Dataframes Part 02 - 01/03
Summary
TLDRThe script is a tutorial on handling data frames in Python using Pandas. It covers loading data, selecting specific columns, and accessing rows. The instructor demonstrates creating data frames from existing data and dictionaries, and explains the difference between series and data frames. They also show how to modify indices, use methods like 'head' and 'tail' for viewing rows, and utilize 'shape' to determine the dimensions of a data frame.
Takeaways
- π **Data Frame Loading**: The script starts by loading a dataset and selecting only the data part of it into a DataFrame called 'diabetes'.
- π **DataFrame Identification**: It's emphasized that a DataFrame is identified by an index and a structure similar to an Excel sheet with rows and columns.
- π’ **DataFrame Structure**: The 'diabetes' DataFrame contains specific columns like age, sex, BMI, BP, and other probabilities.
- π **Building DataFrames**: DataFrames can be constructed from existing libraries, APIs, dictionaries, or CSV files.
- π οΈ **DataFrame from Dictionary**: A DataFrame can be built from a dictionary where keys are column names and values are lists of data.
- π **Series vs DataFrame**: Accessing a single column from a DataFrame results in a Series, while accessing multiple columns retains the DataFrame structure.
- π **Accessing Columns**: Columns in a DataFrame can be accessed using the dot notation similar to accessing keys in a dictionary.
- π **Accessing Rows**: Rows in a DataFrame can be accessed using the `.loc` or `.iloc` methods, with `.loc` using index labels and `.iloc` using integer positions.
- π **Displaying Data**: The `.head()` and `.tail()` methods are used to display the first or last few rows of a DataFrame, which is useful for quick data inspection.
- π **DataFrame Shape**: The `.shape` attribute provides the dimensions of the DataFrame, indicating the number of rows and columns.
Q & A
What does 'dot data' refer to in the context of loading a dataset?
-In the context of loading a dataset, 'dot data' refers to accessing the 'data' attribute of an object, which typically contains the actual data within a dataset, excluding additional information such as descriptions.
How is a DataFrame represented visually in Python's pandas library?
-A DataFrame in pandas is visually represented with an index and columns, similar to an Excel sheet. It has a gray and white line display to indicate the rows and columns, with the first five and last five rows shown by default when the DataFrame is too large to fully display.
What is the significance of the index in a pandas DataFrame?
-The index in a pandas DataFrame is significant as it labels the rows and allows for efficient data retrieval. By default, it starts at 0 and increments by 1, but it can be customized to start at different values or use different labels.
How can you create a DataFrame from a dictionary in pandas?
-You can create a DataFrame from a dictionary by using the `pd.DataFrame()` function, where the dictionary's keys become the column names and the values become the data in the columns.
What must be true for all arrays when creating a DataFrame from a dictionary?
-When creating a DataFrame from a dictionary, all arrays (lists of values for each column) must have the same length, otherwise pandas will raise an error because it requires uniformity in the size of the data.
What is the difference between a Series and a DataFrame in pandas?
-A Series is a one-dimensional labeled array that behaves like a column in a DataFrame. A DataFrame is a two-dimensional labeled data structure with columns that can be of different types. Selecting a single column from a DataFrame results in a Series.
How do you access a single column from a DataFrame?
-To access a single column from a DataFrame, you use the DataFrame name followed by the column name in square brackets, similar to accessing a key in a dictionary.
What is the 'iloc' function used for in pandas DataFrames?
-The 'iloc' function in pandas is used for integer-location based indexing and selection by position. It allows you to access rows by their integer index, which is useful when you don't know the label of the row but know its position.
How can you view the first few rows of a DataFrame using a method?
-You can view the first few rows of a DataFrame using the 'head()' method. By default, it shows the first five rows, but you can specify a different number to see more or fewer rows.
What does the 'shape' attribute of a DataFrame return and what does it represent?
-The 'shape' attribute of a DataFrame returns a tuple where the first element is the number of rows and the second element is the number of columns, representing the dimensions of the DataFrame.
Outlines
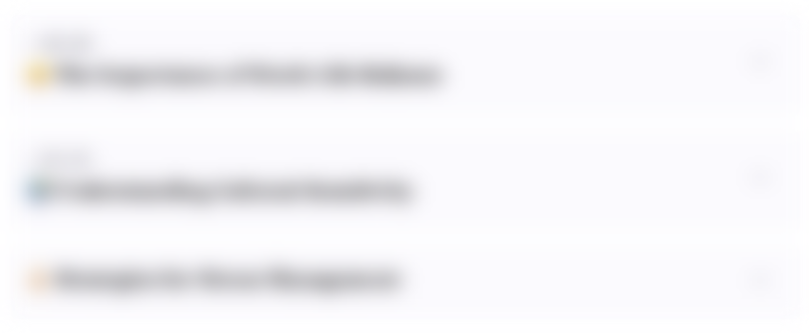
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
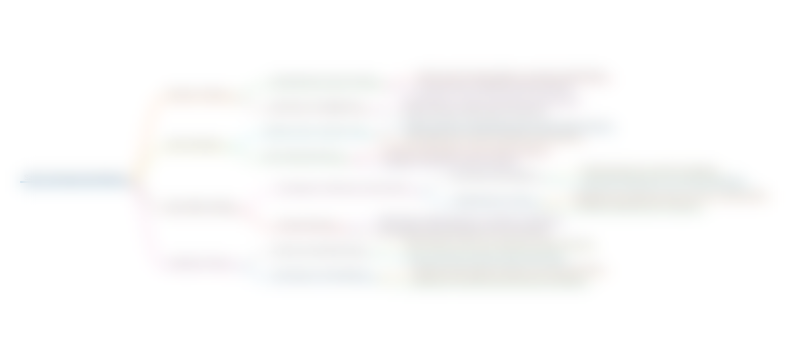
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
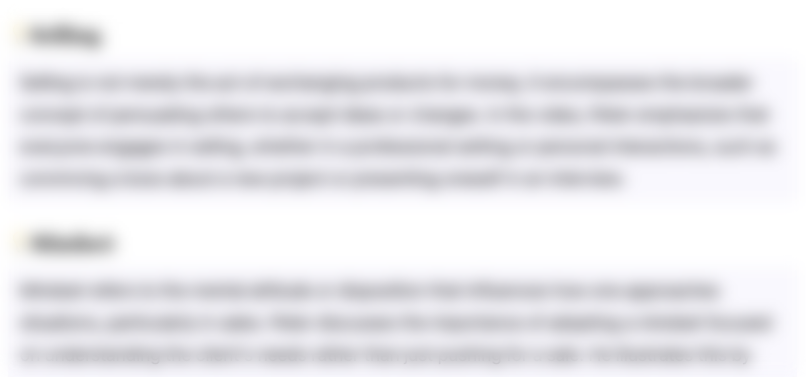
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
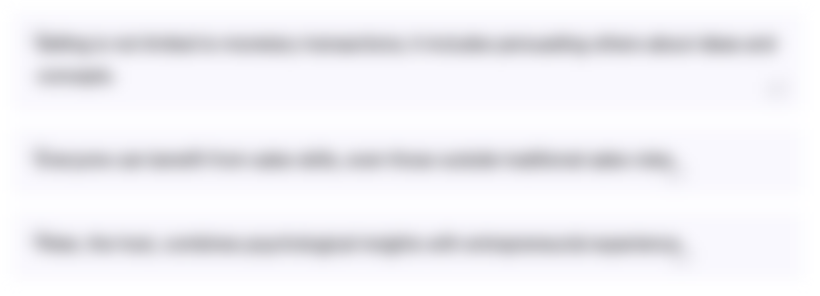
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
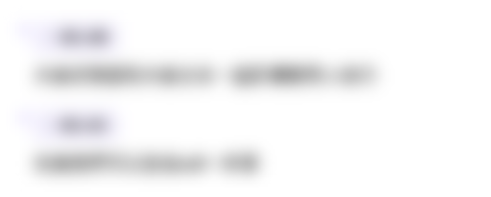
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)