Praktikum PBO - MVC pada proyek winform .NET C#
Summary
TLDRThis tutorial demonstrates how to implement the Model-View-Controller (MVC) architecture in a C# WinForms application, using PostgreSQL for database interaction. It covers how to structure a project with MVC, including setting up folders for Views, Controllers, and Models. The tutorial provides step-by-step guidance on creating and managing CRUD operations (Create, Read, Update, Delete) for student and program data, demonstrating how to connect and query the PostgreSQL database. The lesson is aimed at helping students organize their projects, enhance code modularity, and streamline database management with MVC.
Takeaways
- 😀 **MVC Architecture**: The script explains how to implement the Model-View-Controller (MVC) architecture in a C# WinForms project.
- 😀 **Folder Structure**: Organize your project into three main folders—`Views`, `Models`, and `Controllers`—to maintain clean separation of concerns.
- 😀 **Model Definition**: Models represent your data and map to database tables. For example, `Mahasiswa` (student) and `Prodi` (program) are models in the tutorial.
- 😀 **View Creation**: The View is where users interact with the app. In this case, it's created using WinForms, and includes forms for adding, editing, and deleting data.
- 😀 **Controller Functionality**: The Controller handles business logic, such as performing CRUD operations (Create, Read, Update, Delete) on the database.
- 😀 **Database Interaction**: The tutorial uses **PostgreSQL** for database operations. It teaches how to create tables and perform SQL queries.
- 😀 **CRUD Operations**: CRUD operations are explained in detail, such as adding, editing, and deleting student records in the `Mahasiswa` table.
- 😀 **Helper Methods**: The script emphasizes creating helper methods (e.g., `DatabaseWrapper`) to manage database connections and queries more efficiently.
- 😀 **Avoiding Redundant Code**: Helper functions simplify code by reducing the need to repeat tasks like opening and closing database connections.
- 😀 **Parameterized Queries for Security**: To prevent SQL injection, the tutorial suggests using parameterized queries, which are implemented using `NpgsqlParameter`.
- 😀 **MVC Best Practices**: The video stresses following MVC best practices like not mixing database queries in the View, and ensuring the Controller handles all database interactions.
Q & A
What is the Model-View-Controller (MVC) design pattern, and why is it useful in WinForms applications?
-The MVC design pattern separates an application into three main components: the Model, View, and Controller. This separation allows for easier management and scaling of applications, as well as clearer organization of code. In WinForms applications, MVC helps to separate UI logic (View), data manipulation (Model), and user interaction logic (Controller), making the code more maintainable and testable.
What is the role of the Model in the MVC architecture?
-In the MVC pattern, the Model represents the data and the business logic of the application. It is responsible for retrieving, storing, and processing data. The Model interacts with the database and is updated based on user input from the View, or by the Controller's instructions.
How does the Controller interact with the Model and View in MVC?
-The Controller acts as an intermediary between the Model and the View. It listens to user input (typically from the View), processes that input (often involving changes to the Model), and updates the View accordingly. The Controller receives data from the Model and passes it to the View to be displayed to the user.
Why is it important to separate the database logic into a separate class (e.g., DatabaseWrapper)?
-Separating database logic into a class like DatabaseWrapper provides better code organization, reusability, and maintainability. It abstracts the database interactions from other parts of the application, making it easier to manage connections, queries, and transactions. It also simplifies testing and modifications to database logic without affecting the rest of the code.
What is the significance of using Npgsql for PostgreSQL in this MVC setup?
-Npgsql is a .NET data provider for PostgreSQL, which allows easy integration of PostgreSQL with .NET applications. It provides classes and methods to connect to and interact with PostgreSQL databases, allowing the application to perform operations like executing queries and retrieving results. In this setup, Npgsql is used to communicate with the PostgreSQL database within the Model and DatabaseWrapper.
What does the `DatabaseWrapper` class do, and why is it necessary?
-The `DatabaseWrapper` class encapsulates the logic required to interact with the database, such as opening connections, executing SQL queries, and handling transactions. It centralizes database-related code, ensuring that the application logic is separated from the database interactions, which enhances maintainability and scalability.
How does the `StudentContext` class manage database operations in the MVC setup?
-The `StudentContext` class interacts with the database through the `DatabaseWrapper` class. It contains methods that execute SQL queries to perform CRUD (Create, Read, Update, Delete) operations on student data. For example, the `AddStudent()` method constructs an SQL query to insert a new student's data into the database.
What is the purpose of the `StudentModel` class in the MVC structure?
-The `StudentModel` class defines the data structure for a student in the application, including properties such as `Name`, `Email`, `Semester`, and `ProgramId`. It serves as a representation of the data that the application works with and is used by the Controller to manage the application's business logic.
How does the `MainForm` View interact with the Controller in this MVC application?
-In this application, the `MainForm` (View) contains user interface elements like text boxes and buttons. When a user interacts with the UI, such as clicking the 'Add Student' button, the `MainForm` triggers a corresponding event (like `btnAddStudent_Click`) in the Controller. The Controller then processes the input and updates the Model (e.g., adding a student to the database).
Why is it important to use parameterized queries (e.g., `@Name`, `@Email`) in database operations?
-Parameterized queries prevent SQL injection attacks by separating SQL logic from the data being supplied by the user. This ensures that user input is treated as data rather than executable code, improving security. Additionally, parameterized queries can improve performance by allowing the database to cache query execution plans.
Outlines
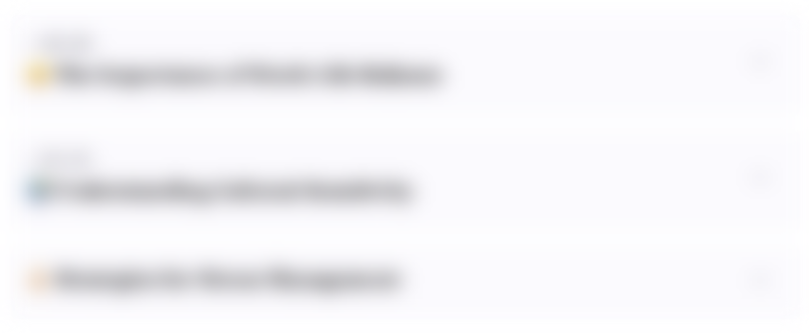
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
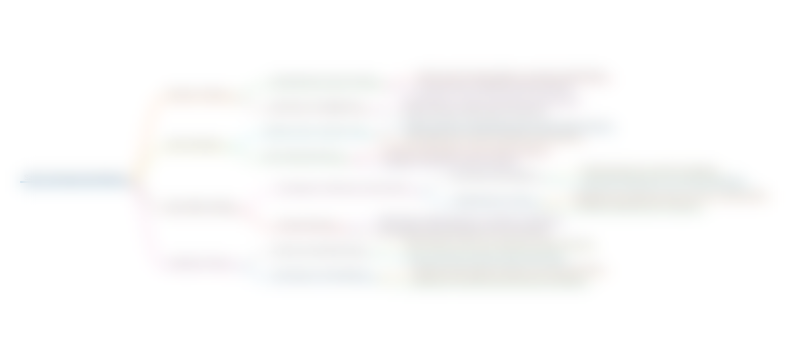
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
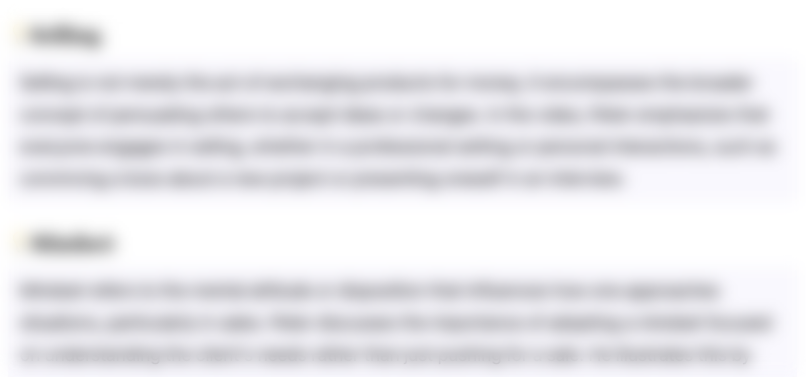
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
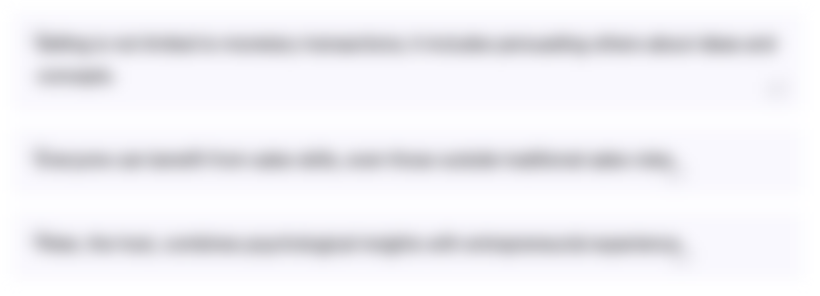
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
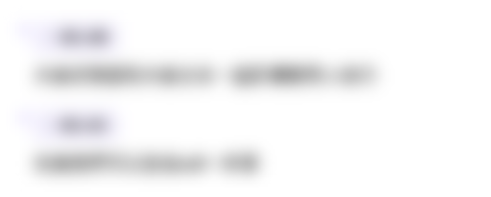
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
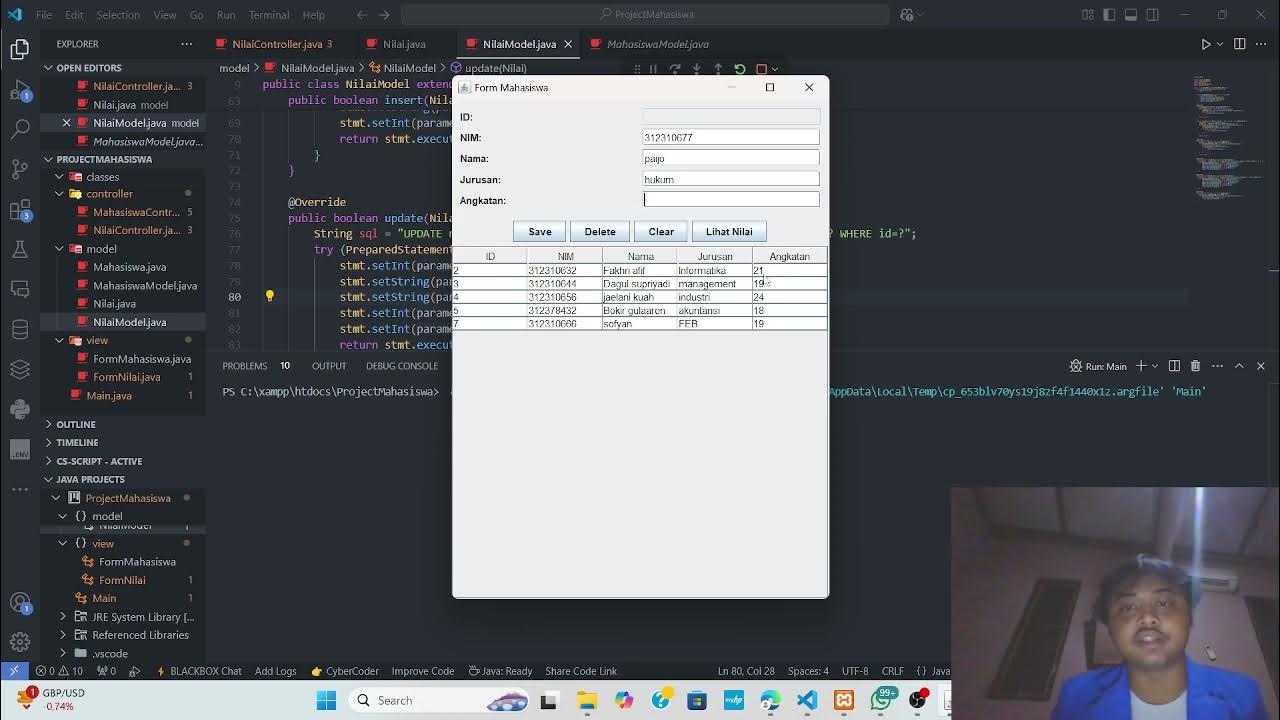
Langkah-Langkah Membuat Aplikasi CRUD Mahasiswa Berbasis MVC dengan Java
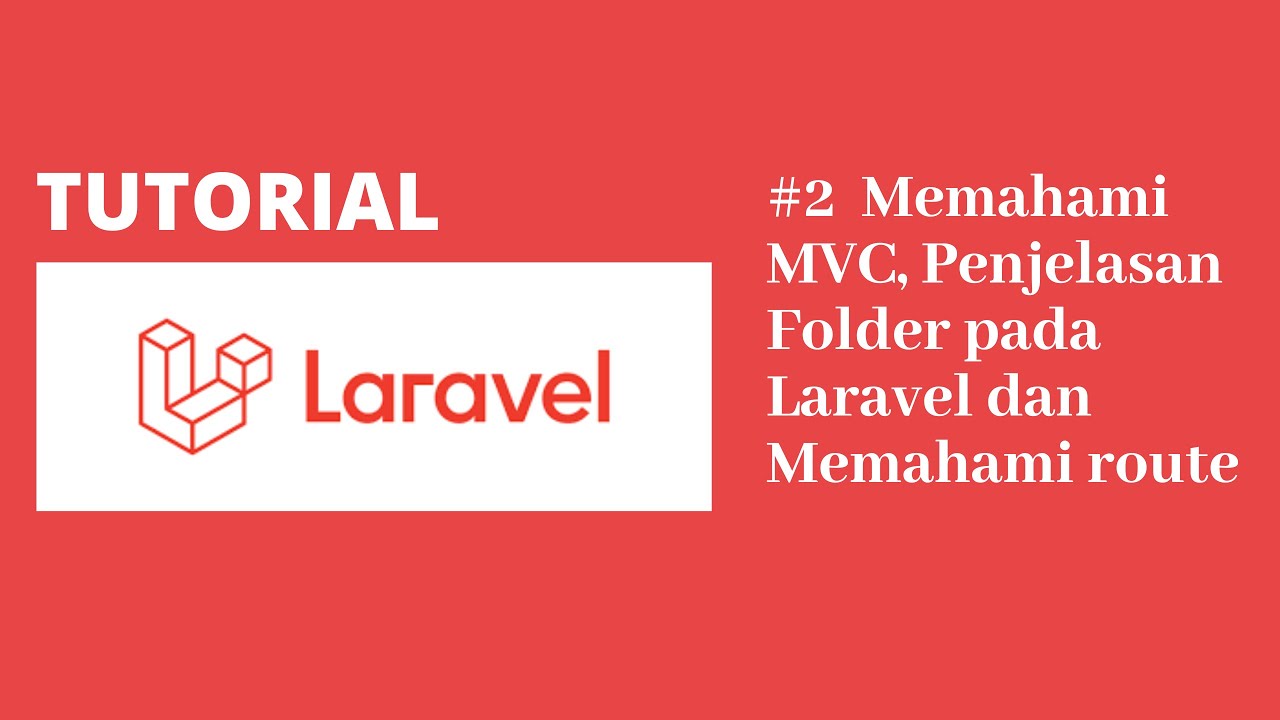
Tutorial Laravel 8 #2 - MVC, Penjelasan Stuktur Folder Project dan Memahami Route
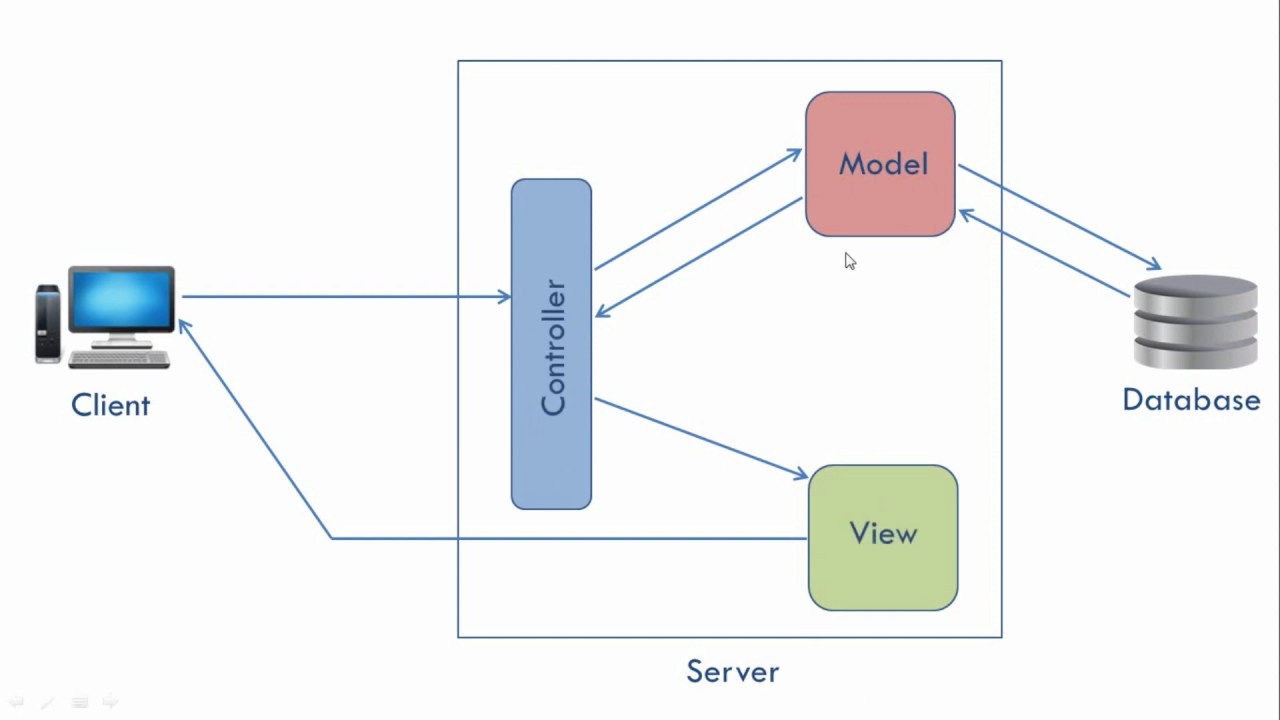
What is MVC architecture?
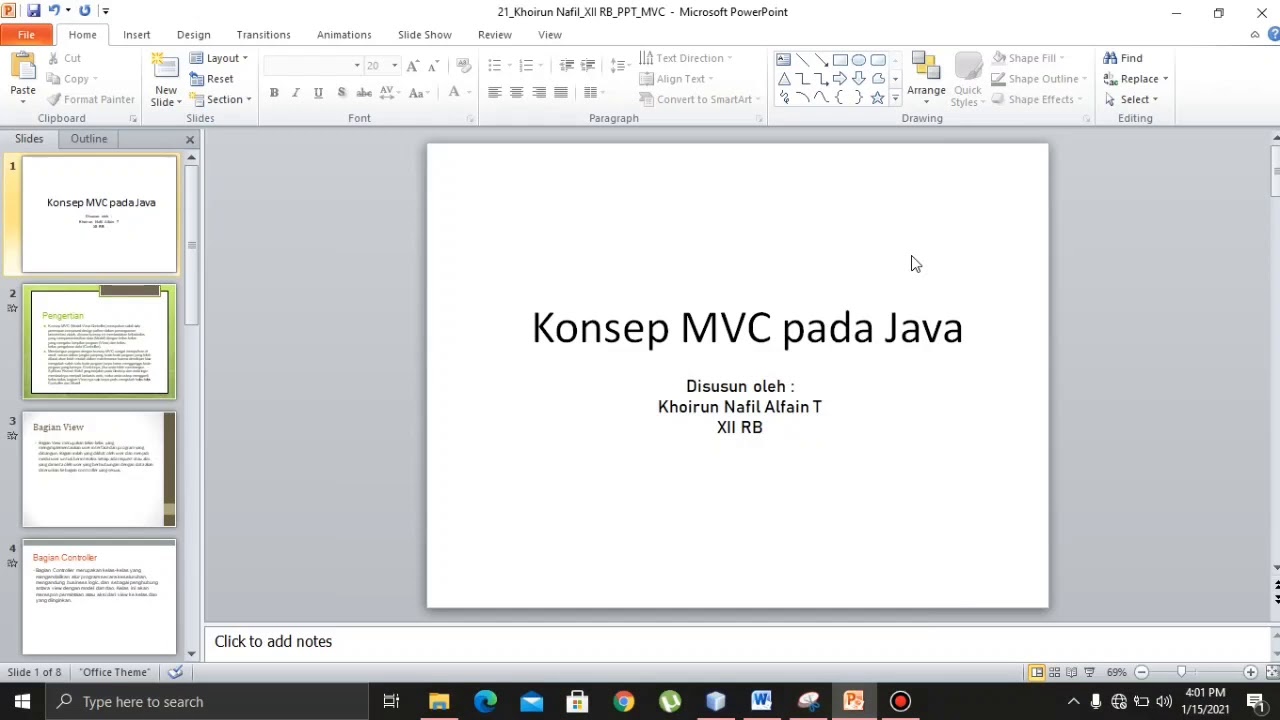
Konsep MVC Pada Java || Presentasi & Program

How to Update (CRUD) | Laravel 10 Tutorial #16
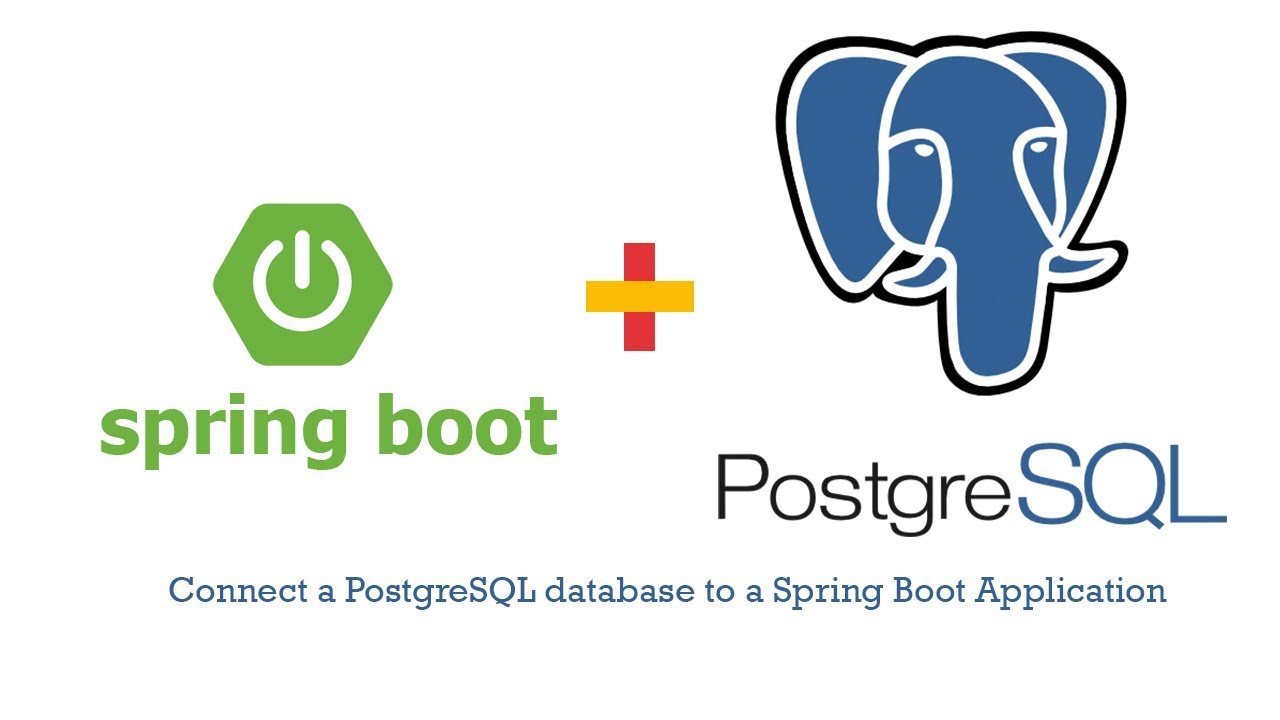
Connect a PostgreSQL database to a Spring Boot Application Tutorial
5.0 / 5 (0 votes)