Filtering Columns and Rows in Pandas | Python Pandas Tutorials
Summary
TLDRIn this comprehensive tutorial on filtering and ordering data frames in Pandas, the presenter demonstrates various techniques to manipulate data effectively. Key methods include filtering based on column values using comparison operators, employing `isin()` for specific entries, and utilizing `str.contains()` for substring searches. The video also explores index-based filtering with `filter()`, `loc`, and `iloc`. Additionally, it covers sorting data frames using `sort_values()` for single and multiple columns, showcasing both ascending and descending order options. Overall, the tutorial equips viewers with essential skills for efficient data analysis in Pandas.
Takeaways
- 😀 Import Pandas and load datasets using 'pd.read_csv' to begin data analysis.
- 📊 Filtering data can be performed using comparison operators, such as '<' or '>' to refine results.
- 🌍 Specific entries can be filtered using the 'isin()' function to include only certain values from a column.
- 🔍 The 'str.contains()' method allows for filtering based on substring matches within string columns.
- 🏷️ Setting an index with 'set_index()' helps streamline data access and filtering based on index labels.
- 📋 The 'filter()' function in Pandas can be used to select specific columns or rows based on conditions.
- 📈 Accessing data can be done via 'loc' for label-based indexing and 'iloc' for position-based indexing.
- 🔄 Sorting data can be achieved using 'sort_values()' to arrange DataFrame entries by specified columns.
- 🔢 Sorting can be done in both ascending and descending order by setting the 'ascending' parameter.
- ⚙️ Multiple columns can be sorted simultaneously, allowing for complex data organization and insights.
Q & A
What is the primary focus of the video?
-The video focuses on filtering and ordering data within DataFrames using the Pandas library in Python.
How do you import a dataset into a Pandas DataFrame?
-You can import a dataset using the code: `import pandas as pd` followed by `data_frame = pd.read_csv('world_population.csv')`.
What are some methods to filter data based on column values?
-You can filter data using comparison operators (e.g., `<`, `>`, `<=`, `>=`), the `.isin()` method for specific values, and the `.str.contains()` method for substring matching.
How can you filter for specific countries in the DataFrame?
-You can filter for specific countries by creating a list of those countries and using the `.isin()` method, like so: `data_frame[data_frame['country'].isin(['Bangladesh', 'Brazil'])]`.
What does the `.str.contains()` method do?
-The `.str.contains()` method checks if the string within a DataFrame column contains a specified substring and returns the filtered rows.
How can you filter data by index in a DataFrame?
-You can filter data by index using the `.filter()` method, or by using `.loc[]` for label-based indexing and `.iloc[]` for integer-based indexing.
What is the purpose of setting an index in a DataFrame?
-Setting an index allows for more intuitive access to rows, enabling easier data selection and manipulation based on specific labels.
How do you sort values in a DataFrame?
-You can sort values using the `.sort_values()` method, specifying the column(s) to sort by and the order (ascending or descending).
Can you sort a DataFrame by multiple columns? If so, how?
-Yes, you can sort by multiple columns by passing a list of column names to the `by` parameter in `.sort_values()`, and specifying their respective sorting order.
What is the difference between `.loc[]` and `.iloc[]`?
-.loc[] is used for label-based indexing, allowing you to access rows by their index labels, while .iloc[] is used for integer-based indexing, allowing you to access rows by their position in the DataFrame.
Outlines
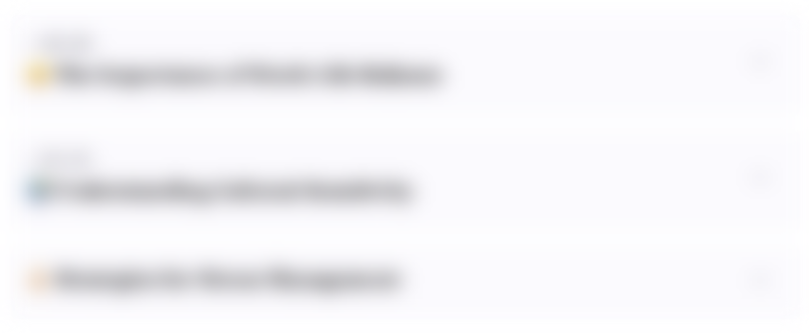
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
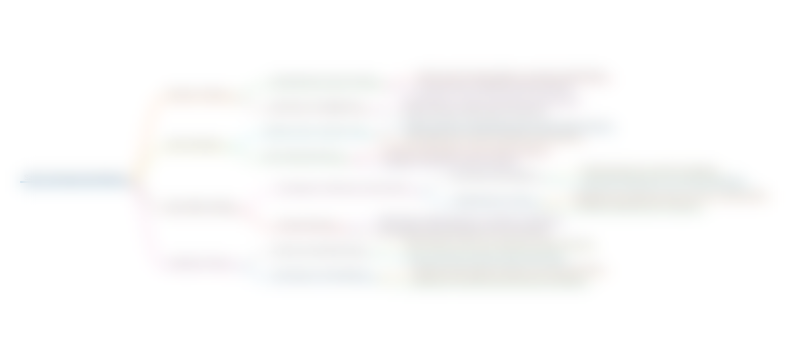
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
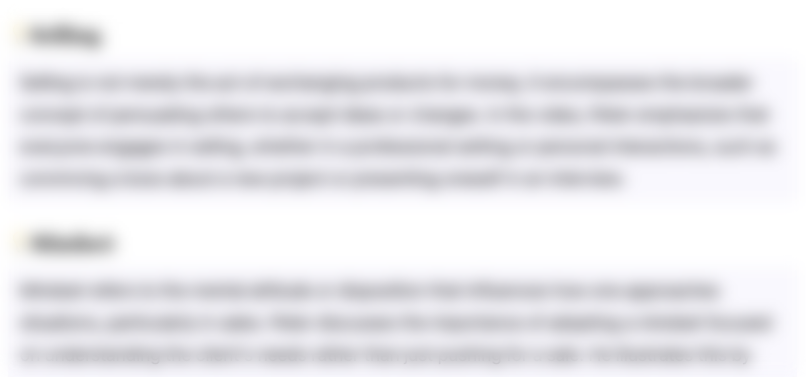
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
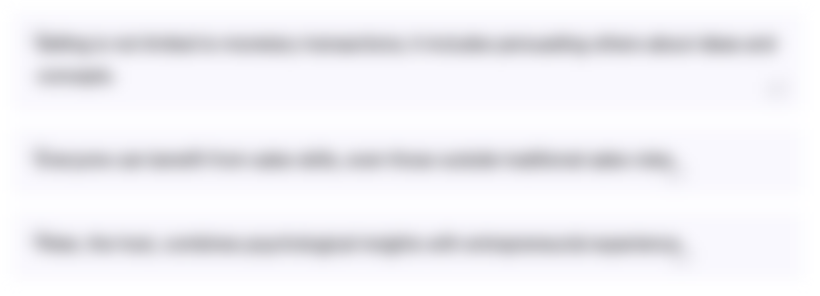
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
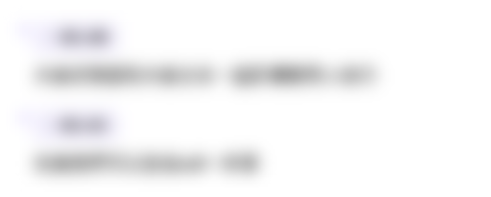
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)