List Comprehension in Python
Summary
TLDRThis video provides an in-depth explanation of list comprehensions in Python, comparing them to traditional loops. It starts by introducing list comprehension as a shorter, more efficient way to create lists from existing ones based on specific conditions. The presenter demonstrates the syntax and functionality of list comprehensions through practical examples, including creating lists with names containing 'J' and copying lists. The video also covers the optional condition-checking feature in list comprehension and concludes with a homework exercise, asking viewers to convert a for loop into a list comprehension.
Takeaways
- π List comprehension is a concise way to create new lists based on existing lists, using shorter syntax compared to traditional methods.
- π In list comprehension, the structure is: `[expression for item in iterable if condition]`, where the condition is optional.
- π§βπ» An example was given where a new list is created from an existing list of names, containing only those names that include the letter 'J'.
- π The traditional method using a for-loop to create a list was compared with list comprehension, demonstrating how the same task can be accomplished in a single line of code.
- π The script emphasizes how list comprehension reduces multiple lines of code into one, while maintaining the same functionality.
- βοΈ List comprehension is shown to work by iterating over an iterable, checking a condition, and then applying an expression to generate the new list.
- π In the syntax, 'for item in iterable' allows the loop to iterate over each item, and 'if condition' checks whether to include the item in the new list.
- π¦ The condition in list comprehension is not mandatory, as shown by an example where a simple copy of a list is created without any condition.
- π The script ends with a homework task, asking the audience to convert a given for-loop into list comprehension.
- β List comprehension is highlighted as a powerful tool for creating new lists efficiently, with examples provided to solidify understanding.
Q & A
What is list comprehension in Python?
-List comprehension is a concise way to create a new list from an existing list based on certain conditions. It provides a shorter syntax compared to traditional methods, such as using for loops.
What is the advantage of using list comprehension over traditional for loops?
-The main advantage of using list comprehension is that it allows you to write the same logic in a more concise and readable format, reducing the number of lines of code needed.
Can you provide an example of a simple list comprehension?
-Certainly! For example, to create a list of names that contain the letter 'J' from an existing list, you can use: `jnames = [name for name in names if 'J' in name]`.
How does the syntax of list comprehension work?
-The basic syntax is: `[expression for item in iterable if condition]`. Here, the `expression` is the value to include in the new list, `item in iterable` is the loop through the iterable, and the `if condition` filters the items to be included.
Is the condition in list comprehension mandatory?
-No, the condition part of list comprehension is optional. If you don't include a condition, all items in the iterable will be included in the new list.
How can you create a copy of a list using list comprehension?
-You can create a copy of a list without any conditions by using: `names_copy = [name for name in names]`, which is equivalent to looping through all items and appending them to the new list.
In the provided example, how did the traditional for loop work?
-The traditional for loop iterated over the `names` list, checking if each name contained the letter 'J'. If it did, the name was appended to the `jnames` list using `jnames.append(name)`.
How does list comprehension replace the for loop in the example?
-In the list comprehension version, the entire for loop is condensed into one line: `jnames = [name for name in names if 'J' in name]`. This replaces the loop, condition checking, and appending in a shorter form.
What is the role of the 'if' condition in list comprehension?
-The 'if' condition filters the items that will be included in the new list. Only items that satisfy the condition are processed and added to the resulting list.
Can list comprehension be used for complex operations?
-Yes, list comprehension can handle more complex expressions, such as mathematical operations or applying functions to each item. However, its primary use is for simple, concise list transformations.
Outlines
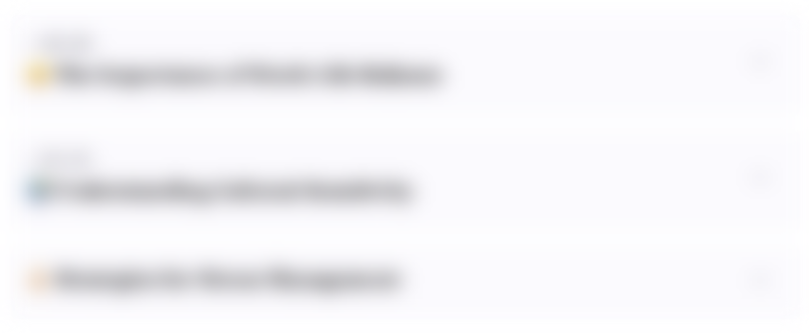
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
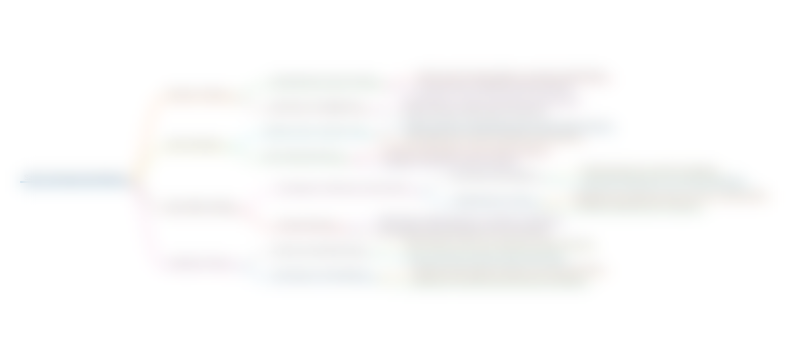
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
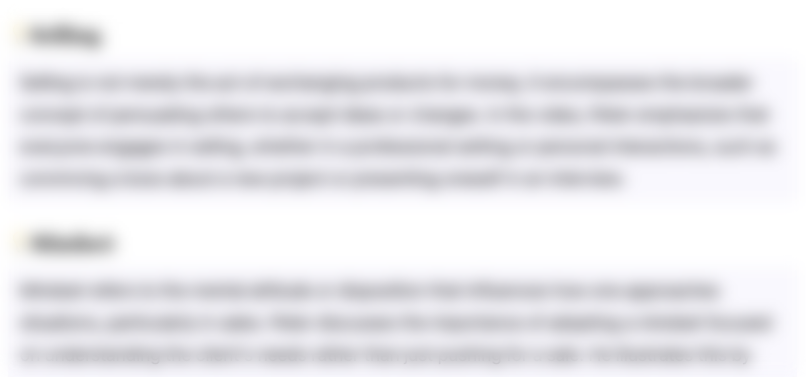
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
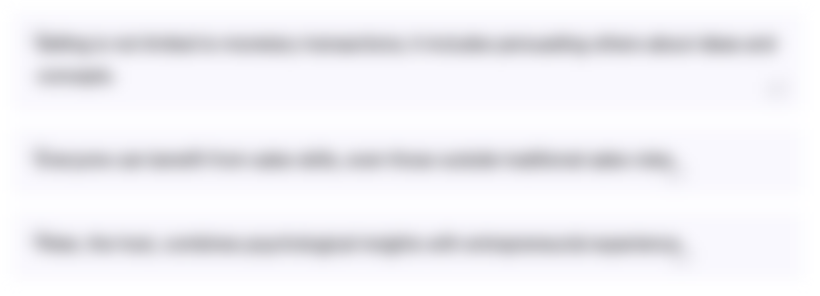
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
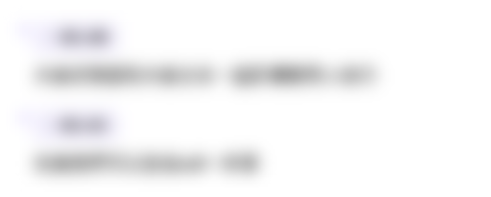
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)