#04 First Node JS Project | Fundamentals of NODE JS | A Complete NODE JS Course
Summary
TLDRIn this tutorial, we learn how to set up a basic Node.js application using VS Code. The video walks through the process of writing a simple JavaScript program that outputs text to the terminal using `console.log()`. It also covers handling user input with the `readline` module, allowing users to enter data in the terminal, and demonstrates how to close the input interface properly. The tutorial further explains event handling when the interface is closed. By the end, users will understand how to handle both output and input in Node.js applications.
Takeaways
- 😀 Node.js allows you to execute JavaScript code outside the browser in a server environment.
- 😀 To create a Node.js application, open VS Code, create a new project folder, and add a JavaScript file (e.g., app.js).
- 😀 To print output to the terminal in Node.js, use the `console.log()` method.
- 😀 Always save your file before running it using the `node` command to ensure your changes are executed.
- 😀 Node.js does not have a built-in input function, but the `readline` module is available to handle user input from the terminal.
- 😀 The `readline.createInterface()` method creates an interface for reading input and output to the terminal, where you specify `process.stdin` for input and `process.stdout` for output.
- 😀 The `question()` method of the `readline` interface prompts the user for input and handles the input through a callback function.
- 😀 After receiving input, close the interface with the `rl.close()` method to cleanly exit the input loop.
- 😀 You can listen to the `close` event on the readline interface using `rl.on('close', callback)` to perform actions when the interface is closed.
- 😀 The process can be exited programmatically using `process.exit(0)` after closing the readline interface, indicating successful completion.
- 😀 This tutorial demonstrates a simple interactive Node.js application that prompts the user for input, displays the input, and closes the input interface.
Q & A
What is the purpose of this tutorial?
-The tutorial demonstrates how to set up a Node.js environment, create a basic Node.js application, output messages to the console, and read user input from the terminal.
How do you create a new file in VS Code for a Node.js project?
-To create a new file in VS Code for a Node.js project, you can open a folder (e.g., `node.js Basics`), right-click, and choose to create a new file. The file should have a `.js` extension, such as `app.js`.
How do you execute a Node.js file from the terminal in VS Code?
-To execute a Node.js file, you open the terminal in VS Code and use the `node` command followed by the filename. For example: `node app.js`.
What happens if you try to run a Node.js file without saving the changes?
-If you try to run a Node.js file without saving the changes, only the last saved version of the file will be executed. Any unsaved changes will not be reflected in the output.
How do you print a message to the terminal in Node.js?
-In Node.js, you can print a message to the terminal using the `console.log()` function. For example, `console.log('Hello from Node.js');` will print the message 'Hello from Node.js' to the terminal.
What is the `readline` module in Node.js, and why is it used?
-The `readline` module in Node.js is used to handle reading input from the terminal and writing output to the terminal. It provides an interface for reading user input interactively.
How do you read user input in a Node.js application?
-To read user input in Node.js, you use the `readline` module. You create an interface using `readline.createInterface()`, then prompt the user with `question()` to collect input.
What does the `question()` method of the `readline` module do?
-The `question()` method of the `readline` module displays a prompt message to the user and waits for their input. Once the user enters a response, it triggers a callback function with the entered value.
How do you close the readline interface after receiving input?
-After receiving user input, you can close the readline interface by calling `rl.close()`. This will terminate the input session.
How do you handle events in the `readline` module, such as the interface closing?
-To handle events in the `readline` module, such as the interface closing, you use the `on()` method to listen for specific events like 'close'. You can define a callback function that runs when the event is triggered.
Outlines
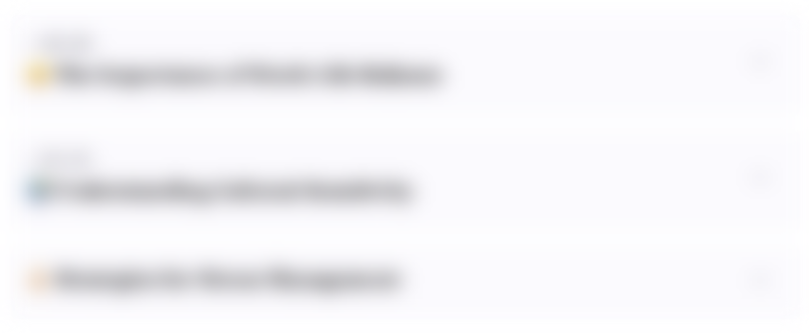
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
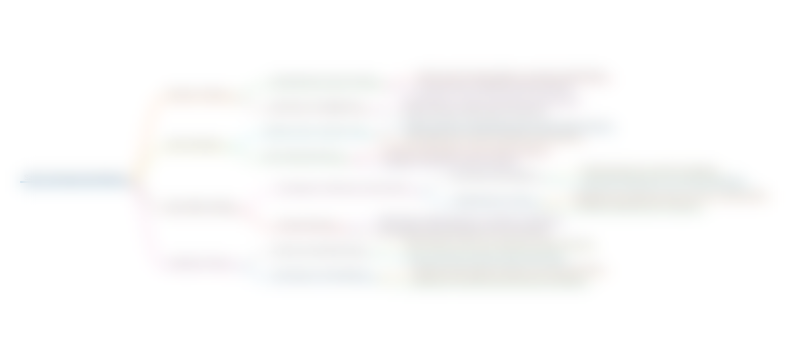
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
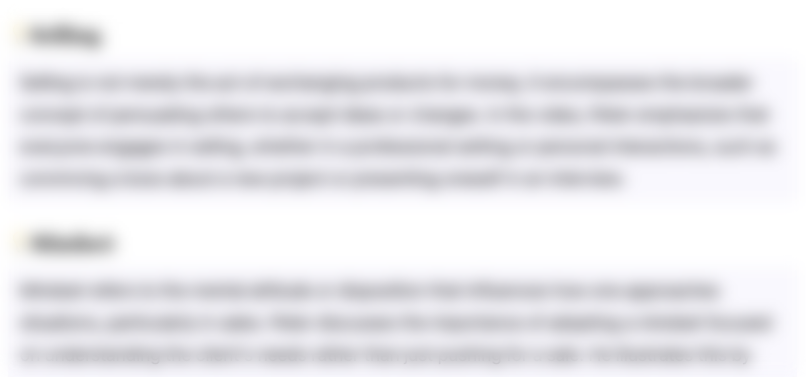
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
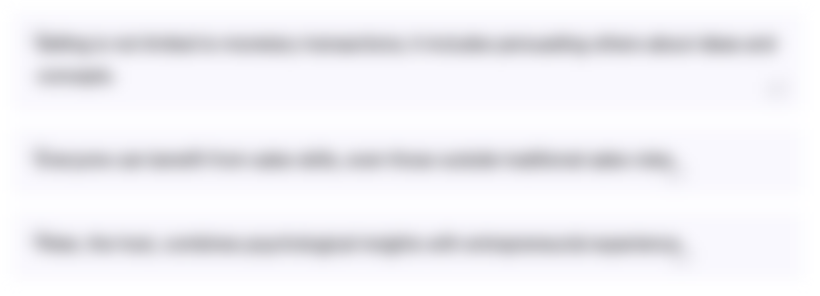
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
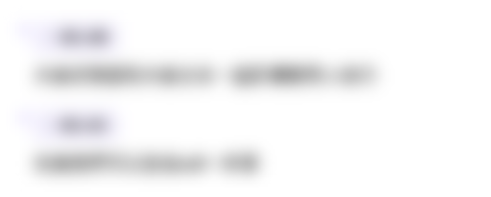
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)