threading vs multiprocessing in python
Summary
TLDRThis video delves into the complexities of Python multiprocessing and threading, focusing on the distinction between parallel and concurrent execution. It explains how the operating system manages processes and threads, as well as how Python's Global Interpreter Lock (GIL) affects multi-threading performance. The video covers key concepts such as locks, thread safety, and using data-sharing techniques like pipes and queues. It emphasizes that while multi-threading might not always provide speed boosts due to the GIL, multi-processing can offer better performance for CPU-bound tasks. Viewers are encouraged to consider the right tools for their specific applications.
Takeaways
- π Multi-threading in Python does not achieve true parallelism due to the Global Interpreter Lock (GIL), meaning threads do not run simultaneously but appear to do so by switching rapidly.
- π Multi-processing, on the other hand, allows Python to run separate processes with their own memory space, achieving true parallelism across multiple CPU cores.
- π Multi-threading is best suited for I/O-bound tasks like file reading or network requests, where threads spend a lot of time waiting for external processes.
- π Multi-processing is ideal for CPU-bound tasks, as it enables parallel execution across multiple CPU cores, significantly improving performance for tasks requiring heavy computation.
- π Threads in Python are lightweight and low-overhead, but the GIL limits the benefit of multi-threading for CPU-heavy operations.
- π Multi-threading can still offer concurrency, allowing multiple threads to execute in a seemingly parallel manner by interleaving their operations.
- π Multi-processing involves more overhead, such as process creation and inter-process communication, but it is more suitable for tasks that require heavy CPU usage.
- π Python's threading system may require synchronization to avoid race conditions, typically using locks to ensure data integrity when multiple threads access shared data.
- π Locks prevent data corruption in multi-threaded environments by ensuring that only one thread can modify or access a resource at any given time.
- π Queues are thread- and process-safe for sharing data between threads or processes, unlike pipes, which may not always work reliably in multi-threaded environments.
- π When designing applications, it's crucial to choose between multi-threading and multi-processing based on the type of workload to achieve the best performance: multi-threading for I/O-bound tasks and multi-processing for CPU-bound tasks.
Q & A
What is the main difference between multi-threading and multi-processing in Python?
-The main difference is that multi-threading runs multiple threads within a single process, but due to Python's Global Interpreter Lock (GIL), these threads cannot execute simultaneously in CPU-bound tasks. In contrast, multi-processing runs separate processes, allowing them to execute in parallel, making it suitable for CPU-bound tasks.
How does Python's Global Interpreter Lock (GIL) affect multi-threading?
-The GIL prevents threads from executing simultaneously in CPU-bound tasks. This means that although multiple threads can run in a Python program, only one thread can execute Python bytecode at a time in a process, limiting true concurrency in CPU-heavy operations.
What types of tasks benefit from multi-threading in Python?
-Multi-threading is most beneficial for I/O-bound tasks, where the program waits for external data (e.g., file I/O, network communication). In these cases, Python releases the GIL during blocking I/O operations, allowing other threads to execute.
Why is multi-processing recommended for CPU-bound tasks in Python?
-Multi-processing is recommended for CPU-bound tasks because each process has its own memory space and can run in parallel, bypassing the GIL. This allows Python to utilize multiple CPU cores effectively, improving performance in tasks that require intensive computation.
What are the key challenges when using multi-threading in Python?
-One key challenge is the need for synchronization, as threads share memory and can interfere with each other when accessing or modifying data. This requires mechanisms like locks to ensure data integrity, and improper handling can lead to issues like race conditions.
How do locks work in multi-threading, and why are they necessary?
-Locks ensure that only one thread can access shared data at a time. Without locks, threads could interrupt each other while accessing or modifying data, potentially leading to inconsistent or corrupted data. Locks prevent such interruptions by making threads wait their turn to access the shared resource.
What is the difference between queues and pipes when sharing data between processes?
-Queues are thread- and process-safe, meaning they can be accessed simultaneously by multiple threads or processes without causing data corruption. Pipes, on the other hand, are simpler and more efficient but are not as safe for simultaneous access by multiple threads or processes.
Why are queues more costly to set up than pipes?
-Queues are more costly because they involve additional overhead to ensure thread and process safety. They include mechanisms to manage concurrent access, whereas pipes are simpler and involve less complexity, making them more lightweight but less safe for shared access.
How do libraries like NumPy, SciPy, and TensorFlow handle multi-threading?
-Libraries like NumPy, SciPy, and TensorFlow implement multi-threading behind the scenes. They use multi-threaded operations internally to optimize performance for numerical computations and machine learning tasks, allowing for concurrency without needing the user to manage threads manually.
What should you consider when deciding whether to use multi-threading or multi-processing in Python?
-You should consider the nature of your tasks. For I/O-bound tasks, multi-threading is usually more efficient. For CPU-bound tasks, multi-processing is better, as it allows for parallel execution on multiple cores. Additionally, the overhead of managing threads or processes should be evaluated to determine if the performance benefits justify the complexity.
Outlines
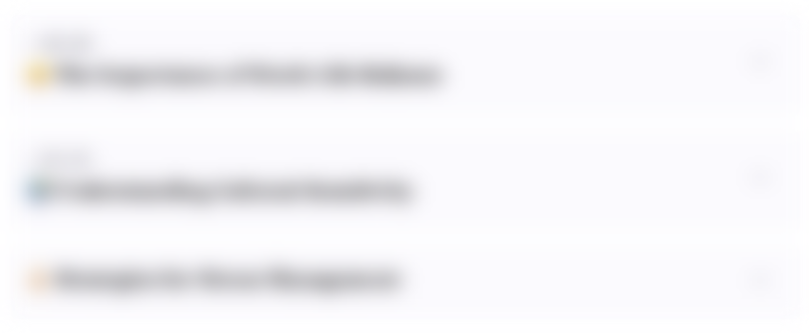
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
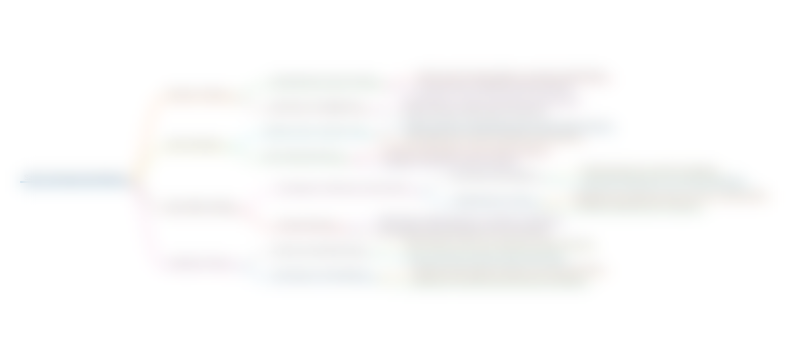
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
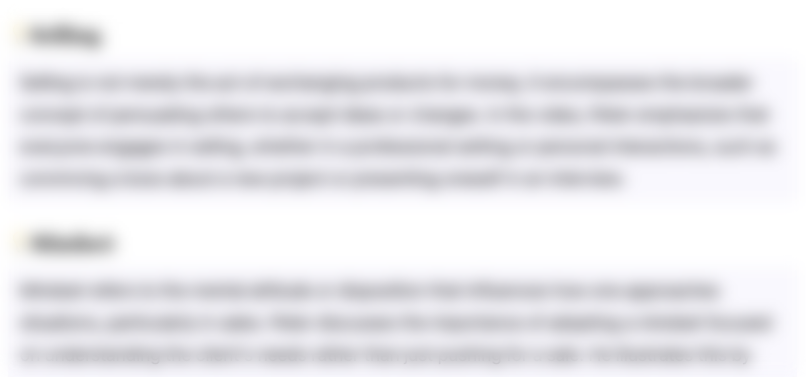
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
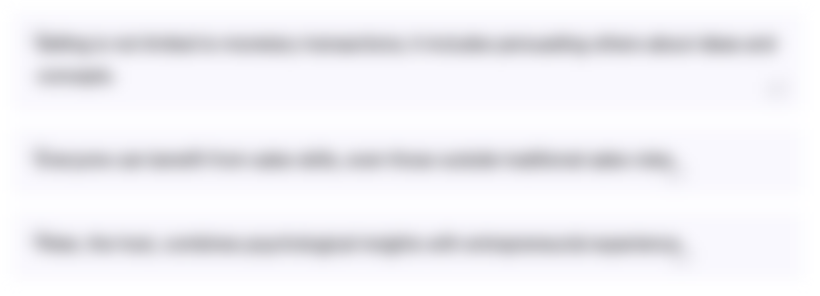
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
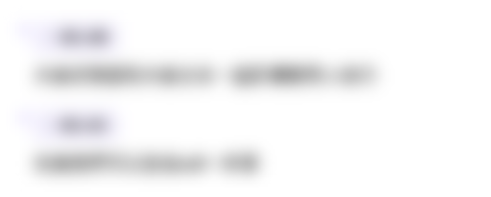
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)