The useEffect Cleanup Function | Lecture 151 | React.JS π₯
Summary
TLDRThe video script discusses the concept of cleanup functions in React's useEffect hook. It explains how cleanup functions are used to synchronize the page title with the application state, ensuring that side effects are managed correctly when a component unmounts or re-renders. The importance of returning a cleanup function from an effect is highlighted, as it helps prevent race conditions and other bugs by allowing for the cancellation of ongoing requests, stopping timers, and removing event listeners. The script also emphasizes the best practice of using multiple useEffect hooks for multiple effects within a component, making each effect more manageable and the cleanup process more straightforward.
Takeaways
- π§Ή The cleanup function is a crucial part of an effect, ensuring that side effects are properly managed.
- β° After an effect runs, the cleanup function is executed to revert any changes made by the side effect.
- π Cleanup functions are also called on re-renders, just before the next effect is executed.
- π When a component unmounts, the cleanup function runs to reset any side effects created by the component.
- π The dependency array is used to run code when a component mounts or re-renders.
- π« You don't always need to return a cleanup function from an effect; it's optional.
- π οΈ Cleanup functions are essential when side effects continue after a component has been re-rendered or unmounted.
- π§ To prevent race conditions, it's a good practice to cancel ongoing HTTP requests in the cleanup function.
- π Subscriptions to API services should be canceled in the cleanup function to avoid memory leaks.
- β±οΈ Timers started in an effect should be stopped in the cleanup function to prevent them from running indefinitely.
- π Event listeners added in an effect should be removed in the cleanup function to prevent potential memory leaks.
- π Each effect should only handle one task. For multiple effects, use multiple useEffect hooks for better organization and cleanup.
Q & A
What is the purpose of a cleanup function in a component's effect?
-The cleanup function is used to execute code when a component unmounts or before the effect is executed again, allowing for the reset or cancellation of side effects to prevent issues like race conditions.
Why is it important to keep the page title synchronized with the application state?
-Keeping the page title synchronized ensures a consistent user experience, reflecting the current state of the application and avoiding confusion when components are mounted or unmounted.
When does the cleanup function run in relation to the component's lifecycle?
-The cleanup function runs after the component instance has unmounted and before the effect is executed again, which can help in cleaning up or resetting side effects.
What is a race condition in the context of component effects?
-A race condition occurs when a component is re-rendered while an asynchronous operation like an HTTP request is still in progress, potentially leading to unexpected behavior or bugs.
How can you prevent race conditions in component effects?
-By using cleanup functions to cancel ongoing requests or subscriptions whenever the component re-renders or unmounts, thus ensuring that only one request is active at a time.
What should you do when you subscribe to an API service in a component effect?
-You should cancel the subscription in the cleanup function to prevent memory leaks and ensure that the API service is not used after the component has unmounted.
Why is it recommended to use multiple useEffect hooks for multiple effects in a component?
-Using multiple useEffect hooks makes each effect easier to understand and manage, and it simplifies the cleanup process, as each effect can have its own dedicated cleanup function.
What is the rule of thumb for structuring effects in a component?
-Each effect should only do one thing. This approach promotes clarity, maintainability, and easier debugging.
How does the dependency array in useEffect relate to the cleanup function?
-The dependency array determines when the effect runs, while the cleanup function is responsible for cleaning up after the effect. Both work together to manage the lifecycle of a component's side effects.
What should you do when you start a timer in a component effect?
-You should stop the timer in the cleanup function to prevent the timer from running indefinitely after the component has unmounted.
Why is it necessary to remove event listeners in the cleanup function?
-Removing event listeners in the cleanup function prevents potential memory leaks and ensures that the component does not continue to respond to events after it has been unmounted.
Outlines
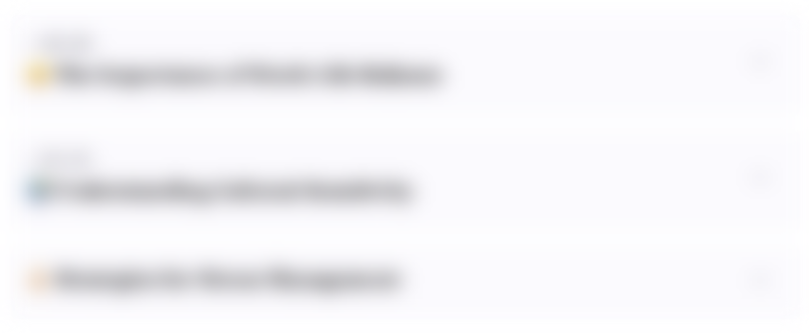
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
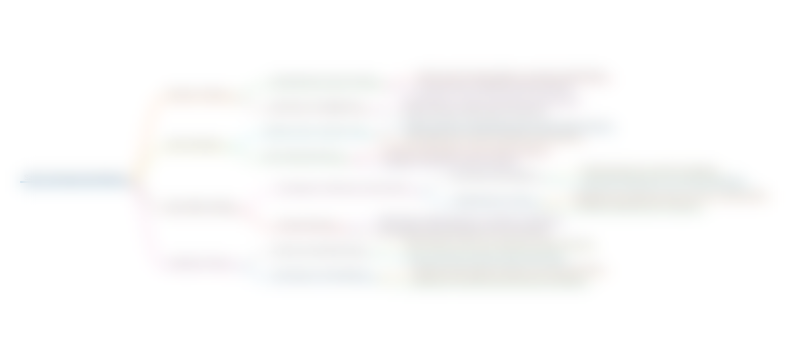
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
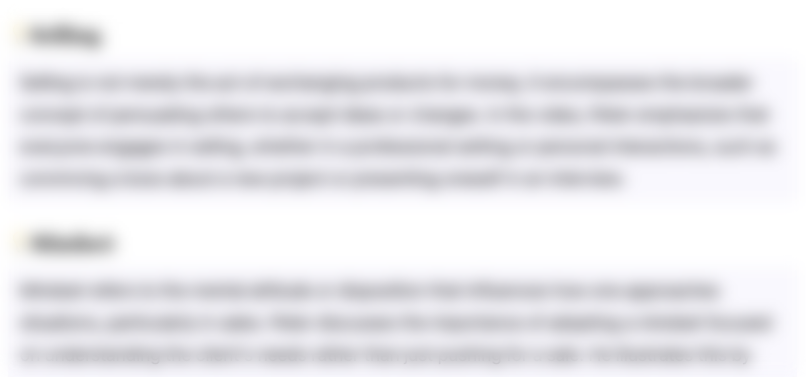
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
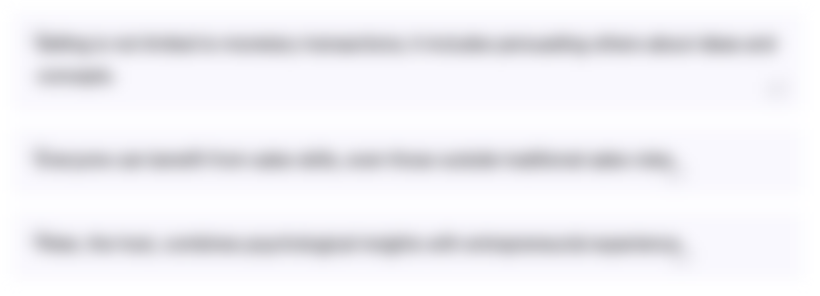
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
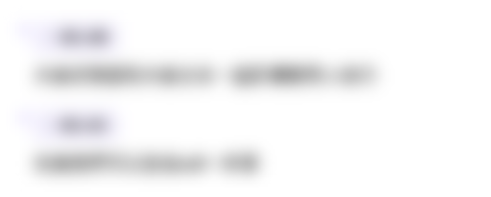
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

A First Look at Effects | Lecture 141 | React.JS π₯

useEffect to the Rescue | Lecture 140 | React.JS π₯

The useEffect Dependency Array | Lecture 145 | React.JS π₯
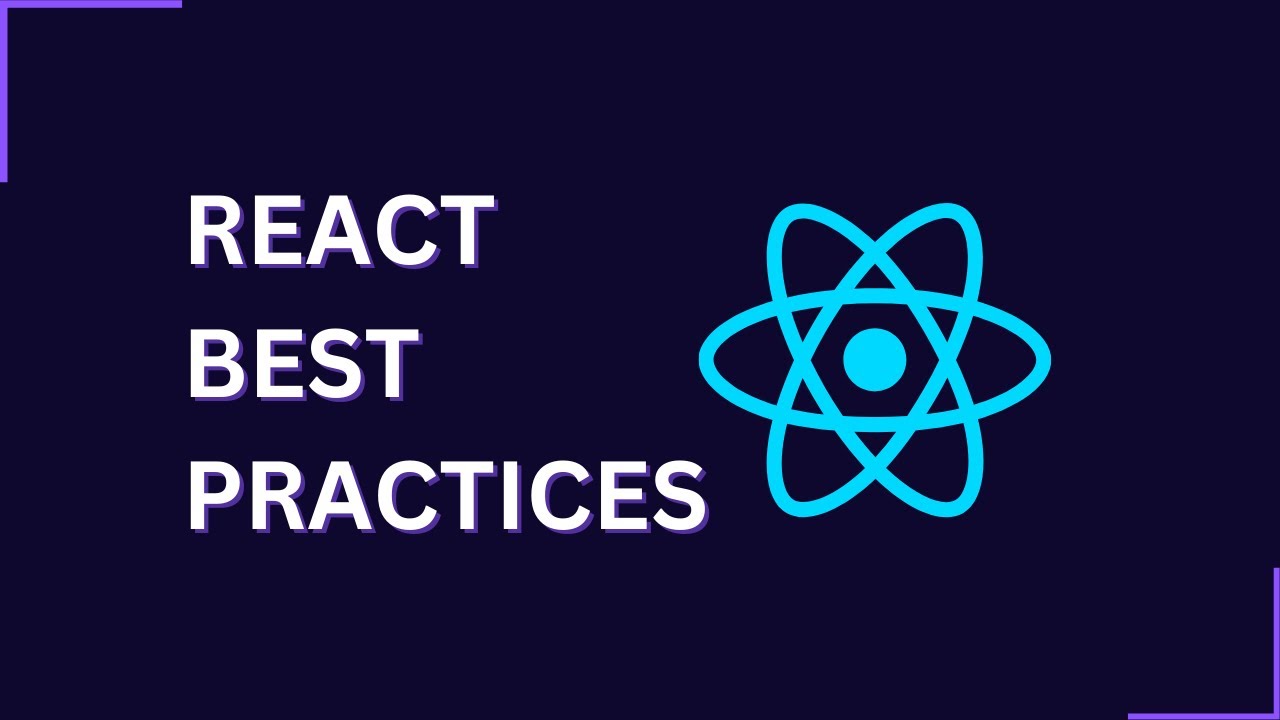
React Like a Pro: React Best Practices

Rules for Render Logic: Pure Components | Lecture 131 | React.JS π₯

How NOT to Fetch Data in React | Lecture 139 | React.JS π₯
5.0 / 5 (0 votes)