C Programming Tutorial - 9 - I Need Arrays
Summary
TLDRIn this educational video, Bucky Roberts explains the concept and utility of arrays in programming, emphasizing their ability to store and manipulate lists of items. He demonstrates how to access individual elements, assign new values, and the importance of memory allocation. The video also covers string terminators and the use of the 'strcpy' function for array manipulation, concluding with an invitation to engage in a forum for further questions and support.
Takeaways
- π Arrays are used in programming to store a list of items, offering more functionality than a simple string type.
- π To access an individual item in an array, you use the array's name followed by the position of the element in square brackets, starting with index 0.
- π You can assign values to an array element by specifying the array name and the index, then setting it equal to a new value, such as changing 'C' to 'Z'.
- πΎ When defining an array, you need to specify the amount of memory it will hold, as the program allocates space for it immediately.
- π If you define and assign a value to an array in the same line, you don't need to specify the size because the compiler counts the characters automatically.
- π½ The script uses a 'food' array as an example to demonstrate assigning and changing values within an array.
- π The 'Strcpy' function is used to assign a new string to an array, requiring the array name and the new string as arguments.
- π οΈ Changing the value of an array with 'Strcpy' updates the content, as shown by changing 'tuna' to 'bacon' in the 'food' array.
- π The script emphasizes the importance of understanding string terminators and memory allocation when working with arrays.
- π€ The video aims to clarify confusion around arrays and offers a forum for further questions and support.
Q & A
Why are arrays used instead of simple string types in programming?
-Arrays are used because they allow for the storage and manipulation of multiple items, enabling programmers to perform operations on lists of data, which is not possible with a simple string type that can only store a single piece of data.
How do you access an individual item in an array?
-You access an individual item in an array by using the array's name followed by the position of the element in square brackets, starting with index 0 for the first element.
Why does array indexing start at zero?
-Array indexing starts at zero because it is a convention in many programming languages, including C, which allows for easier calculations and indexing within the language's implementation.
How can you change the value of an individual element in an array?
-You can change the value of an individual element by specifying the array name, the index of the element in square brackets, and then assigning it a new value.
What is the purpose of the 'Strcpy' function mentioned in the script?
-The 'Strcpy' function is used to copy a string into an array. It requires two parameters: the array you want to change and the new string you want to store in the array.
Why is it called 'Strcpy' and not just 'copy'?
-'Strcpy' is likely a shorthand for 'string copy', indicating that the function is specifically designed for copying strings into arrays or similar data structures.
What is the significance of the string terminator in the context of the script?
-The string terminator, usually a null character, is significant because it signals the end of a string in memory. Understanding string terminators helps in managing memory allocation and ensures that strings are correctly handled in arrays.
How does defining an array and giving it a value later differ from defining a variable?
-When defining an array and giving it a value later, you need to explicitly state the size of the array to allocate memory. However, when defining an array and assigning a value in the same line, the compiler can automatically calculate the required memory based on the string length.
What is the advantage of using arrays over simple variables when storing multiple items?
-Arrays provide the advantage of storing multiple items in a single data structure, allowing for more efficient memory usage and easier manipulation of collections of data compared to using separate variables for each item.
Why is it necessary to define the size of an array when setting it apart from a variable?
-Defining the size of an array when setting it apart from a variable is necessary because the program needs to allocate a specific amount of memory to store the array's elements. This allocation must be determined before the program can use the array.
What is the speaker's name, and what is his approach to helping people with programming questions?
-The speaker's name is Bucky Roberts. He offers to answer questions on his forum, where he or other knowledgeable people can provide help and support to those learning about programming concepts.
Outlines
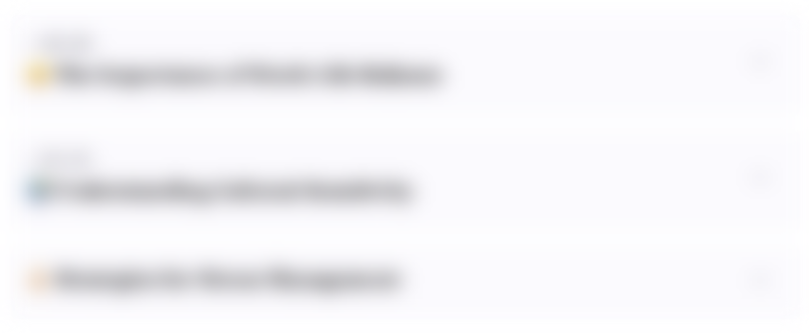
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
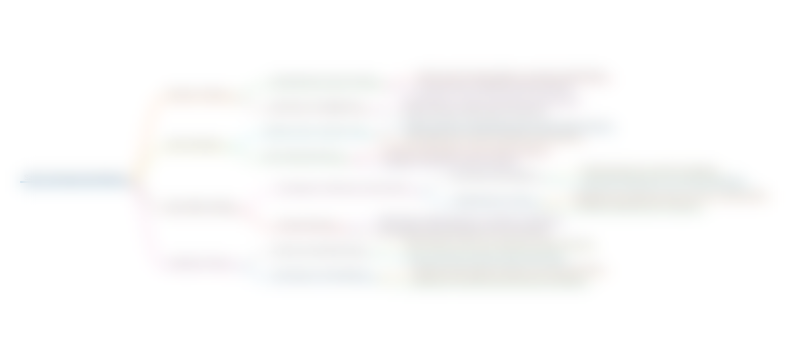
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
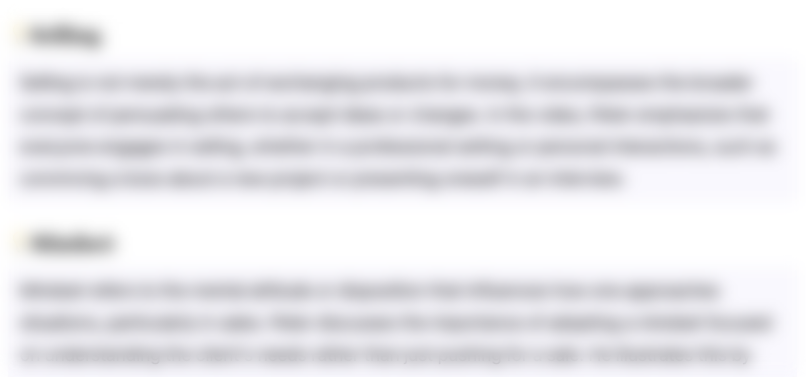
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
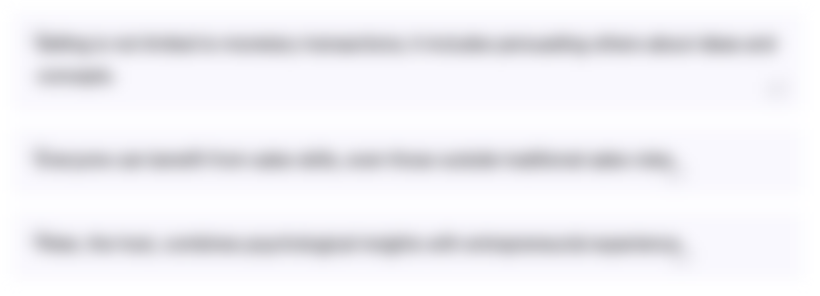
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
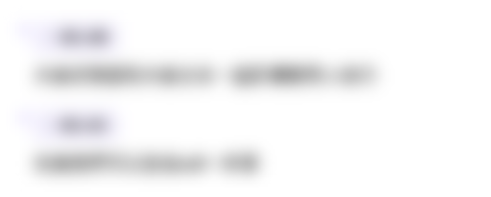
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)