Instances and Elements in Practice | Lecture 122 | React.JS ๐ฅ
Summary
TLDRThis video script delves into the intricacies of component instances and React elements in React code. It explores the significance of logging a component instance to the console, highlighting its type and props. The concept of directly calling components versus rendering them in JSX is discussed, emphasizing the importance of adhering to proper rendering practices to maintain component state management and avoid potential security risks. The script also touches on React's built-in protection against cross-site scripting attacks through the use of symbols. Overall, it provides insights into the inner workings of React components and the importance of following best practices for efficient and secure code implementation.
Takeaways
- ๐ React internally calls a component function and returns a React element representation when rendering a component.
- ๐ง The React element representation contains the component type and props, and uses a symbol for security against cross-site scripting attacks.
- โ ๏ธ Directly calling a component function yourself will not create a proper component instance, and React will treat the result as a raw React element instead.
- ๐ Always render components using JSX, allowing React to properly handle component instances and manage their state.
- ๐ณ Component instances each have their own state and props, separate from the parent component.
- ๐ซ Calling components directly within another component violates the rules of hooks and can lead to issues with state management.
- ๐ก The provided example demonstrates rendering a single component blueprint multiple times, creating separate component instances.
- ๐ React's component rendering process involves creating instances from the component blueprint, managing state, and rendering the appropriate elements.
- ๐ The symbol used in React elements prevents cross-site scripting attacks by ensuring the element cannot be transmitted via JSON from an untrusted source.
- ๐ Logging a component instance to the console shows its type and props, while directly calling the component function shows the raw React element output.
Q & A
What is the purpose of logging the component instance to the console?
-The purpose of logging the component instance to the console is to inspect the internal structure and properties of the component as seen by React. This allows developers to understand how React represents and processes components internally.
What is the significance of the '$$ type' symbol in the component instance output?
-The '$$ type' symbol is a security feature implemented by React to protect against cross-site scripting attacks. Since symbols are JavaScript primitives that cannot be transmitted via JSON, React can identify and reject fake React elements that may be sent from an API, preventing potential security vulnerabilities.
Why is it not recommended to call components directly instead of rendering them through JSX?
-Calling components directly instead of rendering them through JSX can lead to several issues. React may not recognize the component as an instance, which can cause problems with state management and violate the rules of hooks. Additionally, direct component calls bypass React's internal rendering process, which can lead to unexpected behavior and potential performance issues.
What happens when a component is called directly within another component's code?
-When a component is called directly within another component's code, React does not recognize it as a component instance. Instead, it treats the output as a raw React element. This means that the called component cannot manage its own state, and its state and hooks may get merged into the parent component's state, violating the rules of hooks and potentially causing other issues.
How does the script demonstrate the difference between a component instance and a raw React element?
-The script logs both a component instance and a directly called component to the console. The component instance output shows the component type and any passed props, while the directly called component output shows a different type (the rendered content) and different props (like the class name).
What is the recommended way to render a component in React?
-The recommended way to render a component in React is by using JSX syntax, which allows React to recognize it as a component instance and properly handle its rendering, state management, and lifecycle methods.
What is the relationship between a component and its instances in React?
-A component in React is like a blueprint or a template, while component instances are the actual rendered instances of that component. Each instance has its own state and props, allowing for multiple instances of the same component to exist with different data and behavior.
How does the script demonstrate the concept of multiple component instances?
-The script shows that the 'TabContent' component is rendered four times, resulting in four separate component instances. Each instance has its own state and props, as demonstrated by the component tree inspection in the script.
What is the purpose of the 'TabContent' component used in the script?
-The 'TabContent' component is a stateful component used to manage the content displayed within a tab interface. It likely contains state and logic related to rendering and updating the content based on user interactions or other events.
What is the overall message or lesson conveyed by the script?
-The overall message conveyed by the script is the importance of following the recommended practices for rendering and working with components in React. It highlights the potential issues that can arise from directly calling components instead of rendering them through JSX, and emphasizes the need to respect React's internal rendering process and component instance model.
Outlines
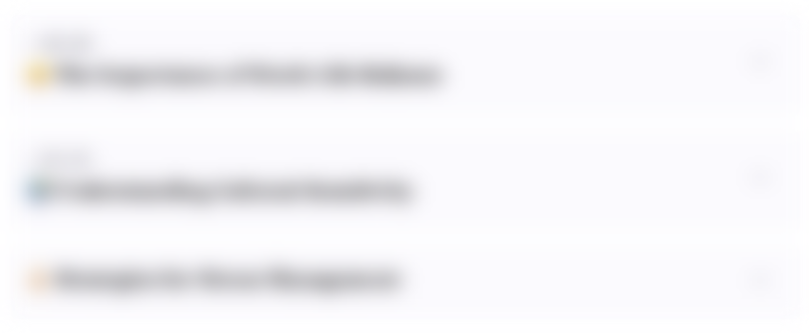
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
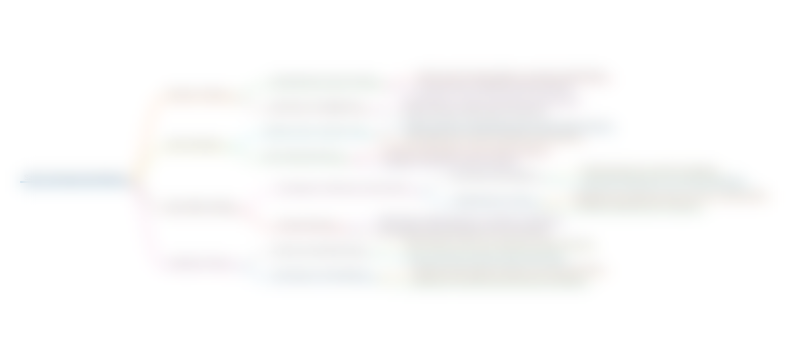
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
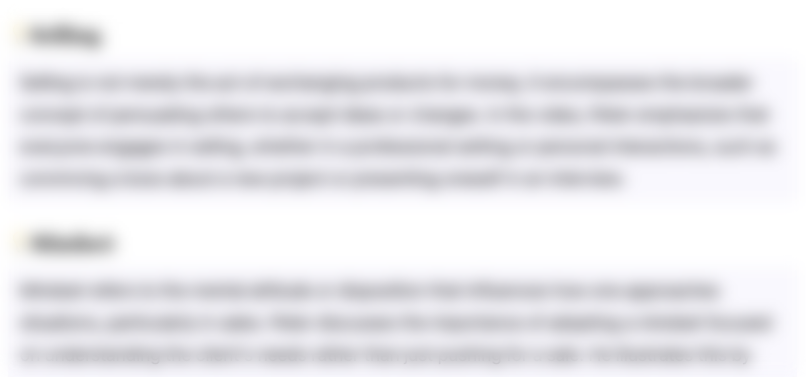
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
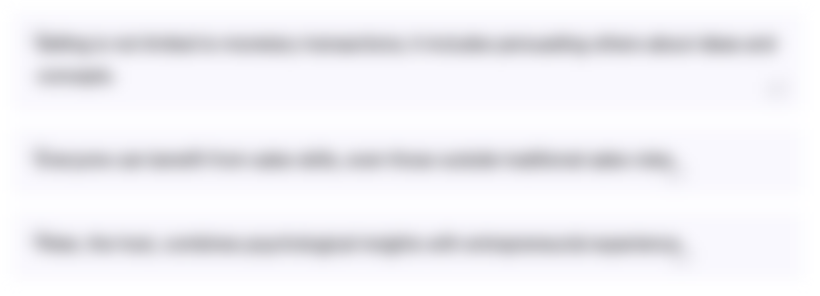
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
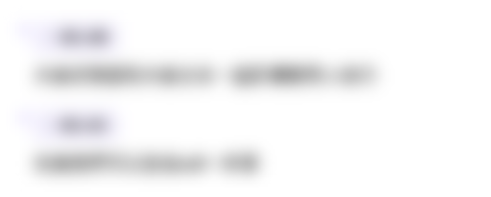
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Components, Instances, and Elements | Lecture 121 | React.JS ๐ฅ

The Key Prop | Lecture 128 | React.JS ๐ฅ

State Update Batching in Practice | Lecture 133 | React.JS ๐ฅ

State Update Batching | Lecture 132 | React.JS ๐ฅ

A First Look at Effects | Lecture 141 | React.JS ๐ฅ
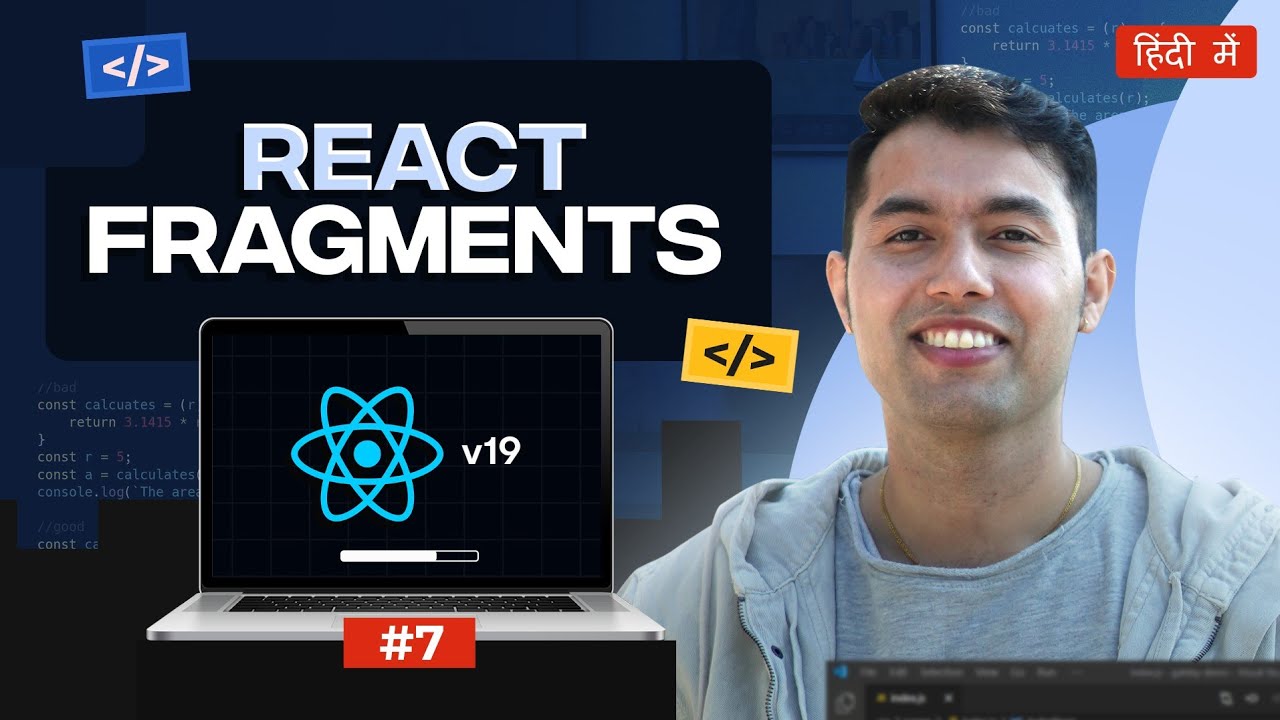
#7: React Fragments: Remove unwanted Nodes & Speed Up Rendering | React v19 Tutorial in Hindi
5.0 / 5 (0 votes)