Components, Instances, and Elements | Lecture 121 | React.JS 🔥
Summary
TLDRThe video script delves into the conceptual differences between React components, component instances, and React elements. It explains that components are JavaScript functions that return React elements, serving as blueprints or templates for the UI. Component instances are the actual manifestations of these components, each holding its own state, props, and lifecycle. React elements, on the other hand, are immutable JavaScript objects created from component instances, containing information to generate DOM elements. The script highlights the journey from writing a component to rendering it as HTML in the DOM, clarifying the distinction between these key React concepts.
Takeaways
- 🔍 Components in React are essentially blueprints or templates for a piece of the UI, described using JSX syntax.
- 🛠️ A React component is a regular JavaScript function that returns React elements, forming an element tree.
- 🔄 Multiple instances of a component can be created from a single blueprint, each instance representing the component's physical presence in the component tree.
- 📦 Each component instance holds its own state and props, and goes through its own lifecycle, akin to a living organism.
- 🔄 Despite technical differences, the terms 'component' and 'component instance' are often used interchangeably in practice.
- 🔧 When a component is used in code, React elements are generated, which are immutable JavaScript objects kept in memory.
- 🔗 React elements contain all necessary information for creating DOM elements for the current component instance.
- 🎨 Ultimately, React elements are converted into actual DOM elements, which are then rendered to the screen by the browser.
- 🌐 The DOM elements are the final, visual representation of a component instance in the browser, not the React elements themselves.
- 🚀 The process from writing a component to it being rendered as HTML elements in the DOM illustrates the lifecycle of components in React applications.
Q & A
What is the conceptual difference between React components, component instances, and React elements?
-React components are the functions we write to describe a piece of the user interface, which return React elements. A component instance is the actual physical manifestation of a component in our componentry, created each time we use the component in our code. React elements are the immutable JavaScript objects created by calling React.createElement, containing information necessary to create DOM elements for the current component instance.
How are component instances created?
-React creates a new component instance each time we use the component in our code. For example, if a component is included three times in an app component, React will call the component function three times, creating three instances.
What does a component instance contain?
-Each component instance holds its own state, props, and lifecycle. It's like a living organism that can be born, live for some time, and eventually die.
What is the role of React elements?
-React elements are the result of using a component in our code. They are immutable JavaScript objects that React keeps in memory, containing information necessary to create DOM elements for the current component instance.
How are DOM elements created from React elements?
-React elements are not directly rendered to the DOM. Instead, they are converted to actual DOM elements, which are then painted onto the screen by the browser.
Can we use the terms 'component' and 'component instance' interchangeably?
-In practice, we often use the terms 'component' and 'component instance' interchangeably, even though 'component instance' would be more technically accurate when referring to the actual manifestation of a component in our componentry.
What is the purpose of JSX?
-JSX is a syntax extension that allows us to write React elements using a syntax that looks like HTML. Behind the scenes, JSX gets converted to multiple React.createElement function calls, which create the React elements.
What is the journey from writing a component to rendering it on the DOM?
-The journey starts with writing a component function, which is used multiple times in our code as a blueprint. Each time it is used, React creates a component instance, which returns a React element. This React element is then converted to actual DOM elements and painted onto the screen by the browser.
Why is understanding the difference between components, instances, and elements important?
-Understanding this difference is important because it helps clarify what actually happens with our components as we use them in our React applications. It's also a common interview question, so it's worth learning about this topic.
Can React elements directly influence the DOM?
-No, React elements themselves have nothing to do with the DOM. They simply live inside the React app and are used to create DOM elements when they are rendered on the screen.
Outlines
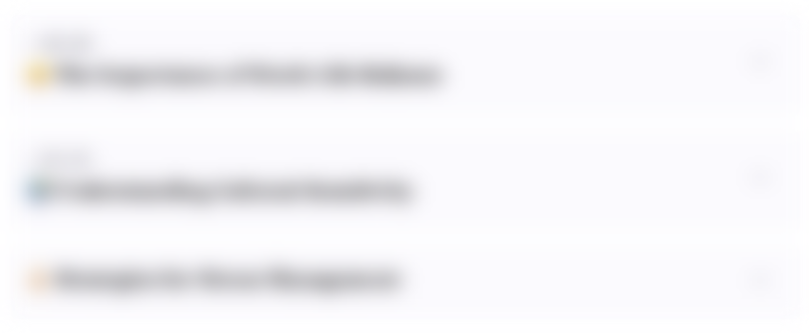
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
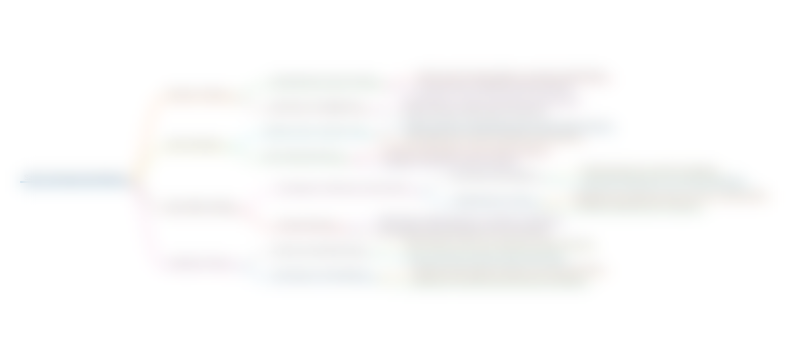
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
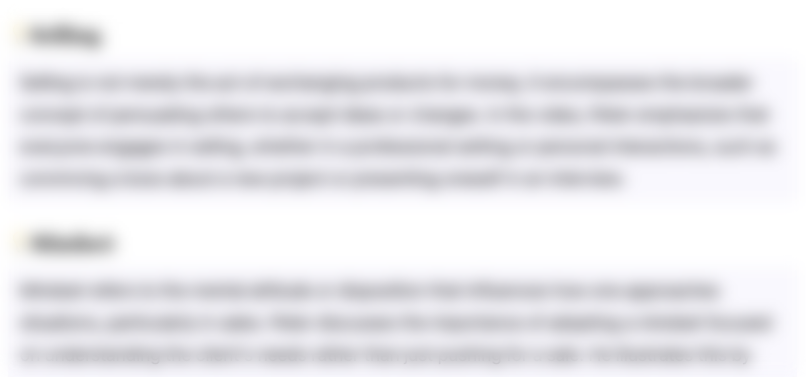
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
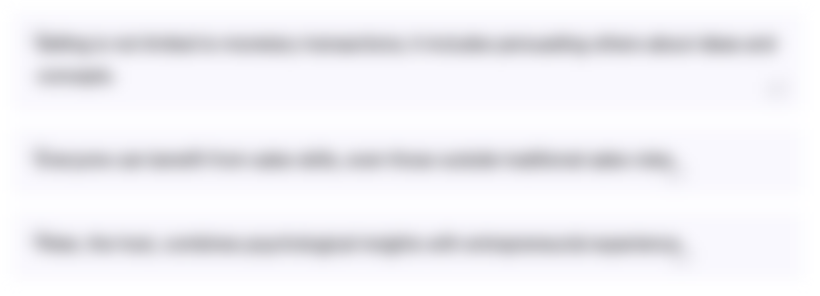
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
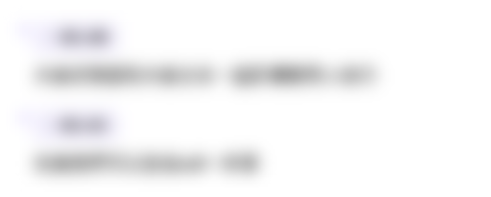
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Instances and Elements in Practice | Lecture 122 | React.JS 🔥

A First Look at Effects | Lecture 141 | React.JS 🔥
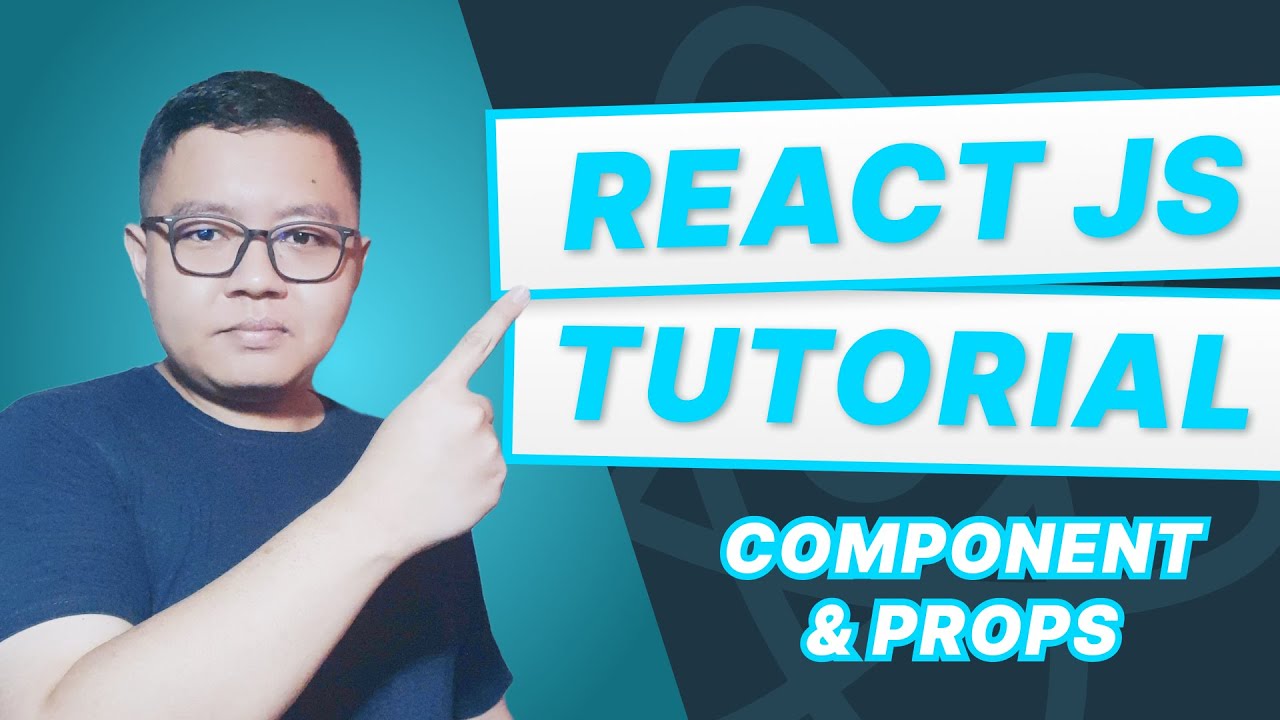
Tutorial React JS Bahasa Indonesia : 3.Component & Props
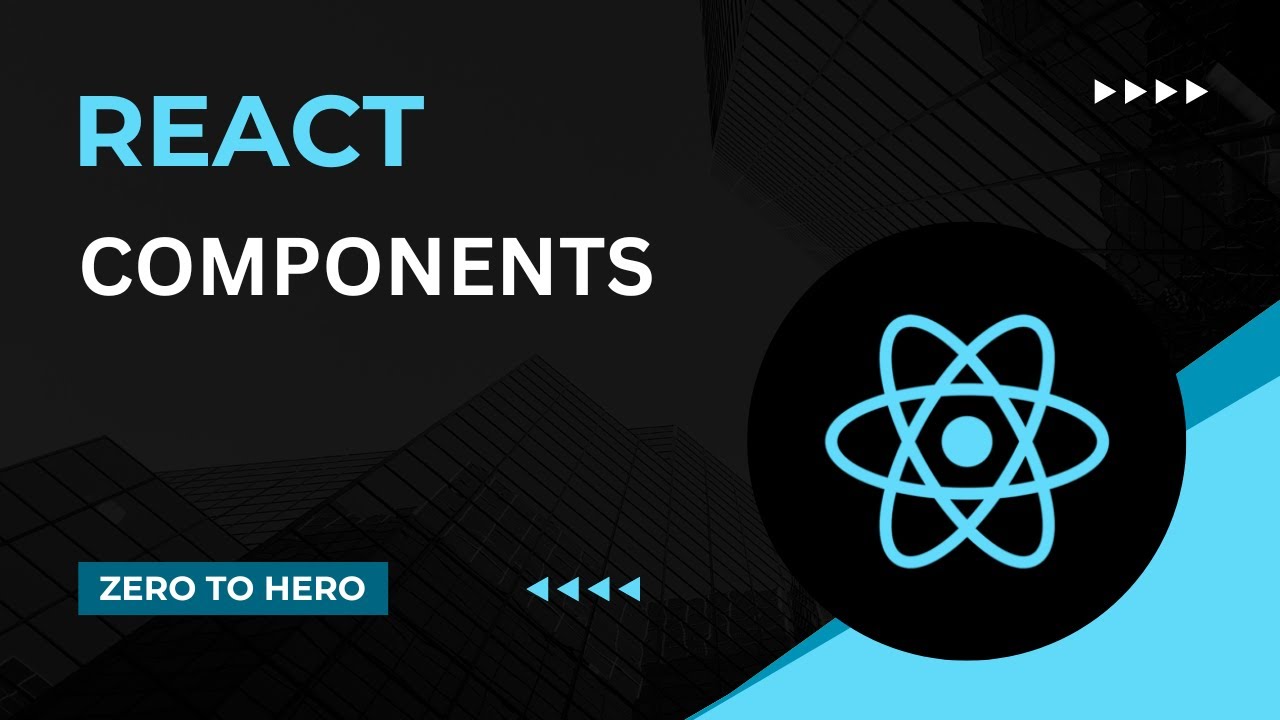
Components | Mastering React: An In-Depth Zero to Hero Video Series

How Rendering Works: Overview | Lecture 123 | React.JS 🔥

The Key Prop | Lecture 128 | React.JS 🔥
5.0 / 5 (0 votes)