JavaScript - Throttle (節流) 常見的面試問題 (Scrollbar, Infinite Scroll) (前端優化)
Summary
TLDRThe video script introduces the concept of Throttling, a technique similar to Debouncing but with distinct behaviors. It demonstrates how Throttle can be applied to implement an Infinite Scroll feature on a webpage. The script provides a step-by-step guide on creating a Throttle function, emphasizing the importance of using a regular function for the callback to avoid arrow function scoping issues. It also explains the difference between Throttle, which executes the callback at regular intervals during an action, and Debounce, which executes the callback after the action has ceased for a specified time.
Takeaways
- 🚦 Throttle and Debounce are similar concepts but have distinct differences in their execution. Throttle is used for controlling the frequency of function execution, while Debounce is for delaying the execution until a certain amount of time has passed without any further triggering.
- 🔄 The Throttle function is implemented with two parameters: the callback function to be executed and the time interval.
- 🔧 In the Throttle function, a timer is used to control the frequency of execution, and it's shared across all invocations of the function through closure.
- ⏱️ The Throttle function should clear the timer before executing the callback to ensure it doesn't accumulate multiple timers.
- 🎯 Throttle is used to solve scenarios where you want a function to fire at a consistent interval while an action is being performed, like loading new elements on scroll.
- 📈 The Infinite Scroll functionality can be achieved by using Throttle to check scroll position and load more content when nearing the end of the list.
- 📊 To determine if the scroll has reached the bottom, the script calculates the sum of scroll position and client height, and compares it to the scroll height.
- 🤔 Floating-point arithmetic issues can cause the scroll position check to be inaccurate, so adding a buffer (like 10 pixels) to the comparison can ensure more reliable results.
- 🔄 The video script suggests that for early loading of content, the check can be triggered at a certain percentage (e.g., 90%) of the scroll height.
- 👷 The use of a regular function (not an arrow function) in Throttle allows for the preservation of the function context, which is important if the callback function expects to have access to specific arguments or the 'this' keyword.
- 🔗 If the callback function requires arguments or a specific 'this' context, the Throttle function should return a version of the callback that has been bound with the correct context and arguments using methods like 'apply'.
Q & A
What is the main topic of the video?
-The main topic of the video is the implementation of the Throttle function in JavaScript, which is used to control the rate at which a function is executed. The context of its use in creating an infinite scroll feature is also discussed.
How does Throttle differ from Debounce, according to the video?
-Throttle differs from Debounce in that Throttle controls the execution rate of a function during continuous actions, executing the function at regular intervals, while Debounce waits for the action to stop and then executes the function after a certain delay.
Why does the presenter decide not to use an arrow function for the Throttle implementation?
-The presenter decides not to use an arrow function for the Throttle implementation because the context of 'this' and the passing of arguments are not concerns for the example shown. However, for situations where 'this' binding or argument passing is necessary, a different approach would be required.
What problem does Throttle solve in the video demo?
-In the video demo, Throttle solves the problem of excessive function executions during continuous events, such as scrolling. It's applied to efficiently load new elements for an infinite scroll feature without overwhelming the browser with too many calls.
What kind of elements are initially created on the webpage for the infinite scroll demo?
-For the infinite scroll demo, the webpage initially creates 30 paragraph ('p') tags to enable scrolling and demonstrate the functionality.
What criteria are used to load more elements in the infinite scroll feature?
-More elements are loaded in the infinite scroll feature when the scrollbar reaches the bottom of the page. This is determined by calculating if the sum of scrollTop and clientHeight equals the scrollHeight, indicating that the bottom has been reached.
What adjustments are made to handle floating point issues when checking if the bottom of the page is reached?
-To handle floating point issues when checking if the bottom of the page is reached, a small additional value (like 10) might be added to the sum of scrollTop and clientHeight to ensure it exceeds scrollHeight, accommodating any discrepancies due to floating point calculations.
How is the Throttle function adjusted to handle parameters and 'this' binding if needed?
-If parameters and 'this' binding are needed, the Throttle function can be adjusted by using 'apply' to bind 'this' and pass arguments to the callback function, ensuring the correct context and parameters are used during execution.
What suggestion from a viewer does the presenter mention at the beginning?
-The presenter mentions a suggestion from a viewer regarding a problem that could be solved using the Throttle function, highlighting the practical application of concepts discussed in the video.
What alternative threshold is suggested for triggering the loading of new elements before reaching the bottom of the page?
-An alternative threshold suggested is checking if the ratio of (scrollTop + clientHeight) to scrollHeight is greater than or equal to 0.9, indicating the scrollbar is 90% down the page, which triggers loading new elements before actually reaching the bottom.
Outlines
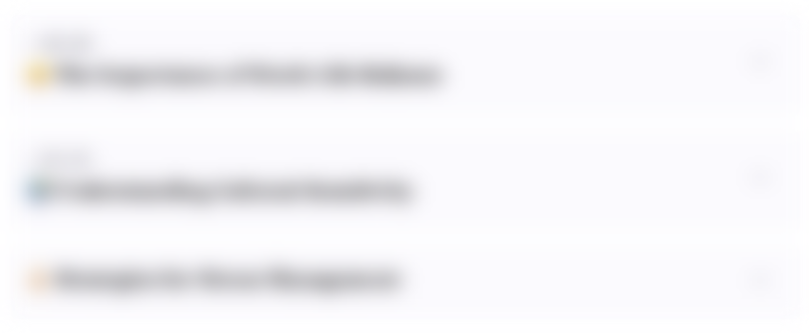
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
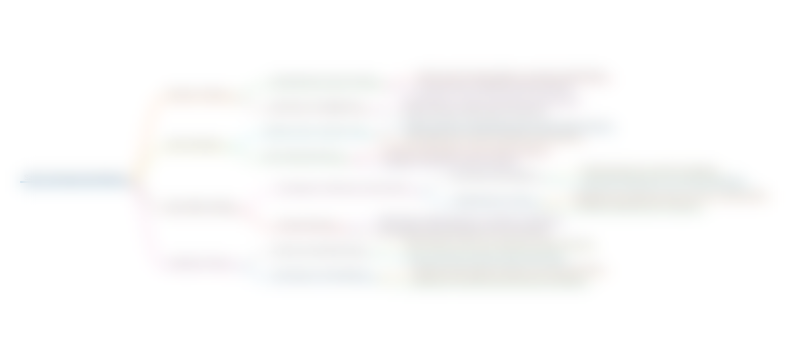
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
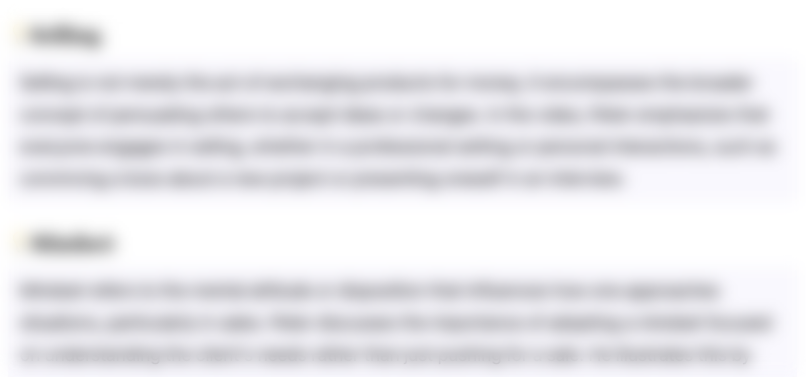
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
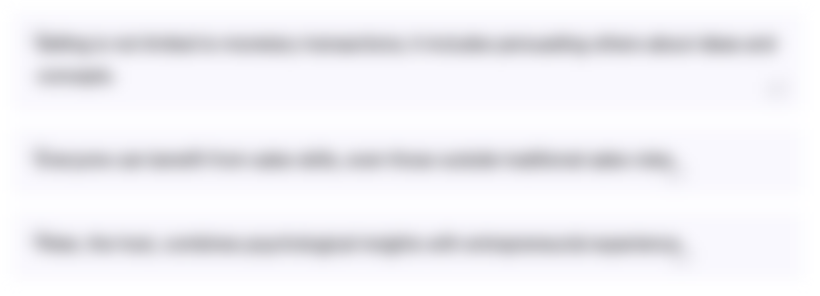
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
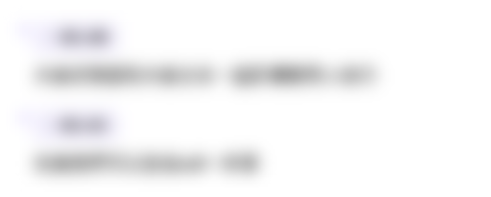
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
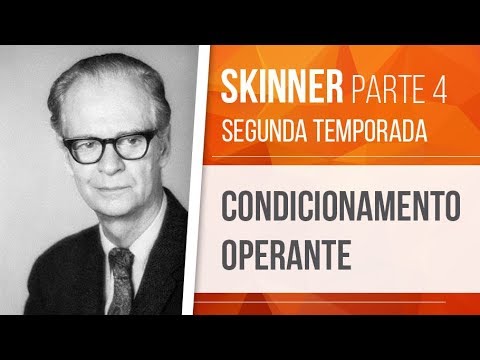
SKINNER (4) – CONDICIONAMENTO OPERANTE | BEHAVIORISMO (SEGUNDA TEMPORADA)

Top 10 JavaScript Interview Questions EXPLAINED! | Tanay Pratap Hindi
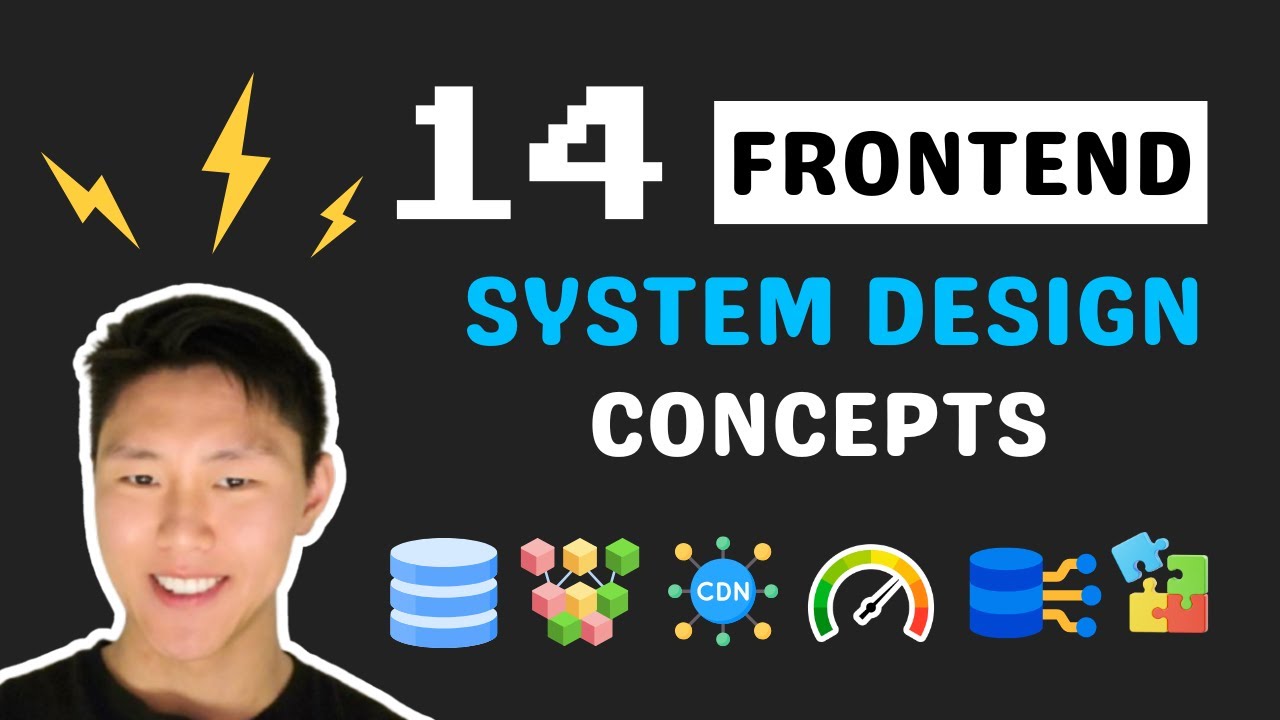
14 Front End System Design Concepts Explained in 10 Minutes

PENGERTIAN DAN CONTOH REINFORCEMENT

삼성 좀 실수한 듯? 갤럭시S24 기본형 | 엑시노스 성능, 발열, 전력소모, 배터리 살펴보기
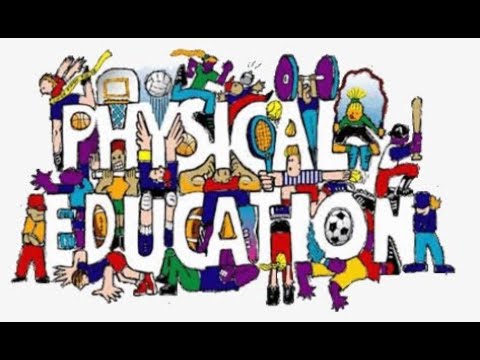
Teknik Mengapung Water Trappen - Materi PJJ PJOK SD
5.0 / 5 (0 votes)