React JS Explained In 10 Minutes
Summary
TLDRThis video introduces core concepts essential for React developers, such as building user interfaces with React, understanding single page applications, components, JSX, React Router, props, state management, the component life cycle, React Hooks, state management at a global level, the Virtual DOM, key props, event handling, form management, conditional rendering, and common commands. It emphasizes the importance of mastering these concepts for efficient web development and offers a crash course for further learning.
Takeaways
- 📚 React is a JavaScript library for building user interfaces, popular among modern web applications like Facebook, Netflix, and Airbnb.
- 🌐 Single page applications with React involve a single template that updates components within the DOM, rather than loading new pages.
- 🧱 Components are the building blocks of React applications, allowing for independent, reusable pieces of the user interface.
- 🔄 Components can be nested and composed, with a parent component holding one or more child components.
- 🎨 JSX (JavaScript XML) is used instead of traditional HTML, allowing for the mixing of JavaScript logic with HTML-like syntax.
- 🔄 React Router enables multiple 'pages' within a single page application, syncing the UI with the URL.
- 🔧 Props are used to pass data from parent components to children, but 'prop drilling' can be mitigated with context or state management libraries.
- 📊 State is used to manage and update dynamic data within components, with modern React favoring functional components and hooks over class-based methods.
- 🔄 Component lifecycle includes mounting, updating, and unmounting phases, with hooks like `useEffect` managing lifecycle events.
- 🔌 State management solutions like Context API or Redux allow for global state sharing across multiple components without prop drilling.
- 🌳 The Virtual DOM is a virtual representation of the real DOM, enabling React to efficiently update the real DOM only where changes have occurred.
- 🛠️ Key commands for React development include `create-react-app` for project setup, `start` for running the development server, and `build` for creating a production build.
Q & A
What is React and what is it used for?
-React is a JavaScript library used for building user interfaces, particularly for single-page applications. It provides a set of tools and structure that makes the process of building UIs faster and easier. Popular websites like Facebook, Netflix, and Airbnb are built using React.
How do single-page applications work in contrast to traditional websites?
-In traditional websites, a separate template is used for each page, which is returned to the user upon request. In contrast, single-page applications use a single template and update the components within the DOM to reflect changes in the user's interaction, making the experience more dynamic and seamless.
What are components in React and how do they function?
-Components are the building blocks of the visual layer in a React application. They are JavaScript classes or functions that return HTML (in the form of JSX). Components can be independent, reusable, and can be nested within one another to create complex UI structures.
What is JSX and how does it differ from traditional HTML?
-JSX stands for JavaScript XML and is a syntax extension used with React. It looks similar to HTML but allows for the embedding of JavaScript code, such as variables and logic, directly into the markup. Unlike HTML, JSX requires a compiler to be converted into JavaScript and native HTML that browsers can understand.
How does React Router facilitate multiple pages within a single-page application?
-React Router is a library that enables URL routing in single-page applications. It keeps the UI in sync with the URL by rendering components into the DOM based on the current URL, allowing users to navigate through different 'pages' or views within the application without needing to reload the entire page.
What is the purpose of props in React and how can they be used?
-Props, short for 'properties', are used to pass data from a parent component to a child component. They can be passed down like function parameters and can be used anywhere within the child component. Props can also be passed down multiple layers, but this can lead to 'prop drilling', which can be managed with solutions like React Context or third-party libraries like Redux.
How does the state work in React and what is its significance?
-State in React is a JavaScript object that represents information about a component. It is traditionally managed using class-based components or, more recently, React hooks like useState. The state can be updated in response to user interactions or data fetching, triggering the component's lifecycle methods and causing re-rendering to reflect the updated information.
What are the main phases of a React component's lifecycle?
-A React component goes through three main lifecycle phases: mounting, updating, and unmounting. The mounting phase occurs when the component is first added to the DOM, the updating phase deals with changes and modifications, and the unmounting phase happens when the component is removed from the DOM. Hooks like useEffect and lifecycle methods in class components help manage these phases effectively.
What are React hooks and why are they important?
-React hooks are functions that allow you to 'hook into' React state and lifecycle features from function components. They are essential for managing state and side effects in functional components without the need for class components. Common hooks include useState for managing state and useEffect for handling lifecycle events and side effects.
How does state management work in React applications?
-While individual components can maintain their own state, there are times when a global state is necessary for data to be accessible across multiple components. State management solutions like React's Context API or third-party libraries like Redux allow for the creation of a centralized state that can be shared across components, avoiding the issue of prop drilling.
What is the Virtual DOM in React and how does it improve performance?
-The Virtual DOM is a virtual representation of the actual DOM used by React. When components are updated, React updates the Virtual DOM instead of the real DOM. This allows React to calculate the most efficient changes and update only the necessary parts of the real DOM, leading to improved performance and faster rendering.
What are some key considerations when rendering lists in React?
-When rendering lists in React, it's important to use the 'key' prop for each item to help React identify which items have changed, added, or removed. The key prop should be unique and helps React optimize the rendering process by only updating the parts of the DOM that have actually changed.
How do event listeners and form handling differ in React compared to traditional JavaScript?
-In React, event listeners are handled by passing inline functions directly to event attributes like 'onClick' or 'onChange'. This differs from traditional JavaScript where methods like addEventListener are used. Form handling in React involves managing state within the component and updating it based on user input, as opposed to relying on the form elements to manage their state, which is the case in traditional HTML forms.
What are conditional rendering and its use cases in React?
-Conditional rendering in React allows certain parts of the UI to be displayed or hidden based on certain conditions. This is useful for scenarios like showing a user's name in a navigation bar only if the user is authenticated. Logical operators and inline conditionals like the ternary operator can be used to control the rendering of elements based on the state of the application.
What are the common commands used in React projects?
-Three common commands used in React projects are 'create-react-app' for generating the boilerplate files for a new React application, 'start' for running the development server to view the project, and 'build' for creating a production-ready build of the application for deployment.
Outlines
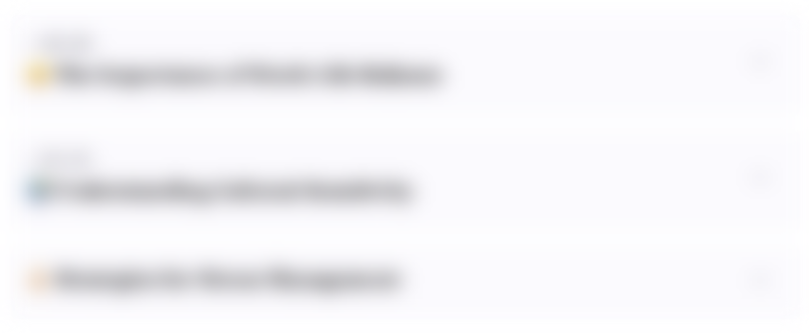
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
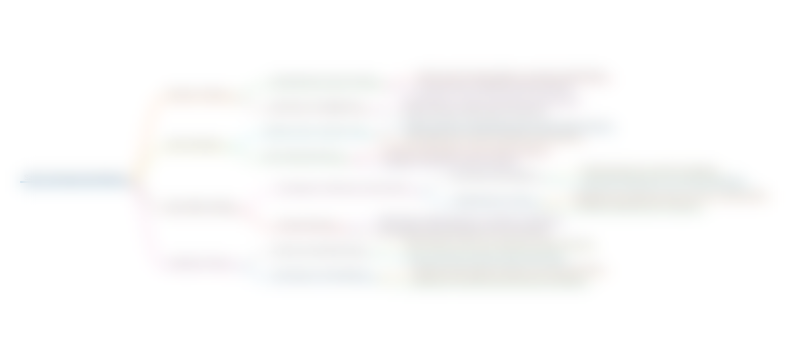
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
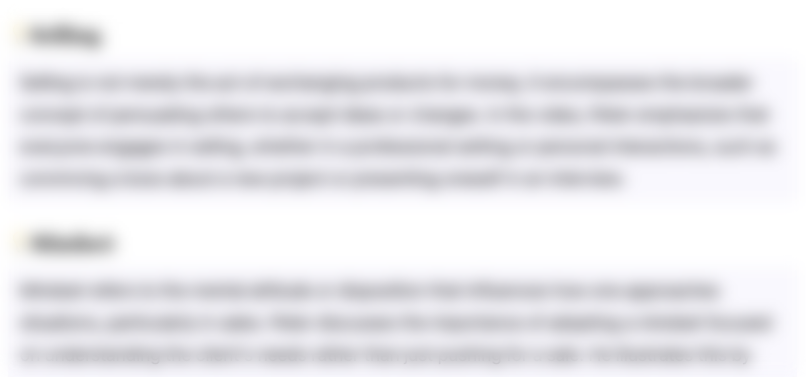
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
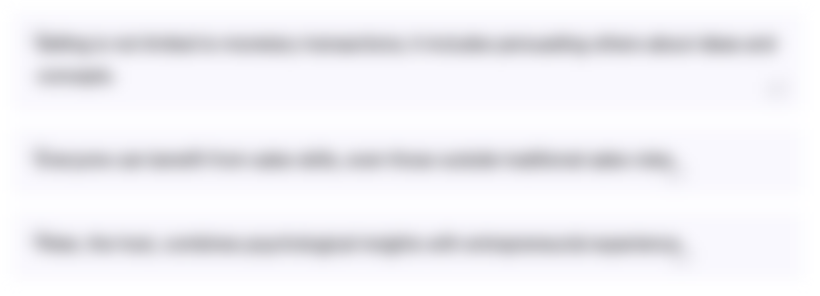
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
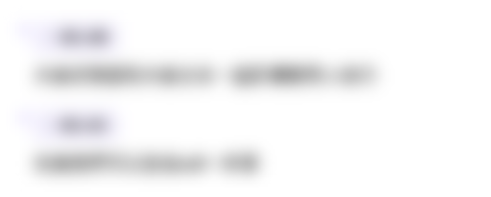
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)