Talk to Your Pico Over Serial | Raspberry Pi Pico UART Tutorial
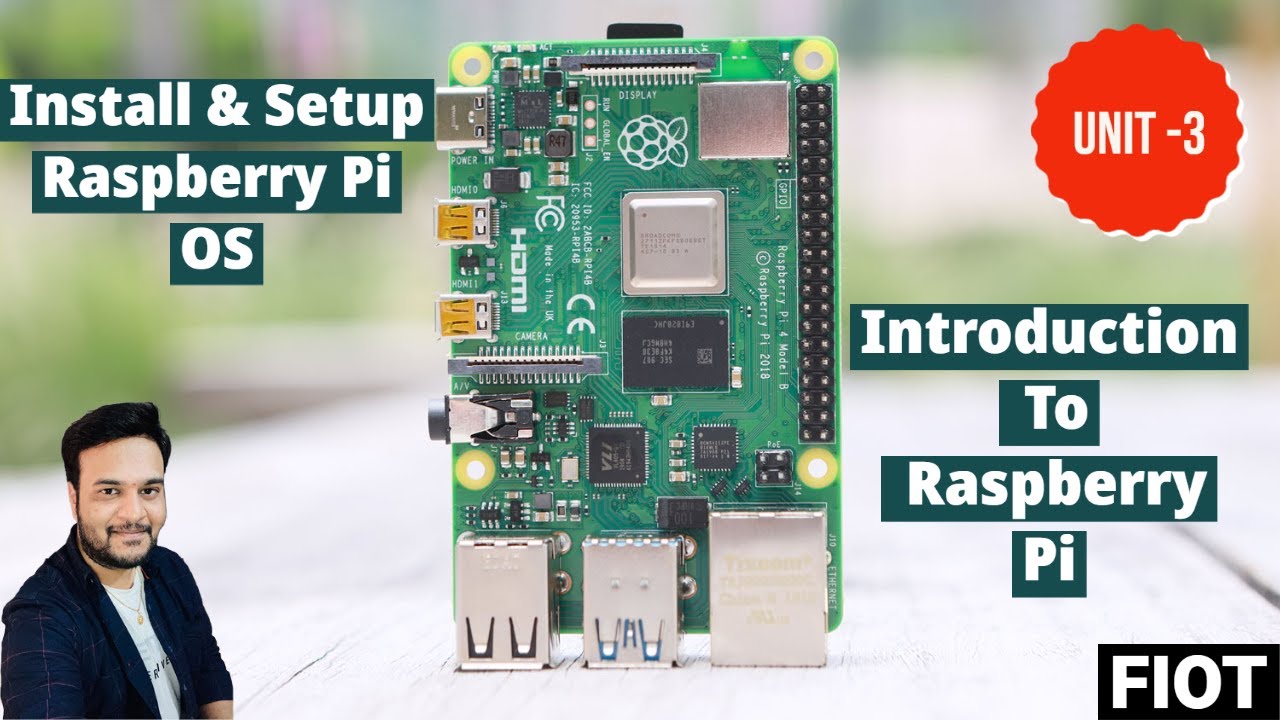
How To Install & Set Up Raspberry Pi OS || New Method to Setup Raspberry Pi (2023) || FIOT || CSE
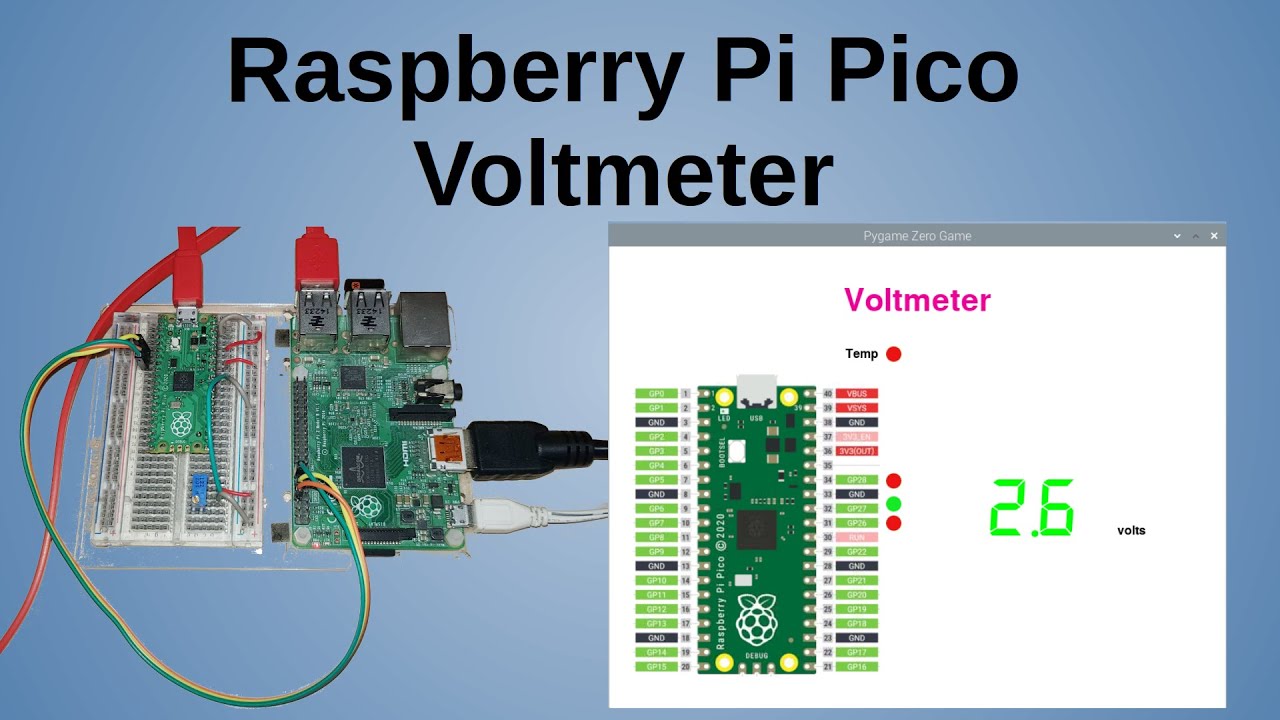
Raspberry Pi Pico Voltmeter - UART serial with the Pico and GUI application in C/C++ and MicroPython
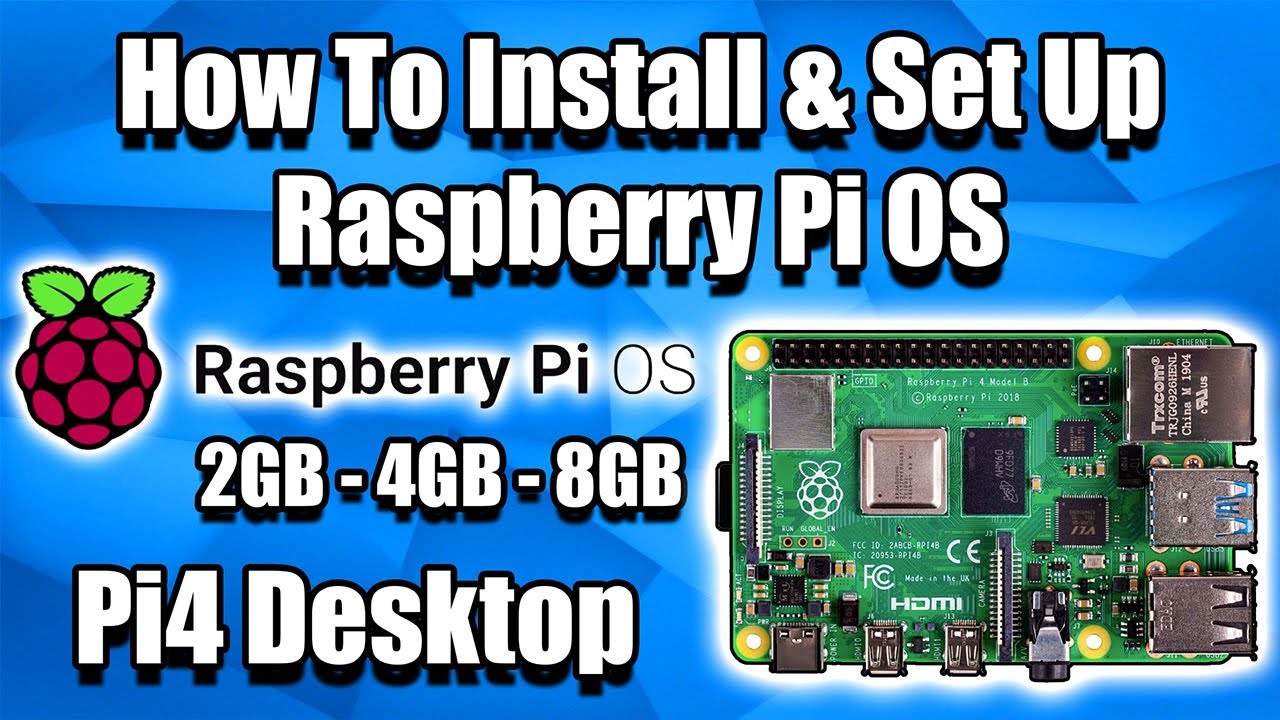
How To Install & Set Up Raspberry Pi OS - Pi4 Pi3 Pi2

Every Developer Needs a Raspberry Pi
5.0 / 5 (0 votes)